Vector DB in Android App
Steps:
- Set Up Project and APIs: Create an Android app and configure Gemini and Pinecone APIs with Retrofit.
- Pick and Extract PDF Text: Use SAF to select PDFs and iText7 to extract text into chunks.
- Generate and Store Embeddings: Convert text chunks to embeddings with Gemini and upsert to Pinecone.
- Test with Feedback: Run the app, show processing feedback, and verify vectors in Pinecone.
Pinecone API Key: https://app.pinecone.io/
Gemini API Key: https://aistudio.google.com/apikey
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Details:
package com.programmerworld.vectordbandoridapp;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import com.programmerworld.vectordbandoridapp.api.GeminiApi;
import com.programmerworld.vectordbandoridapp.api.PineconeApi;
import com.programmerworld.vectordbandoridapp.models.GeminiRequest;
import com.programmerworld.vectordbandoridapp.models.GeminiResponse;
import com.programmerworld.vectordbandoridapp.models.PineconeUpsertRequest;
import okhttp3.OkHttpClient;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
import com.itextpdf.kernel.pdf.PdfDocument;
import com.itextpdf.kernel.pdf.PdfReader;
import com.itextpdf.kernel.pdf.canvas.parser.PdfTextExtractor;
import java.io.InputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MainActivity extends AppCompatActivity {
private static final int PICK_PDF_REQUEST = 102;
private static final String TAG = "PineconeVectorDB";
private static final String GEMINI_API_KEY = "AIzaSyC7JMfto1SkmH5qoLr8dEHuDiew3VK_wdg"; // Replace with your key
private static final String PINECONE_API_KEY = "pcsk_7Uuobj_SRHpjmX1Wz61FhGNK1UknuXjzK4kKWNcrfcMGTEGbZw1t3iwocZ2gjpf6wzgxWY"; // Replace with your key
private GeminiApi geminiApi;
private PineconeApi pineconeApi;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize Retrofit clients
Retrofit retrofitGemini = new Retrofit.Builder()
.baseUrl("https://generativelanguage.googleapis.com/")
.client(new OkHttpClient())
.addConverterFactory(GsonConverterFactory.create())
.build();
geminiApi = retrofitGemini.create(GeminiApi.class);
Retrofit retrofitPinecone = new Retrofit.Builder()
