In this video it shows how to create a widget for your Android App. It shows the steps to develop the widget option for your Android App.
It shows the code to for widget layout and then Java class for widget option. Once the App is installed on the Android device, the widget for the app can be created for the app.
The App demo shown in this video is published in play store at: https://play.google.com/store/apps/details?id=com.programmerworld.timeclockwidget_programmerworld
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete code and details:
package com.programmerworld.timeclockwidget_programmerworld;
import android.os.Bundle;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.Spinner;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.Locale;
import java.util.TimeZone;
import java.util.Timer;
import java.util.TimerTask;
public class MainActivity extends AppCompatActivity {
private LinearLayout timeZoneLayout;
private Spinner timeZoneSpinner1, timeZoneSpinner2, timeZoneSpinner3, timeZoneSpinner4;
private Button showTimeZonesButton;
private List<String> selectedTimeZones;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
timeZoneLayout = findViewById(R.id.timeZoneLayout);
timeZoneSpinner1 = findViewById(R.id.timeZoneSpinner1);
timeZoneSpinner2 = findViewById(R.id.timeZoneSpinner2);
timeZoneSpinner3 = findViewById(R.id.timeZoneSpinner3);
timeZoneSpinner4 = findViewById(R.id.timeZoneSpinner4);
showTimeZonesButton = findViewById(R.id.showTimeZonesButton);
selectedTimeZones = new ArrayList<>();
setupSpinners();
showTimeZonesButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
selectedTimeZones.clear();
selectedTimeZones.addAll(Arrays.asList(
timeZoneSpinner1.getSelectedItem().toString(),
timeZoneSpinner2.getSelectedItem().toString(),
timeZoneSpinner3.getSelectedItem().toString(),
timeZoneSpinner4.getSelectedItem().toString()
));
timeZoneLayout.removeAllViews(); // Clear previous time views
for (String timeZoneId : selectedTimeZones) {
addTimeZone(timeZoneId);
}
updateTimes();
}
});
startClock();
return insets;
});
}
private void setupSpinners() {
String[] availableTimeZones = TimeZone.getAvailableIDs();
