In this video it shows how to add Logs in Android Java Code and then check it in the Logcat in Android Studio IDE. It adds below Log statement in the code for demonstation.
Log.d("Counter Value", "Integer Value increased to " + i);
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete code:
package com.programmerworld.logsinandroidjavacode;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class MainActivity extends AppCompatActivity {
private int i = 0;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
textView = findViewById(R.id.textView);
return insets;
});
}
public void counterIncrement(View view){
textView.setText(String.valueOf(++i));
Log.d("Counter Value", "Integer Value increased to " + i);
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0"
android:textSize="24sp"
android:textStyle="bold"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.369" />
<TextView
android:id="@+id/textView2"
android:layout_width="214dp"
android:layout_height="66dp"
android:layout_marginTop="169dp"
android:onClick="counterIncrement"
android:text="Total Count"
android:textAlignment="center"
android:textSize="34sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
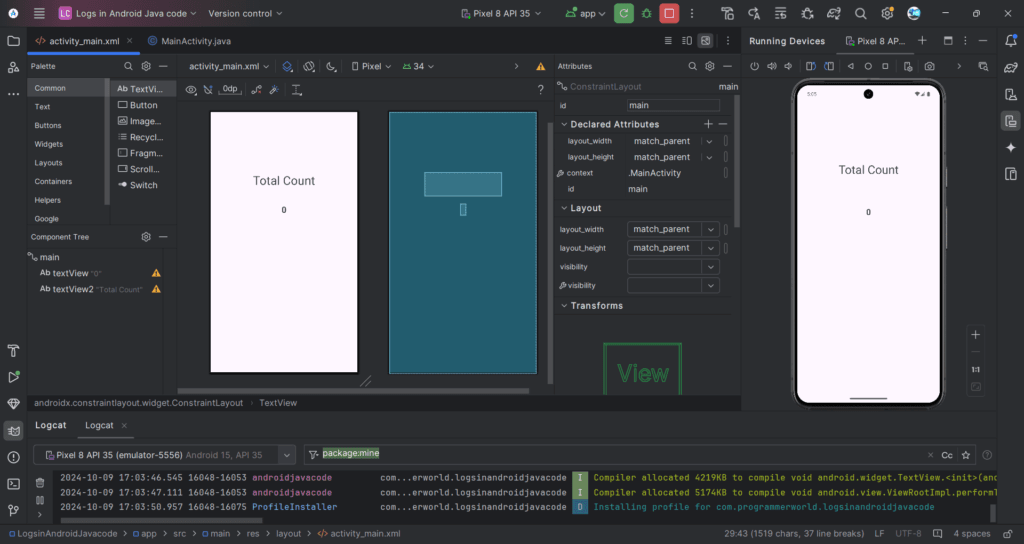
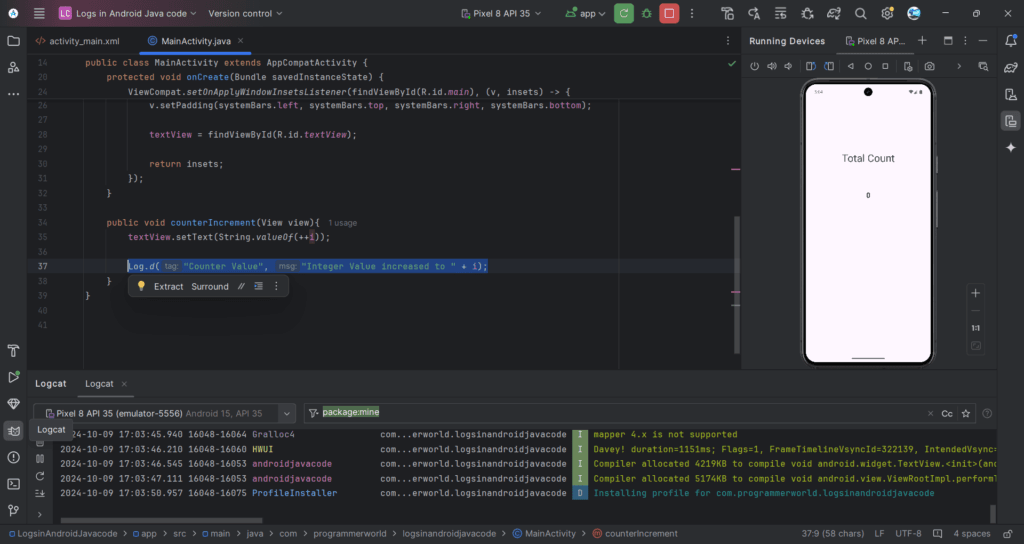
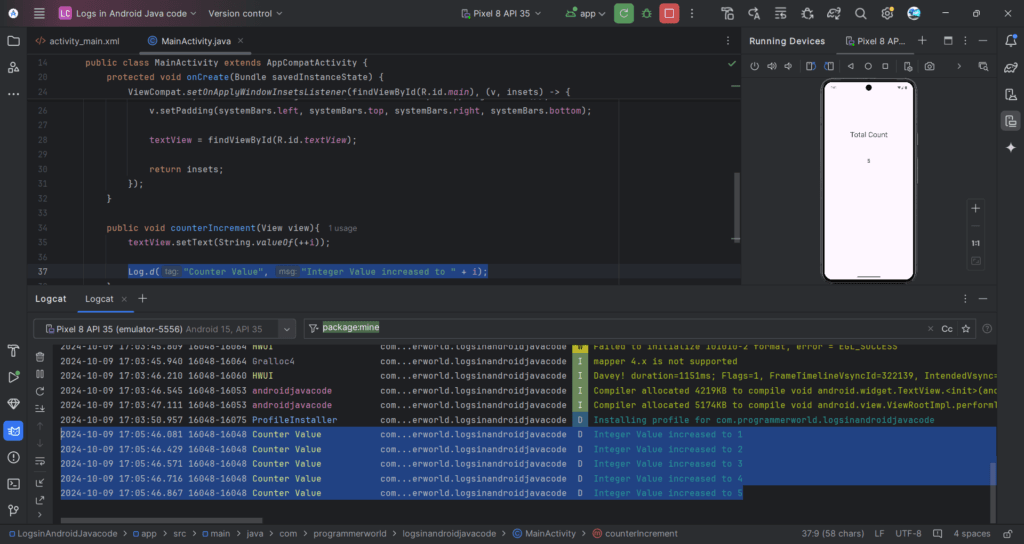
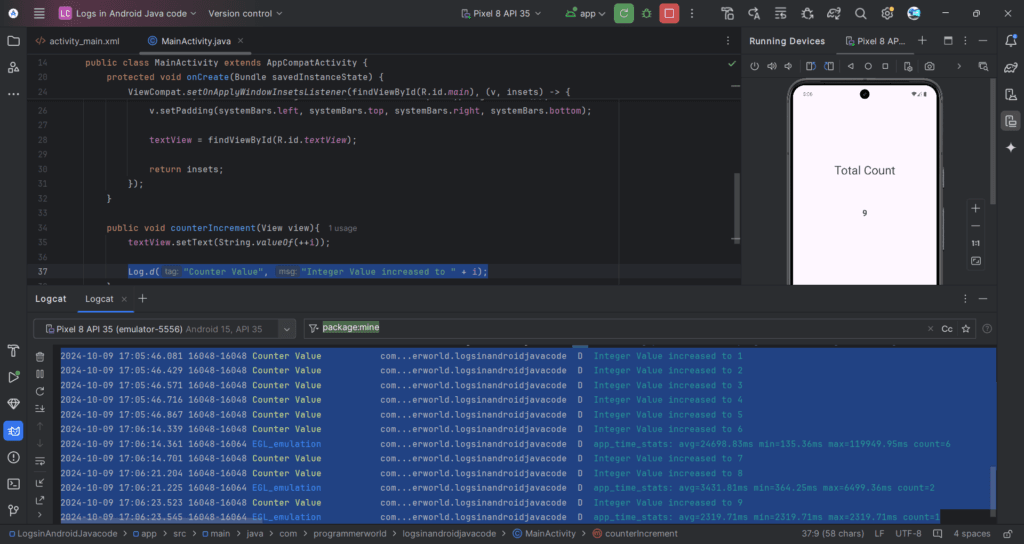
Logs statements printed in the Logcat:
2024-10-09 17:05:46.081 16048-16048 Counter Value com...erworld.logsinandroidjavacode D Integer Value increased to 1
2024-10-09 17:05:46.429 16048-16048 Counter Value com...erworld.logsinandroidjavacode D Integer Value increased to 2
2024-10-09 17:05:46.571 16048-16048 Counter Value com...erworld.logsinandroidjavacode D Integer Value increased to 3
2024-10-09 17:05:46.716 16048-16048 Counter Value com...erworld.logsinandroidjavacode D Integer Value increased to 4
2024-10-09 17:05:46.867 16048-16048 Counter Value com...erworld.logsinandroidjavacode D Integer Value increased to 5
2024-10-09 17:06:14.339 16048-16048 Counter Value com...erworld.logsinandroidjavacode D Integer Value increased to 6