This video shows the steps to build your own AI Chabot from scratch. In this demo it uses the Google’s Gemini APIs to build the chatbot in Kotlin language.
It refers below document for quickstarter guide:
https://ai.google.dev/tutorials/android_quickstart#kotlin
For APIKey generation, one can use below link:
https://aistudio.google.com/app/apikey
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.geminiaichatbbot
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import android.widget.EditText
import com.google.ai.client.generativeai.Chat
import com.google.ai.client.generativeai.GenerativeModel
import com.google.ai.client.generativeai.type.content
import kotlinx.coroutines.MainScope
import kotlinx.coroutines.launch
class MainActivity : AppCompatActivity() {
lateinit var editTextInput: EditText
lateinit var editTextOutput: EditText
lateinit var chat: Chat
var stringBuilder: StringBuilder = java.lang.StringBuilder()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
editTextInput = findViewById(R.id.editTextInput)
editTextOutput = findViewById(R.id.editTextOutput)
val generativeModel = GenerativeModel(
// For text-only input, use the gemini-pro model
modelName = "gemini-pro",
// Access your API key as a Build Configuration variable (see "Set up your API key" above)
apiKey = "AIzaSyDxTPhR0l6hQl5VrKjRsMn8aa-sSFL3kDg"
)
chat = generativeModel.startChat(
history = listOf(
content(role = "user") { text("Hello, I have 2 dogs in my house.") },
content(role = "model") { text("Great to meet you. What would you like to know?") }
)
)
stringBuilder.append("Hello, I have 2 dogs in my house.\n\n")
stringBuilder.append("Great to meet you. What would you like to know?\n\n")
editTextOutput.setText(stringBuilder.toString())
}
public fun buttonSendChat(view: View){
stringBuilder.append(editTextInput.text.toString() + "\n\n")
MainScope().launch {
var result = chat.sendMessage(editTextInput.text.toString())
stringBuilder.append(result.text + "\n\n")
editTextOutput.setText(stringBuilder.toString())
editTextInput.setText("")
}
}
}
plugins {
id("com.android.application")
id("org.jetbrains.kotlin.android")
}
android {
namespace = "com.programmerworld.geminiaichatbbot"
compileSdk = 34
defaultConfig {
applicationId = "com.programmerworld.geminiaichatbbot"
minSdk = 33
targetSdk = 34
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = "1.8"
}
}
dependencies {
implementation("androidx.core:core-ktx:1.12.0")
implementation("androidx.appcompat:appcompat:1.6.1")
implementation("com.google.android.material:material:1.11.0")
implementation("androidx.constraintlayout:constraintlayout:2.1.4")
testImplementation("junit:junit:4.13.2")
androidTestImplementation("androidx.test.ext:junit:1.1.5")
androidTestImplementation("androidx.test.espresso:espresso-core:3.5.1")
implementation("com.google.ai.client.generativeai:generativeai:0.2.0")
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.GeminiAIChatbbot"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="3dp"
android:onClick="buttonSendChat"
android:text="Send"
app:layout_constraintStart_toEndOf="@+id/editTextInput"
app:layout_constraintTop_toBottomOf="@+id/editTextOutput" />
<EditText
android:id="@+id/editTextOutput"
android:layout_width="416dp"
android:layout_height="638dp"
android:ems="10"
android:gravity="bottom|start"
android:hint="Output displayed here ..."
android:inputType="textMultiLine"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editTextInput"
android:layout_width="320dp"
android:layout_height="90dp"
android:ems="10"
android:gravity="start|top"
android:hint="Input here ..."
