In this video it shows the steps to add the watermark in your PDF file. It uses PdfStamper object to put the watermark (stamp) as an over content on your PDF pages.
In this video it re-uses the create PDF method code from our below page:
It further develops the App’s code to show how the watermark can easily be added in the PDF pages. This code uses Android 11 (API level 30).
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Source Code:
package com.programmerworld.watermarkpdfapp;
import android.Manifest;
import android.content.pm.PackageManager;
import android.graphics.Paint;
import android.graphics.pdf.PdfDocument;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import com.itextpdf.text.Element;
import com.itextpdf.text.Font;
import com.itextpdf.text.Phrase;
import com.itextpdf.text.Rectangle;
import com.itextpdf.text.pdf.ColumnText;
import com.itextpdf.text.pdf.PdfContentByte;
import com.itextpdf.text.pdf.PdfGState;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.text.pdf.PdfStamper;
import java.io.File;
import java.io.FileOutputStream;
public class MainActivity extends AppCompatActivity {
private EditText editText;
private File file;
private File fileWaterMark;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.READ_EXTERNAL_STORAGE, Manifest.permission.WRITE_EXTERNAL_STORAGE},
PackageManager.PERMISSION_GRANTED);
editText = findViewById(R.id.editText);
file = new File(getExternalFilesDir(null), "ProgrammerWorld.pdf");
fileWaterMark = new File(getExternalFilesDir(null), "WaterMark.pdf");
}
public void buttonCreatePDF(View view){
PdfDocument pdfDocument = new PdfDocument();
PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder(300, 600, 1).create();
PdfDocument.Page page = pdfDocument.startPage(pageInfo);
Paint paint = new Paint();
String stringPDF = editText.getText().toString();
for (int i = 0; i<5; i++){
stringPDF = stringPDF + "\n" + stringPDF + "\n";
}
int x = 10, y = 25;
for (String line:stringPDF.split("\n")){
page.getCanvas().drawText(line,x,y, paint);
y+=paint.descent()-paint.ascent();
}
pdfDocument.finishPage(page);
try {
pdfDocument.writeTo(new FileOutputStream(file));
}
catch (Exception e){
e.printStackTrace();
}
pdfDocument.close();
}
public void buttonAddWaterMark(View view){
try {
PdfReader pdfReader = new PdfReader(file.getPath());
PdfStamper pdfStamper = new PdfStamper(pdfReader, new FileOutputStream(fileWaterMark));
PdfContentByte pdfContentByte = pdfStamper.getOverContent(1);
Rectangle rectanglePageSize = pdfReader.getPageSize(1);
float horizontal_mid_position = (rectanglePageSize.getLeft() + rectanglePageSize.getRight())/2;
float vertical_mid_position = (rectanglePageSize.getTop() + rectanglePageSize.getBottom())/2;
PdfGState pdfGState = new PdfGState();
pdfGState.setFillOpacity(0.25f);
pdfContentByte.setGState(pdfGState);
ColumnText.showTextAligned(pdfContentByte, Element.ALIGN_CENTER,
new Phrase("Programmer World", new Font(Font.FontFamily.TIMES_ROMAN, 45)),
horizontal_mid_position, vertical_mid_position,45);
pdfStamper.close();
pdfReader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.watermarkpdfapp">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.WaterMarkPDFApp">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="106dp"
android:layout_marginTop="111dp"
android:onClick="buttonCreatePDF"
android:text="@string/create_pdf"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="96dp"
android:layout_marginTop="56dp"
android:onClick="buttonAddWaterMark"
android:text="@string/add_watermark"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
<EditText
android:id="@+id/editText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="68dp"
android:layout_marginTop="23dp"
android:autofillHints=""
android:ems="10"
android:gravity="start|top"
android:hint="@string/enter_the_text_here"
android:inputType="textMultiLine"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:ignore="TouchTargetSizeCheck,SpeakableTextPresentCheck,SpeakableTextPresentCheck,SpeakableTextPresentCheck" />
</androidx.constraintlayout.widget.ConstraintLayout>
plugins {
id 'com.android.application'
}
android {
compileSdk 30
defaultConfig {
applicationId "com.programmerworld.watermarkpdfapp"
minSdk 30
targetSdk 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.3.1'
implementation 'com.google.android.material:material:1.4.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.1'
testImplementation 'junit:junit:4.+'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
implementation 'com.itextpdf:itextg:5.5.10'
}
<resources>
<string name="app_name">WaterMark PDF App</string>
<string name="add_watermark">Add WaterMark</string>
<string name="create_pdf">Create PDF</string>
<string name="enter_the_text_here">Enter the text here...</string>
</resources>
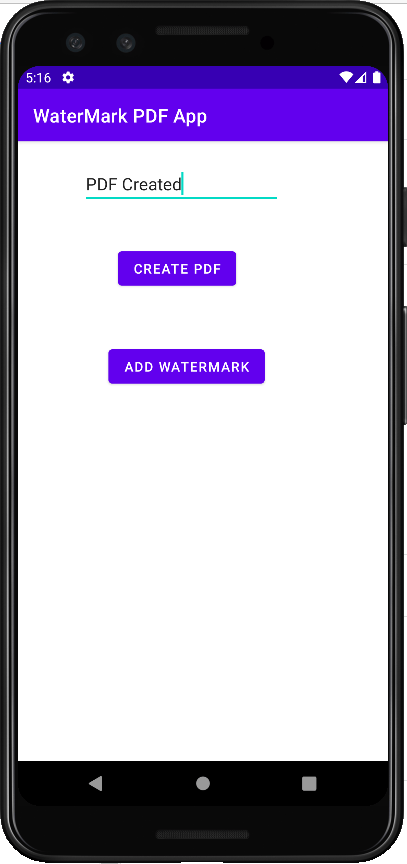
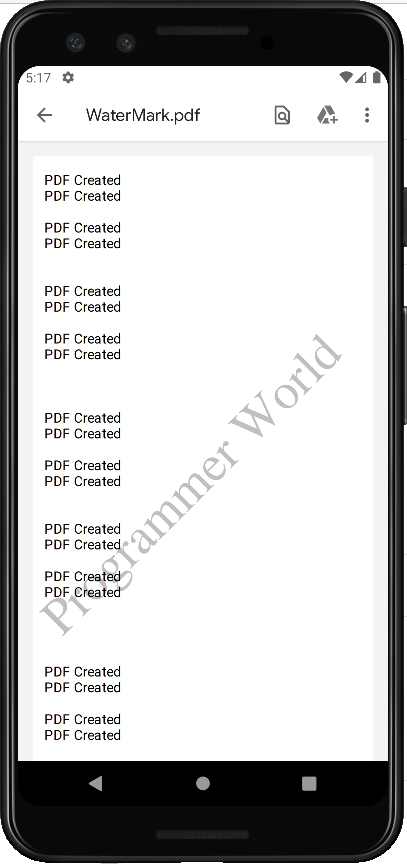