Steps in this session:
1. Create a simple App
2. Build normal APK (non-obfuscated)
3. Build Obfuscated APK
In this video it shows the source code in the APK file for 3 types of APKs built:
1. Debug variant
2. Release non-obfuscated variant
3. Release obfuscated
In this video it refers to the below pages:
– Android developer page: https://developer.android.com/build/shrink-code#kts
– Programmer World page: https://programmerworld.co/android/how-to-get-source-code-from-apk-file-of-an-android-app-api-34android-14/
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Details:
Gradle setting:
buildTypes {
release {
isMinifyEnabled = true
isShrinkResources = true
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
Complete gradle code:
plugins {
id("com.android.application")
}
android {
namespace = "com.programmerworld.obfuscatedcode"
compileSdk = 34
defaultConfig {
applicationId = "com.programmerworld.obfuscatedcode"
minSdk = 34
targetSdk = 34
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = true
isShrinkResources = true
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
}
dependencies {
implementation("androidx.appcompat:appcompat:1.6.1")
implementation("com.google.android.material:material:1.9.0")
implementation("androidx.constraintlayout:constraintlayout:2.1.4")
testImplementation("junit:junit:4.13.2")
androidTestImplementation("androidx.test.ext:junit:1.1.5")
androidTestImplementation("androidx.test.espresso:espresso-core:3.5.1")
}
Java code of the App:
package com.programmerworld.obfuscatedcode;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
}
public void buttonSet(View view){
textView.setText("This is programmer world App");
}
public void buttonClear(View view){
textView.setText("Hello Programmer World!!!");
}
}
XML layout file:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/t"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="38dp"
android:layout_marginTop="55dp"
android:onClick="buttonSet"
