In this video it shows the steps to develop a fingerprint authentication Android App in Android Studio.
It creates a simple layout to trigger the authentication. And shows the success or failure of authentication using a text view and image view.
We will be glad to hear about any suggestions, queries or appreciations at: programmerworld1990@gmail.com
Images to show the fingerprints shown in this tutorial are placed below and can be downloaded to be used in your App development:
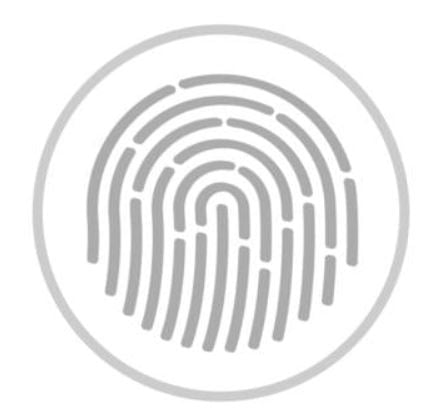
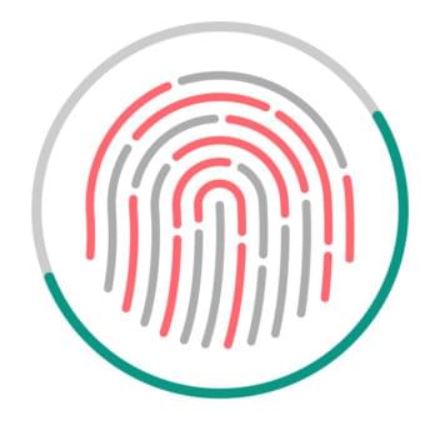
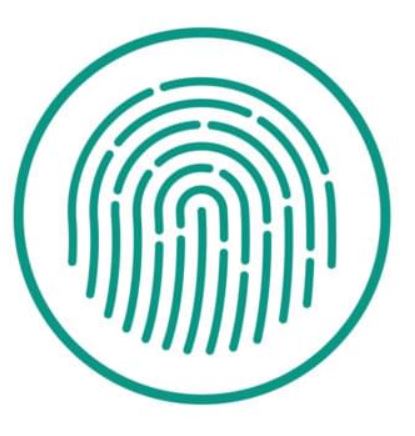
The results of the App tested on the real phone is shown below:
Complete Source Code:
Source code:
package com.example.myfingerprintauthenticationapp;
import android.hardware.fingerprint.FingerprintManager;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private TextView textView;
private ImageView imageView;
private FingerprintManager fingerprintManager;
private FingerprintManager.AuthenticationCallback authenticationCallback;@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);textView = findViewById(R.id.textView);
imageView = findViewById(R.id.imageView);
imageView.setImageResource(R.drawable.image1);
fingerprintManager = (FingerprintManager) getSystemService(FINGERPRINT_SERVICE);authenticationCallback = new FingerprintManager.AuthenticationCallback() {
@Override
public void onAuthenticationError(int errorCode, CharSequence errString) {
textView.setText(“ERROR”);
imageView.setImageResource(R.drawable.image2);
super.onAuthenticationError(errorCode, errString);
}@Override
public void onAuthenticationHelp(int helpCode, CharSequence helpString) {
textView.setText(“HELP”);
imageView.setImageResource(R.drawable.image1);
super.onAuthenticationHelp(helpCode, helpString);
}@Override
public void onAuthenticationSucceeded(FingerprintManager.AuthenticationResult result) {
textView.setText(“SUCCESS”);
imageView.setImageResource(R.drawable.image3);
super.onAuthenticationSucceeded(result);
}@Override
public void onAuthenticationFailed() {
textView.setText(“FAILED”);
imageView.setImageResource(R.drawable.image2);
super.onAuthenticationFailed();
}
};
}public void scanButton(View view){
fingerprintManager.authenticate(null, null, 0, authenticationCallback, null);
}
}
Manifest File:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.myfingerprintauthenticationapp"> <uses-permission android:name="android.permission.USE_FINGERPRINT" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Gradle File:
apply plugin: 'com.android.application' android { compileSdkVersion 29 buildToolsVersion "29.0.2" defaultConfig { applicationId "com.example.myfingerprintauthenticationapp" minSdkVersion 26 targetSdkVersion 29 versionCode 1 versionName "1.0" testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'androidx.appcompat:appcompat:1.1.0' implementation 'androidx.constraintlayout:constraintlayout:1.1.3' testImplementation 'junit:junit:4.12' androidTestImplementation 'androidx.test.ext:junit:1.1.1' androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0' }
Resource File:
<resources> <string name="app_name">My FingerPrintAuthentication App</string> <string name="result_display">Result Display ...</string> <string name="scan">Scan</string> <string name="todo">TODO</string> </resources>
Layout XML File:
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/textView" android:layout_width="212dp" android:layout_height="90dp" android:text="@string/result_display" android:textAlignment="center" android:textSize="24sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.289" /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="158dp" android:layout_marginTop="64dp" android:onClick="scanButton" android:text="@string/scan" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <ImageView android:id="@+id/imageView" android:layout_width="217dp" android:layout_height="266dp" android:layout_marginStart="95dp" android:layout_marginTop="31dp" android:contentDescription="@string/todo" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView" tools:srcCompat="@drawable/image1" /> </androidx.constraintlayout.widget.ConstraintLayout>
