Chatbot Android App using Fine Tuned Gemini
Steps:
- Prepare custom data
- Create Fine Tune model in Google AI Studio. Give access of the model to the API Key.
- Create a chatbot Android App using this fine tuned model
API Key: AIzaSyBmGxiZrYWrxxxxxxxxoHM0T9aaaZm0
Sample Prompts used:
- Can I buy baguette? If yes, then what is it’s price?
- Do you have gluten-free cake? And what’s the cost of a chocolate cake?
- I heard you do not keep oat milk, right?
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Details:
Code:
package com.programmerworld.bakerychatbotcustomapp;
import android.os.Bundle;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import com.google.ai.client.generativeai.GenerativeModel;
import com.google.ai.client.generativeai.java.GenerativeModelFutures;
import com.google.ai.client.generativeai.type.Content;
import com.google.ai.client.generativeai.type.GenerateContentResponse;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
public class MainActivity extends AppCompatActivity {
private EditText questionInput;
private TextView responseView;
private GenerativeModelFutures model;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
questionInput = findViewById(R.id.questionInput);
responseView = findViewById(R.id.responseView);
Button sendButton = findViewById(R.id.sendButton);
// Initialize the fine-tuned model
GenerativeModel gm = new GenerativeModel(
"tunedModels/bakeryandroidapp-sj0xdsjgpm2h", // Replace with your tuned model ID
"AIzaSyBmGxiZrYWrxxxxxxxxoHM0T9aaaZm0" // Replace with your API key
);
model = GenerativeModelFutures.from(gm);
sendButton.setOnClickListener(v -> {
String query = questionInput.getText().toString().trim();
if (!query.isEmpty()) {
getResponse(query);
} else {
responseView.setText("Please enter a question.");
}
});
}
private void getResponse(String query) {
// Create a Content object with the user's query
Content content = new Content.Builder()
.addText(query)
.build();
// Pass the Content object to generateContent
ListenableFuture<GenerateContentResponse> response = model.generateContent(content);
Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() {
@Override
public void onSuccess(GenerateContentResponse result) {
String resultText = result.getText();
runOnUiThread(() -> responseView.setText(resultText));
}
@Override
public void onFailure(Throwable t) {
runOnUiThread(() -> responseView.setText("Error: " + t.getMessage()));
}
}, getMainExecutor());
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.BakeryChatbotCustomApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
plugins {
alias(libs.plugins.android.application)
}
android {
namespace = "com.programmerworld.bakerychatbotcustomapp"
compileSdk = 35
defaultConfig {
applicationId = "com.programmerworld.bakerychatbotcustomapp"
minSdk = 33
targetSdk = 35
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_11
targetCompatibility = JavaVersion.VERSION_11
}
}
dependencies {
implementation(libs.appcompat)
implementation(libs.material)
implementation(libs.activity)
implementation(libs.constraintlayout)
testImplementation(libs.junit)
androidTestImplementation(libs.ext.junit)
androidTestImplementation(libs.espresso.core)
implementation(libs.guava)
implementation(libs.generativeai)
implementation(libs.firebase.crashlytics.buildtools)
}
[versions]
agp = "8.8.1"
junit = "4.13.2"
junitVersion = "1.2.1"
espressoCore = "3.6.1"
appcompat = "1.7.0"
material = "1.12.0"
activity = "1.10.0"
constraintlayout = "2.2.0"
guava = "33.3.1-android"
generativeai = "0.9.0"
firebaseCrashlyticsBuildtools = "3.0.3"
[libraries]
junit = { group = "junit", name = "junit", version.ref = "junit" }
ext-junit = { group = "androidx.test.ext", name = "junit", version.ref = "junitVersion" }
espresso-core = { group = "androidx.test.espresso", name = "espresso-core", version.ref = "espressoCore" }
appcompat = { group = "androidx.appcompat", name = "appcompat", version.ref = "appcompat" }
material = { group = "com.google.android.material", name = "material", version.ref = "material" }
activity = { group = "androidx.activity", name = "activity", version.ref = "activity" }
constraintlayout = { group = "androidx.constraintlayout", name = "constraintlayout", version.ref = "constraintlayout" }
guava = { module = "com.google.guava:guava", version.ref = "guava" }
generativeai = { group = "com.google.ai.client.generativeai", name = "generativeai", version.ref = "generativeai" }
firebase-crashlytics-buildtools = { group = "com.google.firebase", name = "firebase-crashlytics-buildtools", version.ref = "firebaseCrashlyticsBuildtools" }
[plugins]
android-application = { id = "com.android.application", version.ref = "agp" }
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<EditText
android:id="@+id/questionInput"
android:layout_width="379dp"
android:layout_height="119dp"
android:layout_marginTop="80dp"
android:hint="Ask about our bakery..."