.baseUrl("https://vectordb-mtnq2wb.svc.aped-4627-b74a.pinecone.io")
.client(new OkHttpClient())
.addConverterFactory(GsonConverterFactory.create())
.build();
pineconeApi = retrofitPinecone.create(PineconeApi.class);
// Button to pick PDFs
Button processButton = findViewById(R.id.processButton);
processButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
openFilePicker();
}
});
}
private void openFilePicker() {
Intent intent = new Intent(Intent.ACTION_OPEN_DOCUMENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
intent.setType("application/pdf");
intent.putExtra(Intent.EXTRA_ALLOW_MULTIPLE, true);
startActivityForResult(intent, PICK_PDF_REQUEST);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == PICK_PDF_REQUEST && resultCode == RESULT_OK && data != null) {
List<Uri> uris = new ArrayList<>();
if (data.getClipData() != null) {
for (int i = 0; i < data.getClipData().getItemCount(); i++) {
uris.add(data.getClipData().getItemAt(i).getUri());
}
} else if (data.getData() != null) {
uris.add(data.getData());
}
new Thread(new Runnable() {
@Override
public void run() {
for (Uri uri : uris) {
try {
String fileName = uri.getLastPathSegment();
String text = extractTextFromPdf(uri);
List<String> chunks = splitTextIntoChunks(text);
upsertToPinecone(fileName != null ? fileName : "unknown.pdf", chunks);
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(MainActivity.this, "Processed " + fileName, Toast.LENGTH_LONG).show();
}
});
} catch (Exception e) {
Log.e(TAG, "Error processing " + uri.toString() + ": " + e.getMessage());
}
}
}
}).start();
}
}
private String extractTextFromPdf(Uri uri) {
StringBuilder textBuilder = new StringBuilder();
try {
InputStream inputStream = getContentResolver().openInputStream(uri);
if (inputStream != null) {
PdfReader reader = new PdfReader(inputStream);
PdfDocument document = new PdfDocument(reader);
for (int i = 1; i <= document.getNumberOfPages(); i++) {
textBuilder.append(PdfTextExtractor.getTextFromPage(document.getPage(i)));
}
document.close();
inputStream.close();
Log.d(TAG, "Extracted text from " + uri.getLastPathSegment() + ": " + textBuilder.substring(0, Math.min(textBuilder.length(), 50)) + "...");
}
