This video shows the steps to use Google’s App Password approach to authenticate the 3rd party Gmail client Android App. This uses the basic code from this page – https://programmerworld.co/android/how-to-send-email-using-gmail-smtp-server-directly-from-your-android-app/
Then it shows the steps to create a new Gmail account and create App Password to enable it to send the emails from the Gmail account. In this demo it uses dummy phone number and Gmail account. Do not use the same for your trial. I hope you like this video.
For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.gmailclientapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import java.util.Properties;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.AddressException;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class MainActivity extends AppCompatActivity {
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
}
public void buttonSendEmail(View view){
try {
String stringSenderEmail = "senderemail964@gmail.com";
String stringReceiverEmail = "receiveremail963@gmail.com";
String stringPasswordSenderEmail = "zukeypzofcofwzyy";
String stringHost = "smtp.gmail.com";
Properties properties = System.getProperties();
properties.put("mail.smtp.host", stringHost);
properties.put("mail.smtp.port", "465");
properties.put("mail.smtp.ssl.enable", "true");
properties.put("mail.smtp.auth", "true");
javax.mail.Session session = Session.getInstance(properties, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(stringSenderEmail, stringPasswordSenderEmail);
}
});
MimeMessage mimeMessage = new MimeMessage(session);
mimeMessage.addRecipient(Message.RecipientType.TO, new InternetAddress(stringReceiverEmail));
