This video shows step by step process to build a very simple calculator App in Android Studio and then upload it (host it) on the Google Play Store.
While entering the details of the App on the google play store, it uses very simple, easy and short descriptions and images. In commercial Applications, it is recommended to give more details.
The hosted App can be found on the google play store using the below link:
https://play.google.com/store/apps/details?id=com.programmerworld.calculator
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Source code:
package com.programmerworld.calculator;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView textViewResults;
private EditText editTextNo1, editTextNo2, editTextSign;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textViewResults = findViewById(R.id.textView);
editTextNo1 = findViewById(R.id.editTextNumberSigned1);
editTextNo2 = findViewById(R.id.editTextNumberSigned2);
editTextSign = findViewById(R.id.editTextTextPersonName);
}
public void buttonCalculate(View view){
Integer integer1 = Integer.parseInt(editTextNo1.getText().toString());
Integer integer2 = Integer.parseInt(editTextNo2.getText().toString());
Integer integerResult = 0;
switch (editTextSign.getText().toString()){
case("+"):
integerResult = integer1 + integer2;
textViewResults.setText(integerResult.toString());
break;
case ("-"):
integerResult = integer1 - integer2;
textViewResults.setText(integerResult.toString());
break;
case ("*"):
integerResult = integer1 * integer2;
textViewResults.setText(integerResult.toString());
break;
default:
textViewResults.setText("Incorrect Sign");
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/result"
android:textSize="36sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.53" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="152dp"
android:layout_marginTop="16dp"
android:onClick="buttonCalculate"
android:text="@string/calculate"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextNumberSigned2" />
<EditText
android:id="@+id/editTextNumberSigned1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="93dp"
android:layout_marginTop="29dp"
android:ems="10"
android:hint="@string/_1st_number"
android:inputType="numberSigned"
android:textAlignment="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
android:autofillHints="" />
<EditText
android:id="@+id/editTextNumberSigned2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="90dp"
android:layout_marginTop="50dp"
android:ems="10"
android:hint="@string/_2nd_number"
android:inputType="numberSigned"
android:textAlignment="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextTextPersonName"
android:autofillHints="" />
<EditText
android:id="@+id/editTextTextPersonName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="90dp"
android:layout_marginTop="45dp"
android:ems="10"
android:hint="@string/sign_here"
android:inputType="textPersonName"
android:textAlignment="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextNumberSigned1"
android:autofillHints="" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.calculator">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
google()
jcenter()
}
dependencies {
classpath "com.android.tools.build:gradle:4.0.1"
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
apply plugin: 'com.android.application' android { compileSdkVersion 29 buildToolsVersion "29.0.3" defaultConfig { applicationId "com.programmerworld.calculator" minSdkVersion 26 targetSdkVersion 29 versionCode 1 versionName "1.0" testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: "libs", include: ["*.jar"]) implementation 'androidx.appcompat:appcompat:1.2.0' implementation 'androidx.constraintlayout:constraintlayout:2.0.0' testImplementation 'junit:junit:4.12' androidTestImplementation 'androidx.test.ext:junit:1.1.1' androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0' }
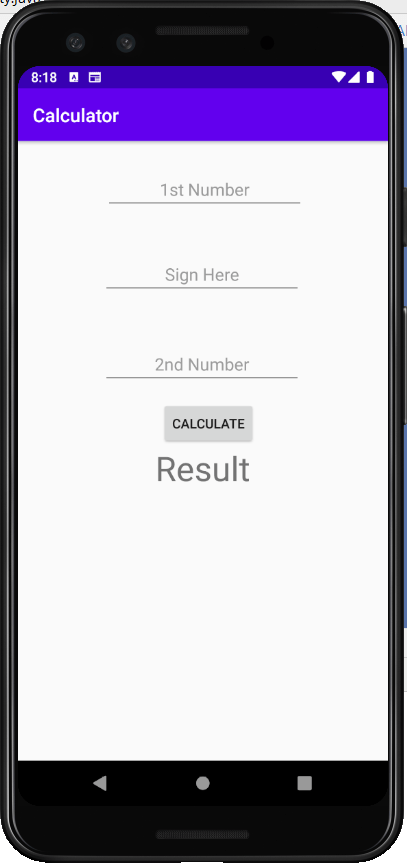
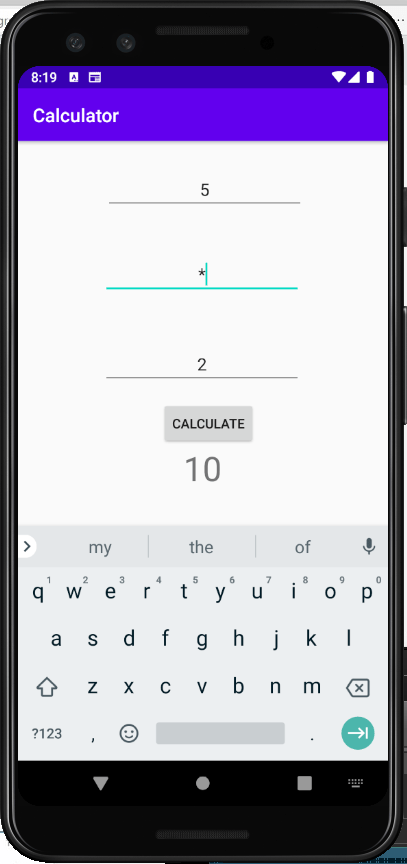