} catch (IOException e) {
Log.e(TAG, "Error reading PDF with iText: " + e.getMessage());
}
return textBuilder.toString();
}
private List<String> splitTextIntoChunks(String text) {
List<String> chunks = new ArrayList<>();
int chunkSize = 100;
int start = 0;
while (start < text.length()) {
int end = Math.min(start + chunkSize, text.length());
chunks.add(text.substring(start, end));
start = end;
}
Log.d(TAG, "Split text into " + chunks.size() + " chunks");
return chunks;
}
private void upsertToPinecone(String fileName, List<String> chunks) {
List<PineconeUpsertRequest.Vector> vectors = new ArrayList<>();
for (int i = 0; i < chunks.size(); i++) {
String chunk = chunks.get(i);
Call<GeminiResponse> call = geminiApi.getEmbedding(GEMINI_API_KEY, new GeminiRequest(chunk));
try {
Response<GeminiResponse> response = call.execute();
if (response.isSuccessful() && response.body() != null) {
List<Float> embedding = response.body().getEmbedding().getValues(); // Updated to getValues()
Map<String, String> metadata = new HashMap<>();
metadata.put("text", chunk);
vectors.add(new PineconeUpsertRequest.Vector(fileName + "-chunk-" + i, embedding, metadata));
Log.d(TAG, "Successfully got embedding for chunk " + i);
} else {
Log.e(TAG, "Failed to get embedding for chunk " + i + ": " + response.message() + " - Code: " + response.code());
Log.e(TAG, "Response body: " + (response.errorBody() != null ? response.errorBody().string() : "No error body"));
}
} catch (Exception e) {
Log.e(TAG, "Error fetching embedding: " + e.getMessage());
}
}
if (!vectors.isEmpty()) {
Call<Object> upsertCall = pineconeApi.upsertVectors(PINECONE_API_KEY, new PineconeUpsertRequest(vectors));
upsertCall.enqueue(new Callback<Object>() {
@Override
public void onResponse(Call<Object> call, Response<Object> response) {
if (response.isSuccessful()) {
Log.d(TAG, "Upserted " + vectors.size() + " vectors for " + fileName);
} else {
Log.e(TAG, "Upsert failed: " + response.message() + " - " + response.code());
}
}
@Override
public void onFailure(Call<Object> call, Throwable t) {
Log.e(TAG, "Upsert error: " + t.getMessage());
}
});
}
}
}
package com.programmerworld.vectordbandoridapp.api;
