This video shows steps to run Google’s AI tool – PaLM – API in MATLAB App Designer. It uses the cURL command of POST method to get the request.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
Main method:
% Button pushed function: Button
function ButtonPushed(app, event)
input = app.InputTextEditField.Value;
server_url = 'https://generativelanguage.googleapis.com/v1beta2/models/text-bison-001:generateText?key=AIzaSyDTCkvMdQlqnfki__444LimmVWIfTyWCrc';
data = struct('prompt', struct('text', input));
options = weboptions('RequestMethod', 'POST', ...
'MediaType','application/json', ...
'Timeout', 60);
response = webwrite(server_url, data, options);
app.OutputTextTextArea.Value = response.candidates.output;
end
Complete code:
classdef GoogleAIPaLMAPI < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
OutputTextTextArea matlab.ui.control.TextArea
OutputTextTextAreaLabel matlab.ui.control.Label
Button matlab.ui.control.Button
InputTextEditField matlab.ui.control.EditField
InputTextEditFieldLabel matlab.ui.control.Label
end
% Callbacks that handle component events
methods (Access = private)
% Button pushed function: Button
function ButtonPushed(app, event)
input = app.InputTextEditField.Value;
server_url = 'https://generativelanguage.googleapis.com/v1beta2/models/text-bison-001:generateText?key=AIzaSyDTCkvMdQlqnfki__444LimmVWIfTyWCrc';
data = struct('prompt', struct('text', input));
options = weboptions('RequestMethod', 'POST', ...
'MediaType','application/json', ...
'Timeout', 60);
response = webwrite(server_url, data, options);
app.OutputTextTextArea.Value = response.candidates.output;
end
