This video shows the code to get the Android’s OS (operating system) version in your Android App.
The version history of Android OS (version number, codename, API level) is mentioned in the below wiki page: https://en.wikipedia.org/wiki/Android_version_history
Below is the recent released versions of Android and used in the code in this tutorial.
10 -> Quince Tart
11 -> Red Velvet Cake
12 -> Snow Cone
13 -> Tiramisu
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.androidversionapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
}
public void buttonAndroidVersion(View view){
String stringAndroidVersion = Build.VERSION.RELEASE;
switch (stringAndroidVersion){
case "13":
stringAndroidVersion = stringAndroidVersion + " -> Tiramisu";
break;
case "12":
stringAndroidVersion = stringAndroidVersion + " -> Snow Cone";
break;
case "11":
stringAndroidVersion = stringAndroidVersion + " -> Red Velvet Cake";
break;
case "10":
stringAndroidVersion = stringAndroidVersion + " -> Quince Tart";
break;
}
textView.setText(stringAndroidVersion);
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="268dp"
android:layout_height="166dp"
android:text="Hello World!"
android:textAlignment="center"
android:textSize="20sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.47"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.38" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="102dp"
android:layout_marginTop="114dp"
android:onClick="buttonAndroidVersion"
android:text="Get Android Version"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
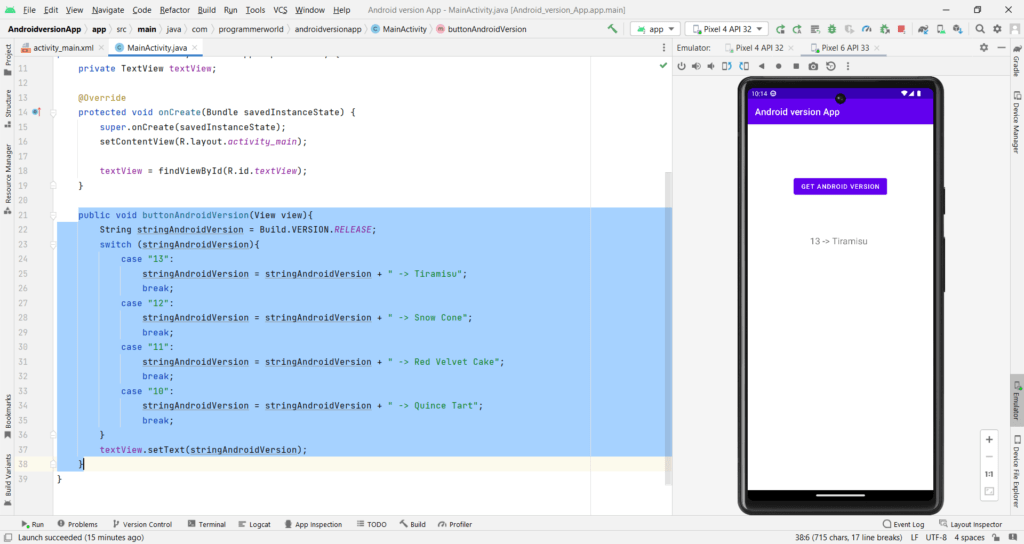
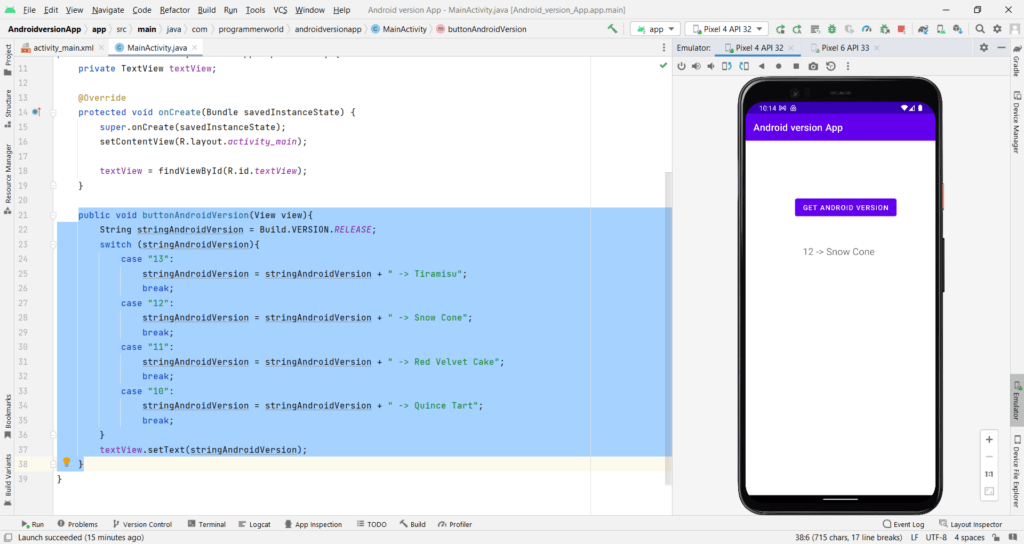