import com.programmerworld.vectordbandoridapp.models.GeminiRequest;
import com.programmerworld.vectordbandoridapp.models.GeminiResponse;
import retrofit2.Call;
import retrofit2.http.Body;
import retrofit2.http.POST;
import retrofit2.http.Query;
public interface GeminiApi {
@POST("v1/models/embedding-001:embedContent")
Call<GeminiResponse> getEmbedding(
@Query("key") String apiKey,
@Body GeminiRequest request
);
}
package com.programmerworld.vectordbandoridapp.api;
import com.programmerworld.vectordbandoridapp.models.PineconeUpsertRequest;
import retrofit2.Call;
import retrofit2.http.Body;
import retrofit2.http.Header;
import retrofit2.http.POST;
public interface PineconeApi {
@POST("vectors/upsert")
Call<Object> upsertVectors(
@Header("Api-Key") String apiKey,
@Body PineconeUpsertRequest request
);
}
package com.programmerworld.vectordbandoridapp.models;
import java.util.Collections;
import java.util.List;
public class GeminiRequest {
private String model = "embedding-001"; // Explicitly specify the model
private Content content;
private String task_type;
public GeminiRequest(String text) {
this.content = new Content(Collections.singletonList(new Part(text)));
this.task_type = "retrieval_document";
}
public String getModel() {
return model;
}
public Content getContent() {
return content;
}
public String getTaskType() {
return task_type;
}
// Nested Content class
public static class Content {
private List<Part> parts;
public Content(List<Part> parts) {
this.parts = parts;
}
public List<Part> getParts() {
return parts;
}
}
// Nested Part class
public static class Part {
private String text;
public Part(String text) {
this.text = text;
}
public String getText() {
return text;
}
}
}
package com.programmerworld.vectordbandoridapp.models;
import java.util.List;
public class GeminiResponse {
private Embedding embedding;
public Embedding getEmbedding() {
return embedding;
}
public static class Embedding {
private List<Float> values;
public List<Float> getValues() {
return values;
}
}
}
package com.programmerworld.vectordbandoridapp.models;
import java.util.List;
import java.util.Map;
public class PineconeUpsertRequest {
private List<Vector> vectors;
