This video shows the steps to run a MATLAB Live script/ function from your App designer code. In this video it creates demo live script and function. Then execute them from MATLAB command line and App Designer’s code using the file name.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
Initialize Array
x = 2:3:50;
Plot the Array
plot(x);
Live Function
function LiveFunctionExample(step)
y = 2:step:50;
assignin("base","y_base",y);
plot(y);
end
classdef Live_MLX_Run_Example < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
LiveFunctionButton matlab.ui.control.Button
LiveScriptButton matlab.ui.control.Button
end
% Callbacks that handle component events
methods (Access = private)
% Button pushed function: LiveScriptButton
function LiveScriptButtonPushed(app, event)
LiveScriptExample;
end
% Button pushed function: LiveFunctionButton
function LiveFunctionButtonPushed(app, event)
LiveFunctionExample(8);
end
end
% Component initialization
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure and hide until all components are created
app.UIFigure = uifigure('Visible', 'off');
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'MATLAB App';
% Create LiveScriptButton
app.LiveScriptButton = uibutton(app.UIFigure, 'push');
app.LiveScriptButton.ButtonPushedFcn = createCallbackFcn(app, @LiveScriptButtonPushed, true);
app.LiveScriptButton.Position = [230 398 100 22];
app.LiveScriptButton.Text = 'Live Script';
% Create LiveFunctionButton
app.LiveFunctionButton = uibutton(app.UIFigure, 'push');
app.LiveFunctionButton.ButtonPushedFcn = createCallbackFcn(app, @LiveFunctionButtonPushed, true);
app.LiveFunctionButton.Position = [230 308 100 22];
app.LiveFunctionButton.Text = 'Live Function';
% Show the figure after all components are created
app.UIFigure.Visible = 'on';
end
end
% App creation and deletion
methods (Access = public)
% Construct app
function app = Live_MLX_Run_Example
% Create UIFigure and components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
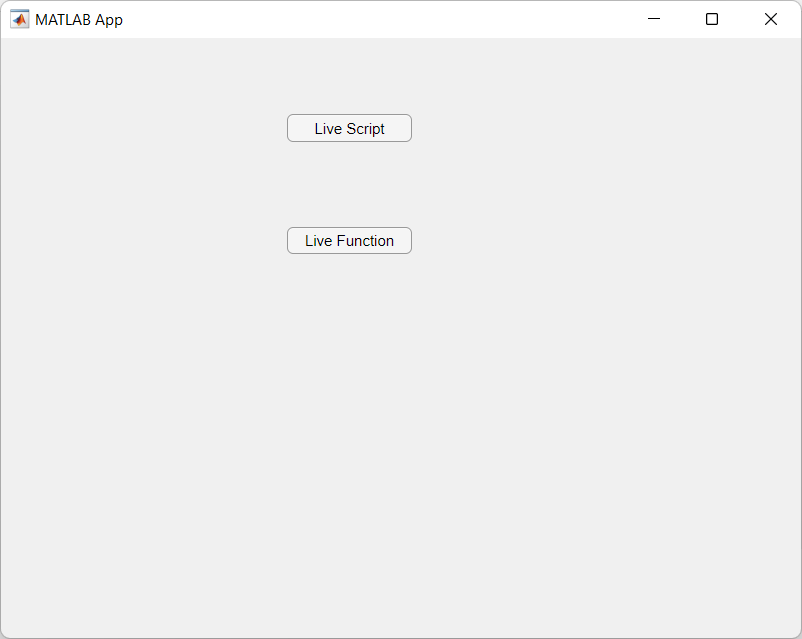
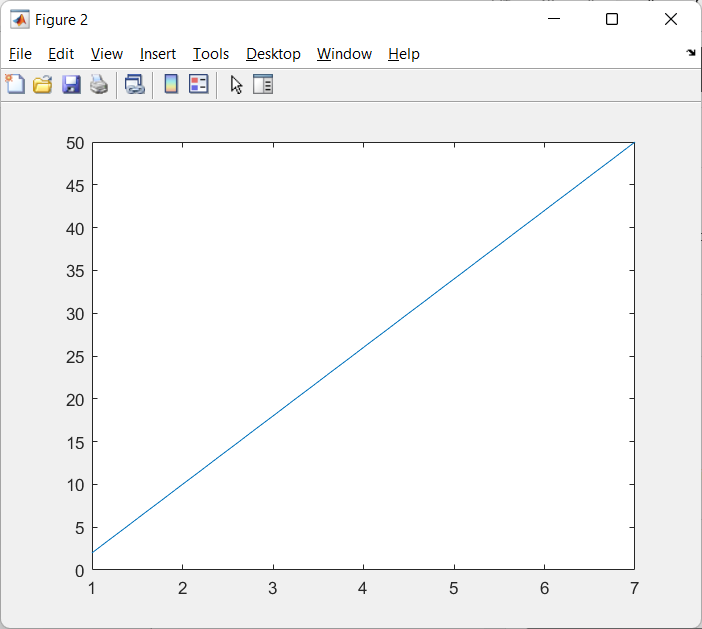
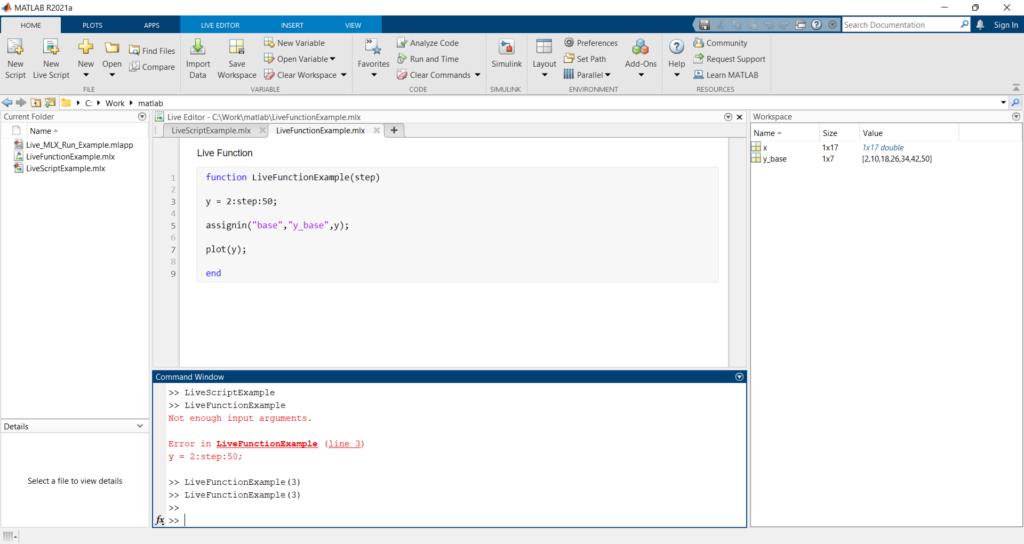
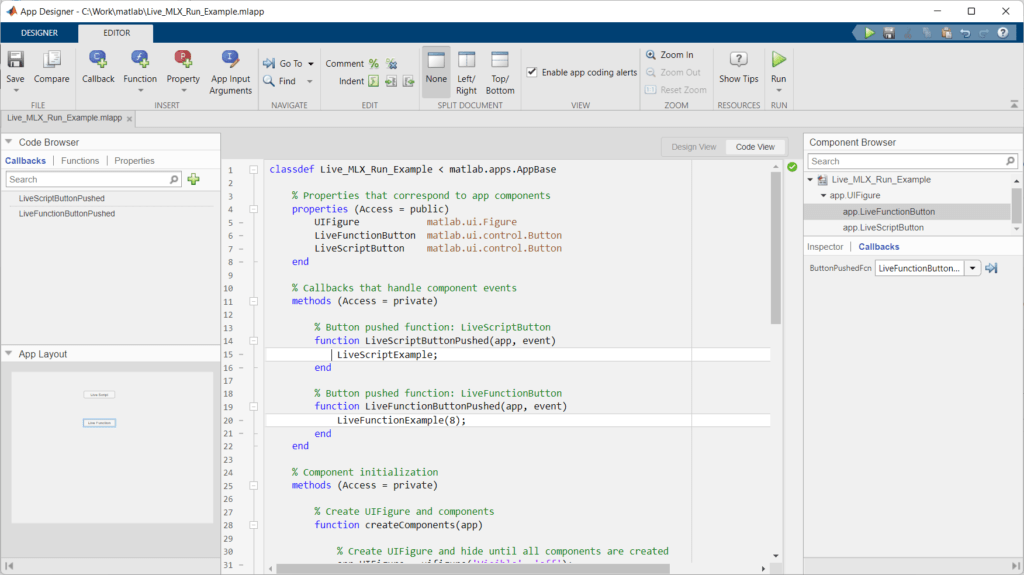