android:textSize="24sp" />
<Button
android:id="@+id/sendButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_marginBottom="20dp"
android:text="Send" />
<TextView
android:id="@+id/responseView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="48sp" />
</LinearLayout>
Screenshots:
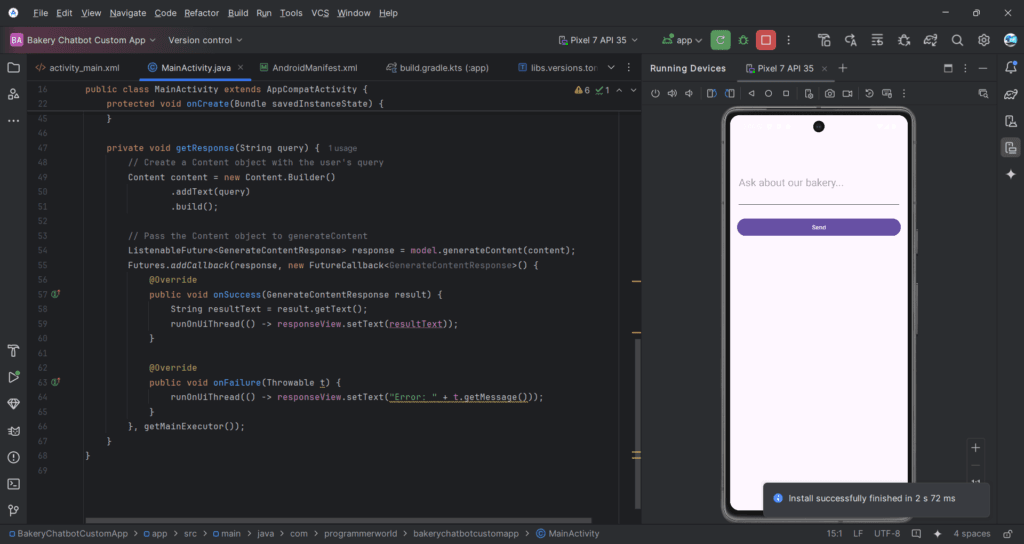
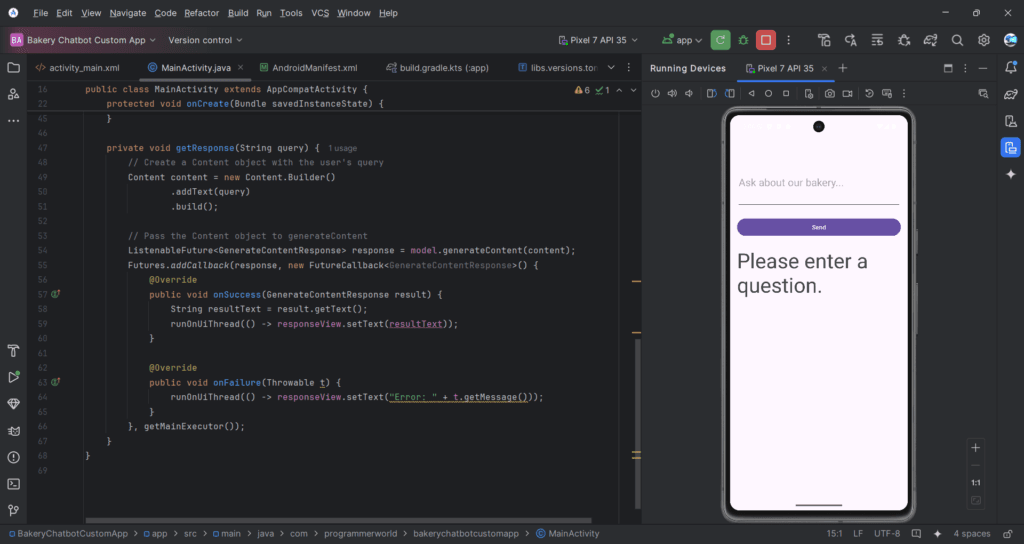
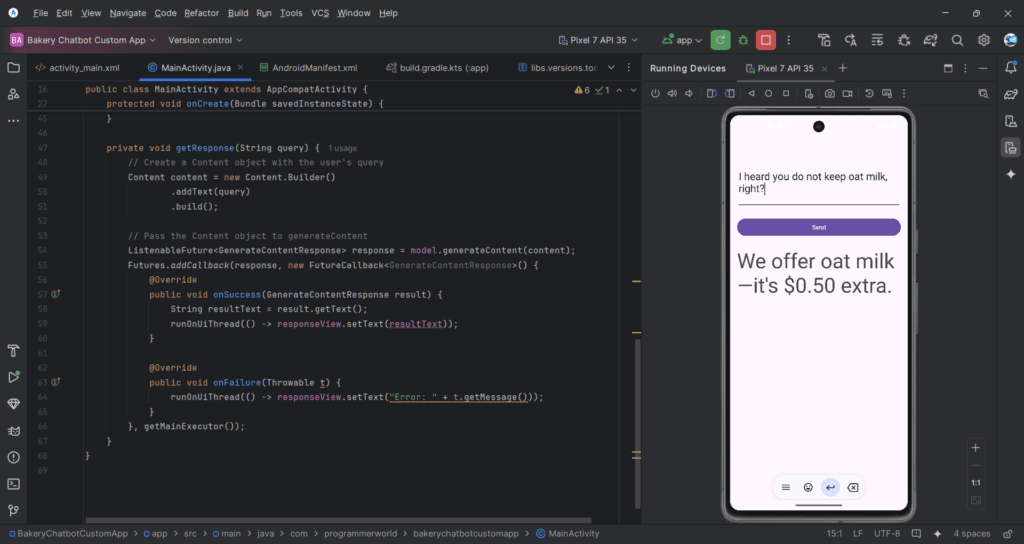
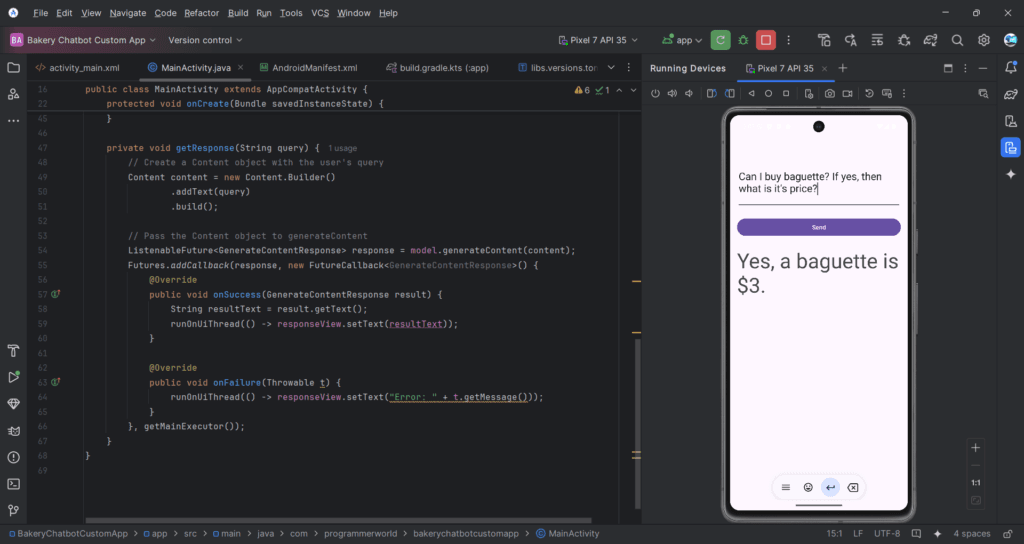
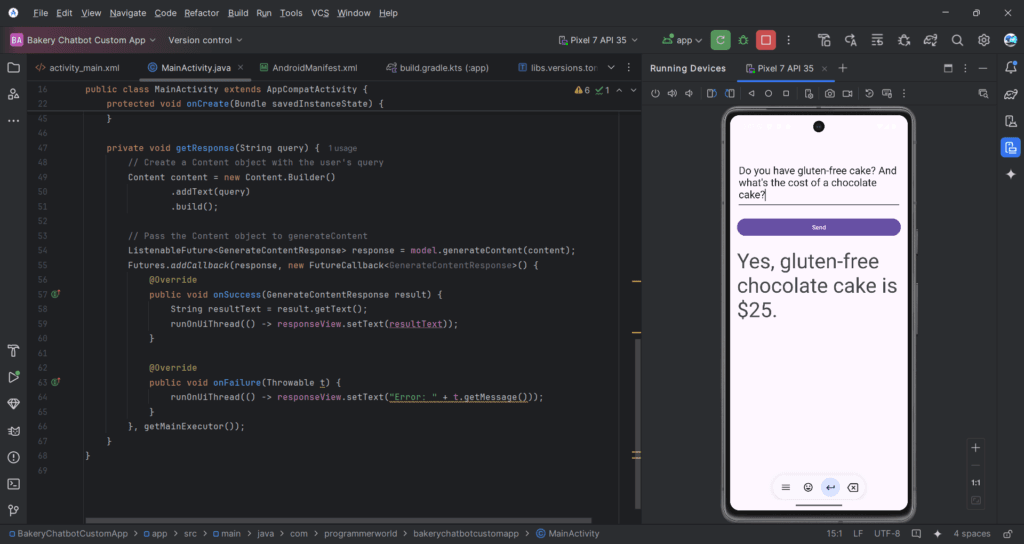
Bakery Data Used in the demo for fine tuning:
Data in JSONL format below. If required, then copy this data in a file and save that as bakery_data.jsonl
{"input": "What’s the price of a chocolate cake?", "output": "Our chocolate cake is $15."}
{"input": "Do you have gluten-free options?", "output": "Yes, we offer gluten-free cupcakes and bread!"}
{"input": "How long does delivery take?", "output": "Delivery usually takes 30-45 minutes."}
{"input": "What’s in your vanilla cupcake?", "output": "Our vanilla cupcake has flour, sugar, butter, eggs, and vanilla extract."}
{"input": "Can I order a custom cake?", "output": "Yes, we take custom cake orders! Please call us to discuss details."}
{"input": "How much is a dozen donuts?", "output": "A dozen donuts costs $12."}
{"input": "Do you have vegan pastries?", "output": "Yes, we have vegan muffins and cookies available."}
{"input": "What time do you open?", "output": "We open at 7 AM every day."}
{"input": "Are your cakes nut-free?", "output": "Most cakes are nut-free, but please specify allergies when ordering."}
{"input": "How much is a loaf of sourdough bread?", "output": "A loaf of sourdough bread is $5."}
{"input": "Do you deliver to downtown?", "output": "Yes, we deliver downtown within a 5-mile radius."}
{"input": "What’s the price of a birthday cake?", "output": "Birthday cakes start at $25, depending on size and design."}
{"input": "Can I get a cake by tomorrow?", "output": "Yes, if ordered by 2 PM today, we can deliver tomorrow."}
{"input": "Do you have sugar-free desserts?", "output": "We offer sugar-free brownies and cookies."}