public PineconeUpsertRequest(List<Vector> vectors) {
this.vectors = vectors;
}
public List<Vector> getVectors() {
return vectors;
}
public static class Vector {
private String id;
private List<Float> values;
private Map<String, String> metadata;
public Vector(String id, List<Float> values, Map<String, String> metadata) {
this.id = id;
this.values = values;
this.metadata = metadata;
}
public String getId() {
return id;
}
public List<Float> getValues() {
return values;
}
public Map<String, String> getMetadata() {
return metadata;
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.VectorDBAndoridApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
plugins {
alias(libs.plugins.android.application)
}
android {
namespace = "com.programmerworld.vectordbandoridapp"
compileSdk = 35
defaultConfig {
applicationId = "com.programmerworld.vectordbandoridapp"
minSdk = 33
targetSdk = 35
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_11
targetCompatibility = JavaVersion.VERSION_11
}
// Add this block to resolve the duplicate file conflict
packaging {
resources {
excludes += "META-INF/DEPENDENCIES"
}
}
}
dependencies {
implementation(libs.appcompat)
implementation(libs.material)
implementation(libs.activity)
implementation(libs.constraintlayout)
testImplementation(libs.junit)
androidTestImplementation(libs.ext.junit)
androidTestImplementation(libs.espresso.core)
implementation("com.squareup.retrofit2:retrofit:2.9.0")
implementation("com.squareup.retrofit2:converter-gson:2.9.0")
implementation("com.squareup.okhttp3:okhttp:4.11.0")
implementation("com.itextpdf:itext7-core:7.2.5")
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp">
<Button
android:id="@+id/processButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="100dp"
android:text="Process PDFs"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
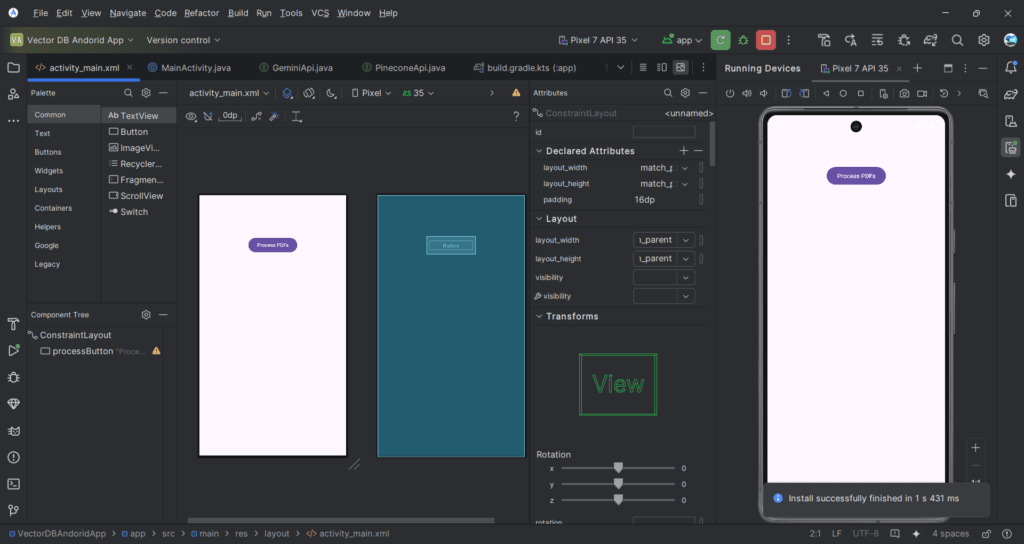
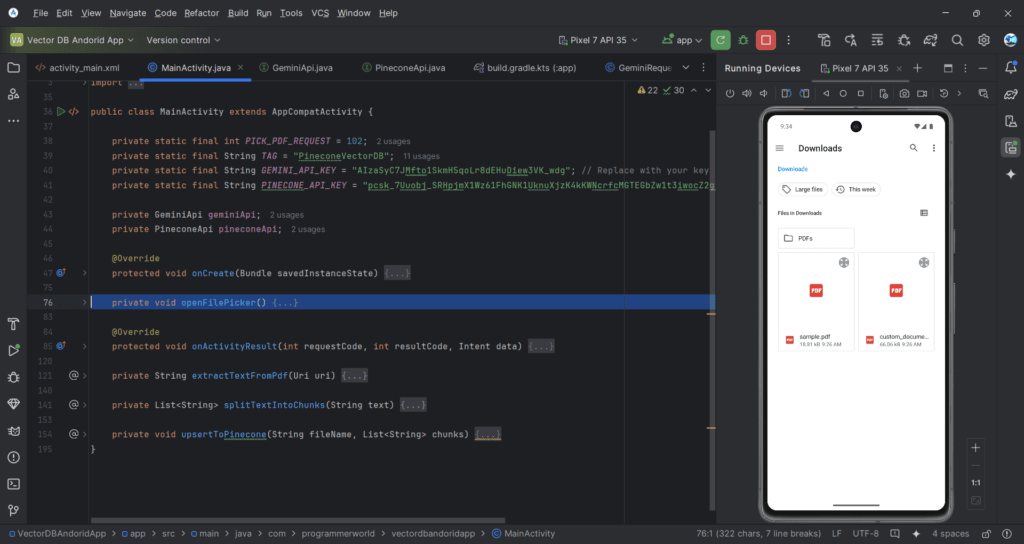
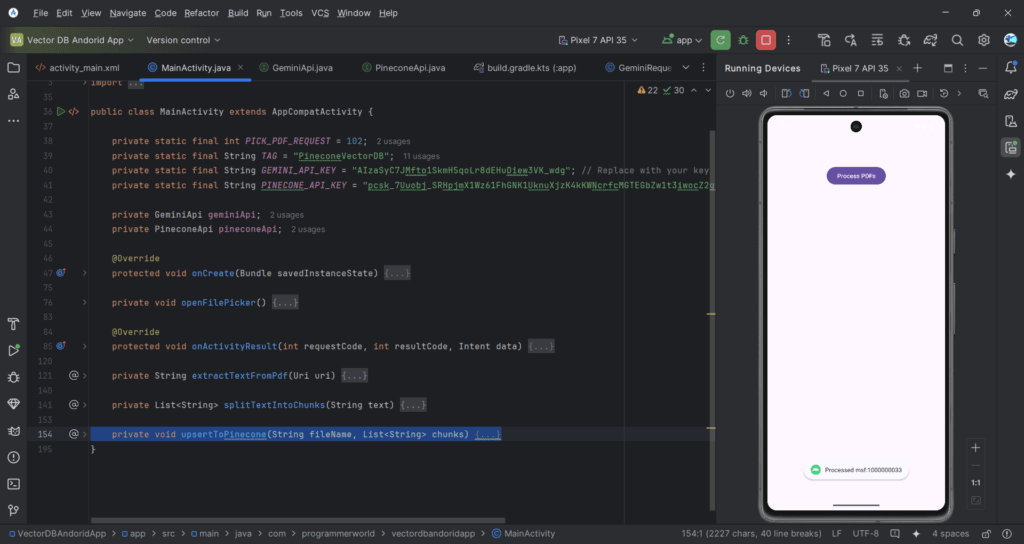
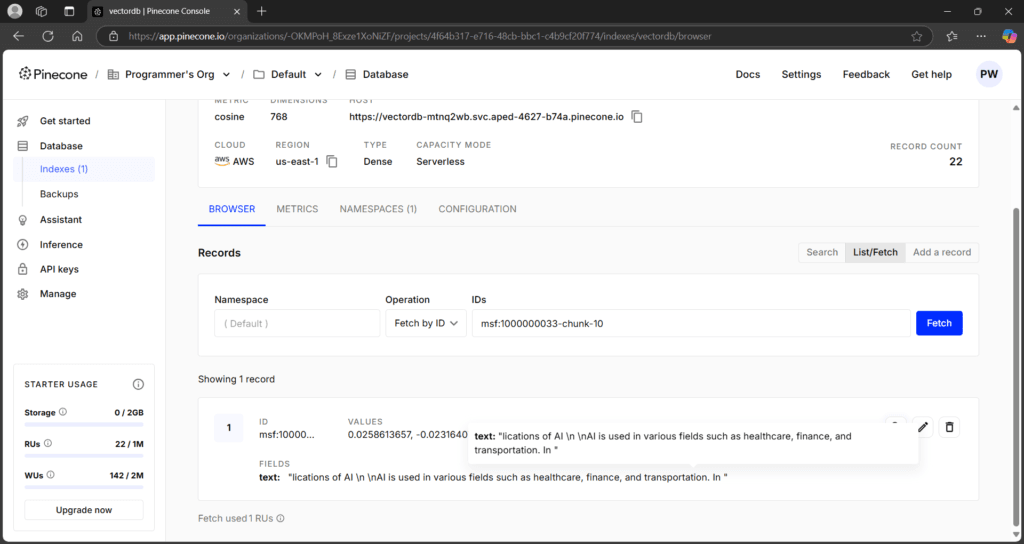
Values of Emedding:
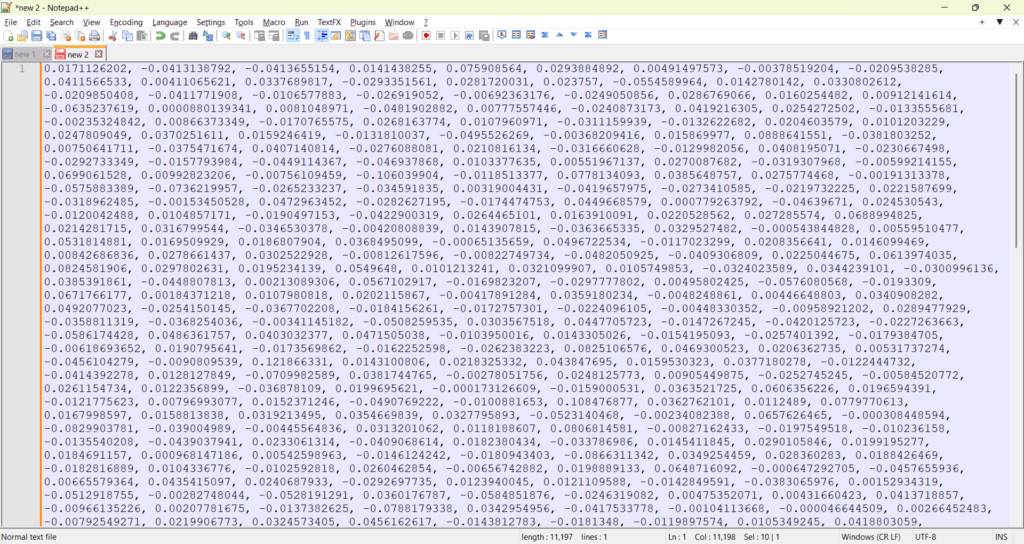
All the chunks in the db:
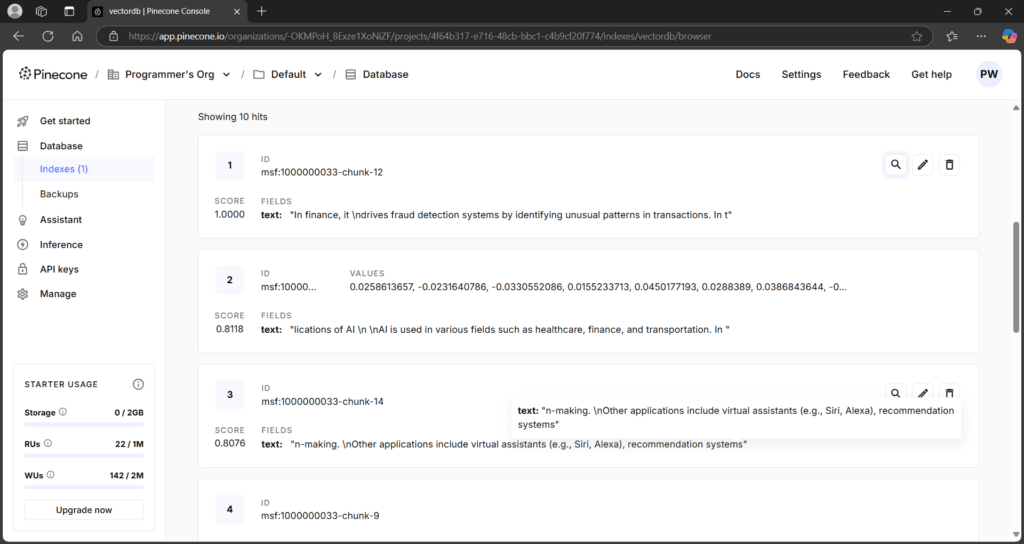
Embedding values – total 768 in numbers.
