This video shows the steps to access the volume level of different stream types of your phone and set it on seekbar in your Android App.
It also uses textview to display the volume levels in integer form.
For setting the volumes from your App, please refer to the below page:
https://programmerworld.co/android/how-to-adjust-the-volume-of-your-android-phone-programmatically-from-your-app-source-code/
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code:
package com.programmerworld.accessvolumelevel;
import androidx.appcompat.app.AppCompatActivity;
import android.media.AudioManager;
import android.os.Bundle;
import android.view.View;
import android.widget.SeekBar;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private AudioManager audioManager;
private TextView textView;
private SeekBar seekBarMusic, seekBarAlarm, seekBarCalls;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
audioManager = (AudioManager) this.getSystemService(AUDIO_SERVICE);
textView = findViewById(R.id.textView);
seekBarAlarm = findViewById(R.id.seekBarAlarm);
seekBarCalls = findViewById(R.id.seekBarCalls);
seekBarMusic = findViewById(R.id.seekBarMusic);
seekBarMusic.setMax(audioManager.getStreamMaxVolume(AudioManager.STREAM_MUSIC));
seekBarAlarm.setMax(audioManager.getStreamMaxVolume(AudioManager.STREAM_ALARM));
seekBarCalls.setMax(audioManager.getStreamMaxVolume(AudioManager.STREAM_VOICE_CALL));
}
public void buttonGetVolume(View view){
seekBarMusic.setProgress(audioManager.getStreamVolume(AudioManager.STREAM_MUSIC));
seekBarAlarm.setProgress(audioManager.getStreamVolume(AudioManager.STREAM_ALARM));
seekBarCalls.setProgress(audioManager.getStreamVolume(AudioManager.STREAM_VOICE_CALL));
textView.setText("Music: " + Integer.toString(audioManager.getStreamVolume(AudioManager.STREAM_MUSIC))
+ ", Alarm: " + Integer.toString(audioManager.getStreamVolume(AudioManager.STREAM_ALARM))
+ ", Calls: " + Integer.toString(audioManager.getStreamVolume(AudioManager.STREAM_VOICE_CALL)) );
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.228" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="110dp"
android:layout_marginTop="50dp"
android:onClick="buttonGetVolume"
android:text="Get Volume Levels"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<SeekBar
android:id="@+id/seekBarMusic"
android:layout_width="275dp"
android:layout_height="83dp"
android:layout_marginStart="68dp"
android:layout_marginTop="20dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<SeekBar
android:id="@+id/seekBarAlarm"
android:layout_width="270dp"
android:layout_height="85dp"
android:layout_marginStart="72dp"
android:layout_marginTop="12dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBarMusic" />
<SeekBar
android:id="@+id/seekBarCalls"
android:layout_width="278dp"
android:layout_height="93dp"
android:layout_marginStart="68dp"
android:layout_marginTop="21dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBarAlarm" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.accessvolumelevel">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.AccessVolumeLevel">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
plugins {
id 'com.android.application'
}
android {
compileSdk 31
defaultConfig {
applicationId "com.programmerworld.accessvolumelevel"
minSdk 31
targetSdk 31
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.4.1'
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
}
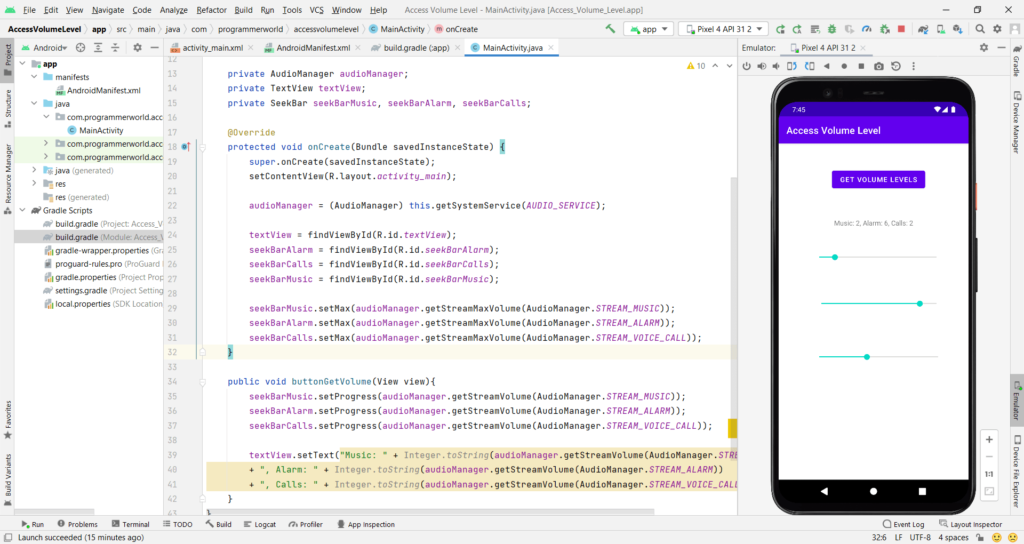