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, availableTimeZones);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
timeZoneSpinner1.setAdapter(adapter);
timeZoneSpinner2.setAdapter(adapter);
timeZoneSpinner3.setAdapter(adapter);
timeZoneSpinner4.setAdapter(adapter);
timeZoneSpinner1.setSelection(adapter.getPosition("Asia/Kolkata"));
timeZoneSpinner2.setSelection(adapter.getPosition("Europe/London"));
timeZoneSpinner3.setSelection(adapter.getPosition("America/New_York"));
timeZoneSpinner4.setSelection(adapter.getPosition("America/Los_Angeles"));
}
private void addTimeZone(String timeZoneId) {
TextView timeTextView = new TextView(this);
timeTextView.setTextSize(20);
timeTextView.setTag(timeZoneId);
timeZoneLayout.addView(timeTextView);
}
private void startClock() {
Timer timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
runOnUiThread(() -> updateTimes());
}
}, 0, 1000);
}
private void updateTimes() {
for (int i = 0; i < timeZoneLayout.getChildCount(); i++) {
TextView timeTextView = (TextView) timeZoneLayout.getChildAt(i);
String timeZoneId = (String) timeTextView.getTag();
SimpleDateFormat sdf = new SimpleDateFormat("hh:mm:ss a - z", Locale.getDefault());
sdf.setTimeZone(TimeZone.getTimeZone(timeZoneId));
String currentTime = sdf.format(new Date());
timeTextView.setText("\n" + timeZoneId + ": " + currentTime);
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/main"
android:padding="16dp">
<Spinner
android:id="@+id/timeZoneSpinner1"
android:layout_width="match_parent"
android:layout_height="50dp" />
<Spinner
android:id="@+id/timeZoneSpinner2"
android:layout_width="match_parent"
android:layout_height="50dp" />
<Spinner
android:id="@+id/timeZoneSpinner3"
android:layout_width="match_parent"
android:layout_height="50dp" />
<Spinner
android:id="@+id/timeZoneSpinner4"
android:layout_width="match_parent"
android:layout_height="50dp" />
<Button
android:id="@+id/showTimeZonesButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Selected Time Zones" />
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="16dp"
android:contentDescription="TODO">
<LinearLayout
android:id="@+id/timeZoneLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical" />