0.0171126202, -0.0413138792, -0.0413655154, 0.0141438255, 0.075908564, 0.0293884892, 0.00491497573, -0.00378519204, -0.0209538285, 0.0411566533, 0.00411065621, 0.0337689817, -0.0293351561, 0.0281720031, 0.023757, -0.0554589964, 0.0142780142, 0.0330802612, -0.0209850408, -0.0411771908, -0.0106577883, -0.026919052, -0.00692363176, -0.0249050856, 0.0286769066, 0.0160254482, 0.00912141614, -0.0635237619, 0.0000880139341, 0.0081048971, -0.0481902882, 0.00777557446, -0.0240873173, 0.0419216305, 0.0254272502, -0.0133555681, -0.00235324842, 0.00866373349, -0.0170765575, 0.0268163774, 0.0107960971, -0.0311159939, -0.0132622682, 0.0204603579, 0.0101203229, 0.0247809049, 0.0370251611, 0.0159246419, -0.0131810037, -0.0495526269, -0.00368209416, 0.015869977, 0.0888641551, -0.0381803252, 0.00750641711, -0.0375471674, 0.0407140814, -0.0276088081, 0.0210816134, -0.0316660628, -0.0129982056, 0.0408195071, -0.0230667498, -0.0292733349, -0.0157793984, -0.0449114367, -0.046937868, 0.0103377635, 0.00551967137, 0.0270087682, -0.0319307968, -0.00599214155, 0.0699061528, 0.00992823206, -0.00756109459, -0.106039904, -0.0118513377, 0.0778134093, 0.0385648757, 0.0275774468, -0.00191313378, -0.0575883389, -0.0736219957, -0.0265233237, -0.034591835, 0.00319004431, -0.0419657975, -0.0273410585, -0.0219732225, 0.0221587699, -0.0318962485, -0.00153450528, 0.0472963452, -0.0282627195, -0.0174474753, 0.0449668579, 0.000779263792, -0.04639671, 0.024530543, -0.0120042488, 0.0104857171, -0.0190497153, -0.0422900319, 0.0264465101, 0.0163910091, 0.0220528562, 0.027285574, 0.0688994825, 0.0214281715, 0.0316799544, -0.0346530378, -0.00420808839, 0.0143907815, -0.0363665335, 0.0329527482, -0.000543844828, 0.00559510477, 0.0531814881, 0.0169509929, 0.0186807904, 0.0368495099, -0.00065135659, 0.0496722534, -0.0117023299, 0.0208356641, 0.0146099469, 0.00842686836, 0.0278661437, 0.0302522928, -0.00812617596, -0.00822749734, -0.0482050925, -0.0409306809, 0.0225044675, 0.0613974035, 0.0824581906, 0.0297802631, 0.0195234139, 0.0549648, 0.0101213241, 0.0321099907, 0.0105749853, -0.0324023589, 0.0344239101, -0.0300996136, 0.0385391861, -0.0448807813, 0.00213089306, 0.0567102917, -0.0169823207, -0.0297777802, 0.00495802425, -0.0576080568, -0.0193309, 0.0671766177, 0.00184371218, 0.0107980818, 0.0202115867, -0.00417891284, 0.0359180234, -0.0048248861, 0.00446648803, 0.0340908282, 0.0492077023, -0.0254150145, -0.0367702208, -0.0184156261, -0.0172757301, -0.0224096105, -0.00448330352, -0.00958921202, 0.0289477929, -0.0358811319, -0.0368254036, -0.00341145182, -0.0508259535, 0.0303567518, 0.0447705723, -0.0147267245, -0.0420125723, -0.0227263663, -0.0586174428, 0.0486361757, 0.0403032377, 0.0471505038, -0.0103950016, 0.0143305026, -0.0154195093, -0.0257401392, -0.0179384705, -0.00618693652, 0.0190795641, -0.0173569862, -0.0162252598, -0.0262383223, 0.0825106576, 0.0469300523, 0.0206362735, 0.00531737274, -0.0456104279, -0.0090809539, 0.121866331, 0.0143100806, 0.0218325332, 0.043847695, 0.0159530323, 0.0377180278, -0.0122444732, -0.0414392278, 0.0128127849, -0.0709982589, 0.0381744765, -0.00278051756, 0.0248125773, 0.00905449875, -0.0252745245, -0.00584520772, 0.0261154734, 0.0122356899, -0.036878109, 0.0199695621, -0.000173126609, -0.0159000531, 0.0363521725, 0.0606356226, 0.0196594391, -0.0121775623, 0.00796993077, 0.0152371246, -0.0490769222, -0.0100881653, 0.108476877, 0.0362762101, 0.0112489, 0.0779770613, 0.0167998597, 0.0158813838, 0.0319213495, 0.0354669839, 0.0327795893, -0.0523140468, -0.00234082388, 0.0657626465, -0.000308448594, -0.0829903781, -0.039004989, -0.00445564836, 0.0313201062, 0.0118188607, 0.0806814581, -0.00827162433, -0.0197549518, -0.010236158, -0.0135540208, -0.0439037941, 0.0233061314, -0.0409068614, 