This video shows the steps to develop your own video recorder Android app and save the video (.mp4) file in Download folder of the device.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.videorecorderapp;
import static android.Manifest.permission.CAMERA;
import static android.Manifest.permission.READ_MEDIA_AUDIO;
import static android.Manifest.permission.READ_MEDIA_IMAGES;
import static android.Manifest.permission.READ_MEDIA_VIDEO;
import static android.Manifest.permission.RECORD_AUDIO;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.content.pm.PackageManager;
import android.media.MediaRecorder;
import android.os.Bundle;
import android.os.storage.StorageManager;
import android.os.storage.StorageVolume;
import android.view.SurfaceView;
import android.view.View;
import android.widget.Toast;
import java.io.File;
import java.io.IOException;
public class MainActivity extends AppCompatActivity {
private SurfaceView surfaceView;
private MediaRecorder mediaRecorder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{CAMERA,
READ_MEDIA_VIDEO,
READ_MEDIA_IMAGES,
READ_MEDIA_AUDIO,
RECORD_AUDIO},
PackageManager.PERMISSION_GRANTED);
surfaceView = findViewById(R.id.surfaceView);
mediaRecorder = new MediaRecorder(this);
}
public void buttonStartVideoRecording(View view){
StorageManager storageManager = (StorageManager) getSystemService(STORAGE_SERVICE);
StorageVolume storageVolume = storageManager.getStorageVolumes().get(0); // 0 for internal storage
File fileVideo = new File(storageVolume.getDirectory().getPath() +
"/Download/" +
System.currentTimeMillis()+".mp4");
mediaRecorder.setVideoSource(MediaRecorder.VideoSource.CAMERA);
mediaRecorder.setAudioSource(MediaRecorder.AudioSource.MIC);
mediaRecorder.setOutputFormat(MediaRecorder.OutputFormat.MPEG_4);
mediaRecorder.setVideoEncoder(MediaRecorder.VideoEncoder.DEFAULT);
mediaRecorder.setAudioEncoder(MediaRecorder.AudioEncoder.DEFAULT);
mediaRecorder.setOutputFile(fileVideo.getPath());
mediaRecorder.setMaxDuration(5000); // 5 seconds of max recording
mediaRecorder.setMaxFileSize(5000000); // Max 5 MB
mediaRecorder.setPreviewDisplay(surfaceView.getHolder().getSurface());
try {
mediaRecorder.prepare();
} catch (IOException e) {
Toast.makeText(this, e.toString(), Toast.LENGTH_LONG).show();
