In this video it shows how to save the edit text information, such as Bold, Italics, underline or Left/ right/ center alignmnent (justification) in a SQLite database.
Part 1 of this tutorial is available in the below link:
https://programmerworld.co/android/how-to-create-your-own-text-editor-android-app-for-making-text-bold-italics-underline-alignment/
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code:
package com.programmerworld.saveedittextsqlitedatabase;
import android.Manifest;
import android.content.ContentValues;
import android.content.pm.PackageManager;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.graphics.Typeface;
import android.os.Bundle;
import android.text.Spannable;
import android.text.SpannableStringBuilder;
import android.text.style.StyleSpan;
import android.text.style.UnderlineSpan;
import android.view.View;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
public class MainActivity extends AppCompatActivity {
private EditText editText;
private mySqliteDBHandler sqliteDBHandler;
private SQLiteDatabase sqLiteDatabaseAttributes;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editTextTextMultiLine);
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE}, PackageManager.PERMISSION_GRANTED);
try {
sqliteDBHandler = new mySqliteDBHandler(this, "EditTextDatabase", null,1);
sqLiteDatabaseAttributes = sqliteDBHandler.getWritableDatabase();
sqLiteDatabaseAttributes.execSQL("CREATE TABLE EditedTextAttributes(EditStyle TEXT," +
"SelectionStart INTEGER, SelectionEnd INTEGER)");
}catch (Exception e){
e.printStackTrace();
}
sqLiteDatabaseAttributes.delete("EditedTextAttributes", null, null);
}
public void buttonFetch(View view){
String stringSQLQuery = "Select * from EditedTextAttributes";
Cursor cursor = sqLiteDatabaseAttributes.rawQuery(stringSQLQuery, null);
try {
cursor.moveToFirst();
Spannable spannableString = new SpannableStringBuilder(editText.getText());
while (!cursor.isNull(0)){
int intSelectionStart = cursor.getInt(1);
int intSelectionEnd = cursor.getInt(2);
switch(cursor.getString(0)){
case "BOLD":
spannableString.setSpan(new StyleSpan(Typeface.BOLD),
intSelectionStart, intSelectionEnd,
0);
editText.setText(spannableString);
break;
case "CENTER":
editText.setTextAlignment(View.TEXT_ALIGNMENT_CENTER);
editText.setText(spannableString);
break;
case "RIGHT":
editText.setTextAlignment(View.TEXT_ALIGNMENT_TEXT_END);
editText.setText(spannableString);
break;
case "LEFT":
editText.setTextAlignment(View.TEXT_ALIGNMENT_TEXT_START);
editText.setText(spannableString);
break;
case "ITALIC":
spannableString.setSpan(new StyleSpan(Typeface.ITALIC),
intSelectionStart, intSelectionEnd,
0);
editText.setText(spannableString);
break;
case "UNDERLINE":
spannableString.setSpan(new UnderlineSpan(),
intSelectionStart, intSelectionEnd,
0);
editText.setText(spannableString);
break;
}
cursor.moveToNext();
}
cursor.close();
}
catch (Exception e){
e.printStackTrace();
