This video shows the steps to read an image file from the download folder and then show it as bitmap in imageView widget of your Android App’s layout.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.imagefilefromdownloadfolder;
import static android.Manifest.permission.READ_MEDIA_IMAGES;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.storage.StorageManager;
import android.os.storage.StorageVolume;
import android.view.View;
import android.widget.EditText;
import android.widget.ImageView;
import java.io.File;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{READ_MEDIA_IMAGES},
PackageManager.PERMISSION_GRANTED);
editText = findViewById(R.id.editText);
imageView = findViewById(R.id.imageView);
}
public void buttonImageShow(View view){
StorageManager storageManager = (StorageManager) getSystemService(STORAGE_SERVICE);
StorageVolume storageVolume = storageManager.getStorageVolumes().get(0); // 0 - internal storage; 1 - external storage
File fileInputImage = new File(storageVolume.getDirectory().getPath()
+ "/Download/"
+ editText.getText().toString()
+ ".jpeg");
Bitmap bitmapImageFile = BitmapFactory.decodeFile(fileInputImage.getPath());
imageView.setImageBitmap(bitmapImageFile);
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.READ_MEDIA_IMAGES"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.ImageFileFromDownloadFolder"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="78dp"
android:layout_marginTop="48dp"
android:onClick="buttonImageShow"
android:text="Show Image Download Folder"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
<EditText
android:id="@+id/editText"
android:layout_width="367dp"
android:layout_height="68dp"
android:layout_marginStart="22dp"
android:layout_marginTop="27dp"
android:ems="10"
android:hint="Image file name ..."
android:inputType="text"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="307dp"
android:layout_height="290dp"
android:layout_marginStart="56dp"
android:layout_marginTop="72dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
app:srcCompat="@drawable/ic_launcher_background" />
</androidx.constraintlayout.widget.ConstraintLayout>
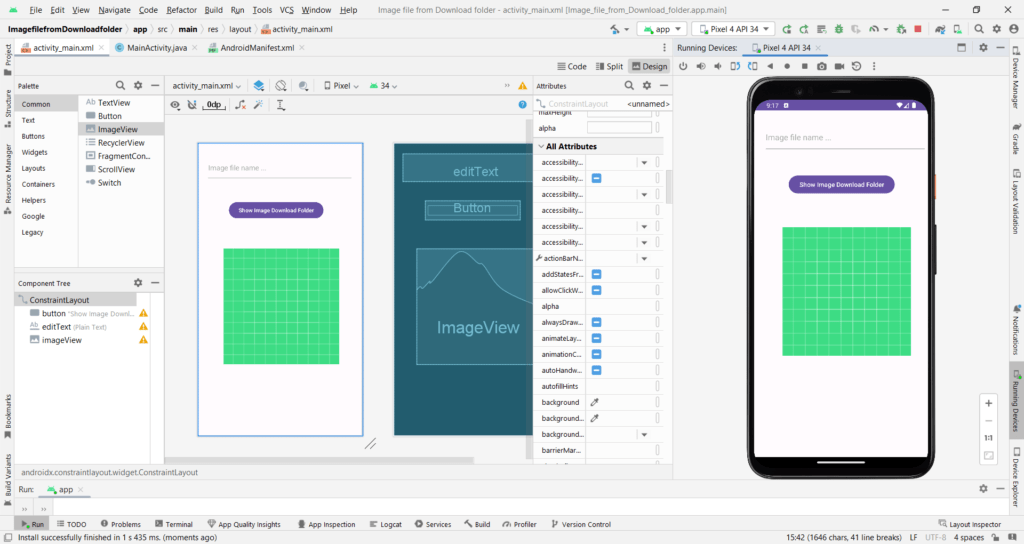
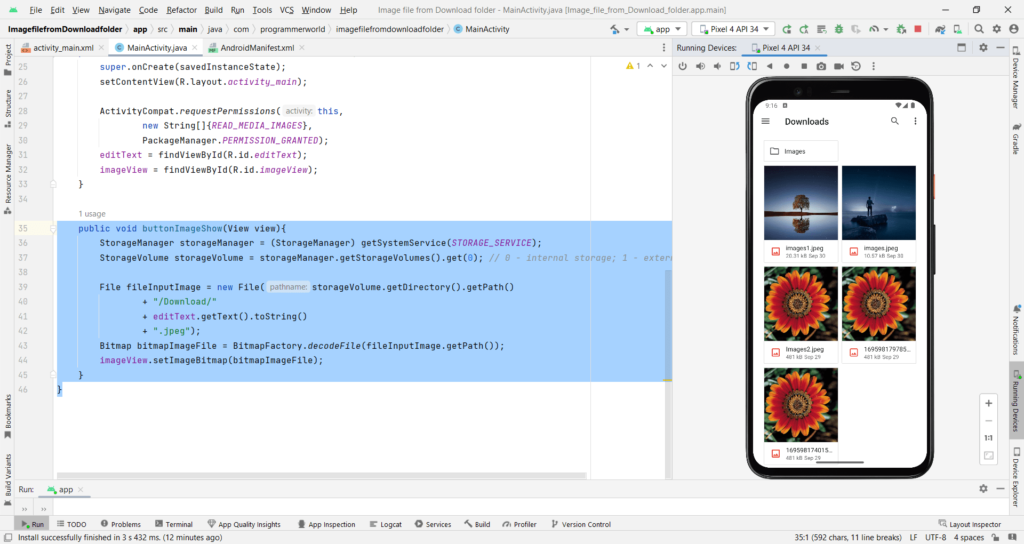
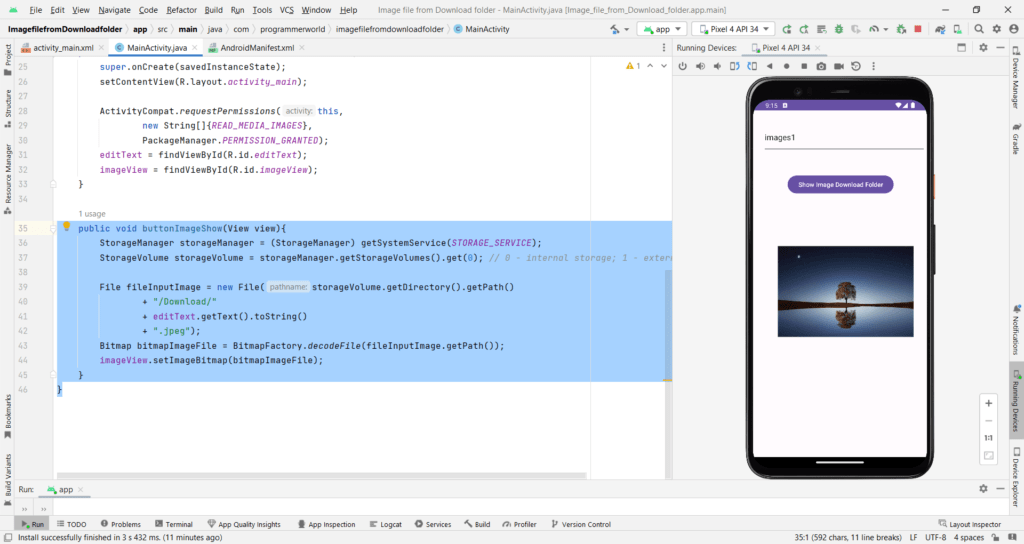
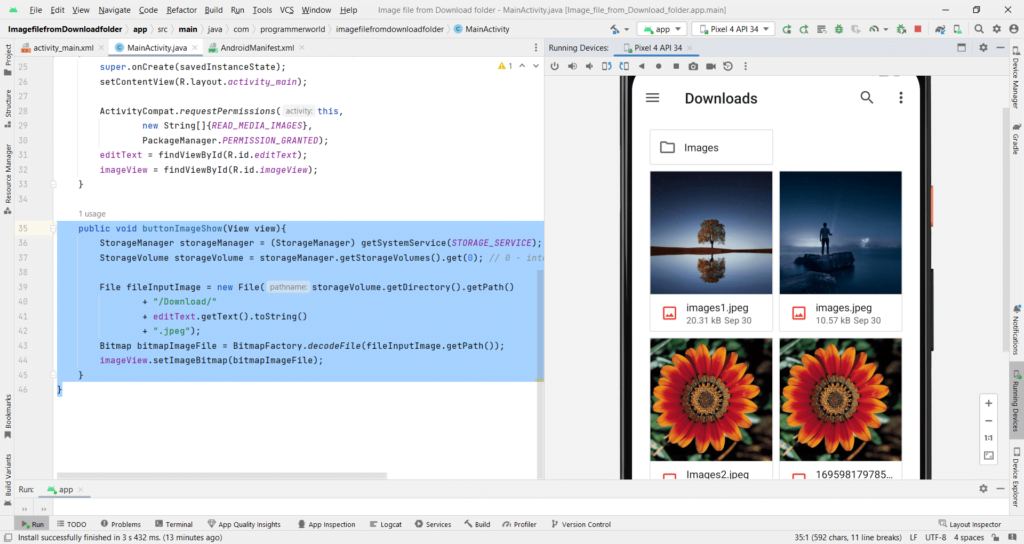
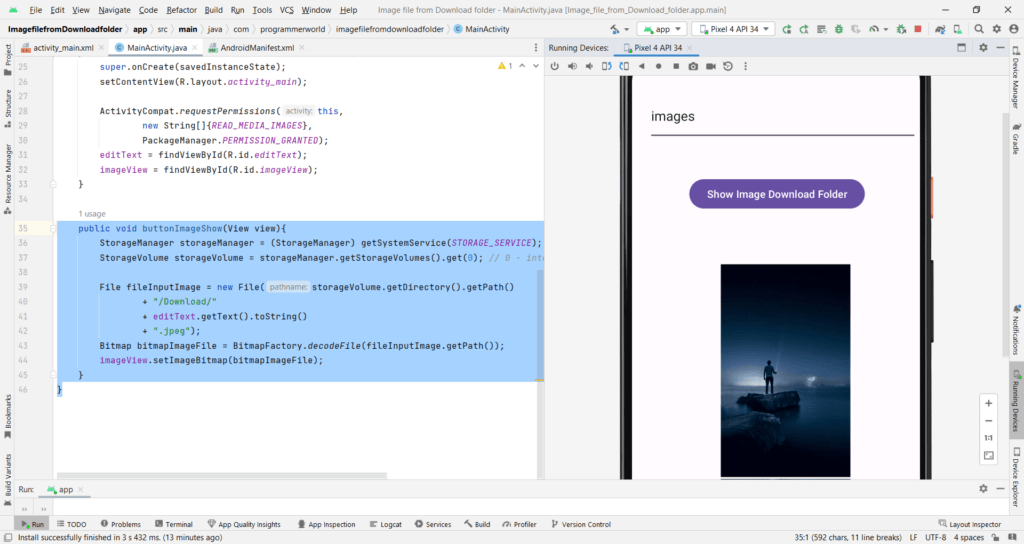