This short video shows the steps to implement Palindrome logic (algorithm) in the Java code of your Android App.
In this tutorial it designs a very simple layout. It takes the input from the user through the Edit Text. It triggers the method to check the palindrome through a button click (onClick attribute) and then prints respective status in a text view.
To check the palindrome, the algorithm used is very simple. It iterates through the characters of the word entered by the user.
It then compares the first character with last character, second with second last and so on till the middle of the word. If any character it finds different then it raises a flag in the form of a boolean variable.
Based on this boolean variable value, it prints the respective status in the text view whether a particular word is Palindrome or not.
Please note, it uses the ASCII value of characters to do the comparison. So, lower case and upper case are treated differently in the comparison. So, the same word which is Palindrome in case insensitive environment may behave like it is not a palindrome.
Now, to make the comparison insensitive of case, one can convert the string to a particular case using either toUpperCase or toLowerCase APIs as shown in the video towards the end.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Source Code:
package com.programmerworld.palindromeapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView textViewStatus;
private EditText editTextInputWord;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textViewStatus = findViewById(R.id.textViewStatus);
editTextInputWord = findViewById(R.id.editTextTextInputWord);
}
public void buttonCheckPalindrome(View view){
char[] charInput = editTextInputWord.getText().toString().toCharArray();
int intLength = charInput.length;
boolean isPalindrome = true;
for (int i=0; i<intLength/2; i++){
if (charInput[i] != charInput[intLength-1-i]){
isPalindrome = false;
break;
}
}
if (isPalindrome){
textViewStatus.setText("Palindrome");
}
else {
textViewStatus.setText("NOT Palindrome");
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textViewStatus"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.47" />
<EditText
android:id="@+id/editTextTextInputWord"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="87dp"
android:layout_marginTop="39dp"
android:ems="10"
android:hint="@string/type_your_word_here"
android:inputType="textPersonName"
android:textAlignment="center"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
android:autofillHints="" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="106dp"
android:layout_marginTop="50dp"
android:onClick="buttonCheckPalindrome"
android:text="@string/check_palindrome"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextTextInputWord" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.programmerworld.palindromeapp">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
google()
jcenter()
}
dependencies {
classpath "com.android.tools.build:gradle:4.0.1"
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
apply plugin: 'com.android.application'
android {
compileSdkVersion 30
buildToolsVersion "29.0.3"
defaultConfig {
applicationId "com.programmerworld.palindromeapp"
minSdkVersion 26
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
implementation fileTree(dir: "libs", include: ["*.jar"])
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'androidx.constraintlayout:constraintlayout:2.0.4'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
}
Screenshots:
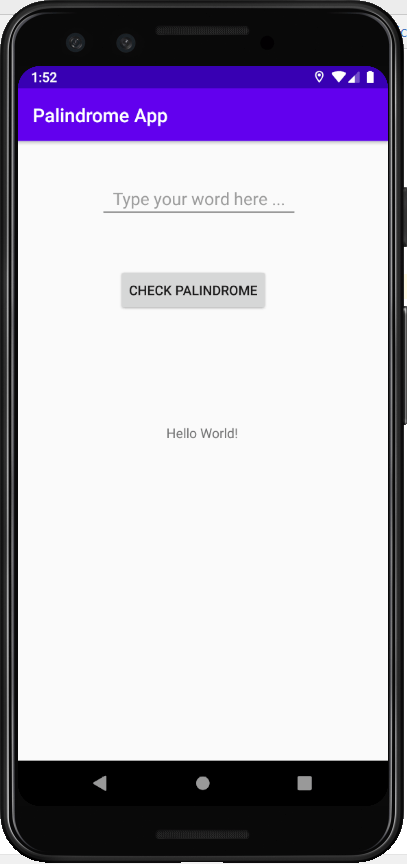
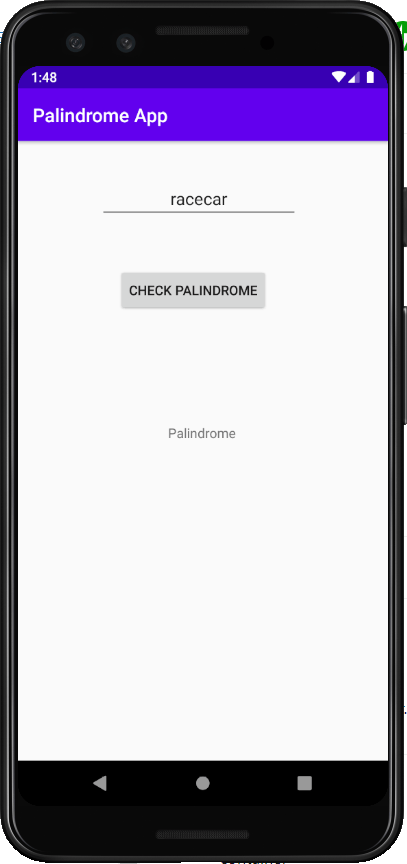
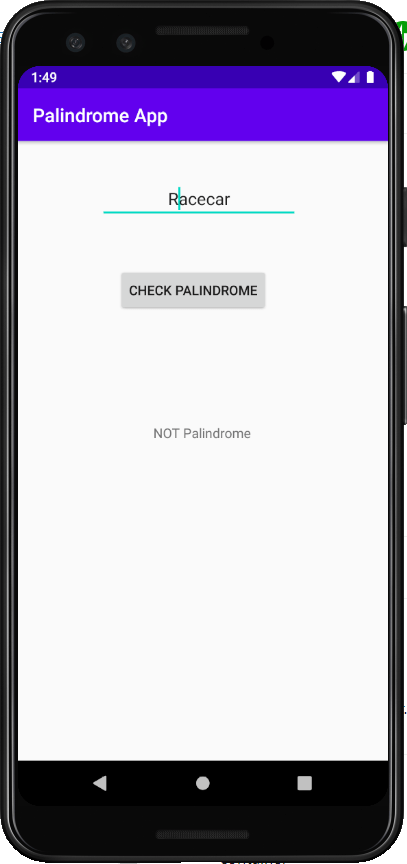
Some other examples of Palindrome words are: level, civic and racecar.
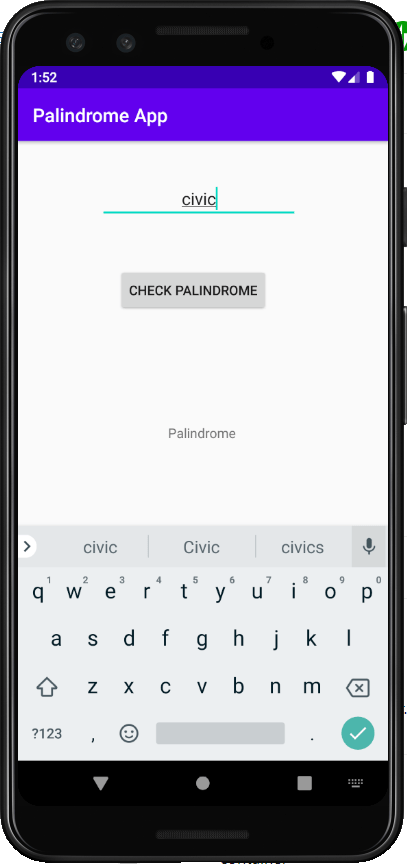