This video shows the steps to integrate Google’s Generative AI’s PaLM API from MakerSuite in your Android App. It uses the cURL command to integrate it.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.googlespalmapi;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import com.android.volley.AuthFailureError;
import com.android.volley.DefaultRetryPolicy;
import com.android.volley.Request;
import com.android.volley.Response;
import com.android.volley.RetryPolicy;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.JsonObjectRequest;
import com.android.volley.toolbox.Volley;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
public class MainActivity extends AppCompatActivity {
private TextView textView;
private EditText editText;
private String stringAPIKey = "AIzaSyCJ1FEQyrDM6gSiEnKgdr18ykAZO1zqcf8";
private String stringURLEndPoint = "https://generativelanguage.googleapis.com/v1beta2/models/text-bison-001:generateText?key="
+ stringAPIKey;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editTextText);
textView = findViewById(R.id.textView);
}
public void buttonPaLMAPI(View view){
String stringInputText = "Write a story about a magic backpack.";
if (!editText.getText().toString().isEmpty()){
stringInputText = editText.getText().toString();
}
JSONObject jsonObject = new JSONObject();
JSONObject jsonObjectText = new JSONObject();
try {
jsonObjectText.put("text", stringInputText);
jsonObject.put("prompt", jsonObjectText);
} catch (JSONException e) {
throw new RuntimeException(e);
}
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest(Request.Method.POST,
stringURLEndPoint,
jsonObject,
new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
String stringOutput = response.getJSONArray("candidates")
.getJSONObject(0)
.getString("output");
textView.setText(stringOutput);
} catch (JSONException e) {
throw new RuntimeException(e);
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
textView.setText("Error");
}
}){
@Override
public Map<String, String> getHeaders() throws AuthFailureError {
Map<String, String> mapHeader = new HashMap<>();
mapHeader.put("Content-Type", "application/json");
return mapHeader;
}
};
int intTimeoutPeriod = 60000; //60 seconds
RetryPolicy retryPolicy = new DefaultRetryPolicy(intTimeoutPeriod,
DefaultRetryPolicy.DEFAULT_MAX_RETRIES,
DefaultRetryPolicy.DEFAULT_BACKOFF_MULT);
jsonObjectRequest.setRetryPolicy(retryPolicy);
Volley.newRequestQueue(getApplicationContext()).add(jsonObjectRequest);
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.GooglesPaLMAPI"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
plugins {
id("com.android.application")
}
android {
namespace = "com.programmerworld.googlespalmapi"
compileSdk = 34
defaultConfig {
applicationId = "com.programmerworld.googlespalmapi"
minSdk = 34
targetSdk = 34
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
}
dependencies {
implementation("androidx.appcompat:appcompat:1.6.1")
implementation("com.google.android.material:material:1.9.0")
implementation("androidx.constraintlayout:constraintlayout:2.1.4")
testImplementation("junit:junit:4.13.2")
androidTestImplementation("androidx.test.ext:junit:1.1.5")
androidTestImplementation("androidx.test.espresso:espresso-core:3.5.1")
implementation("com.android.volley:volley:1.2.1");
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="351dp"
android:layout_height="418dp"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.872" />
<Button
android:id="@+id/button"
android:layout_width="181dp"
android:layout_height="56dp"
android:layout_marginStart="112dp"
android:layout_marginTop="151dp"
android:onClick="buttonPaLMAPI"
android:text="PaLM API"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editTextText"
android:layout_width="319dp"
android:layout_height="73dp"
android:layout_marginStart="42dp"
android:layout_marginTop="40dp"
android:ems="10"
android:hint="Input Text Here ..."