{"input": "What’s your most popular item?", "output": "Our chocolate croissants are the most popular!"}
{"input": "How much is a coffee with a muffin?", "output": "A coffee and muffin combo is $6."}
{"input": "Are your donuts freshly made?", "output": "Yes, our donuts are made fresh every morning."}
{"input": "What flavors of cupcakes do you have?", "output": "We have vanilla, chocolate, red velvet, and lemon cupcakes."}
{"input": "Can I order online?", "output": "Yes, visit our website or app to place an order."}
{"input": "How much is delivery?", "output": "Delivery is $3 within 5 miles, $5 beyond that."}
{"input": "Do you have lactose-free options?", "output": "Yes, we offer lactose-free cookies and cakes."}
{"input": "What’s the price of a wedding cake?", "output": "Wedding cakes start at $100, depending on tiers and design."}
{"input": "Are your breads organic?", "output": "Our sourdough and whole wheat breads are made with organic flour."}
{"input": "Can I pick up an order?", "output": "Yes, you can pick up orders at our store anytime after 8 AM."}
{"input": "What’s in your chocolate croissant?", "output": "It’s made with buttery dough and premium dark chocolate."}
{"input": "Do you offer catering?", "output": "Yes, we cater events—contact us for a quote!"}
{"input": "How much is a cookie?", "output": "A single cookie is $1.50."}
{"input": "What’s your closing time?", "output": "We close at 6 PM daily."}
{"input": "Do you have seasonal specials?", "output": "Yes, check our website for current seasonal items like pumpkin scones!"}
{"input": "Can I order a cake without frosting?", "output": "Yes, we can make a plain cake—just let us know."}
{"input": "How much is a baguette?", "output": "A baguette costs $3."}
{"input": "Are your pastries egg-free?", "output": "Some pastries like our vegan cookies are egg-free."}
{"input": "What’s the price of a lemon tart?", "output": "A lemon tart is $4."}
{"input": "Do you have a loyalty program?", "output": "Yes, buy 10 items and get the 11th free!"}
{"input": "Can I get a refund?", "output": "Refunds are available within 24 hours if there’s an issue."}