0.0182380434, -0.033786986, 0.0145411845, 0.0290105846, 0.0199195277, 0.0184691157, 0.000968147186, 0.00542598963, -0.0146124242, -0.0180943403, -0.0866311342, 0.0349254459, 0.028360283, 0.0188426469, -0.0182816889, 0.0104336776, -0.0102592818, 0.0260462854, -0.00656742882, 0.0198889133, 0.0648716092, -0.000647292705, -0.0457655936, 0.00665579364, 0.0435415097, 0.0240687933, -0.0292697735, 0.0123940045, 0.0121109588, -0.0142849591, -0.0383065976, 0.00152934319, -0.0512918755, -0.00282748044, -0.0528191291, 0.0360176787, -0.0584851876, -0.0246319082, 0.00475352071, 0.00431660423, 0.0413718857, -0.00966135226, 0.00207781675, -0.0137382625, -0.0788179338, 0.0342954956, -0.0417533778, -0.00104113668, -0.000046644509, 0.00266452483, -0.00792549271, 0.0219906773, 0.0324573405, 0.0456162617, -0.0143812783, -0.0181348, -0.0119897574, 0.0105349245, 0.0418803059, 0.0000621185463, 0.0163520444, 0.0106230592, 0.0429844484, -0.0221753065, 0.0527424365, -0.008887982, -0.0384264849, -0.0420062132, 0.079854764, -0.0219751336, -0.0190621372, -0.0210342854, 0.0156964455, -0.0332434475, 0.0428590663, -0.0804348886, 0.0205515176, -0.0302497745, 0.0125316214, -0.122702762, -0.0672991872, 0.00920636952, -0.0202941019, 0.00106469763, 0.0171150155, 0.00621623313, -0.0509510562, -0.00513696577, -0.0172317307, -0.0513402559, 0.0169848073, 0.0794682056, 0.0397386663, 0.0140792765, 0.0617026277, -0.0296565257, -0.0170477778, 0.00556224538, -0.000915368, 0.0524185896, -0.0327968933, 0.0245426148, -0.0494712926, -0.0110581275, 0.0482152216, -0.0187044777, -0.0298525784, 0.0155694773, -0.0328852311, -0.0272817984, 0.00831281, -0.0537045151, 0.0460623428, 0.0269883387, 0.0137870479, 0.0352527462, -0.0498163253, -0.00175774843, -0.0155452751, -0.0440303236, -0.0114932451, 0.0478274152, -0.0114281643, -0.00399764162, 0.0367397852, 0.0429940559, 0.0187294297, 0.0127980672, 0.0225534812, 0.0473462716, 0.0550254062, -0.0365922153, 0.025729591, -0.0529196598, 0.0447942652, 0.150303781, -0.00775993802, 0.0387826338, 0.0050584944, -0.0151732, -0.0493844822, -0.00963133201, -0.0110590933, -0.0191965941, -0.0474916883, -0.046515014, 0.00863815844, -0.0139690759, 0.00857010577, 0.0289602987, -0.040529307, -0.0322589129, 0.0220073909, -0.0471771285, 0.00872785784, 0.0308130868, -0.0688744932, -0.0108240694, 0.0023758118, 0.0310669802, -0.021818297, -0.00192189578, 0.0113624437, -0.031743452, -0.0235402435, 0.00128078077, -0.0224595424, -0.0487478897, -0.0495209396, 0.0428095125, 0.0373613238, 0.0324983522, 0.0303819217, 0.0720488504, -0.0284526516, -0.00989410933, -0.00915001798, -0.0147128, -0.0492300838, 0.00139380735, 0.00598470122, -0.0446958952, -0.0296883527, 0.0503042713, 0.0256826431, -0.00863996521, -0.0177934449, -0.0319383591, 0.00092931313, -0.0312854722, -0.0451314971, 0.00571729522, -0.0972783417, 0.0475802086, -0.0234254468, -0.034915477, -0.0780117735, -0.0175752472, -0.0317830034, 0.000255242427, 0.0377536751, -0.0128828743, 0.0299072433, 0.0241886098, -0.0434051491, 0.014673722, -0.0546375588, 0.00961268321, -0.0211342964, -0.017667545, -0.0339432359, 0.0314289741, 0.0405506156, 0.00435516192, -0.0328461826, -0.00517820194, 0.0180747099, -0.0161598772, -0.0572435632, -0.0556176677, 0.0493280143, -0.0648457333, 0.00029373166, -0.000608431583, -0.000408385938, 0.0206259675, -0.016083058, -0.0411464311, 0.0014538822, 0.0183345713, -0.0131523544, 0.00502125965, 0.0259253047, -0.00158368063, -0.0255837236, 0.00953696854, -0.0315240137, -0.0348462164, 0.0454261191, -0.0143036144, 0.0775837, 0.0406196, 0.014908338, -0.0187895037, -0.0229803976, 0.00627870485, 0.00827395823, 0.0559199974, -0.0736092702, -0.00315360748, 0.0126427766, 0.0223777369, 1.06943794e-7, 0.0203626491, 