In this video it shows steps to display a list of strings (array) in the recycler view of your Android App. It shows the steps to create the recycler view adapter class and use it to set the adapter value of the recycler view.
It refers to below page from Android developer for the recycler view adapter class: https://developer.android.com/develop/ui/views/layout/recyclerview
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.recyclerviewsetarray;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.GridLayoutManager;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.os.Bundle;
import android.view.View;
public class MainActivity extends AppCompatActivity {
private RecyclerView recyclerView;
private RecyclerView.LayoutManager layoutManagerRecyclerView;
private RecyclerViewCustomAdapter recyclerViewCustomAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recyclerView = findViewById(R.id.recyclerView);
// 1 Linear Layout
//layoutManagerRecyclerView = new LinearLayoutManager(MainActivity.this);
// 2 Grid Layout
layoutManagerRecyclerView = new GridLayoutManager(MainActivity.this, 2);
recyclerView.setLayoutManager(layoutManagerRecyclerView);
}
public void buttonRecyclerViewList(View view){
String[] stringsArray = {"1 ABC\nThis if the first comment\nby Programmer World",
"2 DEF\nThis if the second comment\nby Programmer World",
"3 GHI\nThis if the third comment\nby Programmer World",
"4 JKL\nThis if the fourth comment\nby Programmer World"};
recyclerViewCustomAdapter = new RecyclerViewCustomAdapter(stringsArray);
recyclerView.setAdapter(recyclerViewCustomAdapter);
}
}
package com.programmerworld.recyclerviewsetarray;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import androidx.recyclerview.widget.RecyclerView;
public class RecyclerViewCustomAdapter extends RecyclerView.Adapter<RecyclerViewCustomAdapter.ViewHolder> {
private String[] localDataSet;
/**
* Provide a reference to the type of views that you are using
* (custom ViewHolder)
*/
public static class ViewHolder extends RecyclerView.ViewHolder {
private final TextView textView;
public ViewHolder(View view) {
super(view);
// Define click listener for the ViewHolder's View
textView = (TextView) view.findViewById(R.id.textView);
}
public TextView getTextView() {
return textView;
}
}
/**
* Initialize the dataset of the Adapter
*
* @param dataSet String[] containing the data to populate views to be used
* by RecyclerView
*/
public RecyclerViewCustomAdapter(String[] dataSet) {
localDataSet = dataSet;
}
// Create new views (invoked by the layout manager)
@Override
public ViewHolder onCreateViewHolder(ViewGroup viewGroup, int viewType) {
// Create a new view, which defines the UI of the list item
View view = LayoutInflater.from(viewGroup.getContext())
.inflate(R.layout.text_row_item, viewGroup, false);
return new ViewHolder(view);
}
// Replace the contents of a view (invoked by the layout manager)
@Override
public void onBindViewHolder(ViewHolder viewHolder, final int position) {
// Get element from your dataset at this position and replace the
// contents of the view with that element
viewHolder.getTextView().setText(localDataSet[position]);
}
// Return the size of your dataset (invoked by the layout manager)
@Override
public int getItemCount() {
return localDataSet.length;
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="108dp"
android:layout_marginTop="64dp"
android:onClick="buttonRecyclerViewList"
android:text="Recycler View List"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="308dp"
android:layout_height="277dp"
android:layout_marginStart="48dp"
android:layout_marginTop="96dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="TextView"
android:textSize="24sp" />
</FrameLayout>
For Grid Layout with Span count of 2:
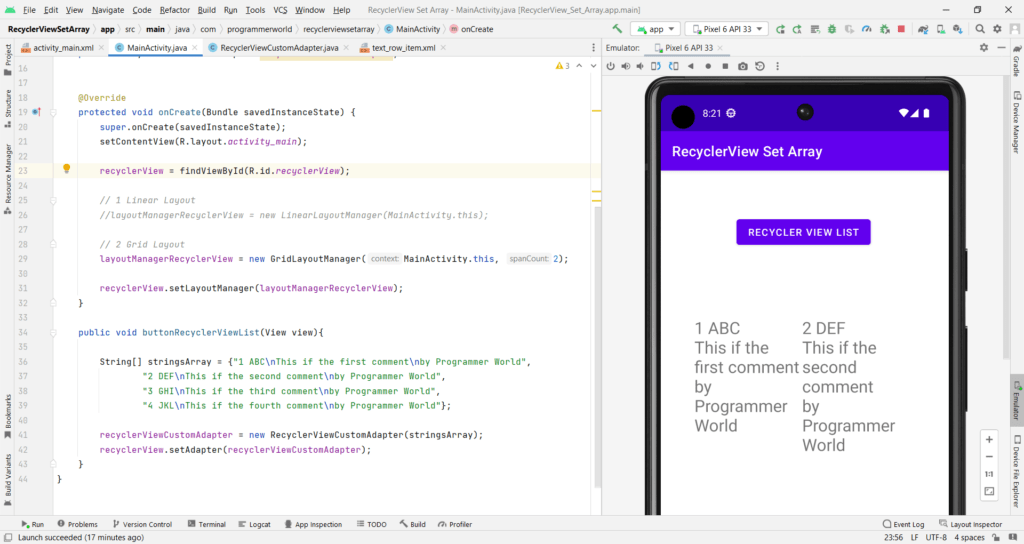
For Linear layout
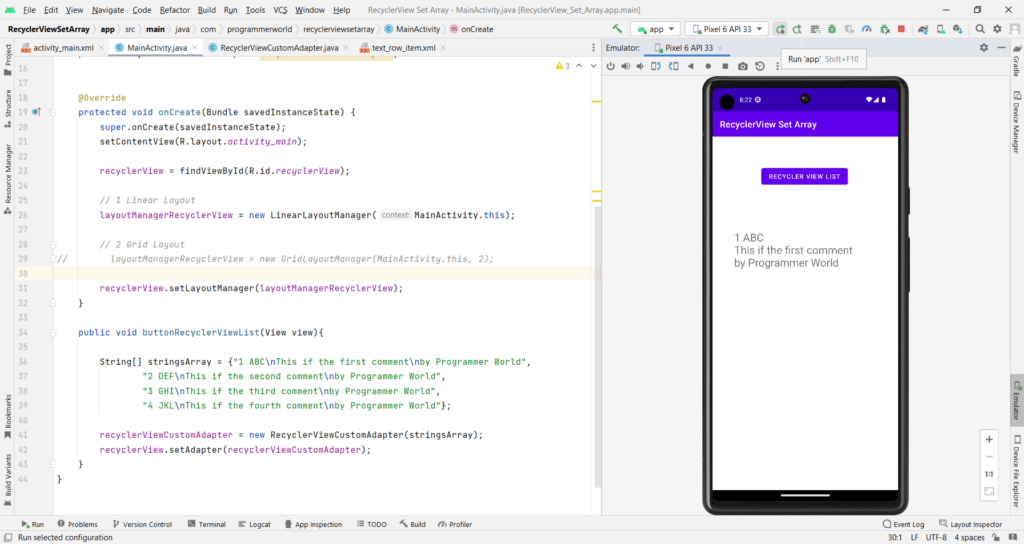