{"input": "How fresh is your bread?", "output": "All bread is baked fresh daily."}
{"input": "What’s in your red velvet cake?", "output": "It’s made with cocoa, buttermilk, and cream cheese frosting."}
{"input": "Do you have low-carb options?", "output": "Yes, we offer low-carb almond flour cookies."}
{"input": "How much is a pie?", "output": "Pies start at $10, depending on the flavor."}
{"input": "Can I order for a party?", "output": "Absolutely, we can prepare party trays—call us!"}
{"input": "What’s the price of a cinnamon roll?", "output": "A cinnamon roll is $3."}
{"input": "Do you use artificial sweeteners?", "output": "No, our sugar-free items use natural sweeteners like stevia."}
{"input": "How long do your cakes stay fresh?", "output": "Our cakes stay fresh for 3-5 days if refrigerated."}
{"input": "What’s your cheapest dessert?", "output": "Our $1 cookies are the cheapest option."}
{"input": "Do you have a kids’ menu?", "output": "We offer mini cupcakes and cookies for kids."}
{"input": "How much is a latte?", "output": "A latte costs $4."}
{"input": "Can I order gluten-free bread?", "output": "Yes, our gluten-free bread is available for $6 per loaf."}
{"input": "What’s in your blueberry muffin?", "output": "It’s made with fresh blueberries, flour, sugar, and butter."}
{"input": "Do you ship nationwide?", "output": "No, we only deliver locally at this time."}
{"input": "How much is a cheesecake?", "output": "A cheesecake is $20."}
{"input": "Are your ingredients local?", "output": "We source flour and eggs from local farms."}
{"input": "What’s the price of a brownie?", "output": "A brownie is $2.50."}
{"input": "Do you have dairy-free cakes?", "output": "Yes, we offer dairy-free chocolate and vanilla cakes."}
{"input": "Can I cancel an order?", "output": "Yes, cancellations are free if made 24 hours in advance."}
{"input": "How much is a scone?", "output": "A scone costs $2."}
{"input": "What’s your return policy?", "output": "Returns accepted within 24 hours for quality issues."}