}
}
private void InsertAttributesDatabase(String stringStyleName, int intSelectionStart, int intSelectionEnd){
ContentValues contentValues = new ContentValues();
contentValues.put("EditStyle", stringStyleName);
contentValues.put("SelectionStart", intSelectionStart);
contentValues.put("SelectionEnd", intSelectionEnd);
sqLiteDatabaseAttributes.insert("EditedTextAttributes", null, contentValues);
}
public void buttonBold(View view){
Spannable spannableString = new SpannableStringBuilder(editText.getText());
spannableString.setSpan(new StyleSpan(Typeface.BOLD),
editText.getSelectionStart(),
editText.getSelectionEnd(),
0);
InsertAttributesDatabase("BOLD", editText.getSelectionStart(), editText.getSelectionEnd());
editText.setText(spannableString);
}
public void buttonItalics(View view){
Spannable spannableString = new SpannableStringBuilder(editText.getText());
spannableString.setSpan(new StyleSpan(Typeface.ITALIC),
editText.getSelectionStart(),
editText.getSelectionEnd(),
0);
InsertAttributesDatabase("ITALIC", editText.getSelectionStart(), editText.getSelectionEnd());
editText.setText(spannableString);
}
public void buttonUnderline(View view){
Spannable spannableString = new SpannableStringBuilder(editText.getText());
spannableString.setSpan(new UnderlineSpan(),
editText.getSelectionStart(),
editText.getSelectionEnd(),
0);
InsertAttributesDatabase("UNDERLINE", editText.getSelectionStart(), editText.getSelectionEnd());
editText.setText(spannableString);
}
public void buttonNoFormat(View view){
String stringText = editText.getText().toString();
editText.setTextAlignment(View.TEXT_ALIGNMENT_TEXT_START);
editText.setText(stringText);
}
public void buttonAlignmentLeft(View view){
editText.setTextAlignment(View.TEXT_ALIGNMENT_TEXT_START);
Spannable spannableString = new SpannableStringBuilder(editText.getText());
InsertAttributesDatabase("LEFT", 0, 0);
editText.setText(spannableString);
}
public void buttonAlignmentCenter(View view){
editText.setTextAlignment(View.TEXT_ALIGNMENT_CENTER);
Spannable spannableString = new SpannableStringBuilder(editText.getText());
InsertAttributesDatabase("CENTER", 0, 0);
editText.setText(spannableString);
}
public void buttonAlignmentRight(View view){
editText.setTextAlignment(View.TEXT_ALIGNMENT_TEXT_END);
Spannable spannableString = new SpannableStringBuilder(editText.getText());
InsertAttributesDatabase("RIGHT", 0, 0);
editText.setText(spannableString);
}}
package com.programmerworld.saveedittextsqlitedatabase;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import androidx.annotation.Nullable;
public class mySqliteDBHandler extends SQLiteOpenHelper {
public mySqliteDBHandler(@Nullable Context context, @Nullable String name, @Nullable SQLiteDatabase.CursorFactory factory, int version) {
super(context, name, factory, version);
}
@Override
public void onCreate(SQLiteDatabase sqLiteDatabase) {
}
@Override
public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) {
