In this video it shows the fix for the error “Value of type org.json.JSONArray cannot be converted to JSONObject”.
It reproduces this error by trying to convert a JSONArray to JSONObject. And then fixes it by rightly converting it into JSONArray and then using the index to get the right string from this JSON Array.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.jsonarraytojsonobjecterror;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
public class MainActivity extends AppCompatActivity {
private String stringJson = "[{\n" +
" \"quiz\": {\n" +
" \"sport\": {\n" +
" \"q1\": {\n" +
" \"question\": \"Which one is correct team name in NBA?\",\n" +
" \"options\": [\n" +
" \"New York Bulls\",\n" +
" \"Los Angeles Kings\",\n" +
" \"Golden State Warriros\",\n" +
" \"Huston Rocket\"\n" +
" ],\n" +
" \"answer\": \"Huston Rocket\"\n" +
" }\n" +
" },\n" +
" \"maths\": {\n" +
" \"q1\": {\n" +
" \"question\": \"5 + 7 = ?\",\n" +
" \"options\": [\n" +
" \"10\",\n" +
" \"11\",\n" +
" \"12\",\n" +
" \"13\"\n" +
" ],\n" +
" \"answer\": \"12\"\n" +
" },\n" +
" \"q2\": {\n" +
" \"question\": \"12 - 8 = ?\",\n" +
" \"options\": [\n" +
" \"1\",\n" +
" \"2\",\n" +
" \"3\",\n" +
" \"4\"\n" +
" ],\n" +
" \"answer\": \"4\"\n" +
" }\n" +
" }\n" +
" }\n" +
"}]";
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
}
public void buttonReadJson(View view){
try {
// JSONObject jsonObject = new JSONObject(stringJson); // Gives error - "Value of type org.json.JSONArray cannot be converted to JSONObject"
JSONArray jsonArray = new JSONArray(stringJson);
textView.setText(jsonArray.getString(0));
} catch (Exception e) {
textView.setText(e.toString());
}
}
}
