This guide outlines the conversion process for running existing Java-based Android apps on both web and mobile platforms through Dart, the language powering Flutter. This enables multi-platform deployment with a single codebase for efficient development and wider app reach.
This guide explores the process of migrating existing Android applications developed in Java to Dart, the programming language powering Flutter. By leveraging Dart’s capabilities, developers can achieve multi-platform deployment, extending the reach of their applications to both web and mobile environments. This approach offers several benefits, including code maintainability due to a unified codebase and the ability to leverage Flutter’s rich set of widgets and functionalities for a streamlined development experience.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
Java Code:
package com.programmerworld.counterandroidapp;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class MainActivity extends AppCompatActivity {
private int value = 0;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
return insets;
});
textView = findViewById(R.id.textView);
}
public void increment(View view) {
value = value + 5;
textView.setText(String.valueOf(value));
}
}
Dart Code:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int value = 0;
@override
Widget build(BuildContext context) {
return Scaffold(
body: Padding(
padding: EdgeInsets.only(
left: MediaQuery.of(context).viewInsets.left,
top: MediaQuery.of(context).viewInsets.top,
right: MediaQuery.of(context).viewInsets.right,
bottom: MediaQuery.of(context).viewInsets.bottom,
),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'$value',
style: TextStyle(fontSize: 32),
),
ElevatedButton(
onPressed: () => setState(() => value += 5),
child: Text('Increment'),
),
],
),
],
),
),
);
}
}
Screenshots:
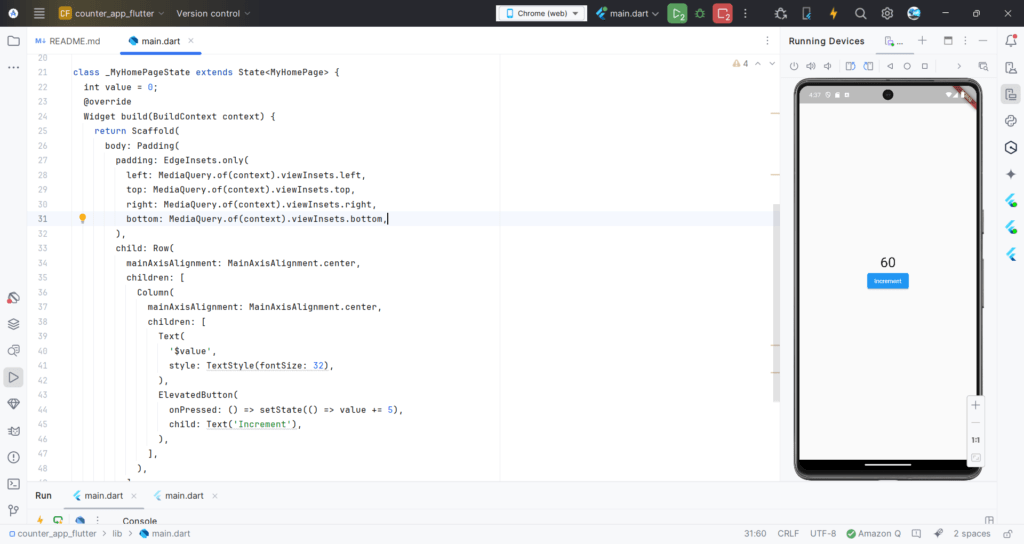
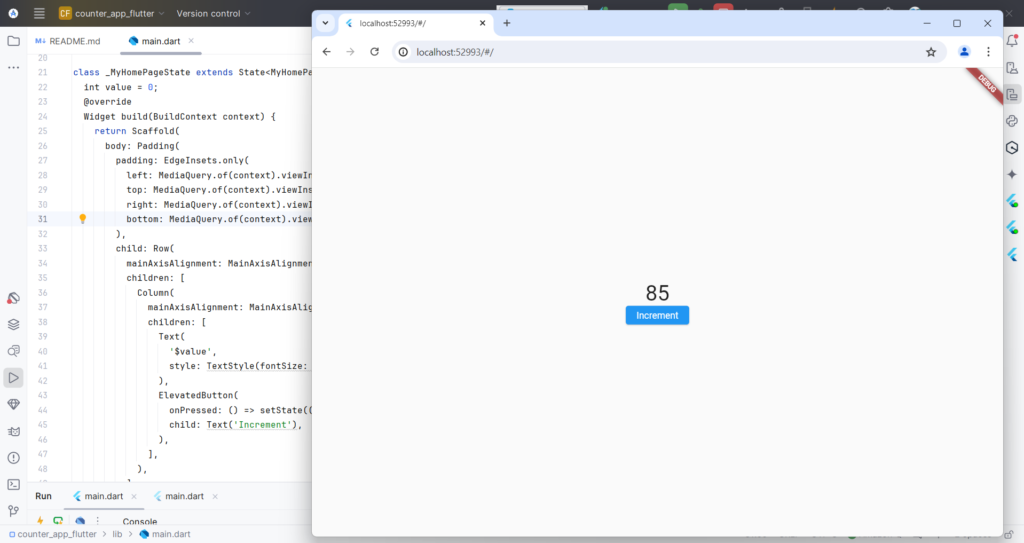
Gemini code conversion:
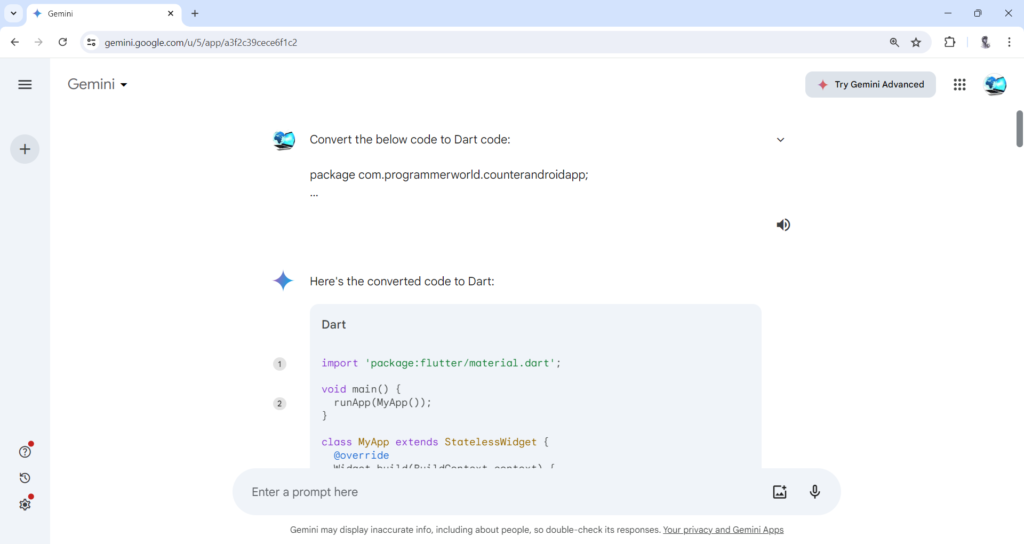