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.saveedittextsqlitedatabase">
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.SaveEditTextSqliteDatabase">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editTextTextMultiLine"
android:layout_width="320dp"
android:layout_height="217dp"
android:layout_marginStart="47dp"
android:layout_marginTop="7dp"
android:ems="10"
android:gravity="start|top"
android:hint="@string/type_text_here"
android:inputType="textMultiLine"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button4"
android:autofillHints="" />
<Button
android:id="@+id/button"
android:layout_width="88dp"
android:layout_height="55dp"
android:layout_marginStart="22dp"
android:layout_marginTop="8dp"
android:onClick="buttonBold"
android:text="@string/bold"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="42dp"
android:layout_marginTop="8dp"
android:onClick="buttonItalics"
android:text="@string/italics"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="46dp"
android:layout_marginTop="10dp"
android:onClick="buttonUnderline"
android:text="@string/underline"
app:layout_constraintStart_toEndOf="@+id/button2"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="240dp"
android:layout_marginTop="10dp"
android:onClick="buttonNoFormat"
android:text="@string/no_formatting"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button3" />
<Button
android:id="@+id/button5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="22dp"
android:layout_marginTop="35dp"
android:onClick="buttonAlignmentLeft"
android:text="@string/left"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextTextMultiLine" />
<Button
android:id="@+id/button6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="35dp"
android:layout_marginTop="35dp"
android:onClick="buttonAlignmentCenter"
android:text="@string/center"
app:layout_constraintStart_toEndOf="@+id/button5"
app:layout_constraintTop_toBottomOf="@+id/editTextTextMultiLine" />
<Button
android:id="@+id/button7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="27dp"
android:layout_marginTop="33dp"
android:onClick="buttonAlignmentRight"
android:text="@string/right"
app:layout_constraintStart_toEndOf="@+id/button6"
app:layout_constraintTop_toBottomOf="@+id/editTextTextMultiLine" />
<Button
android:id="@+id/button8"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="25dp"
android:layout_marginTop="5dp"
android:onClick="buttonFetch"
android:text="Fetch"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
plugins {
id 'com.android.application'
}
android {
compileSdk 31
defaultConfig {
applicationId "com.programmerworld.saveedittextsqlitedatabase"
minSdk 31
targetSdk 31
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.4.1'
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
}
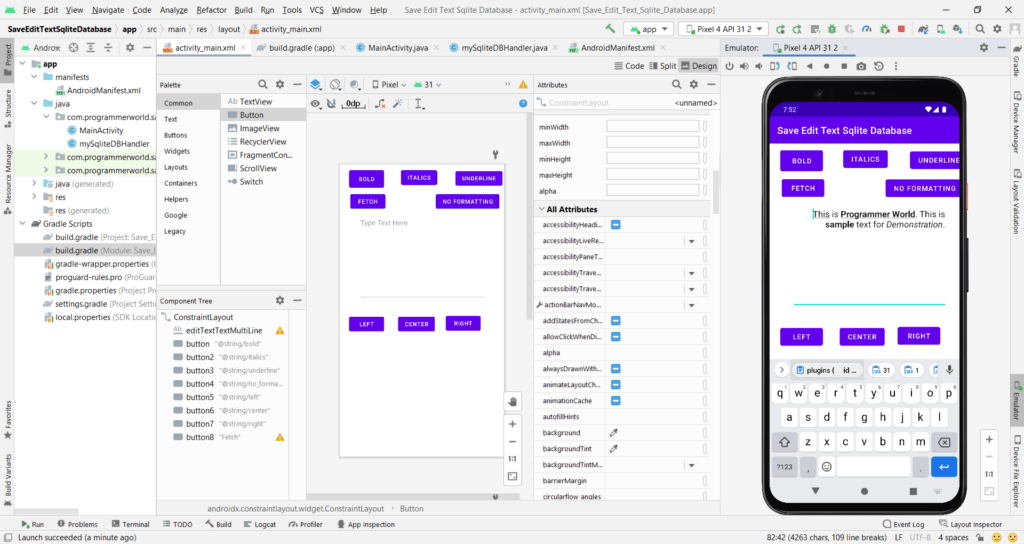
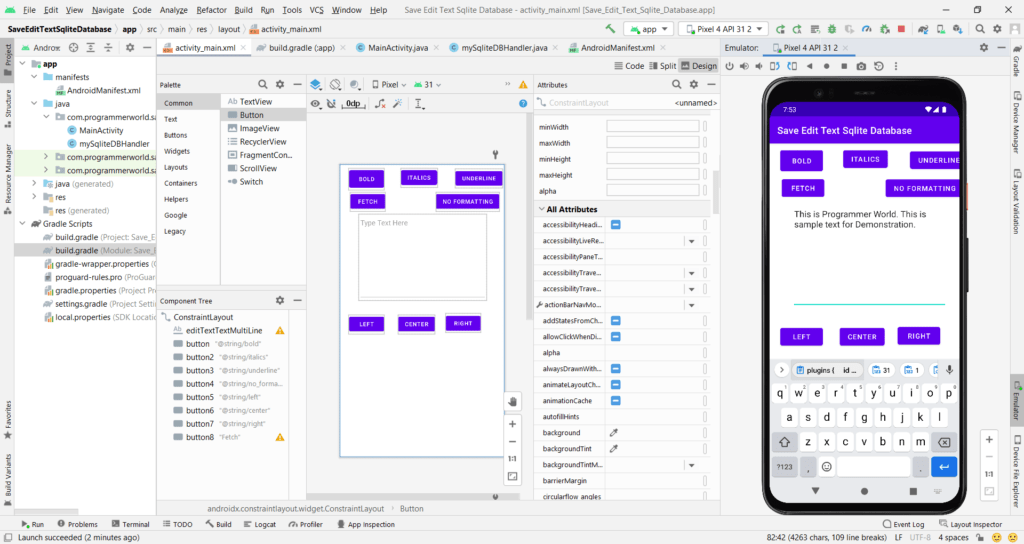
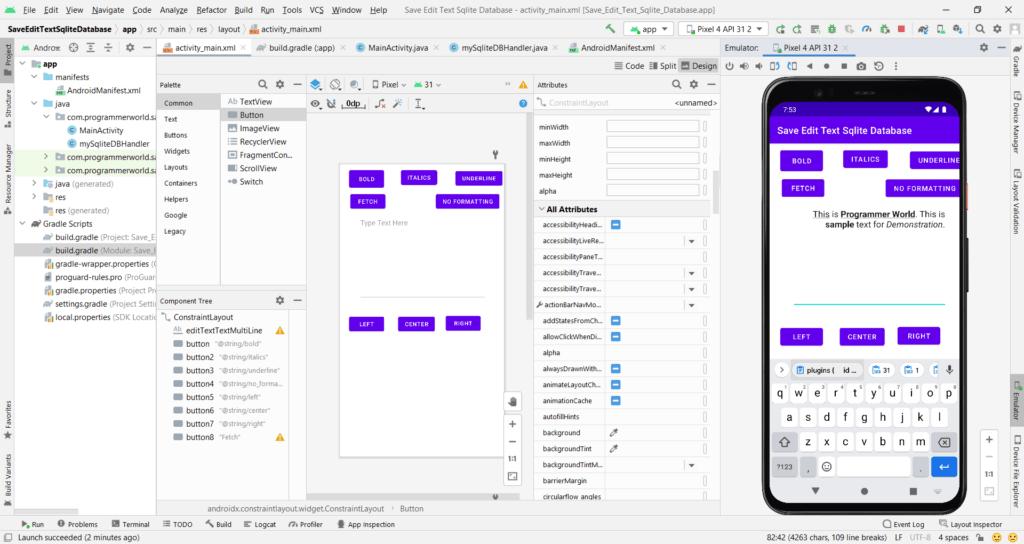