mimeMessage.setSubject("Subject: Android App email");
mimeMessage.setText("Hello Programmer, \n\nProgrammer World has sent you this 2nd email. \n\n Cheers!\nProgrammer World");
Thread thread = new Thread(new Runnable() {
@Override
public void run() {
try {
Transport.send(mimeMessage);
} catch (MessagingException e) {
textView.setText("MessagingException1");
e.printStackTrace();
}
}
});
thread.start();
} catch (AddressException e) {
textView.setText("AddressException");
e.printStackTrace();
} catch (MessagingException e) {
textView.setText("MessagingException2");
e.printStackTrace();
}
textView.setText("Email sent SUCCESSFULLY!!!");
}
}
plugins {
id 'com.android.application'
}
android {
namespace 'com.programmerworld.gmailclientapp'
compileSdk 33
defaultConfig {
applicationId "com.programmerworld.gmailclientapp"
minSdk 33
targetSdk 33
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
packagingOptions{
exclude 'META-INF/NOTICE.md'
exclude 'META-INF/LICENSE.md'
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.6.1'
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.4'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.5'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.5.1'
implementation 'com.sun.mail:android-mail:1.6.6'
implementation 'com.sun.mail:android-activation:1.6.7'
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.GmailClientApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="127dp"
android:layout_marginTop="67dp"
android:onClick="buttonSendEmail"
android:text="Send the Email"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
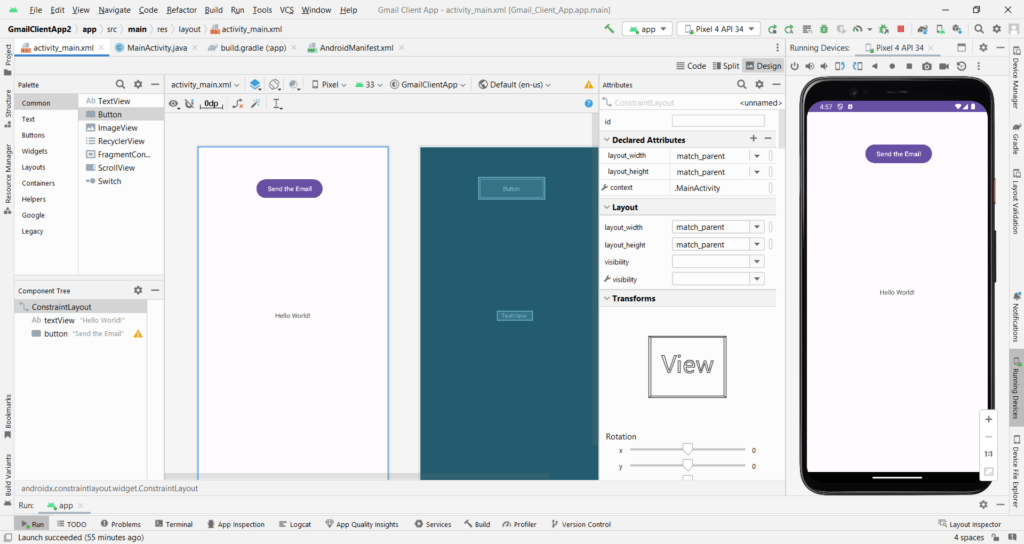
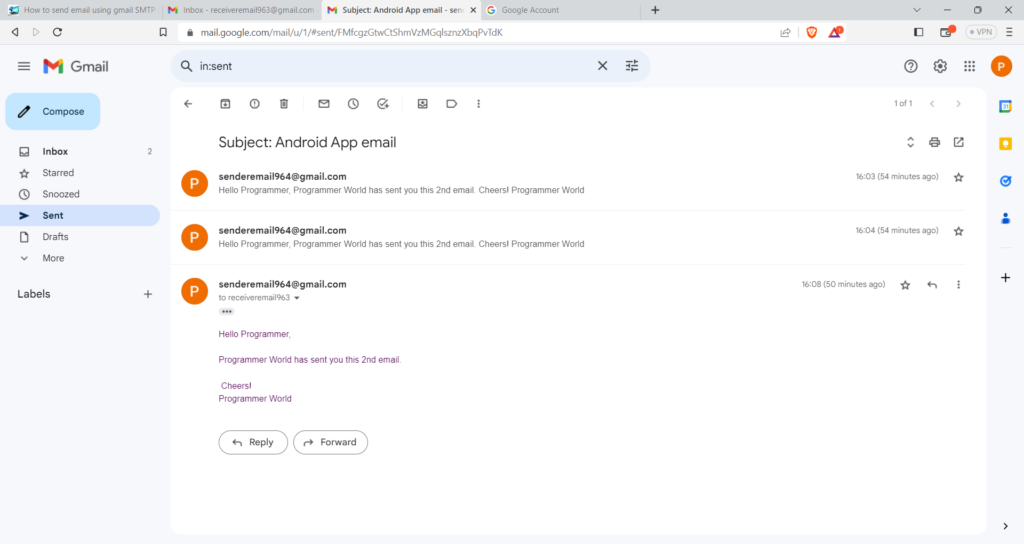
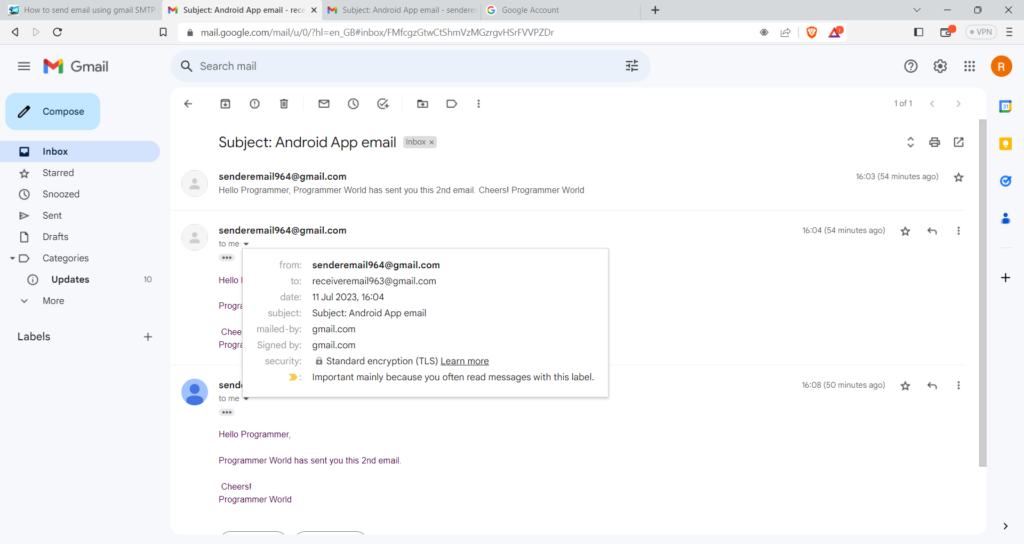