</ScrollView>
</LinearLayout>
package com.programmerworld.timeclockwidget_programmerworld;
import android.appwidget.AppWidgetManager;
import android.appwidget.AppWidgetProvider;
import android.content.Context;
import android.content.Intent;
import android.os.Handler;
import android.widget.RemoteViews;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import java.util.TimeZone;
public class TimeWidgetProvider extends AppWidgetProvider {
private final Handler handler = new Handler();
private Runnable updateRunnable;
@Override
public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
for (int appWidgetId : appWidgetIds) {
updateTimeWidget(context, appWidgetManager, appWidgetId);
}
startUpdateTimer(context, appWidgetManager, appWidgetIds);
}
private void updateTimeWidget(Context context, AppWidgetManager appWidgetManager, int appWidgetId) {
RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget_layout);
// Configure time zones to display
String[] timeZones = {"Asia/Kolkata", "Europe/London", "America/New_York", "America/Los_Angeles"};
for (int i = 0; i < timeZones.length; i++) {
String timeZoneId = timeZones[i];
SimpleDateFormat sdf = new SimpleDateFormat("hh:mm:ss a", Locale.getDefault());
sdf.setTimeZone(TimeZone.getTimeZone(timeZoneId));
String currentTime = sdf.format(new Date());
switch (i){
case 0:
views.setTextViewText(R.id.widgetTimeZone1, timeZoneId + ": " + currentTime);
break;
case 1:
views.setTextViewText(R.id.widgetTimeZone2, timeZoneId + ": " + currentTime);
break;
case 2:
views.setTextViewText(R.id.widgetTimeZone3, timeZoneId + ": " + currentTime);
break;
case 3:
views.setTextViewText(R.id.widgetTimeZone4, timeZoneId + ": " + currentTime);
break;
}
}
appWidgetManager.updateAppWidget(appWidgetId, views);
}
private void startUpdateTimer(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
if (updateRunnable == null) {
updateRunnable = new Runnable() {
@Override
public void run() {
onUpdate(context, appWidgetManager, appWidgetIds);
handler.postDelayed(this, 1000); // Update every second
}
};
handler.post(updateRunnable);
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="8dp"