<?xml version=”1.0″ encoding=”utf-8″?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android=”http://schemas.android.com/apk/res/android”
xmlns:app=”http://schemas.android.com/apk/res-auto”
xmlns:tools=”http://schemas.android.com/tools”
android:layout_width=”match_parent”
android:layout_height=”match_parent”
tools:context=”.MainActivity”>
<TextView
android:id=”@+id/textView”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
android:text=”Hello World!”
app:layout_constraintBottom_toBottomOf=”parent”
app:layout_constraintEnd_toEndOf=”parent”
app:layout_constraintHorizontal_bias=”0.464″
app:layout_constraintStart_toStartOf=”parent”
app:layout_constraintTop_toTopOf=”parent”
app:layout_constraintVertical_bias=”0.217″ />
<Button
android:id=”@+id/button”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
android:layout_marginStart=”139dp”
android:layout_marginTop=”28dp”
android:onClick=”buttonReadJson”
android:text=”Read Json”
app:layout_constraintStart_toStartOf=”parent”
app:layout_constraintTop_toTopOf=”parent” />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
Layout start:
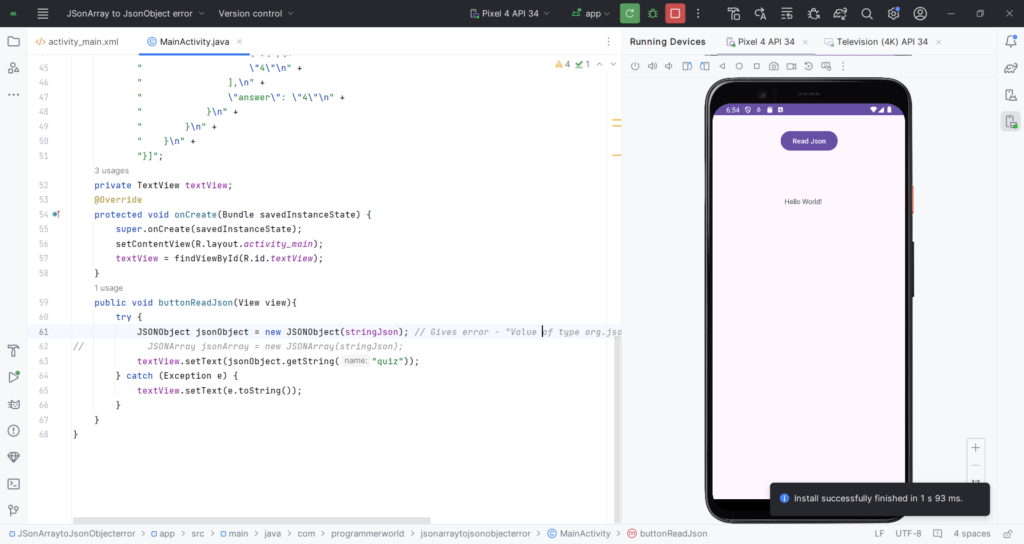
Error:
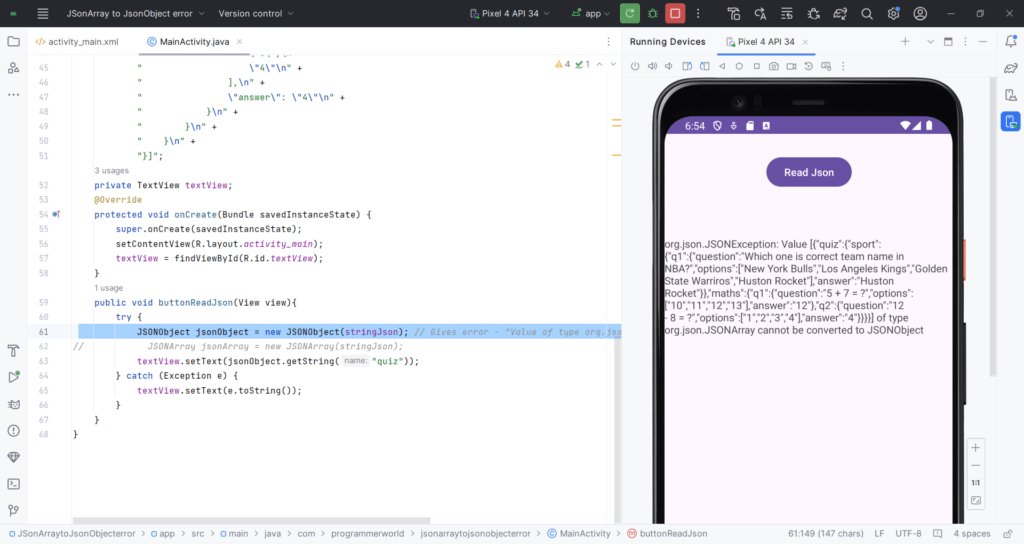
Corrected version
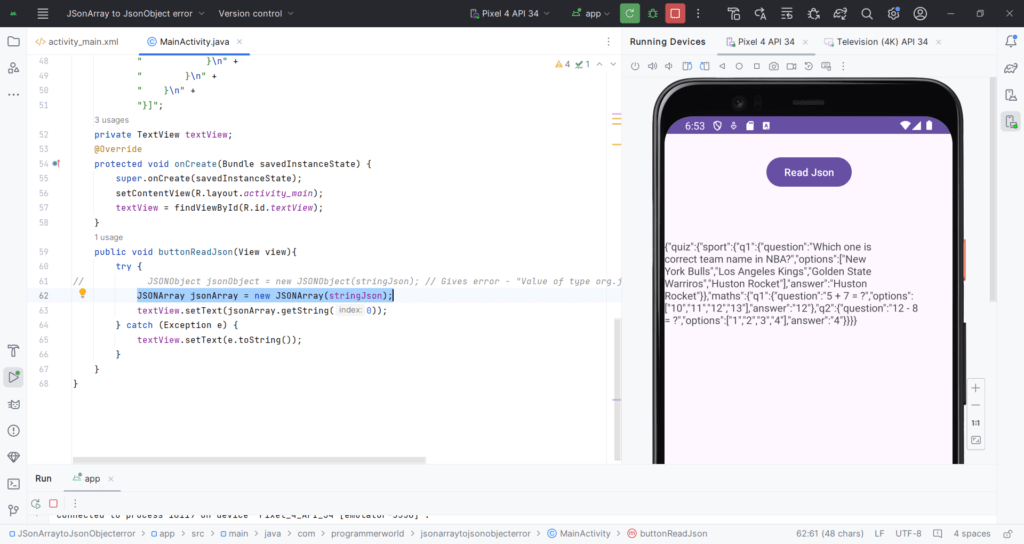
Sample JSON Array used in thei demo:
[
{
"quiz": {
"sport": {
"q1": {
"question": "Which one is correct team name in NBA?",
"options": [
"New York Bulls",
"Los Angeles Kings",
"Golden State Warriros",
"Huston Rocket"
],
"answer": "Huston Rocket"
}
},
"maths": {
"q1": {
"question": "5 + 7 = ?",
"options": [
"10",
"11",
"12",
"13"
],
"answer": "12"
},
"q2": {
"question": "12 - 8 = ?",
"options": [
"1",
"2",
"3",
"4"
],
"answer": "4"
}
}
}
}
]