{"input": "Do you have pumpkin spice items?", "output": "Yes, we have pumpkin spice muffins in the fall!"}
{"input": "How much is a macaron?", "output": "A macaron is $1.75."}
{"input": "Are your cakes made daily?", "output": "Yes, all cakes are baked fresh each day."}
{"input": "What’s in your apple pie?", "output": "It’s made with fresh apples, cinnamon, and a flaky crust."}
{"input": "Do you offer gift cards?", "output": "Yes, gift cards start at $10."}
{"input": "How much is a sandwich?", "output": "A bakery sandwich with bread and fillings is $7."}
{"input": "Can I order a dozen cupcakes?", "output": "Yes, a dozen cupcakes is $18."}
{"input": "What’s the price of an éclair?", "output": "An éclair costs $3.50."}
{"input": "Do you have keto options?", "output": "Yes, we offer keto-friendly brownies."}
{"input": "How much is a bagel?", "output": "A bagel is $1.25."}
{"input": "Are your cookies crispy or soft?", "output": "We offer both—specify your preference!"}
{"input": "What’s in your carrot cake?", "output": "It’s made with carrots, walnuts, and cream cheese frosting."}
{"input": "Do you have hot chocolate?", "output": "Yes, hot chocolate is $3."}
{"input": "How much is a fruit tart?", "output": "A fruit tart is $5."}
{"input": "Can I order for pickup tomorrow?", "output": "Yes, place your order by 5 PM today for tomorrow pickup."}
{"input": "What’s the price of a pretzel?", "output": "A pretzel costs $2."}
{"input": "Do you have whole wheat bread?", "output": "Yes, our whole wheat bread is $4 per loaf."}
{"input": "How much is a Danish pastry?", "output": "A Danish pastry is $3."}
{"input": "Are your items preservative-free?", "output": "Yes, we avoid artificial preservatives."}
{"input": "What’s in your lemon cake?", "output": "It’s made with lemon zest, sugar, and a light glaze."}
{"input": "Do you have decaf coffee?", "output": "Yes, decaf coffee is available for $3."}
{"input": "How much is a pound cake?", "output": "A pound cake is $8."}
{"input": "Can I order a gluten-free cake?", "output": "Yes, gluten-free cakes start at $20."}
{"input": "What’s the price of a roll?", "output": "A dinner roll is $0.75."}
