In this video it shows how to create a password locked pdf from your Android App. It uses PSPDFKit libraries for the same. Please note, there is no ‘setPassword’ or similar api available to set password for the pdf file in the android.graphics.pdf.PdfDocument’s PdfDocument class as of API 32.
It also reuses part of code from below page which shows how to create a PDF file using android graphics’ PDFDocument: https://programmerworld.co/android/how-to-create-and-read-a-pdf-file-in-your-android-11-app/
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.pdfpasswordlockapp;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.pm.PackageManager;
import android.graphics.Paint;
import android.graphics.pdf.PdfDocument;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.pspdfkit.document.DocumentSaveOptions;
import com.pspdfkit.document.PdfDocumentLoader;
import com.pspdfkit.document.processor.PdfProcessor;
import com.pspdfkit.document.processor.PdfProcessorTask;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Calendar;
public class MainActivity extends AppCompatActivity {
private EditText editTextPassword;
private File filePDF_initial, filePDF_finalOutput;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.READ_EXTERNAL_STORAGE,
Manifest.permission.WRITE_EXTERNAL_STORAGE},
PackageManager.PERMISSION_GRANTED);
editTextPassword = findViewById(R.id.editTextTextPassword);
filePDF_initial = new File(this.getExternalFilesDir(Environment.DIRECTORY_DOCUMENTS),
"Initial "+Calendar.getInstance().getTime().toString()+".pdf");
filePDF_finalOutput = new File(this.getExternalFilesDir(Environment.DIRECTORY_DOCUMENTS),
"PasswordLocked "+Calendar.getInstance().getTime().toString()+".pdf");
}
public void buttonCreatePasswordLockPDF(View view){
PdfDocument pdfDocument = new PdfDocument();
PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder(300, 600, 1).create();
PdfDocument.Page page = pdfDocument.startPage(pageInfo);
Paint paint = new Paint();
String stringPDF1 = Calendar.getInstance().getTime().toString();
String stringPDF2 = "Hello Programmer World";
page.getCanvas().drawText(stringPDF1,10,100, paint);
page.getCanvas().drawText(stringPDF2,10,160, paint);
pdfDocument.finishPage(page);
try {
pdfDocument.writeTo(new FileOutputStream(filePDF_initial));
}
catch (Exception e){
e.printStackTrace();
}
pdfDocument.close();
// Password mechanism below:
try {
com.pspdfkit.document.PdfDocument psPdfDocument = PdfDocumentLoader.openDocument(this,
Uri.fromFile(filePDF_initial));
PdfProcessorTask pdfProcessorTask = PdfProcessorTask.fromDocument(psPdfDocument);
DocumentSaveOptions documentSaveOptions = psPdfDocument.getDefaultDocumentSaveOptions();
documentSaveOptions.setPassword(editTextPassword.getText().toString());
PdfProcessor.processDocument(pdfProcessorTask,
filePDF_finalOutput,
documentSaveOptions);
Toast.makeText(this,
"PDF Created Successfully",
Toast.LENGTH_LONG).show();
} catch (IOException e) {
e.printStackTrace();
Toast.makeText(this,
"PDF Creation Failed",
Toast.LENGTH_LONG).show();
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="64dp"
android:layout_marginTop="196dp"
android:onClick="buttonCreatePasswordLockPDF"
android:text="Create Password Locked PDF"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editTextTextPassword"
android:layout_width="240dp"
android:layout_height="65dp"
android:layout_marginStart="85dp"
android:layout_marginTop="65dp"
android:ems="10"
android:hint="Enter Password Here ..."
