In this video it shows the code to detect any kind of rotation movement on touch screen. And then it rotates the Android App layout respectively to Portrait and Landscape orientation.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: contact@programmerworld.co / programmerworld1990@gmail.com
Complete source code and other details/ steps of this video are posted in the below link:
package com.programmerworld.rotatethescreen;
import android.content.pm.ActivityInfo;
import android.os.Bundle;
import android.view.MotionEvent;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class MainActivity extends AppCompatActivity {
private float previousX, previousY;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
return insets;
});
}
@Override
public boolean onTouchEvent(MotionEvent event) {
float x = event.getX();
float y = event.getY();
if (event.getAction() == MotionEvent.ACTION_MOVE) {
float dx = Math.abs(x - previousX);
float dy = Math.abs(y - previousY);
if (dx > 10 && dy > 10) {
if (getRequestedOrientation() == ActivityInfo.SCREEN_ORIENTATION_PORTRAIT) {
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
} else {
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
}
}
}
previousX = x;
previousY = y;
return true;
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello Programmer World!"
android:textSize="34sp"
android:textStyle="bold"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
Initial layout:
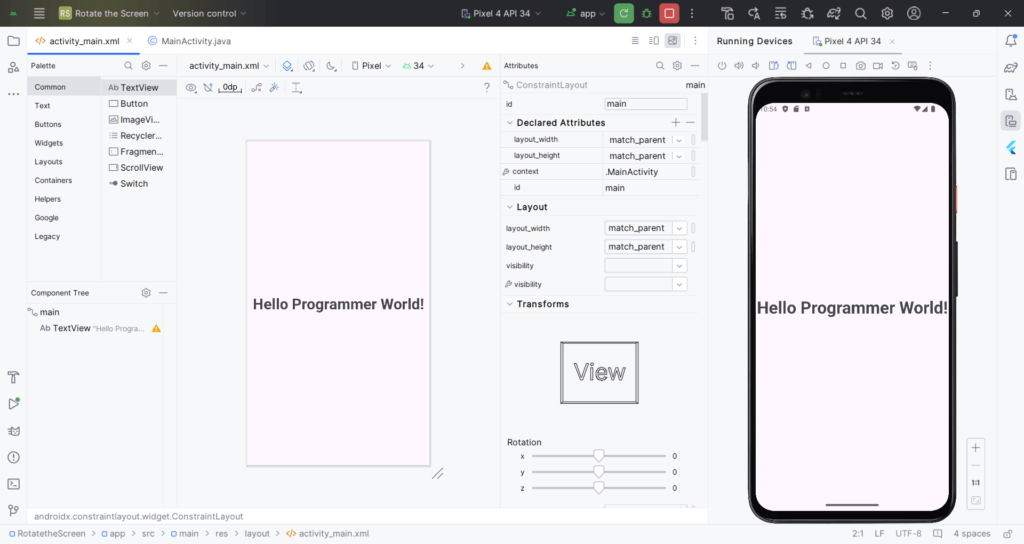
After rotation:
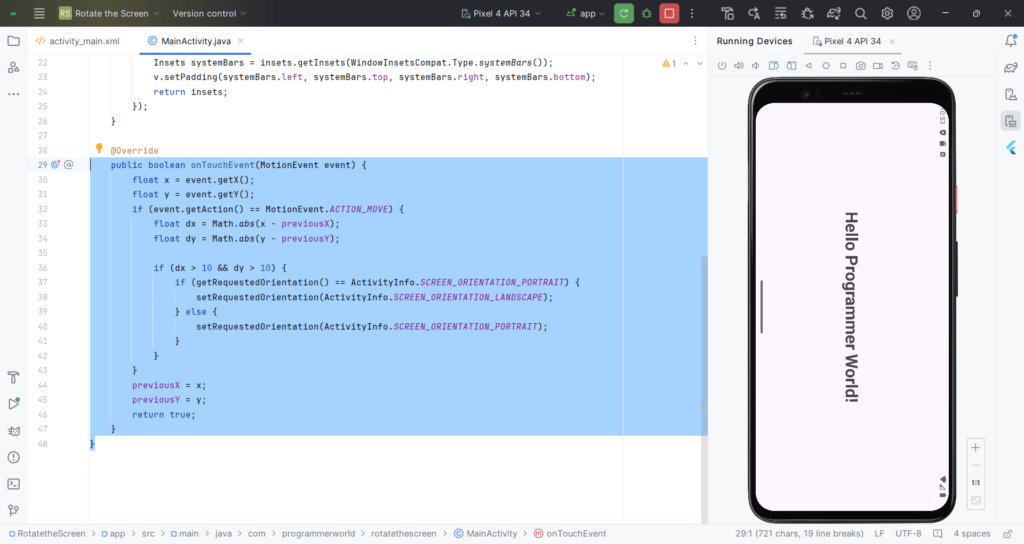
Project files can be accessed from the following link on payment of USD 9.
https://drive.google.com/file/d/1HLJw7YR7H2iaqdkoSVfWlLuiZKt4rs7T/view?usp=drive_link
Excerpt:
In the shared video, a method is demonstrated to detect rotational movements on a touchscreen and accordingly rotate an Android application’s layout between Portrait and Landscape orientations. The code includes handling touch events in such a way that if swiping is detected, the app’s orientation switches. Full source code is provided along with screenshots showcasing the initial layout and the layout after the application has been rotated. Contact information and additional details related to the video are available through the given contact links and email addresses.