android:inputType="text"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
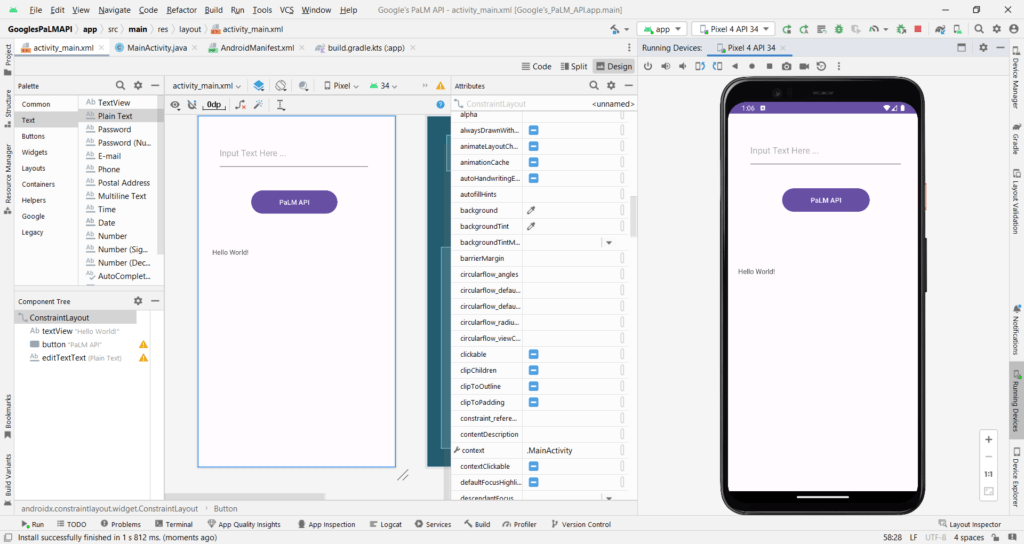
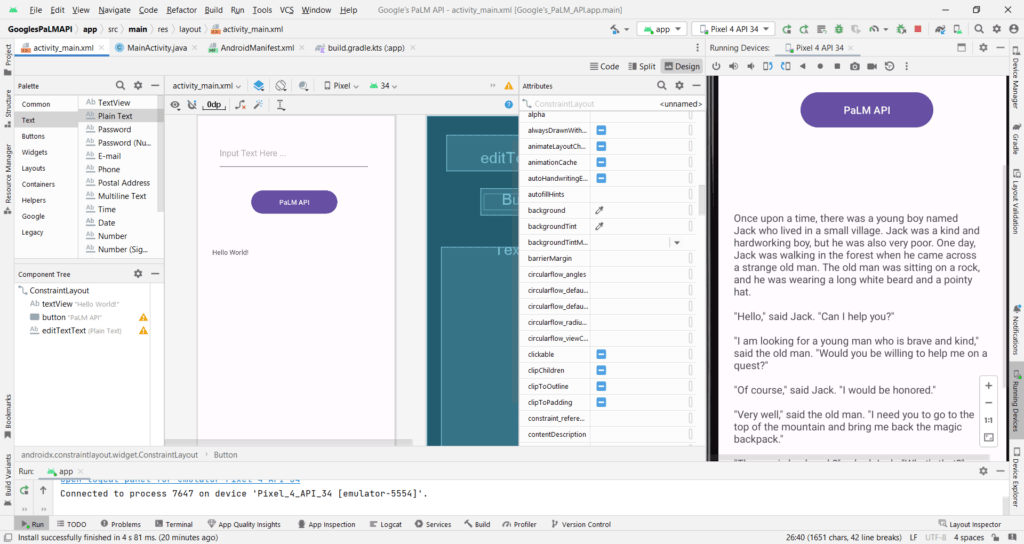
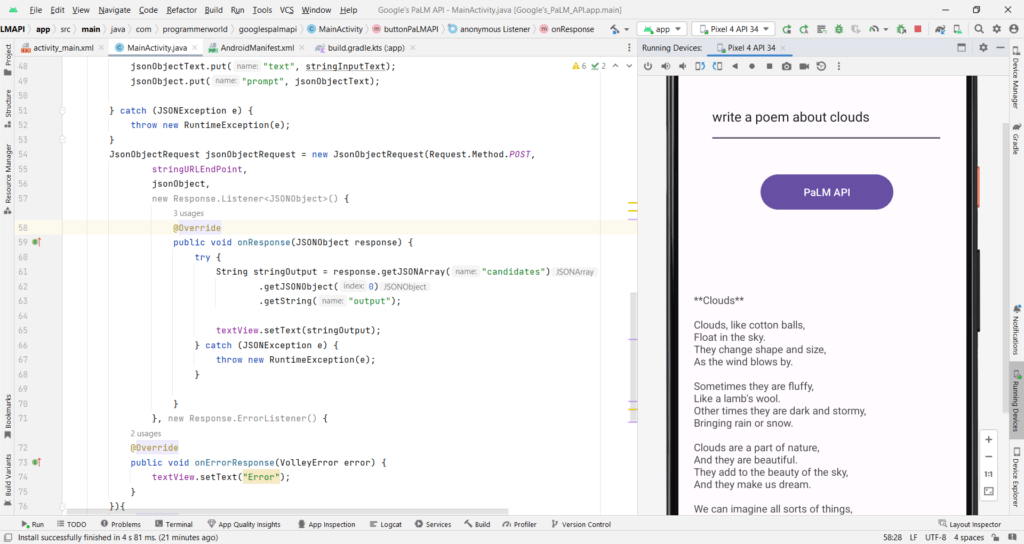