android:inputType="textPassword"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.PDFPasswordLockApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data
android:name="android.app.lib_name"
android:value="" />
</activity>
</application>
</manifest>
pluginManagement {
repositories {
gradlePluginPortal()
google()
mavenCentral()
}
}
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
maven {
url 'https://customers.pspdfkit.com/maven/'
}
}
}
rootProject.name = "PDF Password Lock App"
include ':app'
plugins {
id 'com.android.application'
}
android {
namespace 'com.programmerworld.pdfpasswordlockapp'
compileSdk 32
defaultConfig {
applicationId "com.programmerworld.pdfpasswordlockapp"
minSdk 32
targetSdk 32
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.5.1'
implementation 'com.google.android.material:material:1.6.1'
implementation 'androidx.constraintlayout:constraintlayout:2.1.4'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
implementation 'com.pspdfkit:pspdfkit:8.4.0'
}
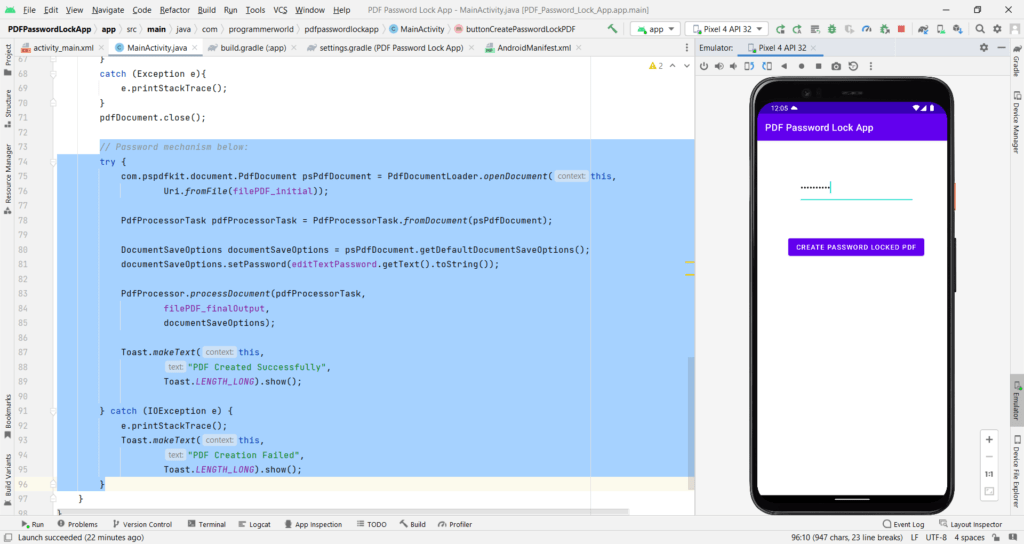
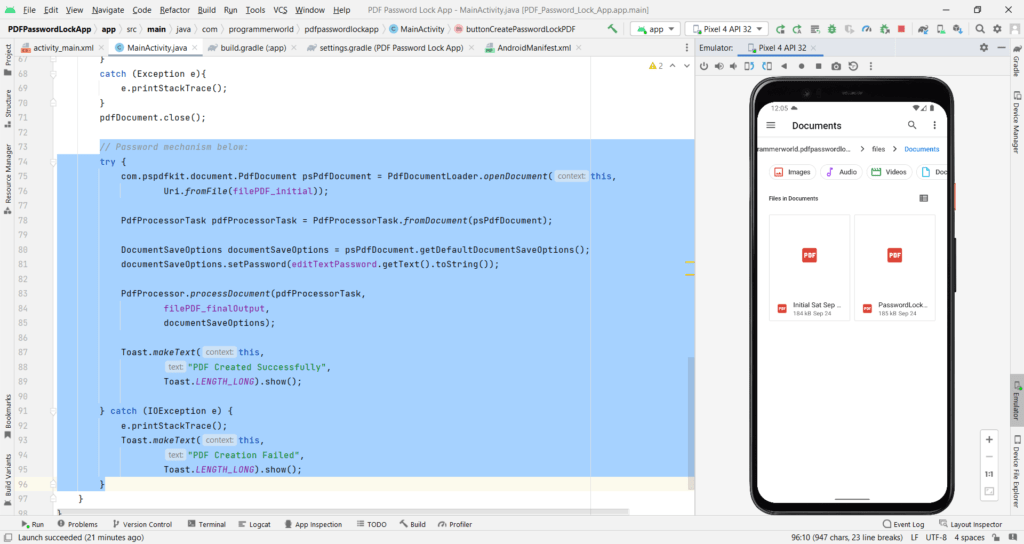
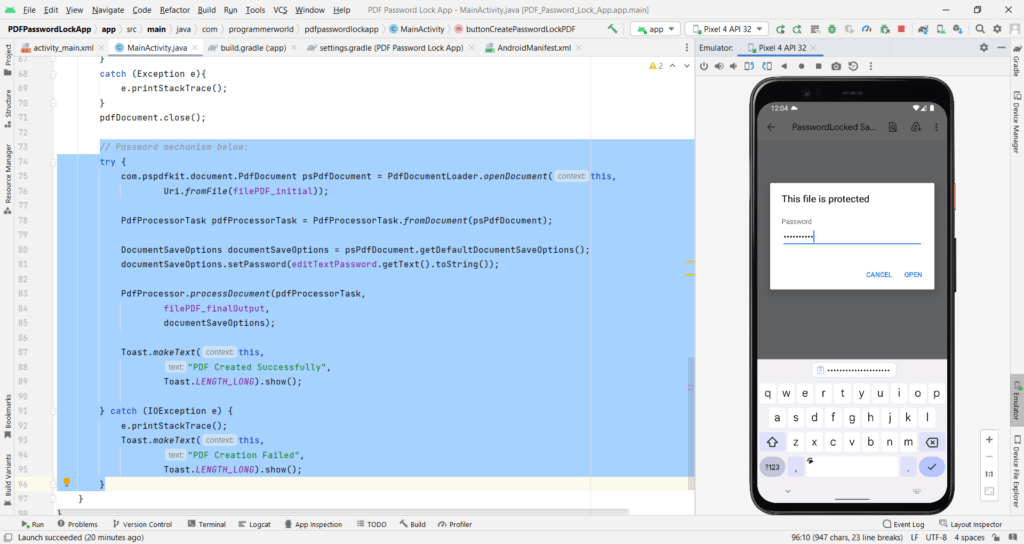
On using the free/ evaluation version of the PSPDFKit library, it may embed evaluation text in the pdf as seen in the image.
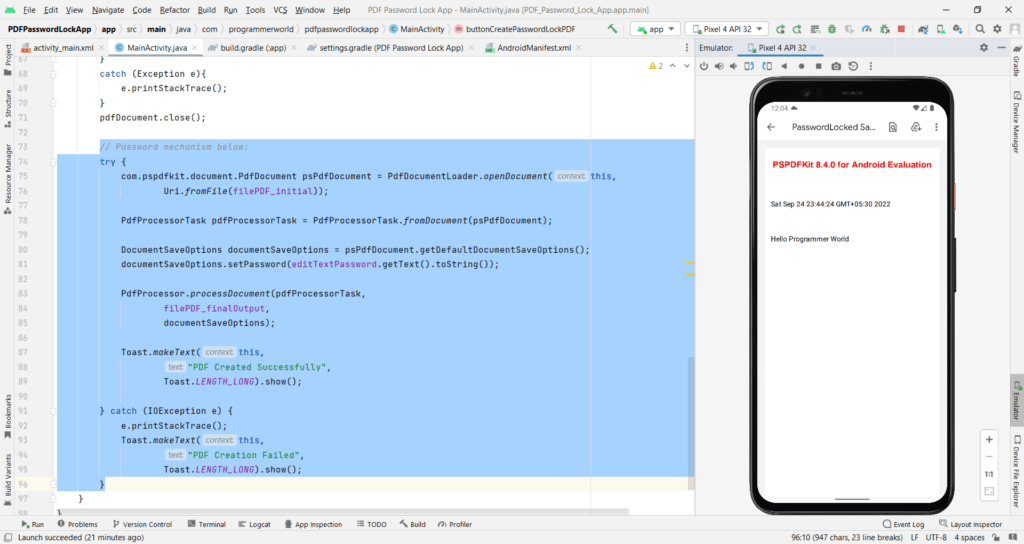