How to create your own complete Text Editor Android App to make the selected text bold, italics, underline or set the alignment?
This video shows the steps to create a text editor App in very simple approach.
In this tutorial, it shows how once can make the selected text in the Edit Text or multiline Text box as Bold, Italics or underline. It also shows how to remove any kind of formatting done in the text editor.
It further shows how one can align the text in the multiline text box to Left, Center or Right.
For setting the style of the selected text it uses Spannable APIs of Android. However, for setting the alignment, it uses setTextAlignment property (attribute) of the Multiline Text.
Part 2 of this tutorial can be referred at: https://youtu.be/AAmAeMnpSwQ
or
How to save edited text (bold, italic, underline, alignment) in SQLite database in your Android App?
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete Source Code:
package com.programmerworld.texteditorapp;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Typeface;
import android.os.Bundle;
import android.text.Spannable;
import android.text.SpannableStringBuilder;
import android.text.style.StyleSpan;
import android.text.style.UnderlineSpan;
import android.view.View;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
private EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editTextTextMultiLine);
}
public void buttonBold(View view){
Spannable spannableString = new SpannableStringBuilder(editText.getText());
spannableString.setSpan(new StyleSpan(Typeface.BOLD),
editText.getSelectionStart(),
editText.getSelectionEnd(),
0);
editText.setText(spannableString);
}
public void buttonItalics(View view){
Spannable spannableString = new SpannableStringBuilder(editText.getText());
spannableString.setSpan(new StyleSpan(Typeface.ITALIC),
editText.getSelectionStart(),
editText.getSelectionEnd(),
0);
editText.setText(spannableString);
}
public void buttonUnderline(View view){
Spannable spannableString = new SpannableStringBuilder(editText.getText());
spannableString.setSpan(new UnderlineSpan(),
editText.getSelectionStart(),
editText.getSelectionEnd(),
0);
editText.setText(spannableString);
}
public void buttonNoFormat(View view){
String stringText = editText.getText().toString();
editText.setText(stringText);
}
public void buttonAlignmentLeft(View view){
editText.setTextAlignment(View.TEXT_ALIGNMENT_TEXT_START);
Spannable spannableString = new SpannableStringBuilder(editText.getText());
editText.setText(spannableString);
}
public void buttonAlignmentCenter(View view){
editText.setTextAlignment(View.TEXT_ALIGNMENT_CENTER);
Spannable spannableString = new SpannableStringBuilder(editText.getText());
