In this video it shows how one can customize the long press menu item list for the Android App.
When a user presses the icon of any App, Android shows up some of the options. This list of options is called shortcut info list and the dialog is shortcut manager.
In this video, it shows how one can customize this list to do certain actions based on the intent. In this video it uses two menu items. First is to open the programmerworld’s home page in a browser and the second is to open one of the subpages.
The code simply uses the ShortcutManager system service to do the customization of the long press list.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Source Code:
package com.programmerworld.longpressiconlist;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.content.pm.ShortcutInfo;
import android.content.pm.ShortcutManager;
import android.graphics.drawable.Icon;
import android.net.Uri;
import android.os.Bundle;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ShortcutManager shortcutManager = getSystemService(ShortcutManager.class);
ShortcutInfo shortcutInfo1 = new ShortcutInfo.Builder(this, "ID1")
.setShortLabel("Home Page")
.setLongLabel("Open Home Page")
.setIcon(Icon.createWithResource(this, R.drawable.ic_launcher_background))
.setIntent(new Intent(Intent.ACTION_VIEW, Uri.parse("https://programmerworld.co/")))
.build();
ShortcutInfo shortcutInfo2 = new ShortcutInfo.Builder(this, "ID2")
.setShortLabel("Android Page")
.setLongLabel("Open Android Page")
.setIcon(Icon.createWithResource(this, R.drawable.ic_launcher_foreground))
.setIntent(new Intent(Intent.ACTION_VIEW, Uri.parse("https://programmerworld.co/android/")))
.build();
List<ShortcutInfo> shortcutInfoList = new ArrayList<>();
shortcutInfoList.add(shortcutInfo1);
shortcutInfoList.add(shortcutInfo2);
shortcutManager.setDynamicShortcuts(shortcutInfoList);
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath "com.android.tools.build:gradle:7.0.3"
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.longpressiconlist">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.LongPressIconList">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
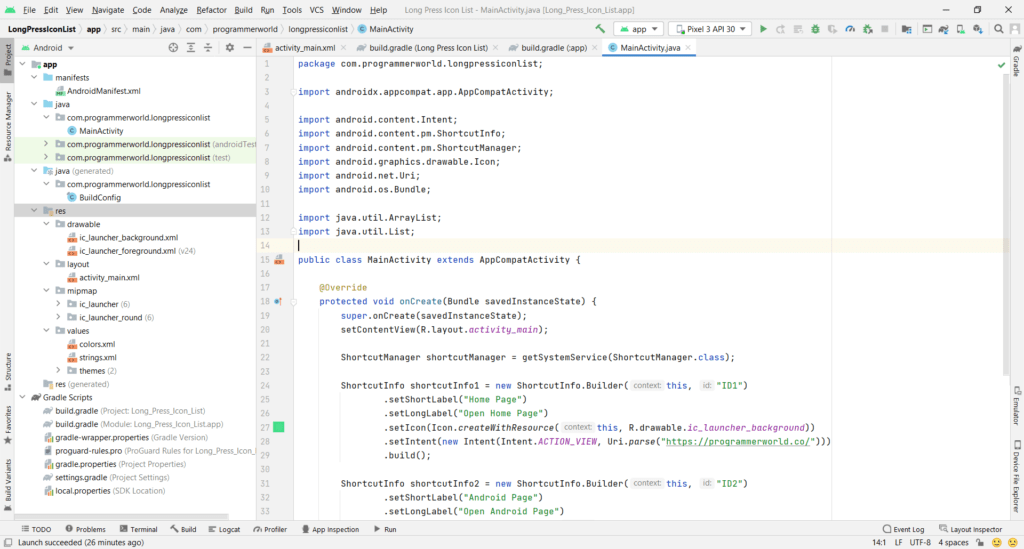
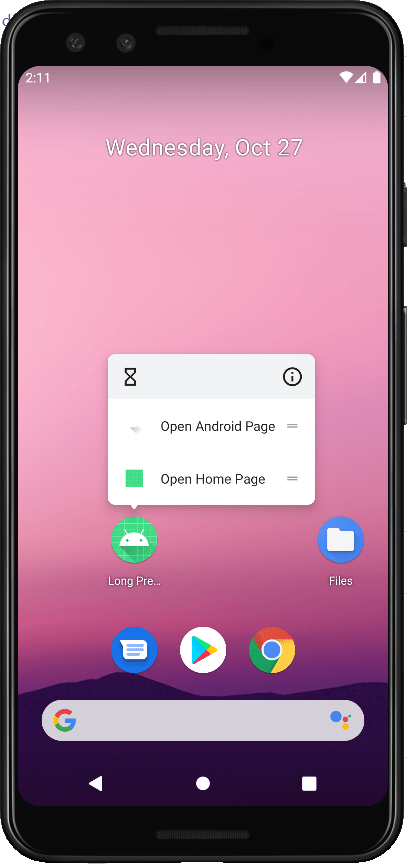
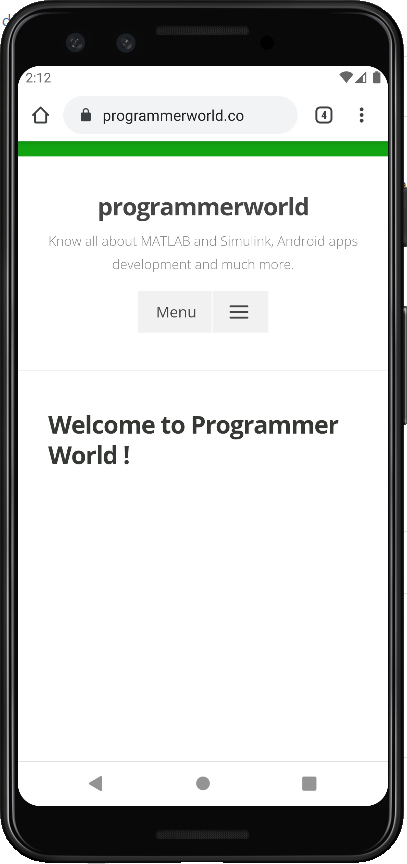
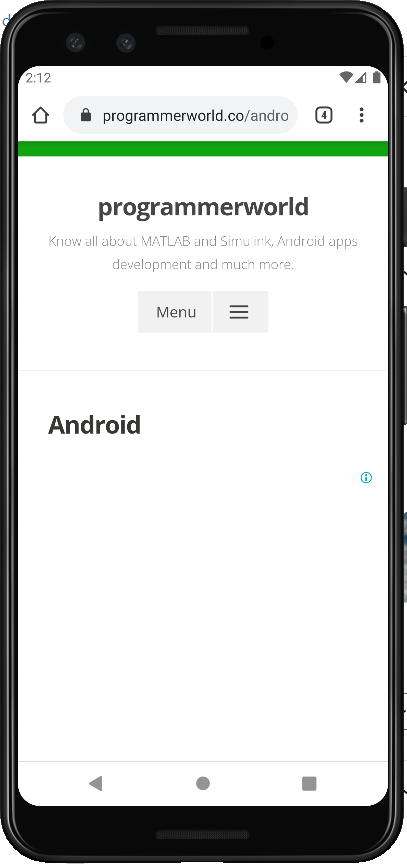
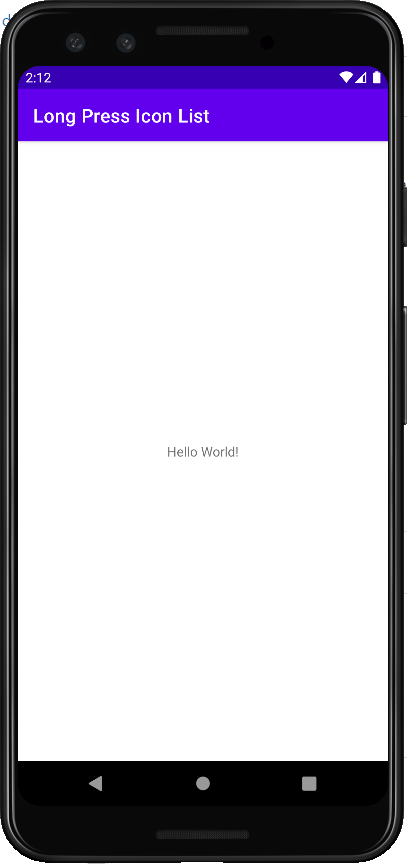