This video shows the steps to enable your App to access the files outside of your App’s data folder. Using these methods one can access the files available in external public directories which can be easily accessed by all the Apps.
In this video it shows two ways to get the files access. In first method it is hard-coding the complete path. However, in the 2nd approach it is using MediaStore to get the content URI and then access the file through this URI.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Source Code:
package com.programmerworld.accessexternalpublicfiles;
import android.Manifest;
import android.content.pm.PackageManager;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.ImageDecoder;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
public class MainActivity extends AppCompatActivity {
private TextView textView;
private ImageView imageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE},
PackageManager.PERMISSION_GRANTED);
textView = findViewById(R.id.textView);
imageView = findViewById(R.id.imageView);
}
public void buttonDirectAccess(View view){
try {
String stringFileName = "/storage/emulated/0/Download/download.jpeg";
Bitmap bitmap = BitmapFactory.decodeFile(stringFileName);
imageView.setImageBitmap(bitmap);
textView.setText("Image printed successfully");
}
catch (Exception e){
textView.setText("Failed - Exception");
}
}
public void buttonUsingURI(View view){
try{
Uri uriImage = MediaStore.Images.Media.getContentUri("external");
String[] stringsProjection = {MediaStore.Images.ImageColumns._ID};
Cursor cursor = getContentResolver().query(uriImage, stringsProjection, MediaStore.Images.ImageColumns.DISPLAY_NAME
+ " LIKE?", new String[]{"download.jpeg"}, null);
cursor.moveToFirst();
Uri uriPath = Uri.parse(MediaStore.Images.Media.EXTERNAL_CONTENT_URI.toString()+"/"+cursor.getString(0));
Bitmap bitmapImage = ImageDecoder.decodeBitmap(ImageDecoder.createSource(this.getContentResolver(), uriPath));
imageView.setImageBitmap(bitmapImage);
textView.setText("URI method is successful");
}
catch (Exception e){
textView.setText("Failure in URI method");
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.514"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.247" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="45dp"
android:layout_marginTop="98dp"
android:onClick="buttonDirectAccess"
android:text="Direct Access"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="52dp"
android:layout_marginTop="100dp"
android:onClick="buttonUsingURI"
android:text="Using URI"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="248dp"
android:layout_height="275dp"
android:layout_marginStart="77dp"
android:layout_marginTop="119dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView"
app:srcCompat="@drawable/ic_launcher_background" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.accessexternalpublicfiles">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.AccessExternalPublicFiles">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
plugins {
id 'com.android.application'
}
android {
compileSdk 31
defaultConfig {
applicationId "com.programmerworld.accessexternalpublicfiles"
minSdk 31
targetSdk 31
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.4.1'
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
}
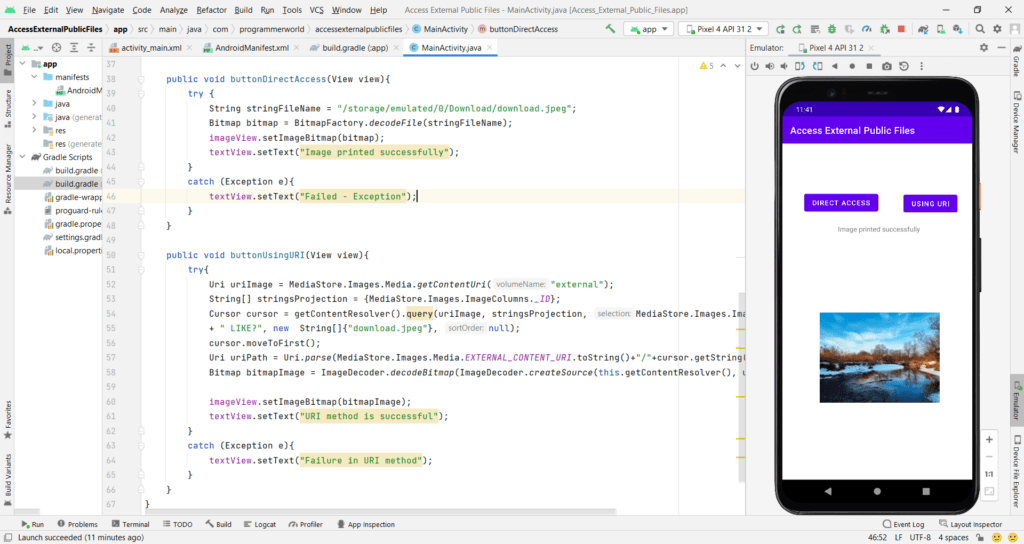