editText.setText(spannableString);
}
public void buttonAlignmentRight(View view){
editText.setTextAlignment(View.TEXT_ALIGNMENT_TEXT_END);
Spannable spannableString = new SpannableStringBuilder(editText.getText());
editText.setText(spannableString);
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editTextTextMultiLine"
android:layout_width="320dp"
android:layout_height="217dp"
android:layout_marginStart="47dp"
android:layout_marginTop="7dp"
android:ems="10"
android:gravity="start|top"
android:hint="@string/type_text_here"
android:inputType="textMultiLine"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button4"
android:autofillHints="" />
<Button
android:id="@+id/button"
android:layout_width="68dp"
android:layout_height="45dp"
android:layout_marginStart="22dp"
android:layout_marginTop="8dp"
android:onClick="buttonBold"
android:text="@string/bold"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="42dp"
android:layout_marginTop="8dp"
android:onClick="buttonItalics"
android:text="@string/italics"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="46dp"
android:layout_marginTop="10dp"
android:onClick="buttonUnderline"
android:text="@string/underline"
app:layout_constraintStart_toEndOf="@+id/button2"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="240dp"
android:layout_marginTop="10dp"
android:onClick="buttonNoFormat"
android:text="@string/no_formatting"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button3" />
<Button
android:id="@+id/button5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="22dp"
android:layout_marginTop="35dp"
android:onClick="buttonAlignmentLeft"
android:text="@string/left"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextTextMultiLine" />
<Button
android:id="@+id/button6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="35dp"
android:layout_marginTop="35dp"
android:onClick="buttonAlignmentCenter"
android:text="@string/center"
app:layout_constraintStart_toEndOf="@+id/button5"
app:layout_constraintTop_toBottomOf="@+id/editTextTextMultiLine" />
<Button
android:id="@+id/button7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="27dp"
android:layout_marginTop="33dp"
android:onClick="buttonAlignmentRight"
android:text="@string/right"
app:layout_constraintStart_toEndOf="@+id/button6"
app:layout_constraintTop_toBottomOf="@+id/editTextTextMultiLine" />
</androidx.constraintlayout.widget.ConstraintLayout>
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
google()
jcenter()
}
dependencies {
classpath "com.android.tools.build:gradle:4.0.1"
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
apply plugin: 'com.android.application'
android {
compileSdkVersion 29
buildToolsVersion "29.0.3"