{"input": "Do you have soy milk options?", "output": "Yes, we offer soy milk for coffee drinks."}
{"input": "How much is a fudge square?", "output": "A fudge square is $2."}
{"input": "Are your muffins nut-free?", "output": "Most muffins are nut-free unless specified."}
{"input": "What’s in your pecan pie?", "output": "It’s made with pecans, brown sugar, and a buttery crust."}
{"input": "Do you have almond croissants?", "output": "Yes, almond croissants are $4 each."}
{"input": "How much is a tea?", "output": "A cup of tea costs $2.50."}
{"input": "Can I order a vegan cake?", "output": "Yes, vegan cakes start at $22."}
{"input": "What’s the price of a biscuit?", "output": "A biscuit is $1."}
{"input": "Do you have oat milk?", "output": "Yes, we offer oat milk for $0.50 extra."}
{"input": "How much is a chocolate muffin?", "output": "A chocolate muffin is $2.75."}
{"input": "Are your cakes vegan-friendly?", "output": "We have specific vegan cakes—just ask!"}
{"input": "What’s in your banana bread?", "output": "It’s made with ripe bananas, flour, and a hint of cinnamon."}
{"input": "Do you have espresso?", "output": "Yes, an espresso shot is $2."}
{"input": "How much is a shortbread cookie?", "output": "A shortbread cookie is $1.25."}
{"input": "Can I order a dozen bagels?", "output": "Yes, a dozen bagels is $12."}
{"input": "What’s the price of a turnover?", "output": "A turnover costs $3."}
{"input": "Do you have birthday candles?", "output": "Yes, we sell candles for $1 per pack."}
{"input": "How much is a cappuccino?", "output": "A cappuccino is $4.50."}
{"input": "Are your items freshly baked?", "output": "Yes, everything is baked fresh daily!"}
{"input": "What’s the price of a chocolate cake?", "output": "Our chocolate cake is $15."}
{"input": "Do you have gluten-free options?", "output": "Yes, we offer gluten-free cupcakes and bread!"}
{"input": "How long does delivery take?", "output": "Delivery usually takes 30-45 minutes."}
Screenshot of the fine-tuned model in google ai studio:
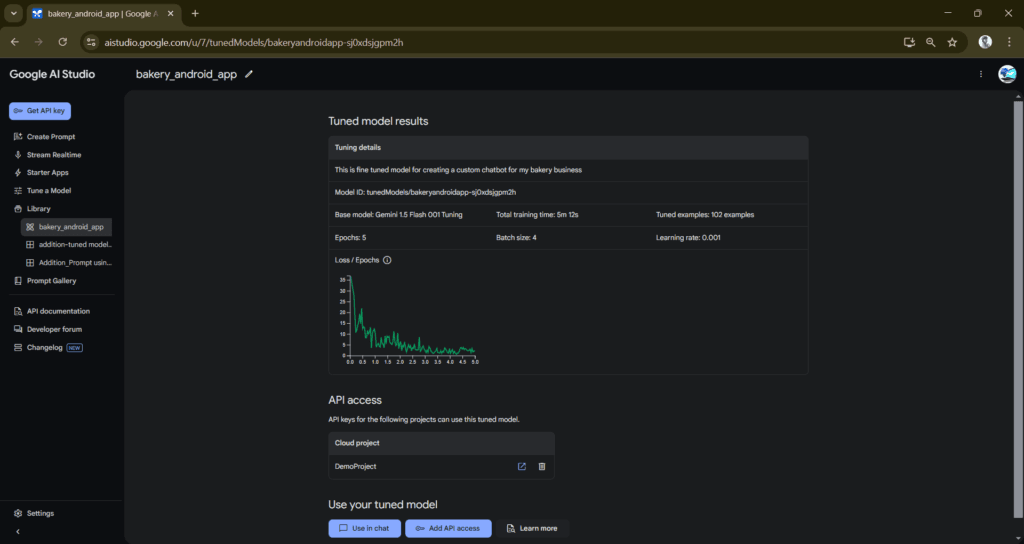