This video shows the steps to create an Excel file in your Android App. It uses third party library (not available as in-built APIs) from org.apache.poi.
It hard-codes the file name and sheet name in the code. However, that can be taken as an input from the user.
For the content it takes the input from the user through an Edit Text widget. Though it uses a single line edit text but same can be done using a multi-line plain text (Edit text) user input.
It creates the file in the emulator’s external storage. However, any location on the mobile device can be used to create the file.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Source Code
package com.programmerworld.excelfileapp;
import android.Manifest;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.os.Environment;
import android.view.View;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import java.io.File;
import java.io.FileOutputStream;
public class MainActivity extends AppCompatActivity {
private EditText editTextExcel;
private File filePath = new File(Environment.getExternalStorageDirectory() + "/Demo.xls");
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE}, PackageManager.PERMISSION_GRANTED);
editTextExcel = findViewById(R.id.editText);
}
public void buttonCreateExcel(View view){
HSSFWorkbook hssfWorkbook = new HSSFWorkbook();
HSSFSheet hssfSheet = hssfWorkbook.createSheet("Custom Sheet");
HSSFRow hssfRow = hssfSheet.createRow(0);
HSSFCell hssfCell = hssfRow.createCell(0);
hssfCell.setCellValue(editTextExcel.getText().toString());
try {
if (!filePath.exists()){
filePath.createNewFile();
}
FileOutputStream fileOutputStream= new FileOutputStream(filePath);
hssfWorkbook.write(fileOutputStream);
if (fileOutputStream!=null){
fileOutputStream.flush();
fileOutputStream.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
apply plugin: 'com.android.application'
android {
compileSdkVersion 29
buildToolsVersion "29.0.3"
defaultConfig {
applicationId "com.programmerworld.excelfileapp"
minSdkVersion 26
targetSdkVersion 29
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
implementation fileTree(dir: "libs", include: ["*.jar"])
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'androidx.constraintlayout:constraintlayout:2.0.2'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
implementation 'org.apache.poi:poi:3.17'
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.excelfileapp">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="128dp"
android:layout_marginTop="60dp"
android:onClick="buttonCreateExcel"
android:text="@string/create_excel"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="78dp"
android:layout_marginTop="57dp"
android:ems="10"
android:hint="@string/your_text_here"