android:inputType="textMultiLine"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextOutput" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
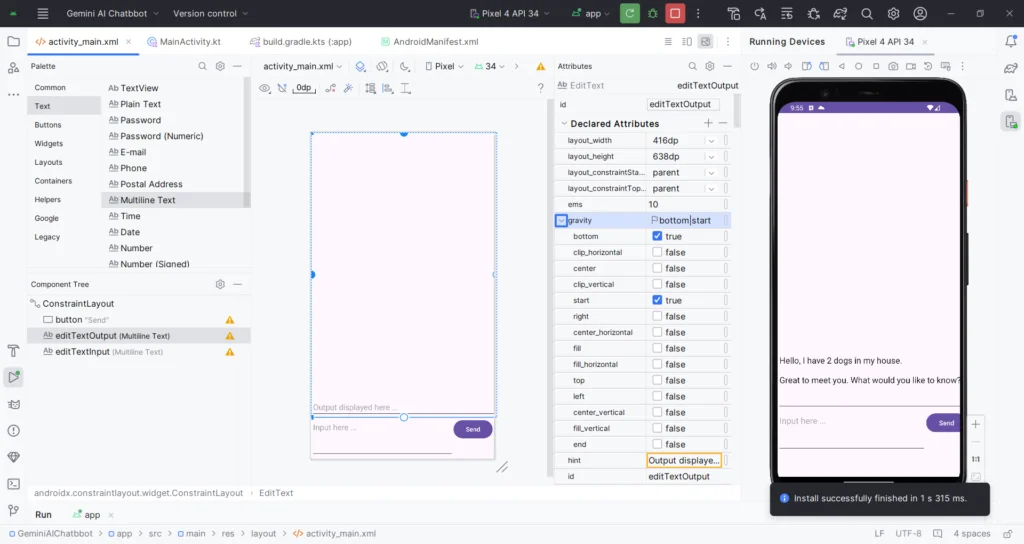
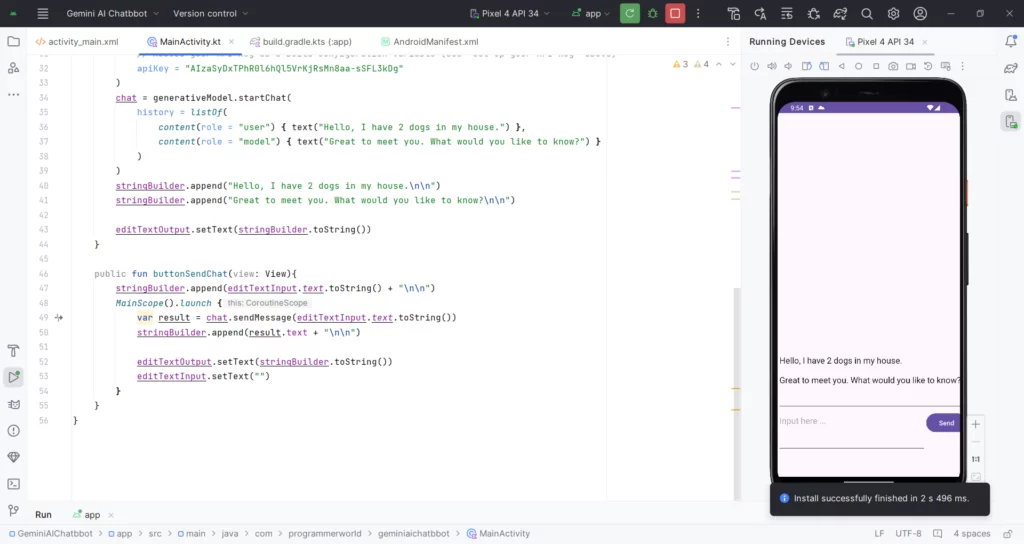
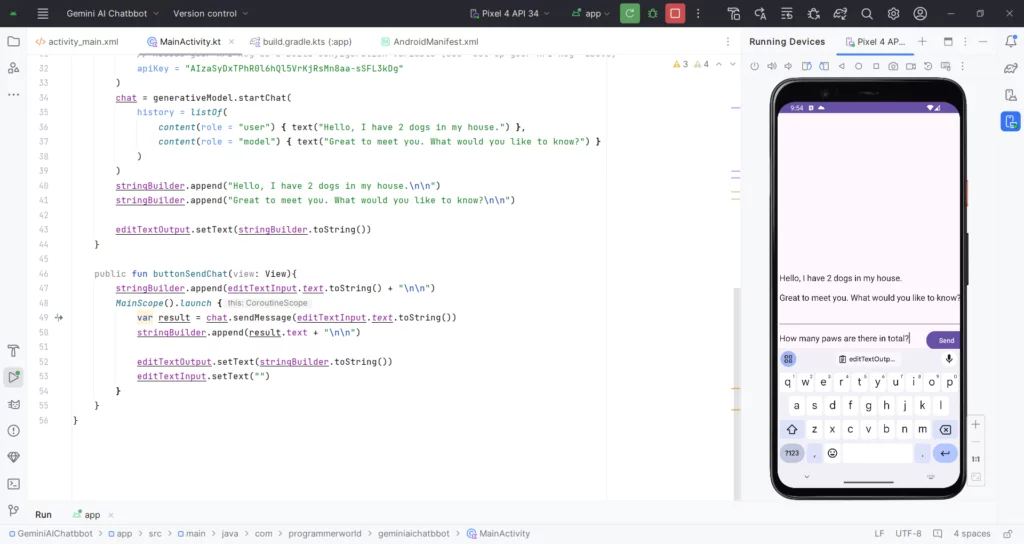
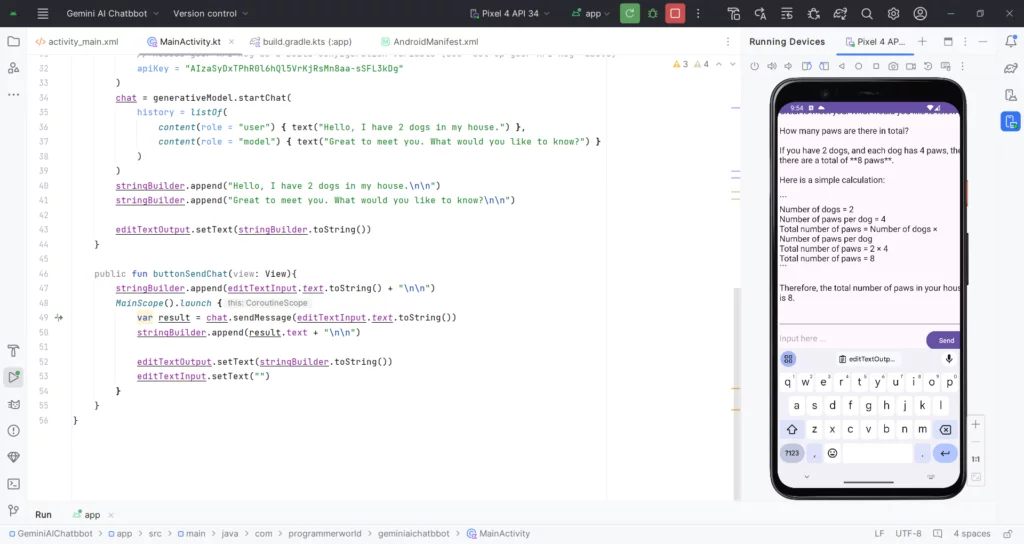
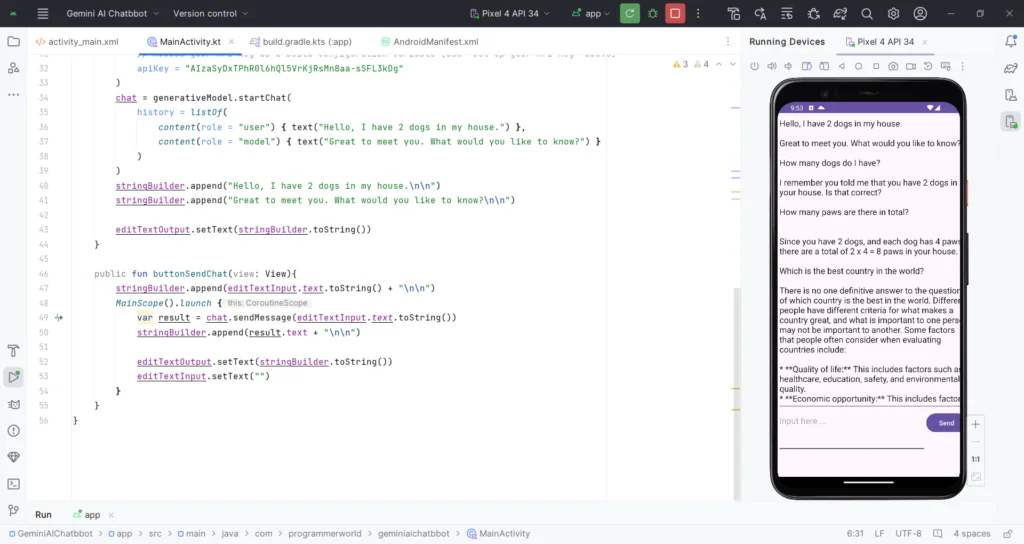
Complete Project Folder can be accessed from the below path Link on payment of USD 9:
https://drive.google.com/file/d/1nHuECU-L4s5UWfn3AdWpJgrevsPfyscH/view?usp=drive_link
Excerpt:
This content provides a video tutorial on how to create an AI Chatbot using Google’s Gemini APIs in Kotlin language. It includes a quickstart guide, API key generation link, and a YouTube demonstration.
The content further shares the complete source code for the Android application with package names, classes, XML layout files, and build configuration details. Additionally, it provides screenshots of the project and offers access to the full project folder upon payment.
Key Resources:
- Quickstart Guide: Google AI Quickstart in Kotlin
- API Key Generation: Google AI Studio API Key
- Demo Video: YouTube Link
- Contact Information: Contact Page or programmerworld1990@gmail.com
- Full Project Access: Google Drive Link (USD 9)