end
% Component initialization
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure and hide until all components are created
app.UIFigure = uifigure('Visible', 'off');
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'MATLAB App';
% Create InputTextEditFieldLabel
app.InputTextEditFieldLabel = uilabel(app.UIFigure);
app.InputTextEditFieldLabel.HorizontalAlignment = 'right';
app.InputTextEditFieldLabel.Position = [33 388 57 22];
app.InputTextEditFieldLabel.Text = 'Input Text';
% Create InputTextEditField
app.InputTextEditField = uieditfield(app.UIFigure, 'text');
app.InputTextEditField.Position = [105 388 322 22];
% Create Button
app.Button = uibutton(app.UIFigure, 'push');
app.Button.ButtonPushedFcn = createCallbackFcn(app, @ButtonPushed, true);
app.Button.Position = [464 388 128 22];
% Create OutputTextTextAreaLabel
app.OutputTextTextAreaLabel = uilabel(app.UIFigure);
app.OutputTextTextAreaLabel.HorizontalAlignment = 'right';
app.OutputTextTextAreaLabel.Position = [22 334 66 22];
app.OutputTextTextAreaLabel.Text = 'Output Text';
% Create OutputTextTextArea
app.OutputTextTextArea = uitextarea(app.UIFigure);
app.OutputTextTextArea.Position = [103 53 489 305];
% Show the figure after all components are created
app.UIFigure.Visible = 'on';
end
end
% App creation and deletion
methods (Access = public)
% Construct app
function app = GoogleAIPaLMAPI
% Create UIFigure and components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
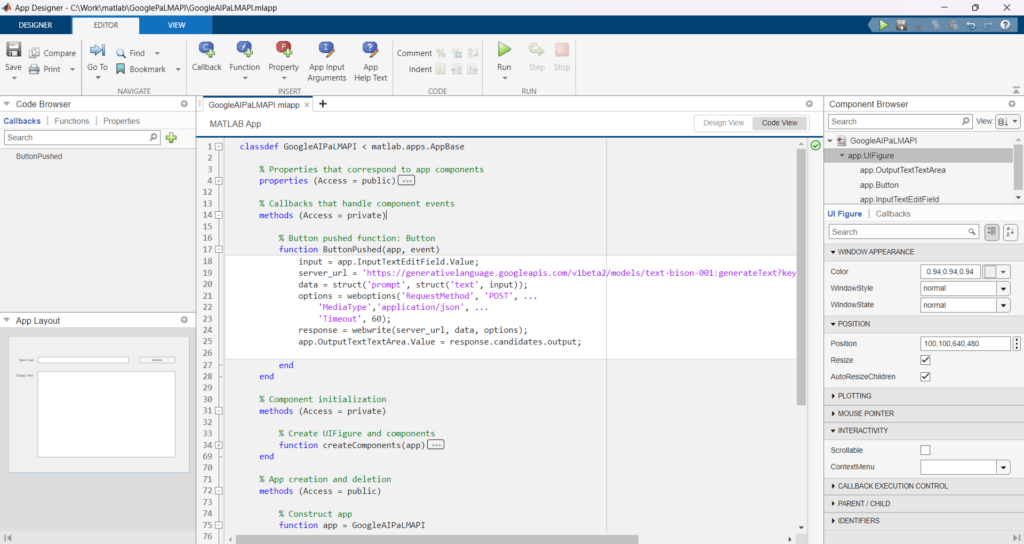
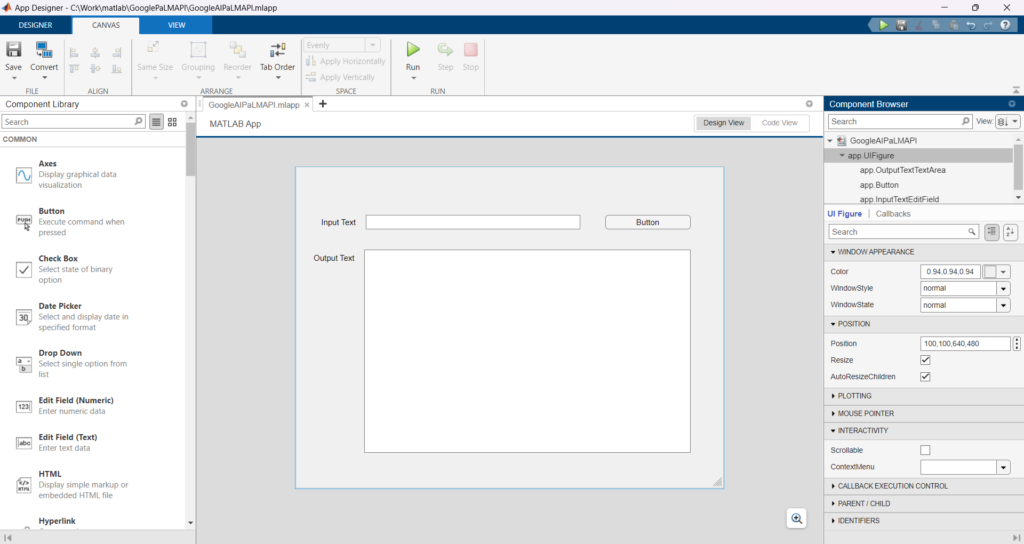
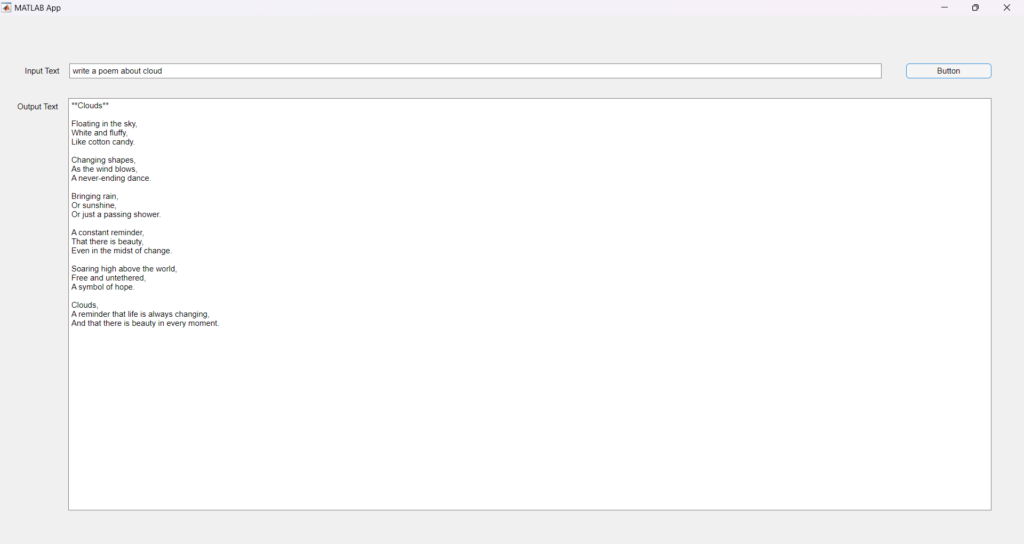
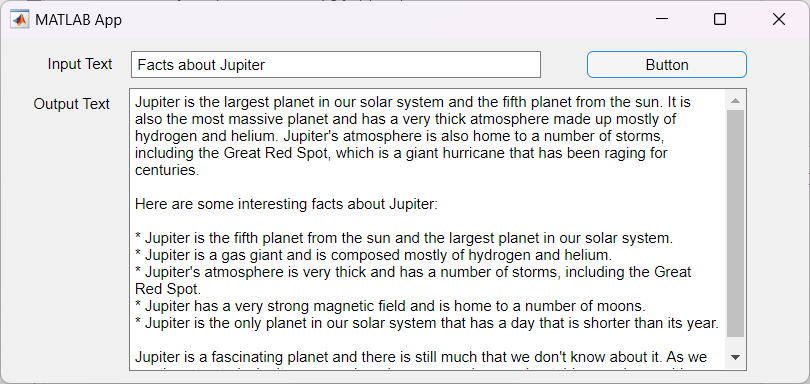