android:inputType="textPersonName"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
android:autofillHints="" />
</androidx.constraintlayout.widget.ConstraintLayout>
<resources>
<string name="app_name">Excel File App</string>
<string name="create_excel">Create Excel</string>
<string name="your_text_here">Your text here ...</string>
</resources>
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
google()
jcenter()
}
dependencies {
classpath "com.android.tools.build:gradle:4.0.1"
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
App’s Output
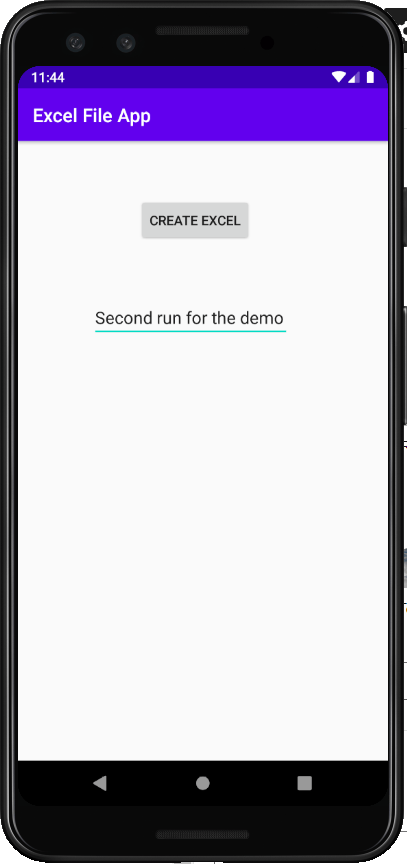
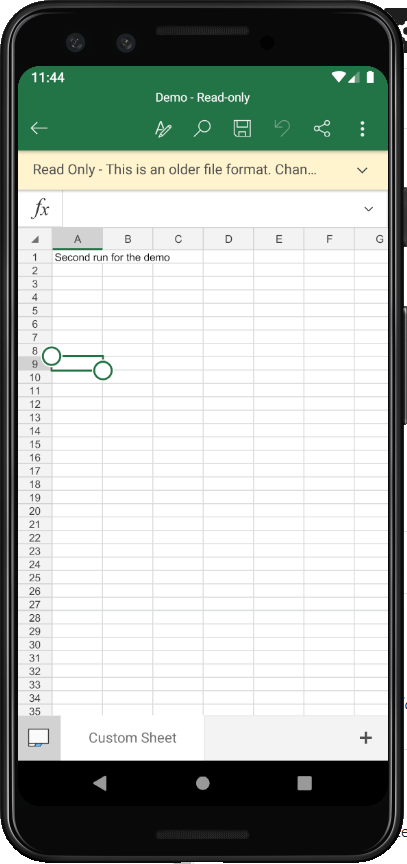
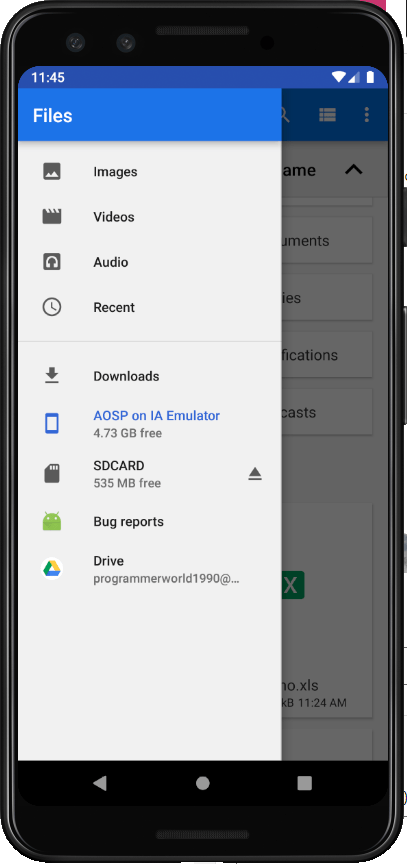
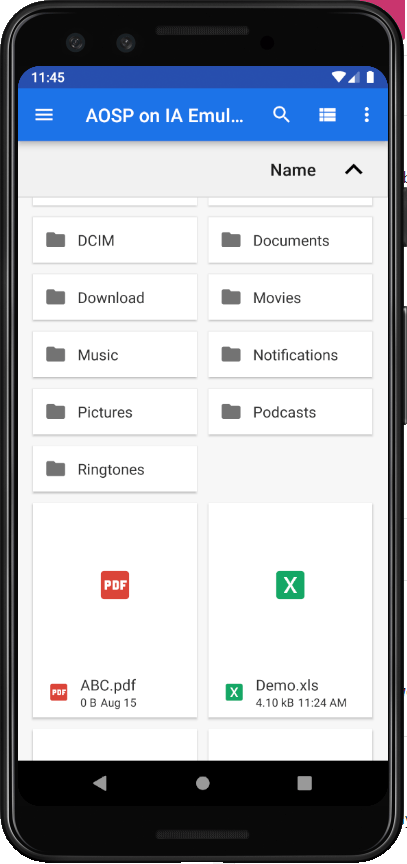
Hello sir, i’ve tried this code. same as you wrote, but for me its not working. on button click nothing happends. and if i use progress dialog and dismiss the progress dialog after hssfWorkbook.write(fileOutputStream);
it doesn’t dismiss. which means the code is not writing the file at all. and of course not output.
i’ve tried many more methods with jar libraries with XSSWorkbook but nothing is working for me.
i would very much appreciate if you could help me.
Thank you
Did you try to debug the code? Any error or exception is thrown?
I also hope you have implemented the required library in your gradle file. Also, have set the Onclick attribute of the button.
If all the above is done and still gives issue then please paste your code here. We will check.
Cheers
Programmer World
–
Wow thank you sir for replying so fast .
I’ve debug the code . The log says no such location or file name found .
But the code is trying to make new once it shouldn’t be a problem I think .
But still I changed the directory and the file name and also tired it by creating new folder for it .
Still not working.
Once again Thank you for your reply.
Then the issue is that the file path location chosen is not correct. I have used the below line in my code:
private File filePath = new File(Environment.getExternalStorageDirectory() + “/Demo.xls”);
But check whether your mobile or emulator has this path. Else use any other path which exists on your device.
I hope the above information helps.
Cheers
Programmer World
–
Thank you sir
hello sir I tried to create an xls file by using your steps mentioned above but it throws an IO exception : permission denied at this line:
filePath.createNewFile();
I had given all the required permissions in the manifests and java file but still stuck .
I would be very thankful if you help me
Thank you
Please check the below:
– You have given WRITE TO EXTERNAL STORAGE (WRITE_EXTERNAL_STORAGE) permission both in Java code and Manifest file.
