In this video it shows how to develop a Matlab App to connect and work with Oracle Database. It runs a simple query to fetch the data from the database and display it in the TextArea widget in the App.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
classdef OracleDBApp < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
OutputTextArea matlab.ui.control.TextArea
OutputTextAreaLabel matlab.ui.control.Label
OracleDBButton matlab.ui.control.Button
end
% Callbacks that handle component events
methods (Access = private)
% Button pushed function: OracleDBButton
function OracleDBButtonPushed(app, event)
connection = database('', ...
'CTXSYS', ...
'CTXSYS', ...
'Vendor', 'Oracle', ...
'driver', 'ojdbc14.jar', ...
'URL', ['jdbc:oracle:thin:@(Description =' ...
'(ADDRESS = (PROTOCOL = TCP)(HOST = localhost)' ...
'(PORT=1521)) (CONNECT_DATA=' ...
'(SERVER = DEDICATED)(SERVICE_NAME=XE)))' ]);
query = "SELECT table_name FROM user_tables fetch first 10 rows only";
data = fetch(connection, query);
close(connection);
data = table2array(data);
app.OutputTextArea.Value = data;
end
end
% Component initialization
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure and hide until all components are created
app.UIFigure = uifigure('Visible', 'off');
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'MATLAB App';
% Create OracleDBButton
app.OracleDBButton = uibutton(app.UIFigure, 'push');
app.OracleDBButton.ButtonPushedFcn = createCallbackFcn(app, @OracleDBButtonPushed, true);
app.OracleDBButton.Position = [33 256 100 22];
app.OracleDBButton.Text = 'Oracle DB';
% Create OutputTextAreaLabel
app.OutputTextAreaLabel = uilabel(app.UIFigure);
app.OutputTextAreaLabel.HorizontalAlignment = 'right';
app.OutputTextAreaLabel.Position = [190 419 42 22];
app.OutputTextAreaLabel.Text = 'Output';
% Create OutputTextArea
app.OutputTextArea = uitextarea(app.UIFigure);
app.OutputTextArea.Position = [247 58 354 385];
% Show the figure after all components are created
app.UIFigure.Visible = 'on';
end
end
% App creation and deletion
methods (Access = public)
% Construct app
function app = OracleDBApp
% Create UIFigure and components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
Code View:
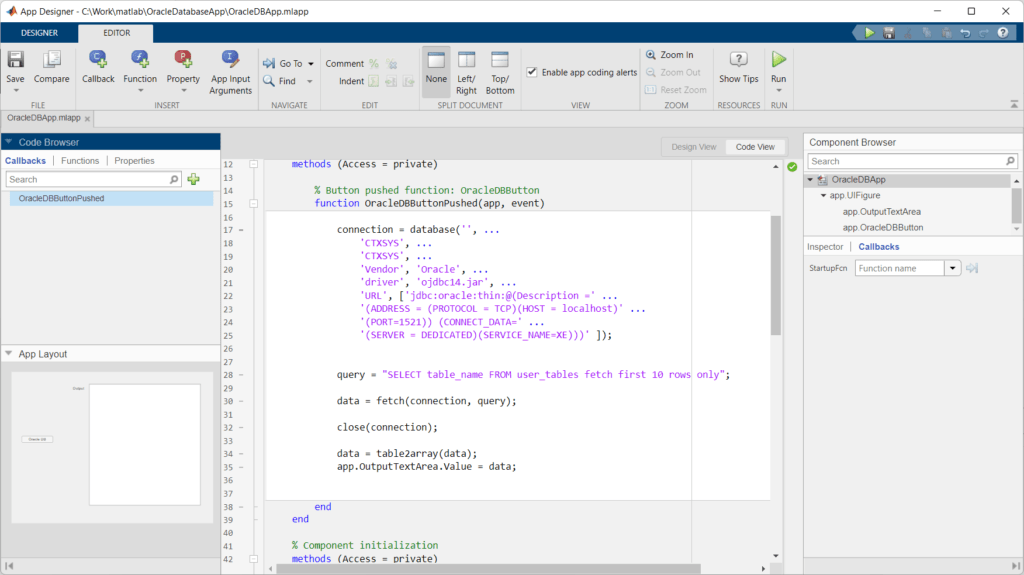
Design View:
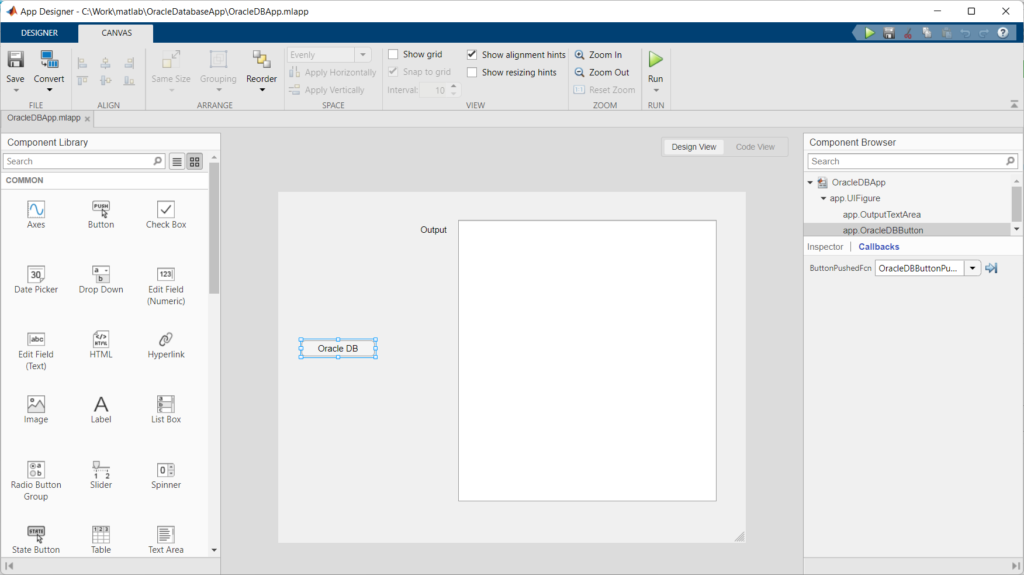
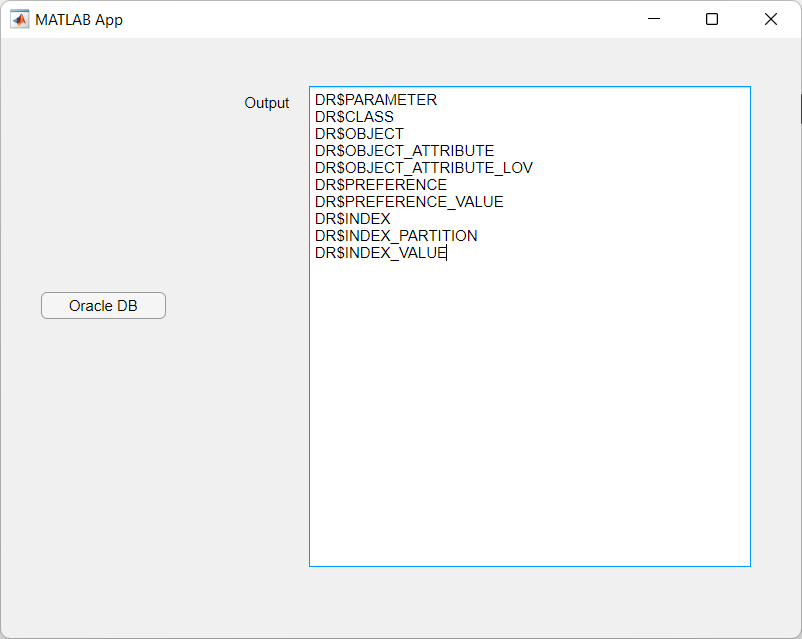
Connection through sqlplus:
C:\>sqlplus CTXSYS/CTXSYS@//localhost:1521/XE
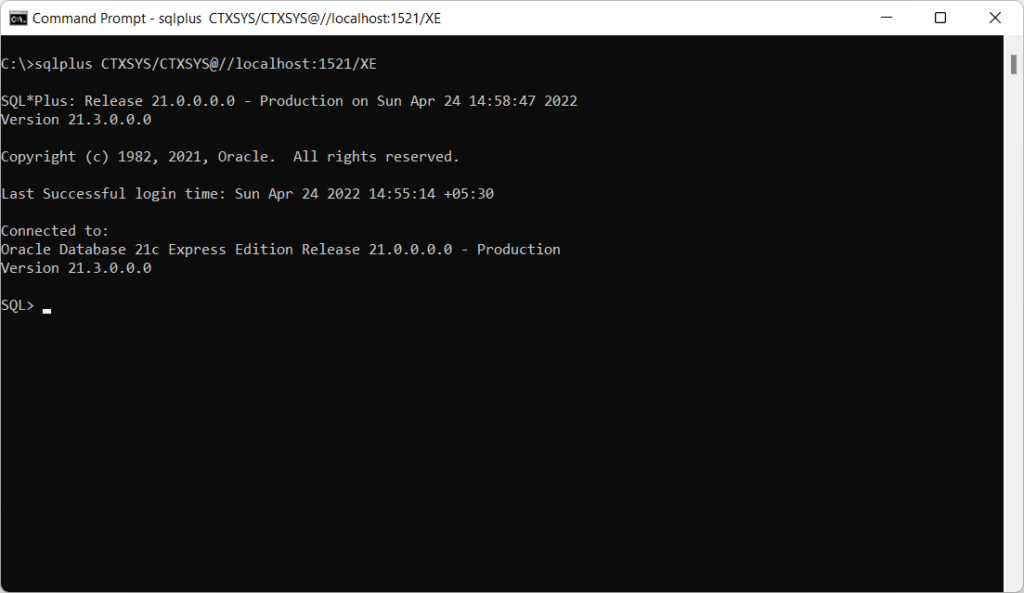
JAR File:
https://drive.google.com/drive/folders/1XRSIc-driOh0EkGnLlrJ4M6EZSPLfs6O?usp=sharing