This video shows the steps to implement a rotating/ spinning roulette when in your Android App.
It uses simple image view to insert a wheel image and a pointer arrow image. It rotates the roulette wheel image at some angle based on the random number generated in the code.
It then captures the value which comes aligned to the pointer arrow mark. It uses getRotation API to get the rotation of the image and then compute respective number from the rotation values (which is in degrees).
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.rotatingroulettewheelapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import java.util.Random;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = findViewById(R.id.imageView);
textView = findViewById(R.id.textView);
}
public void buttonImageRouletteWheel(View view){
Runnable runnable = new Runnable() {
@Override
public void run() {
float floatRandomRotation = new Random().nextFloat()*500;
imageView.setRotation(floatRandomRotation);
imageView.refreshDrawableState();
float floatGetRotation = 10-(imageView.getRotation()%360)/36;
floatGetRotation = (float) Math.ceil(floatGetRotation);
textView.setText(String.valueOf(floatGetRotation));
}
};
Handler handler = new Handler(Looper.getMainLooper());
for (int i=1; i<20; i++){
handler.postDelayed(runnable, i*1000);
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="316dp"
android:layout_height="262dp"
android:layout_marginStart="47dp"
android:layout_marginTop="27dp"
android:contentDescription="@string/app_name"
android:onClick="buttonImageRouletteWheel"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView2"
app:srcCompat="@mipmap/roulette_wheel_foreground" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="151dp"
android:layout_marginTop="72dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@mipmap/pointer_foreground" />
<TextView
android:id="@+id/textView"
android:layout_width="129dp"
android:layout_height="56dp"
android:layout_marginStart="141dp"
android:layout_marginTop="53dp"
android:text="TextView"
android:textAlignment="center"
android:textSize="24sp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.RotatingRouletteWheelApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data
android:name="android.app.lib_name"
android:value="" />
</activity>
</application>
</manifest>
Screenshots of Android Studio Environment:
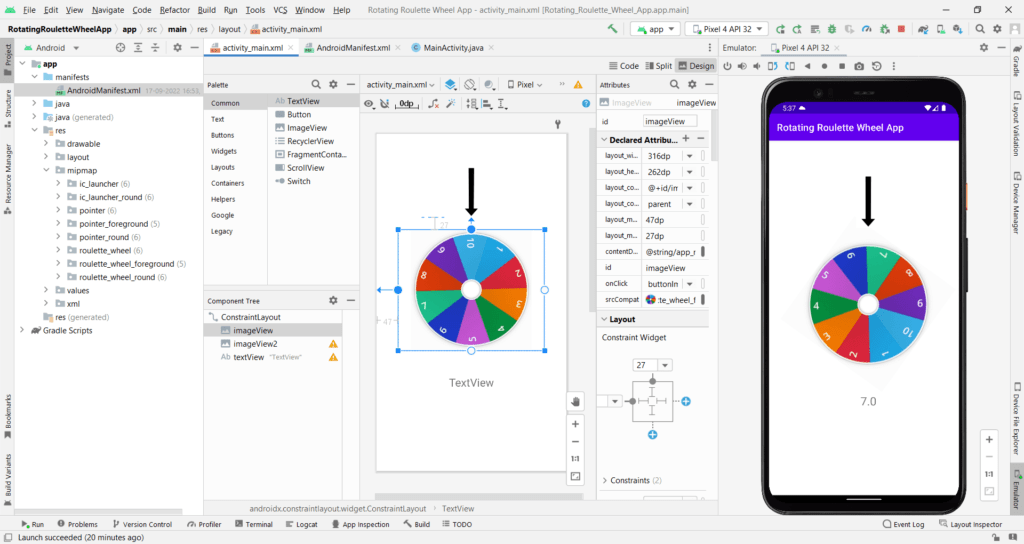
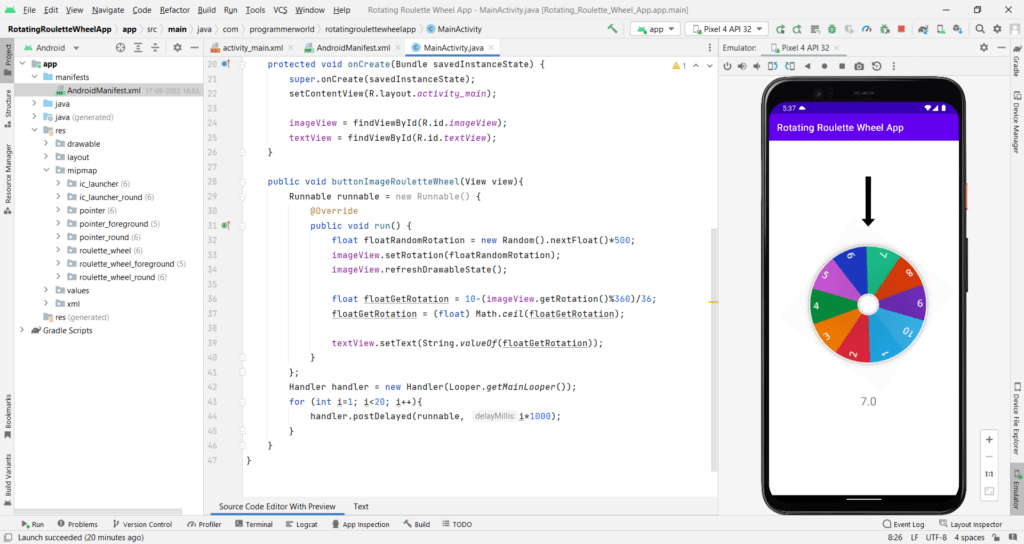
Images used in this session is available below:
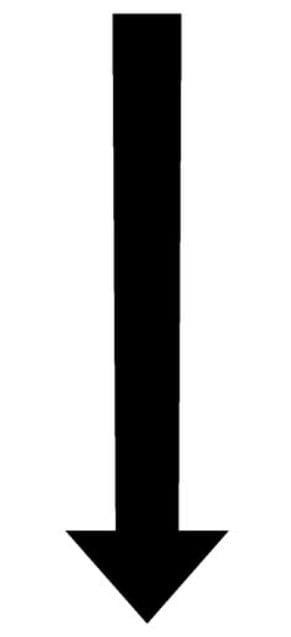
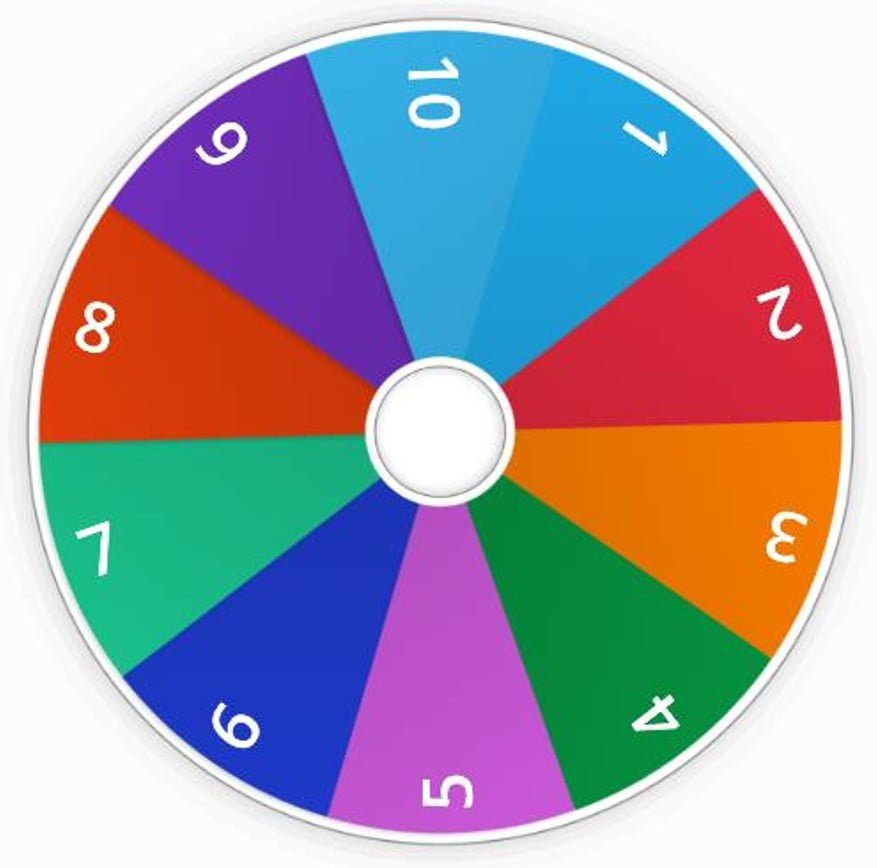