}
mediaRecorder.start();
}
public void buttonStopVideoRecording(View view){
mediaRecorder.stop();
mediaRecorder.release();
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-feature
android:name="android.hardware.camera"
android:required="false" />
<uses-permission android:name="android.permission.READ_MEDIA_VIDEO"/>
<uses-permission android:name="android.permission.READ_MEDIA_IMAGES"/>
<uses-permission android:name="android.permission.READ_MEDIA_AUDIO"/>
<uses-permission android:name="android.permission.CAMERA"/>
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.RECORD_AUDIO"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.VideoRecorderApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="42dp"
android:onClick="buttonStartVideoRecording"
android:text="Start Video Recording"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:layout_marginTop="42dp"
android:onClick="buttonStopVideoRecording"
android:text="Stop Video Recording"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="parent" />
<SurfaceView
android:id="@+id/surfaceView"
android:layout_width="376dp"
android:layout_height="491dp"
android:layout_marginStart="16dp"
android:layout_marginTop="50dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
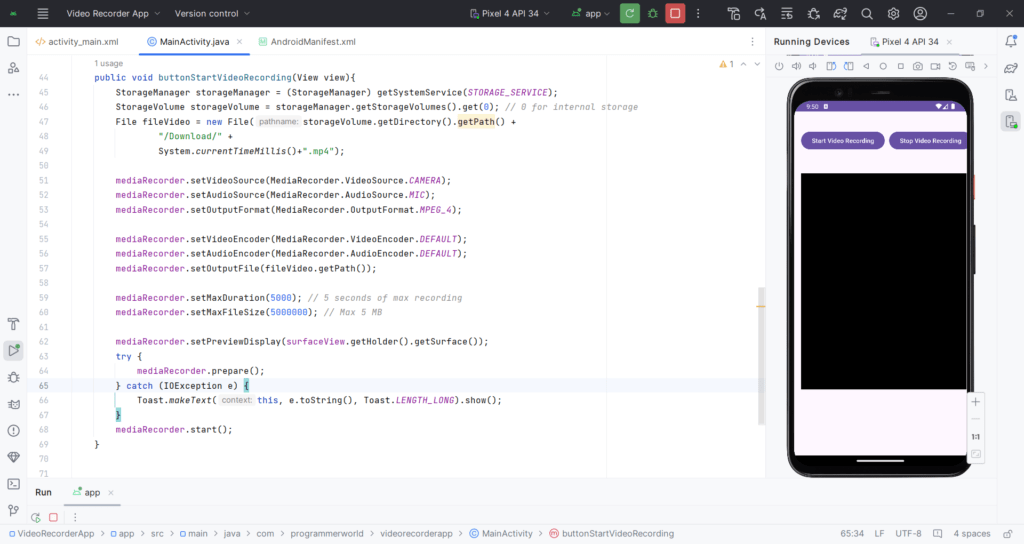
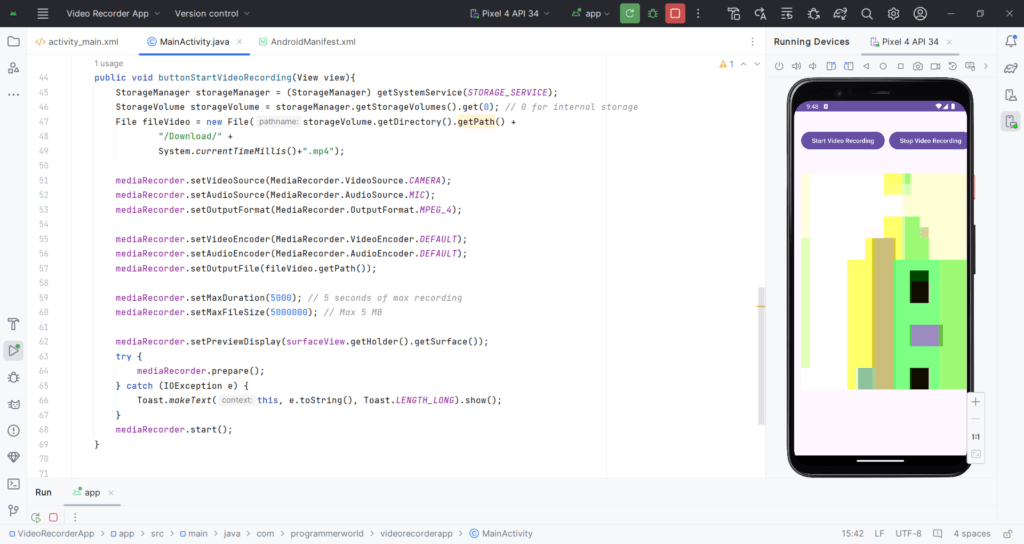
Recorded Video:
In Emulator:
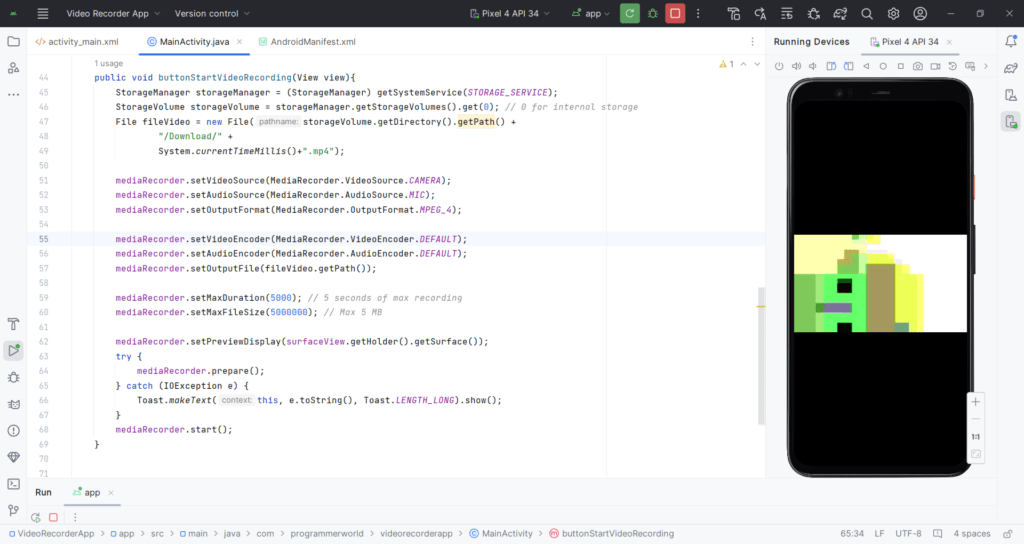
In Actual Device:
Project Folder:
Complete project folder can be accessed from the below link on Payment of USD 9.
https://drive.google.com/file/d/18QopihFEQnFsBODhCiwnLpq4VyCK1Lme/view?usp=drive_link
Excerpt:
This video tutorial demonstrates the process of creating an Android app for video recording and saving the video file in the device’s Download folder. It includes the necessary code and permission requirements. The app utilizes the device’s camera and microphone and provides options to start and stop video recording. The accompanying manifest and layout files are also provided. The complete project folder can be obtained by purchasing it for $10. After payment confirmation, access can be requested by contacting the developer. For further details, the developer can be reached at programmerworld1990@gmail.com or through https://programmerworld.co/contact/.