This video shows the steps to check the signal strength of the mobile network from your Android App. It uses telephonyManager.getSignalStrength().getLevel() API for the same. The value of this signal strength would vary between 0 to 4. As per below documentation, the return value of getLevel() API is as follows:
https://developer.android.com/reference/android/telephony/SignalStrength#getLevel()
“a single integer from 0 to 4 representing the general signal quality. This may take into account many different radio technology inputs. 0 represents very poor signal strength while 4 represents a very strong signal strength.”
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.phonesignalstrength;
import android.os.Bundle;
import android.telephony.TelephonyManager;
import android.view.View;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
}
public void buttonGetSignalStrenght(View view){
TelephonyManager telephonyManager = (TelephonyManager) getSystemService(TELEPHONY_SERVICE);
textView.setText(String.valueOf(telephonyManager.getSignalStrength().getLevel()));
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textSize="34sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="112dp"
android:layout_marginTop="68dp"
android:onClick="buttonGetSignalStrenght"
android:text="Get Signal Strength"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
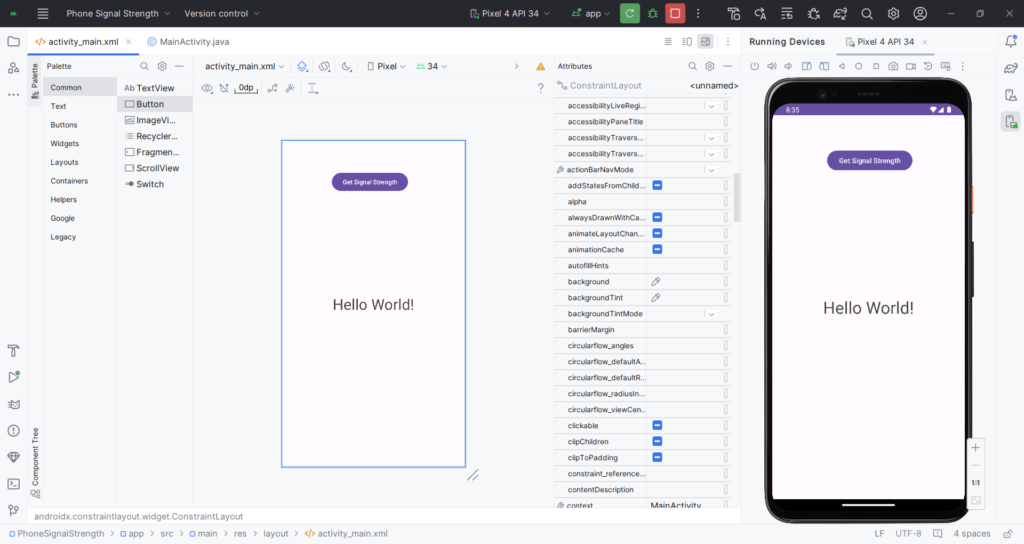
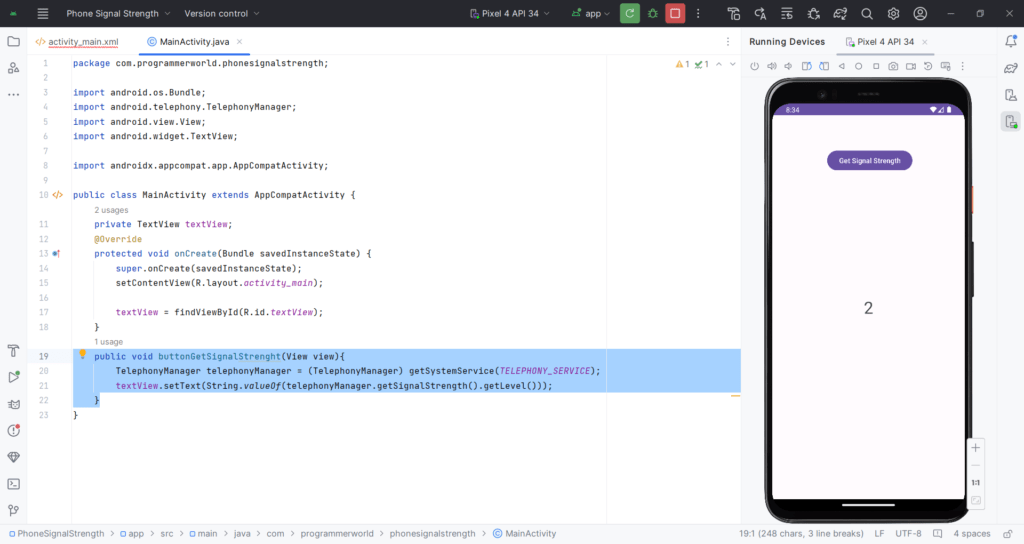
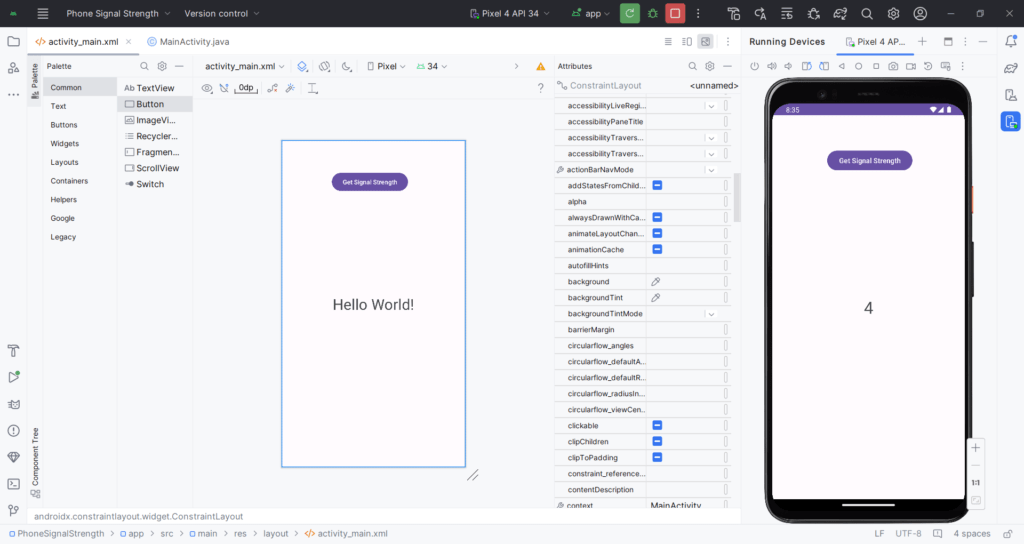
Excerpt:
The content provides a tutorial on how to check mobile network signal strength using an Android app. It suggests using the telephonyManager.getSignalStrength().getLevel() API. The value returns on a scale of 0-4, providing a gauge of signal quality; 0 signifies very poor signal strength, while 4 indicates very strong signal strength. The remaining part of the content includes a detailed walkthrough of an Android app’s code that utilizes the aforementioned method to display the signal strength. The resource for additional guidance is also supplied via a video link and contact information for further queries or recommendations.