android:text="set"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="87dp"
android:layout_marginTop="50dp"
android:onClick="buttonClear"
android:text="Clear"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
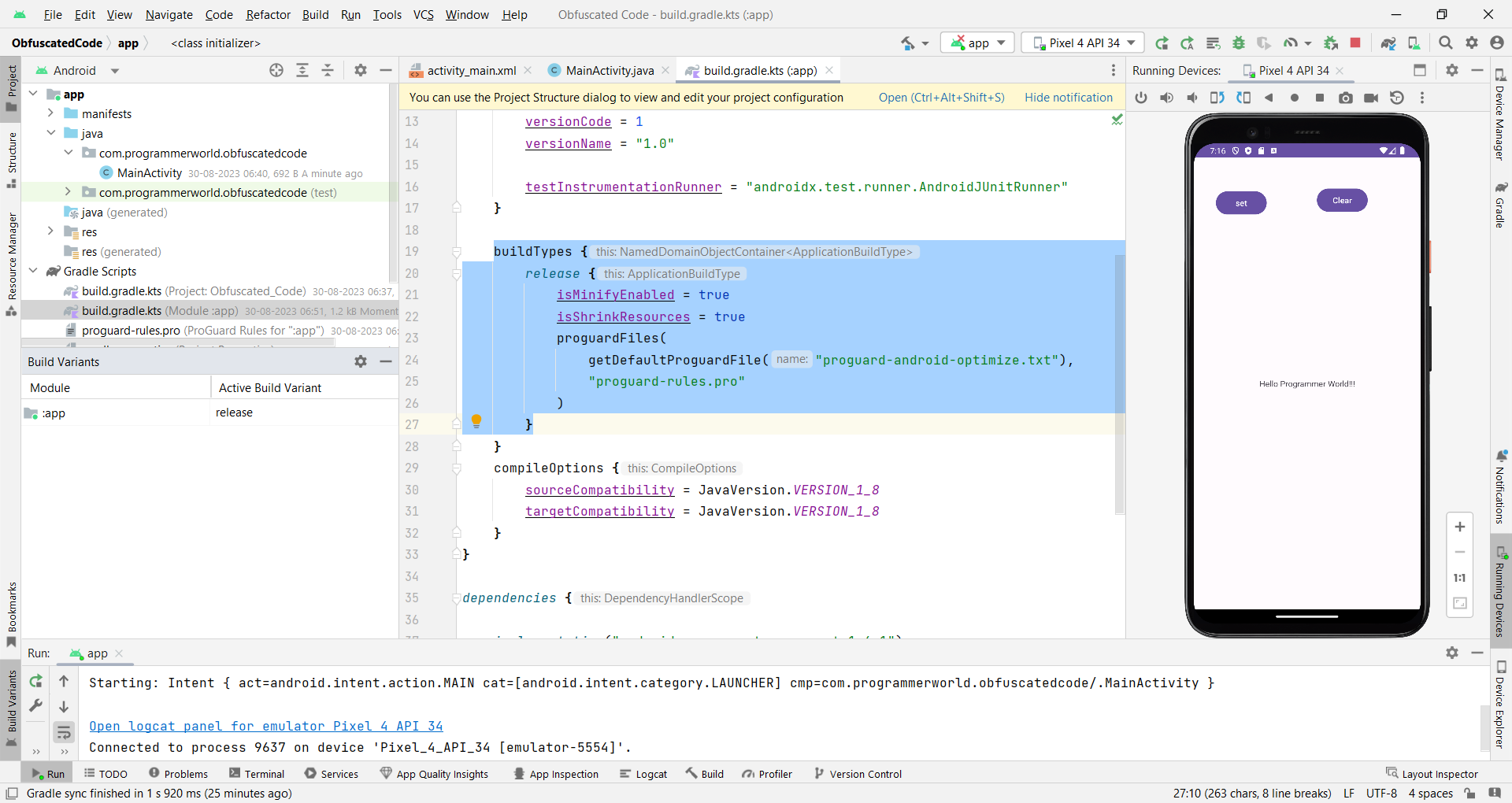
Debug – non-obfuscated code:
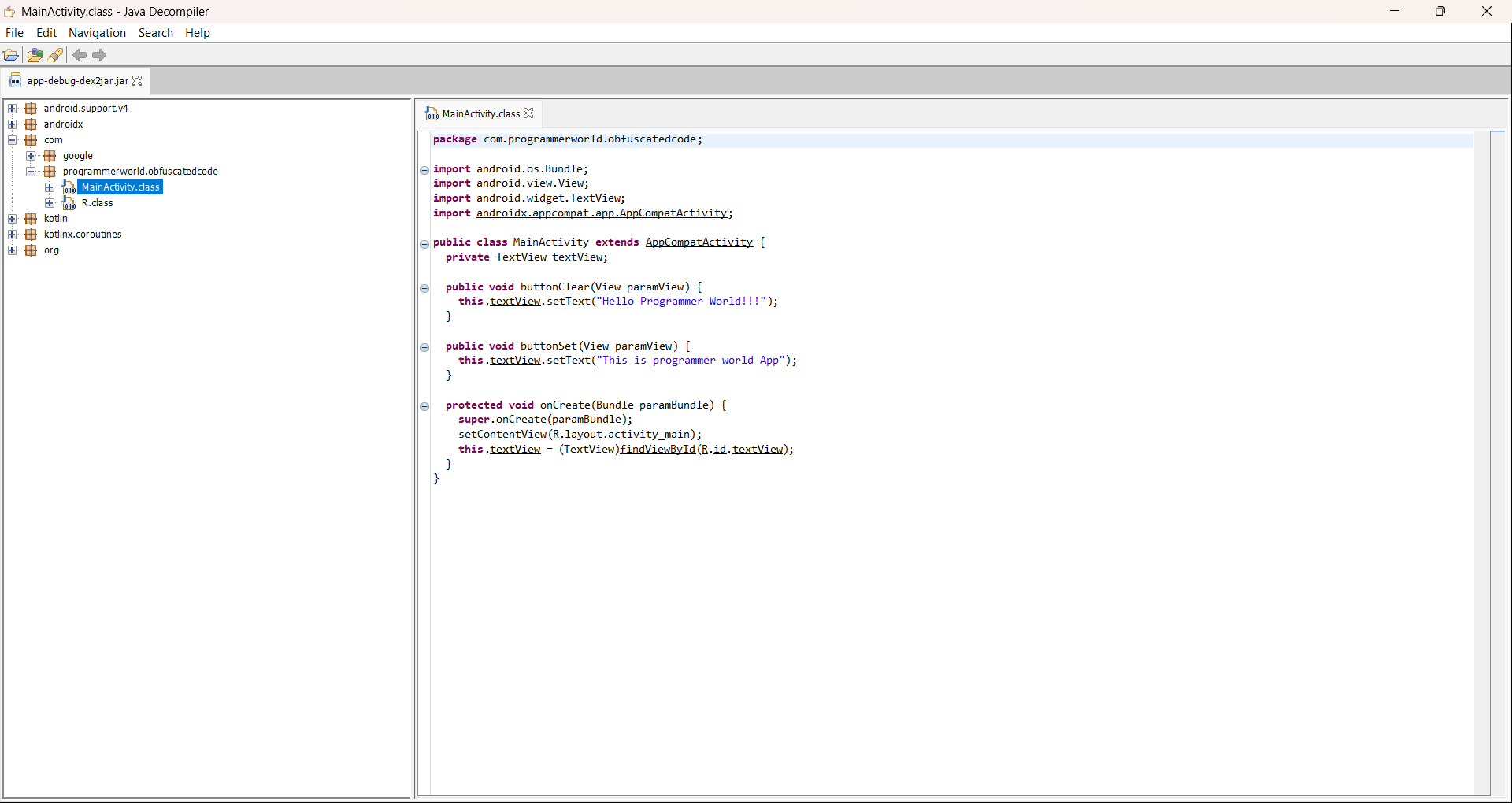
Release – non-obfuscated code:
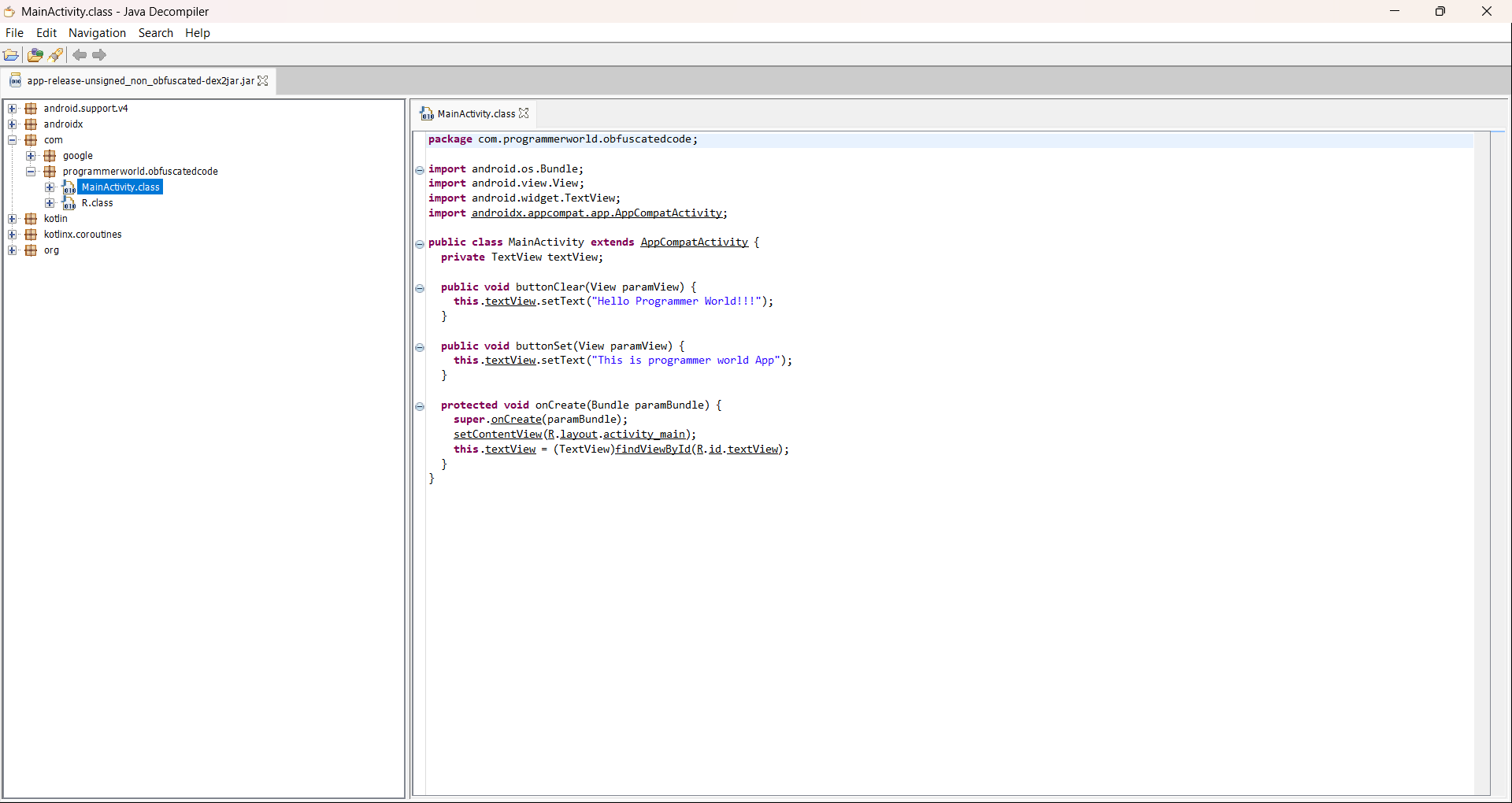
Release – obfuscated code:
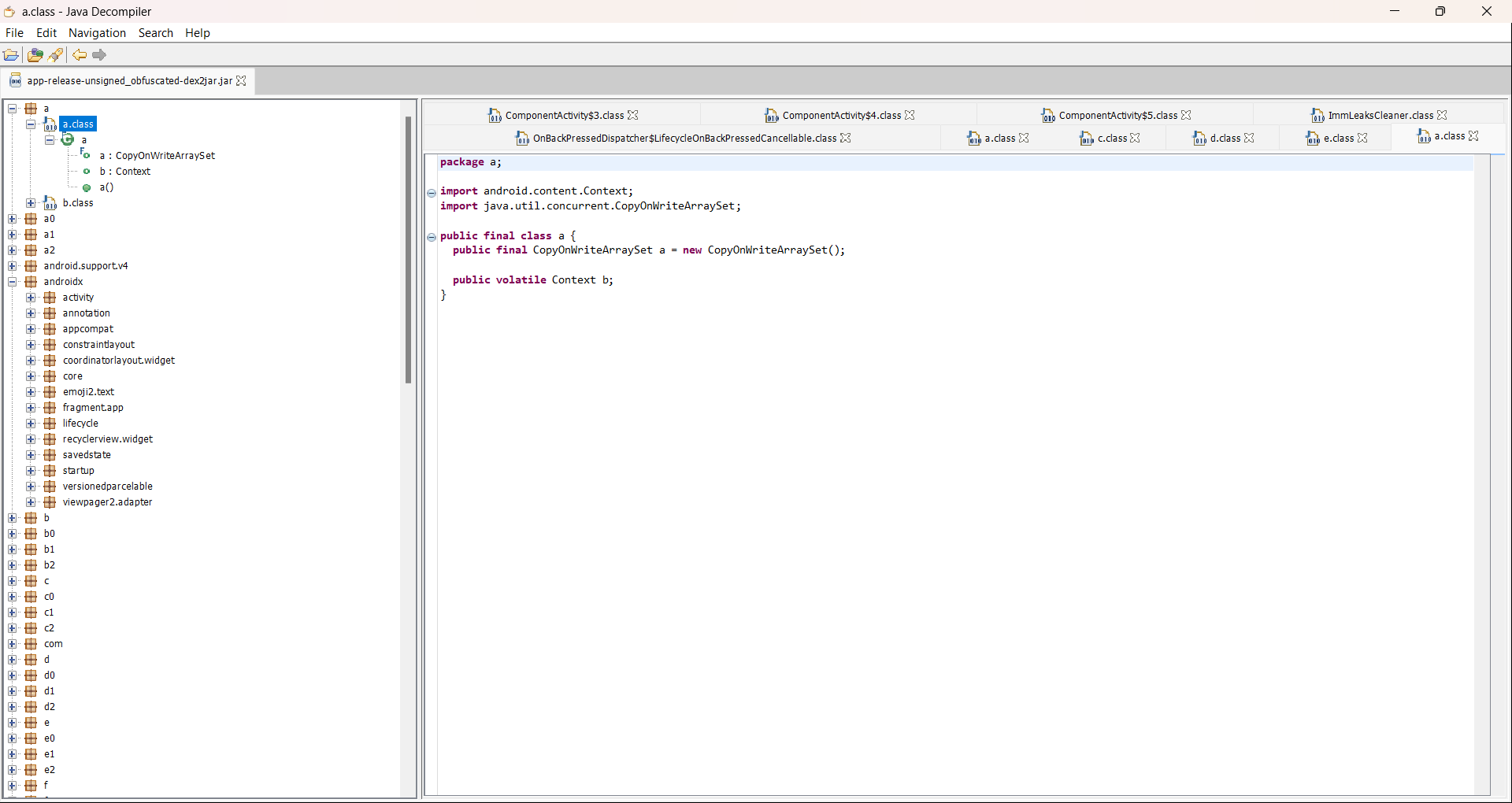
APK to JAR conversion command:
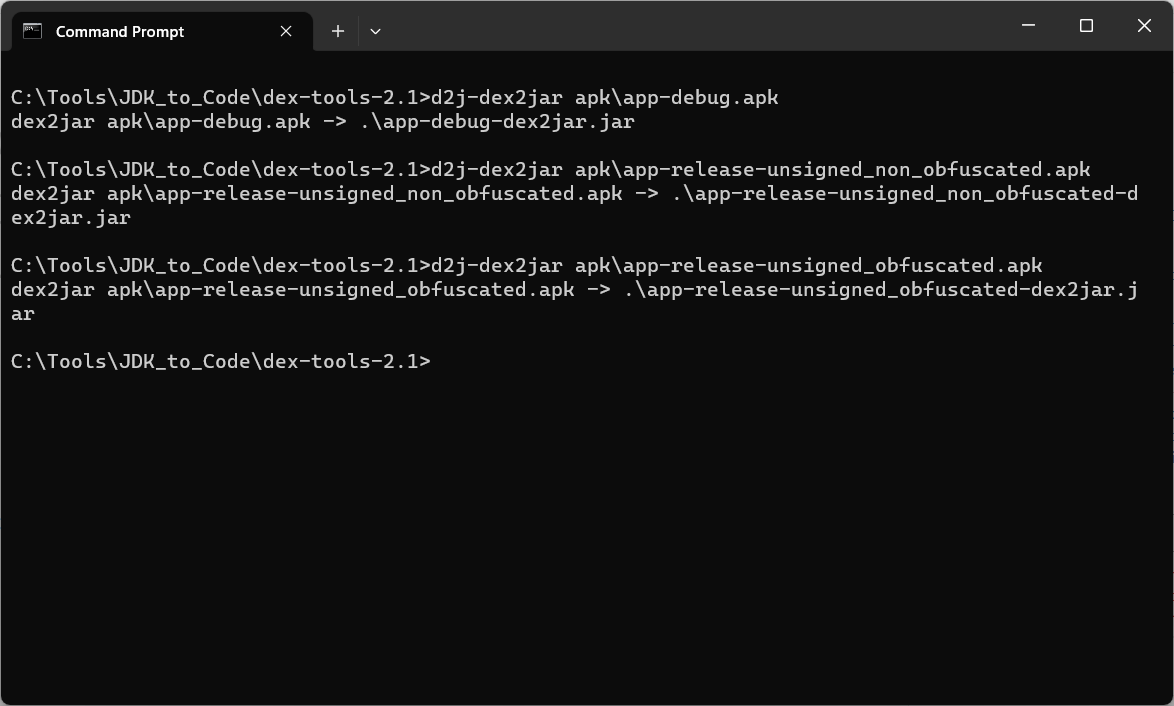
C:\Tools\JDK_to_Code\dex-tools-2.1>d2j-dex2jar apk\app-debug.apk
dex2jar apk\app-debug.apk -> .\app-debug-dex2jar.jar
C:\Tools\JDK_to_Code\dex-tools-2.1>d2j-dex2jar apk\app-release-unsigned_non_obfuscated.apk
dex2jar apk\app-release-unsigned_non_obfuscated.apk -> .\app-release-unsigned_non_obfuscated-dex2jar.jar
C:\Tools\JDK_to_Code\dex-tools-2.1>d2j-dex2jar apk\app-release-unsigned_obfuscated.apk
dex2jar apk\app-release-unsigned_obfuscated.apk -> .\app-release-unsigned_obfuscated-dex2jar.jar
C:\Tools\JDK_to_Code\dex-tools-2.1>
Tools used in this session:
Dex2Jar:
https://drive.google.com/file/d/1BaFRPTbhDD7BlPnmToq9AJxB0IAWpwmn/view?usp=drive_link
JD-GUI/ Java decompiler:
https://drive.google.com/file/d/1eZOX6USPyy4Uq6YSXPFZN43OiF5ze75J/view?usp=drive_link