How do i implement fingerprint funtionality on webview
For webview, this functionality has to be converted into a http page which opens up in a browser. In that case, it is the browser App which will check the fingerprint authentication on behalf of your http webpage.
So, in your website design you have to insert that functionality of getting fingerprint authentication from browser application.
In this tutorial, I have focussed on showing the biometric authentication for an Android App. Creating a webpage is very different and is not covered in my blog pages.
Hope the above explanation helps.
Cheers
Programmer World
–
Bro is it posiible that i press a button and it asks for fingerprint authentication and takes us to new activity
Yes, that is definitely possible. In this tutorial it shows how the authentication is done once the ‘Scan’ button is pressed.
If you want to start or navigate to a new activity once the authentication is successful, then you can insert the StartActivity code in the onAuthenticationSucceeded method of FingerprintManager.
I hope above helps.
For more tutorials on Android, you can refer the list mentioned in the below link:
https://programmerworld.co/android/
Good Luck
Programmer World
https://www.youtube.com/c/programmerworld
new FingerprintManager.AuthenticationCallback() deprecated please update code
Yes, you are right. FingerprintManager is deprecated.
However, as shown in the video also, I have made this App in SDK 26. That’s why I used FingerprintManager. But anyone going to develop it for later versions of SDK should use the newer API – BiometricManager. As told in the video also, the syntax remains same between the two commands, so, one should not face issues in adapting the code to either of the API after watching this tutorial.
The reason for choosing older SDK (26) is because many of my viewers still does programming for older phones (older Android OS), so, I have to ensure that the Application covers larger number of phones and not only 1% of phones if I choose latest SDKs.
Cheers
Programmer World
https://programmerworld.co
–
Nice article.
How do I make the biometric prompt pop up as soon as I launch the app. I want the biometric app to pop up when i open the app and if i click on cancel button on the biometric prompt, i want to return to the usual login page with user id / password. Could you give me insights into how this can be achieved?
It’s simple. You will have to create a separate layout for fingerprint scanning.
Then call/set this layout in your onCreate method from the setContentView API call.
In the cancel button’s (of fingerprint scanning layout) onClick method set it to go to your login (with user ID/password) layout and proceed.
Good Luck
Programmer World
Hi,
Can you please share refs.xml file code please, By mistake i have deleted that code now it is howing me error while exicuting .
Whatever code of this tutorial was there has already been pasted in the above webpage. I don’t think I have the source code files anymore. However, if you can elaborate a bit on what you are exactly looking for then probably we can try to check.
Cheers
Programmer World
–
I want to store the finger print of the users in database for letter to use for viewing.
Storing an information in database is not a problem. There are several videos on my website which shows this:
https://programmerworld.co/android/
However, the bigger challenge will be to get the fingerprint information in some data format which can be stored in the database. The default fingerprint scanner provided in the phone will not let the users to get the fingerprint data extracted in any format (due to security reasons). So, only option will be to use an external fingerprint scanner device which let’s the users to export the information in a particular data type. And then save that data in the database.
Cheers
Programmer World
–
I would want my app to detect change in the initially enrolled fingerprint and disable the app
I would want my app to detect change in the initially enrolled fingerprint and disable the app