android:background="@android:color/white">
<TextView
android:id="@+id/widgetTimeZone1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center|start"
android:text="Time Zone 1"
android:textColor="@android:color/black"
android:textSize="16sp" />
<TextView
android:id="@+id/widgetTimeZone2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Time Zone 2"
android:textSize="16sp"
android:textColor="@android:color/black"
android:gravity="center|start"/>
<TextView
android:id="@+id/widgetTimeZone3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Time Zone 3"
android:textSize="16sp"
android:textColor="@android:color/black"
android:gravity="center|start"/>
<TextView
android:id="@+id/widgetTimeZone4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Time Zone 4"
android:textSize="16sp"
android:textColor="@android:color/black"
android:gravity="center|start" />
<!-- Add more TextView elements as needed -->
</LinearLayout>
time_widget_info.xml
<?xml version="1.0" encoding="utf-8"?>
<appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android"
android:minWidth="250dp"
android:minHeight="50dp"
android:updatePeriodMillis="0"
android:initialLayout="@layout/widget_layout"
android:resizeMode="horizontal|vertical"
android:widgetCategory="home_screen" />
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/timeclockappicon"
android:label="@string/app_name"
android:roundIcon="@mipmap/timeclockappicon_round"
android:supportsRtl="true"
android:theme="@style/Theme.TimeClockWidgetProgrammerWorld"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<receiver android:name=".TimeWidgetProvider" android:exported="true">
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_UPDATE" />
</intent-filter>
<meta-data
android:name="android.appwidget.provider"
android:resource="@xml/time_widget_info" />
</receiver>
</application>
</manifest>
Screenshots:
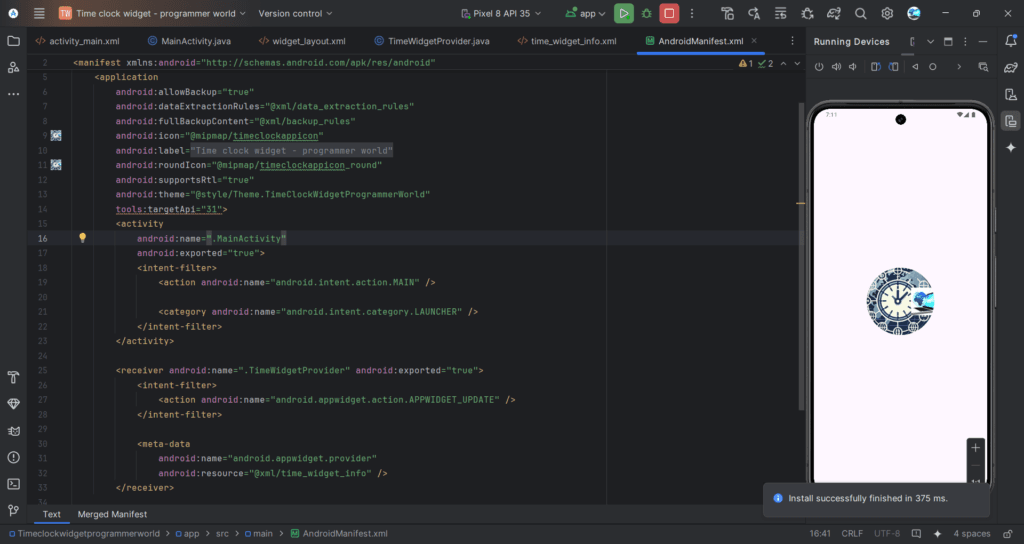
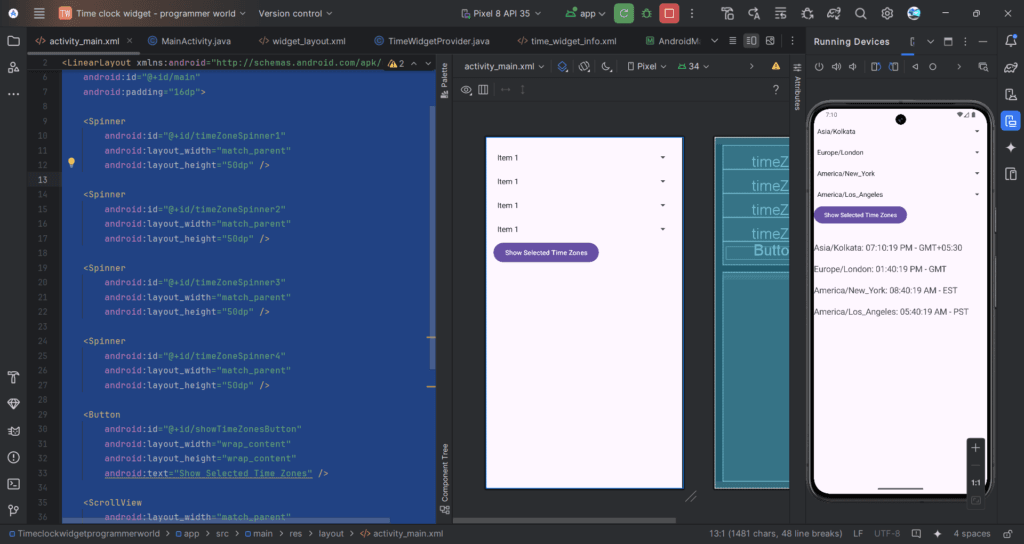
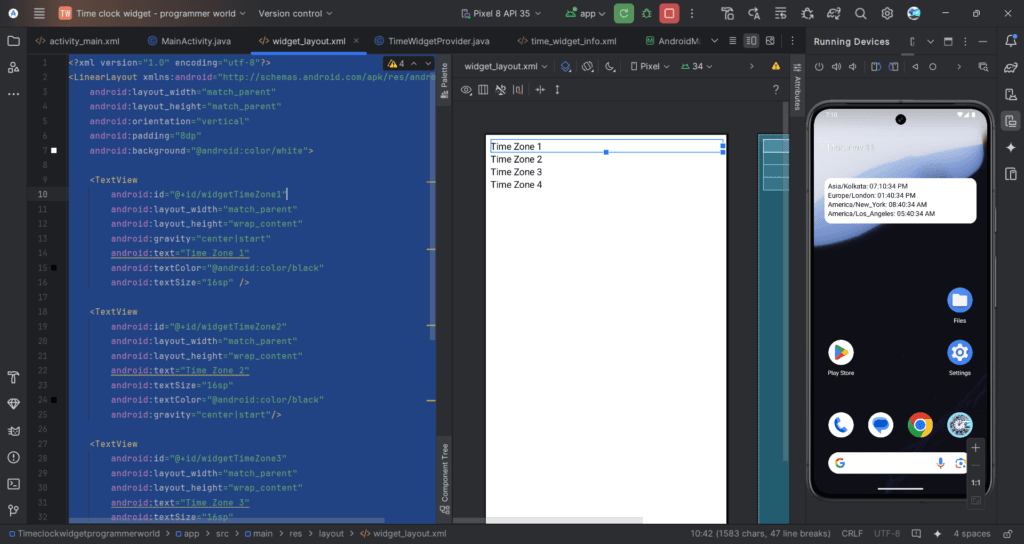
Application is available in Google’s play store:
https://play.google.com/store/apps/details?id=com.programmerworld.timeclockwidget_programmerworld
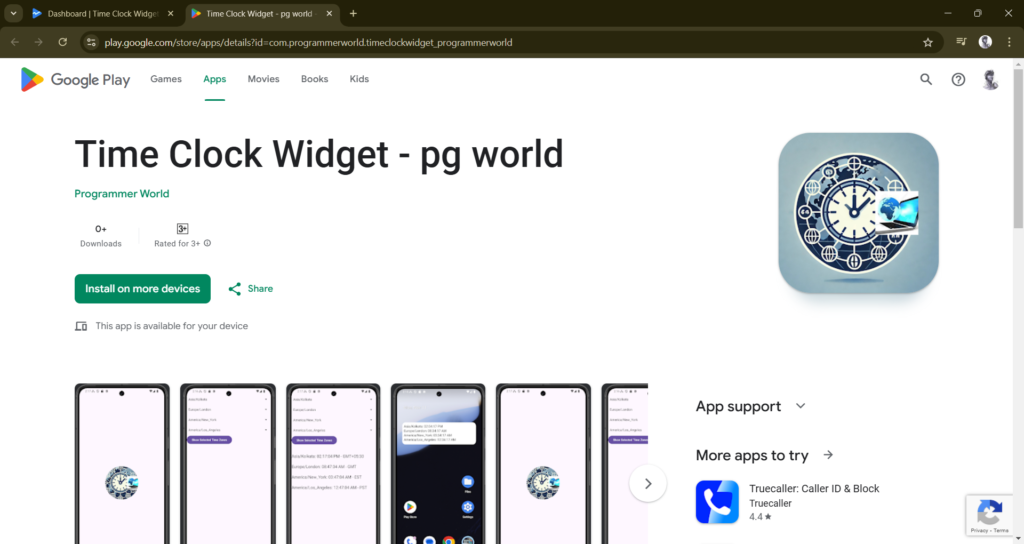