– In the first time usage, App would ask from user to grant this permission. Please make sure user has granted this permission to the App.
– Also, please check that the file path (folder location) exists. Different phones may have different locations. So, ensure that the path you are using in code is valid.
The above checks should help in debugging the IO (input-output) exception you are getting. If the issue still persists then please comment here.
Cheers
Programmer World
–
Isn’t this function Environment.getExternalStorageDirectory() is sufficient for making an excel file? i had given permissions in both java code and manifest. but it is also showing a warning that ” WRITE_EXTERNAL_STORAGE no longer provides write access when targeting Android 10+”
please help sir 😐
Hello sir , thank you for your response.
I’ve corrected that issue by putting legacy storage true in manifest file .
Thank you very much for everything
Oh, good to know this. Good that you have found another solution.
Cheers
Programmer World
–
Android:requestLagecyExrernalStorage = “true”
In manifest application
For Android 10 (API level 29) onwards the External Storage related permissions have changed a bit. Now, one can directly contribute to a well-defined media collections such as MediaStore.Downloads without requesting any storage-related permissions. (This is also mentioned in the android developer portal).
Alternatively, one can also write to the App specific data without requiring any Storage privilege. Use “getExternalFilesDir” API for this. An example of this can be found in my below webpage:
https://programmerworld.co/android/how-to-create-microsoft-word-document-docx-file-from-your-android-app/
Cheers
Programmer World
–
thank you sir its creating an excel sheet now by using that and sorry for thanking you late.
THANK YOU SIR!!!
Hi everyone,
I solve the problem of no action botton modify the code as follow:
private File filePath = new File(Environment.getExternalStorageDirectory().toString() + “/Demo.xls”);
Thanks.
Yes, explicitly enforcing the path to string datatype using the “toString” will help.
Cheers
Programmer World
–
Hi bro,
Thanks for your guide.
I follow and create excel file success.
But have a small problem, I see file created on device but when attach cable USB to PC (Window)
it not show this file.
(with text file or other file, i can see and view from my PC).
Do you have any solution for this problem?
Thanks so much!
That’s weird. I assume you are able to see the other files in the same folder. To be honest I don’t know, but can propose few options:
– Try copying the excel file to some other location/ folder. My guess is that folder may have certain access restriction.
– Try renaming the file or changing the type of file (extension) on your phone and see if it is visible from your PC. In this case there might be some issue with a particular file name or file type.
Please let us know if you could find any solution.
Cheers
Programmer World
–
what is meaning of this error
/Choreographer: Skipped 11 frames! The application may be doing too much work on its main thread.
This error comes when the App is trying to do lots of stuffs and processes and the device (phone) is not able to handle them. So, the OS skips some of the frames. For a small number, like less than 100, it can ignored during the development phase of the App. However, if the frames skipped is a fairly large number then one should either check the resource of the device (RAM, Processor) or debug the code and make the code more optimized. One quick way to optimize the code processing is to push the unnecessary task into child threads and keep the main thread light.
Hope above helps.
Cheers
Programmer World
–
but if we add new name in app it stores the name to the first name instead of using another row , and the first name hides …….. is there any solution????
I think if you want to write the contents in multiple lines, then you may have to create new rows and cell in the workbook sheet. So, please keep repeating below code (iteratively) for each new line of the text needed to be written in the excel.
HSSFRow hssfRow = hssfSheet.createRow(0);
HSSFCell hssfCell = hssfRow.createCell(0);
hssfCell.setCellValue(editTextExcel.getText().toString());
Hope the above helps.
Cheers
Programmer World
–
i have tried this :
Row row = sheet1.createRow(i);
Cell cell = row.createCell(0);
Cell cell1 = row.createCell(1);
cell.setCellValue(name.getText().toString());
cell1.setCellValue(phone.getText().toString());
it creates new row after first entry but when we enter second data it erases first data and new data save on another row… i want that previous rows data also to be saved
is there any solution???
Instead, can you try below and check if it works:
Row row = sheet1.createRow(0);
Row row1 = sheet1.createRow(1);
Cell cell = row.createCell(0);
Cell cell1 = row1.createCell(0);
cell.setCellValue(name.getText().toString());
cell1.setCellValue(phone.getText().toString());
Cheers
Programmer World
–
Hello the I am using a slightly modified version of the code and it is not actually creating the excel file any help would be wonderful
What is the error or issue you are observing?
Would it be possible to share your code here?
Cheers
Programmer World
–