defaultConfig {
applicationId "com.programmerworld.texteditorapp"
minSdkVersion 26
targetSdkVersion 29
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
implementation fileTree(dir: "libs", include: ["*.jar"])
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'androidx.constraintlayout:constraintlayout:2.0.1'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
}
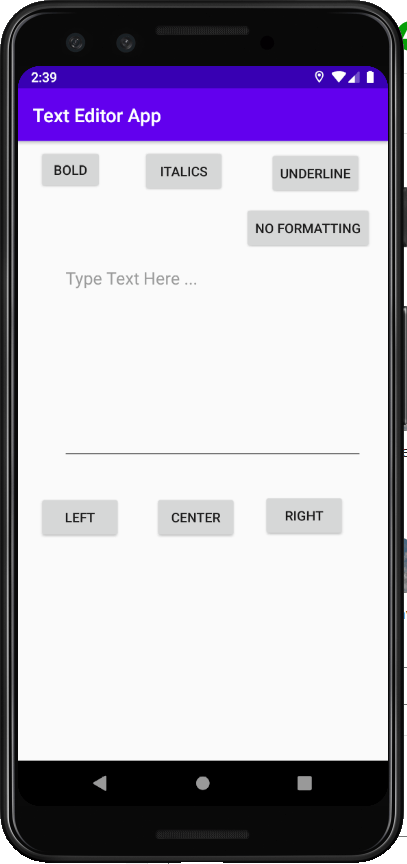
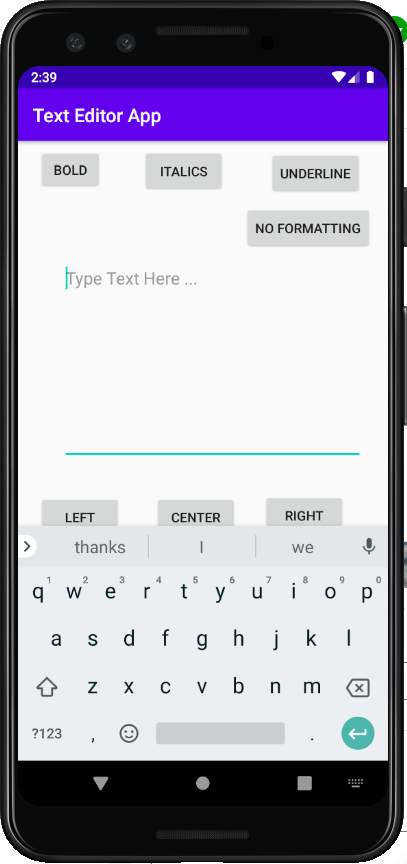
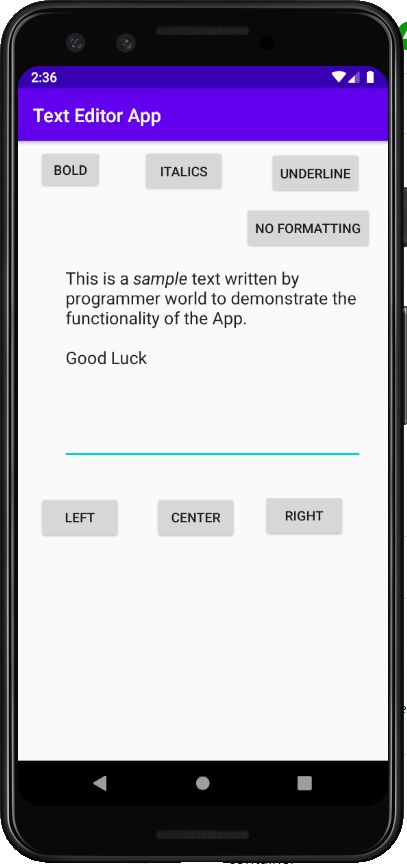
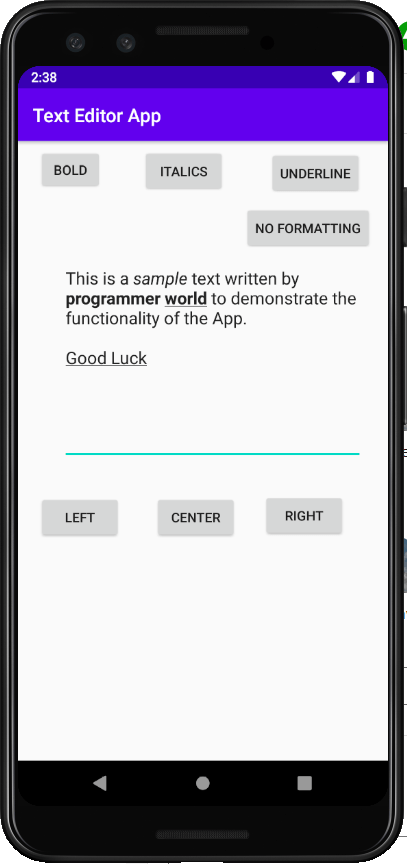
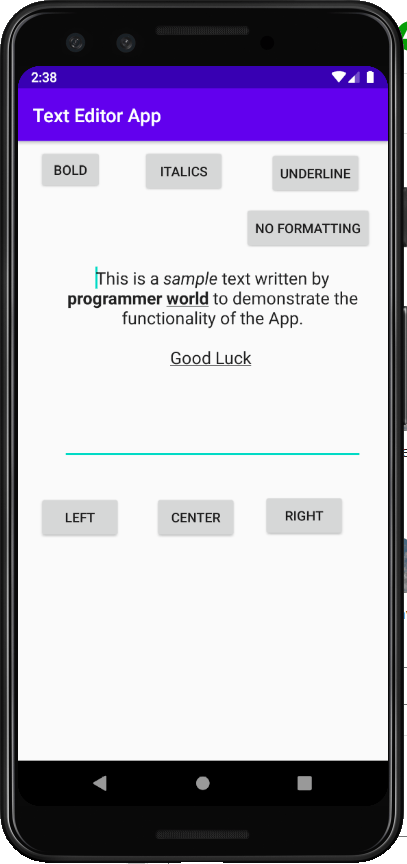
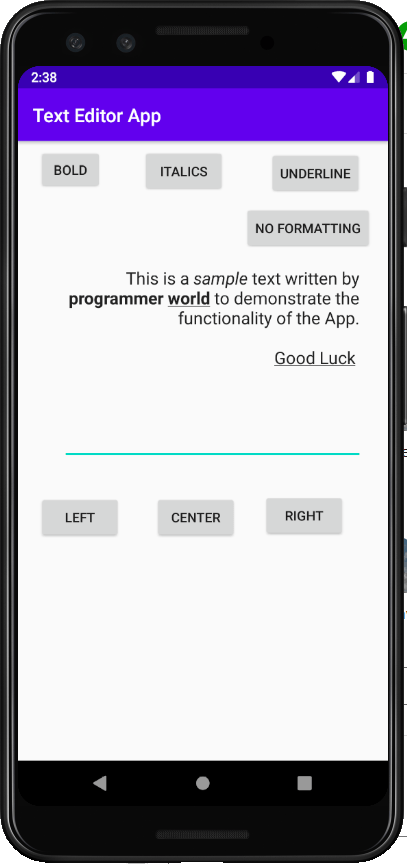
Sir, Please tell how to implement different functions also like word spacing, line spacing, font change and font size in the text editor app.
These functions can be implemented in different ways. Say for example, a font change option can be provided through some floating window and the respective choice of font for the text is set in the Java code.
However, thanks a lot for your suggestion. We will come up with a video with details on how to do implement more text editing functions?
Cheers
Programmer World
–
Thank you sir! For helping and eagerly waiting for your upcoming video upon this!
How do I implement the Save, SaveAs commands in the app
As far as I understand, one will have to save the text and the meta-data (the edit information) separately in SQL/SQLite database. Edit information will get lost on storing just the text in the database.
Cheers
Programmer World
–
Hot to save the bold,italic,underline,alignment,fomating data in room database. Please Help me.
Editing information, such as Bold, italic or underline, has to be separately saved in the database. In any database, while saving the text, it will not be able to capture and save the editing information. on its own. So, editing details can be saved as meta data information in the database.
For example, for saving Text Bold information used in below method:
public void buttonBold(View view){
Spannable spannableString = new SpannableStringBuilder(editText.getText());
spannableString.setSpan(new StyleSpan(Typeface.BOLD),
editText.getSelectionStart(),
editText.getSelectionEnd(),
0);
editText.setText(spannableString);
}
one should save below attributes in the database:
– StyleSpan(Typeface.BOLD)
– editText.getSelectionStart()
– editText.getSelectionEnd()
Hope above details help.
Cheers
Programmer World
–
Please refer to below page also for the complete code and steps on saving the editing information in a database:
https://programmerworld.co/android/how-to-save-edited-text-bold-italic-underline-alignment-in-sqlite-database-in-your-android-app/
Cheers
ProgrammerWorld
–