0.0448351204, -0.0106854336, 0.00961303432, 0.0625405759, -0.0283993594, -0.00797006115, 0.0322017483, 0.00811043847, -0.0499815419, 0.0345282443, -0.0200668015, -0.12136206, -0.0354960933, 0.0110223303, -0.0630991384, -0.0237084, 0.0167485829, -0.0474326648, 0.0252802763, -0.046451848, 0.0176646914, -0.0439870395, -0.00098519423, 0.0285743903, 0.0118465228, -0.00230779289, 0.0385119691, -0.00982595421, -0.0253661312, 0.0329012387, -0.0357301533, 0.00693393685, 0.0112899467, -0.0315710865, 0.023849573, -0.00348846079, -0.0812018812, 0.0256718658, -0.0165414345, 0.00124009512, 0.0279416107, 0.0401234627, -0.0263865534, 0.0522716492, -0.0101951249, -0.00604866, 0.0117166508, 0.0233523306, -0.0246571377, -0.0239673108, 0.0395403728, -0.0239646509, 0.0219023619, 0.0799588636, 0.0228590779, -0.0201361291, -0.0293070879, 0.0274223331, -0.0126628838, -0.00630186545, 0.00944195595, 0.0243655946, -0.0141438413, 0.0250730403, -0.0248987172, -0.0428539775, 0.000950167247, -0.0296488479, -0.00274666422, 0.0396385603, 0.00198415341, -0.00734852627, 0.0534252524, 0.00234301621, -0.0110279238, 0.0452375039, 0.0715284571, 0.0294076223, 0.00340904482, -0.0770526901, 0.0601337701, -0.0293243937, -0.0170268957, -0.0178707018, 0.0100318454, 0.0448708199, -0.00252206274, -0.0466133468, 0.0137387291, -0.00973425061, 0.00170051737, 0.0869789124, -0.02730125, 0.0636261925, 0.0222574156, 0.0333542265, 0.0359283499, 0.0211869329, 0.050946787, -0.0311558843, 0.00433267374, 0.0354820117, -0.0362325907, -0.0251802281, -0.0720199645, 0.110308975, 0.0164107103, -0.00495841727, -0.0487392098, -0.00461043837, 0.00614177808, 0.0352631882, -0.0409972221, 0.015035064, 0.000259311142, -0.0439835638, 0.00681404537, 0.0881132334, 0.0482852608, 0.093764849, 0.0352031216, 0.00145122723, -0.0000598431543, -0.0370550342, -0.0069598509, -0.0431644693, -0.00240591867, 0.0288469512, 0.0056472877, -0.063503094, 0.00829233788, 0.00537586911, -0.0258161202, -0.0156090027, 0.0493945479, -0.00203718338, -0.0942106098, -0.0851449668, 0.00557609322, -0.0343499072, -0.00976363756, 0.0318788737, -0.0271680467, 0.0694014505, -0.0199826863, 0.00117550837, -0.0433353372, 0.00449752156, 0.0246520173, 0.0171718467, 0.0215099901, 0.000140797172, 0.0479089729, -0.00240754778, -0.0312637202, -0.019243395, -0.0798093304, -0.040988382, 0.0350426957, -0.0635187626, 0.0317882709, 0.0192320794, 0.00093849015, 0.0846776813, 0.0240872372, -0.0278143734, 0.0523475297, 0.0384688377, -0.00704463664, -0.0212811455, 0.0112307575, 0.0014880565, 0.00206317659, -0.00908502564, 0.0514326803, 0.0109356353, -0.0105288709, -0.0590190254, -0.0304751322, -0.0103260148, -0.0174679663, -0.0108450186, 0.0410749726, 0.00909738895, 0.0394226275, -0.0326562934, -0.00440268824, 0.0397463404, 0.0115225818, -0.00771901, -0.0533390716, -0.0163378026, 0.0470615104, 0.0132104447, 0.000782420742, 0.0175926816, 0.0168249775, 0.0599288344, 0.0261172466, 0.0631494522, -0.0724594593, -0.00171897723, 0.040651653, 0.011903706, -0.0312600359, 0.0454075821, 0.00461096503, -0.0413357466, 0.0247185025, 0.051268138, 0.0527890511, 0.0205552392, -0.0331087448, -0.0431820229, 0.0426023751, -0.0494130179, -0.0162378307, -0.0237101596, -0.0227171, 0.031335149, -0.0278467312, -0.054331705, 0.0216461103, -0.00665543787, 0.108279295, 0.050384637, 0.0352939479, -0.0210265126, -0.0868032053, 0.00534259295, -0.0328087099, 0.0358449444, 0.0834443793, 0.0373895392, -0.0297959186, -0.0192442127, -0.030495137, -0.0135315619, -0.0812246874, -0.0155040221, 0.00757033424, -0.0148639912, 0.0558121055, 0.0680984929, -0.0157717541, -0.0228057187, -0.00122083363, -0.00563389296, 0.0209955033, -0.000290243, 0.0126902284, -0.0404501818, -0.00104762136, 0.0470051579, 0.0254178233, 0.0254415926, 0.0119384825