This video shows the code for displaying a image from Android’s local storage (Download folder) into a WebView. The image is converted into Bitmap and then loaded in the WebView using the loadDataWithBaseURL API.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.bitmapinawebview;
import static android.Manifest.permission.READ_MEDIA_IMAGES;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.storage.StorageManager;
import android.os.storage.StorageVolume;
import android.util.Base64;
import android.view.View;
import android.webkit.WebView;
import android.widget.ImageView;
import java.io.ByteArrayOutputStream;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private WebView webView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{READ_MEDIA_IMAGES},
PackageManager.PERMISSION_GRANTED);
webView = findViewById(R.id.webView);
imageView = findViewById(R.id.imageView);
}
public void buttonBitmapToWebView(View view){
// ImageView part of the code below
StorageManager storageManager = (StorageManager) getSystemService(STORAGE_SERVICE);
StorageVolume storageVolume = storageManager.getStorageVolumes().get(0); // 0 for internal storage
String stringFile = storageVolume.getDirectory().getPath() + "/Download/flower.jpg";
Bitmap bitmapImage = BitmapFactory.decodeFile(stringFile);
imageView.setImageBitmap(bitmapImage);
// WebView part of the code below
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
bitmapImage.compress(Bitmap.CompressFormat.PNG, 10, byteArrayOutputStream);
byte[] bytesArray = byteArrayOutputStream.toByteArray();
String stringImageBytes = Base64.encodeToString(bytesArray, Base64.DEFAULT);
String stringHTMLURL = "<html><body><img src='IMAGE'/></body></html>";
stringHTMLURL = stringHTMLURL.replace("IMAGE", "data:image/png;base64," + stringImageBytes);
webView.loadDataWithBaseURL("file:///android_asset/", stringHTMLURL,
"text/html",
"utf-8", "");
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.READ_MEDIA_IMAGES"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.BitmapInAWebView"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="124dp"
android:layout_marginTop="16dp"
android:onClick="buttonBitmapToWebView"
android:text="Bitmap into WebView"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="189dp"
android:layout_height="155dp"
android:layout_marginStart="108dp"
android:layout_marginTop="16dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
app:srcCompat="@drawable/ic_launcher_background" />
<WebView
android:id="@+id/webView"
android:layout_width="356dp"
android:layout_height="386dp"
android:layout_marginStart="27dp"
android:layout_marginTop="28dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
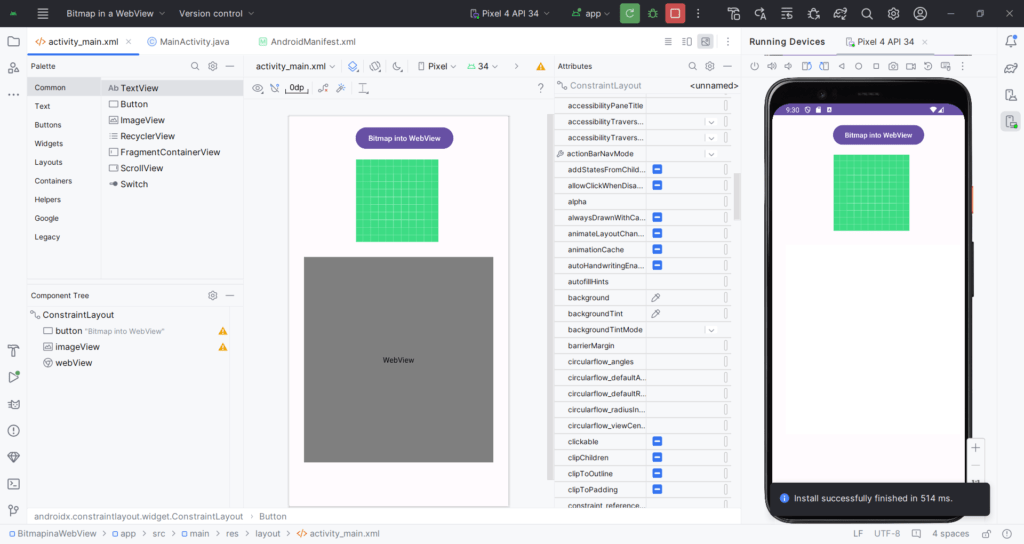
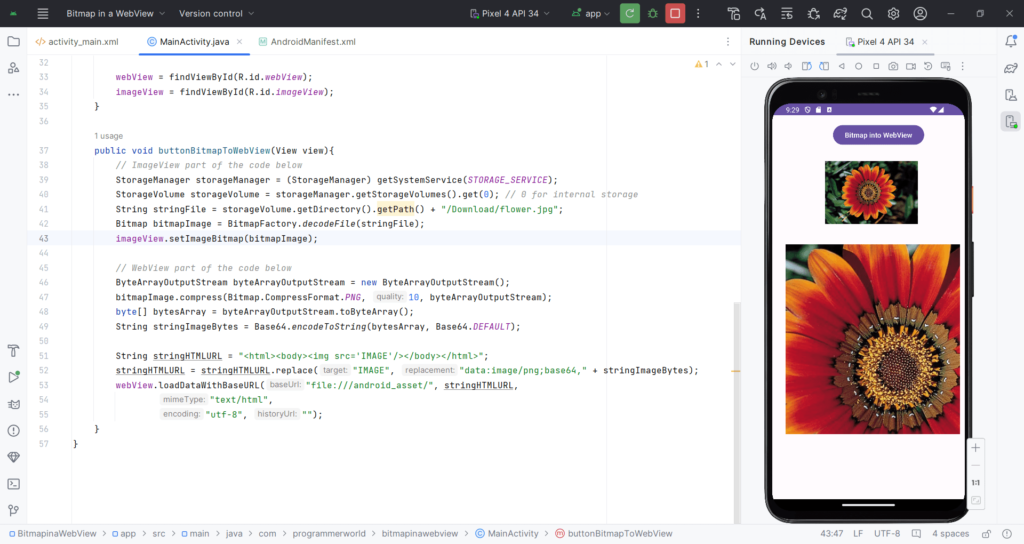
File used:
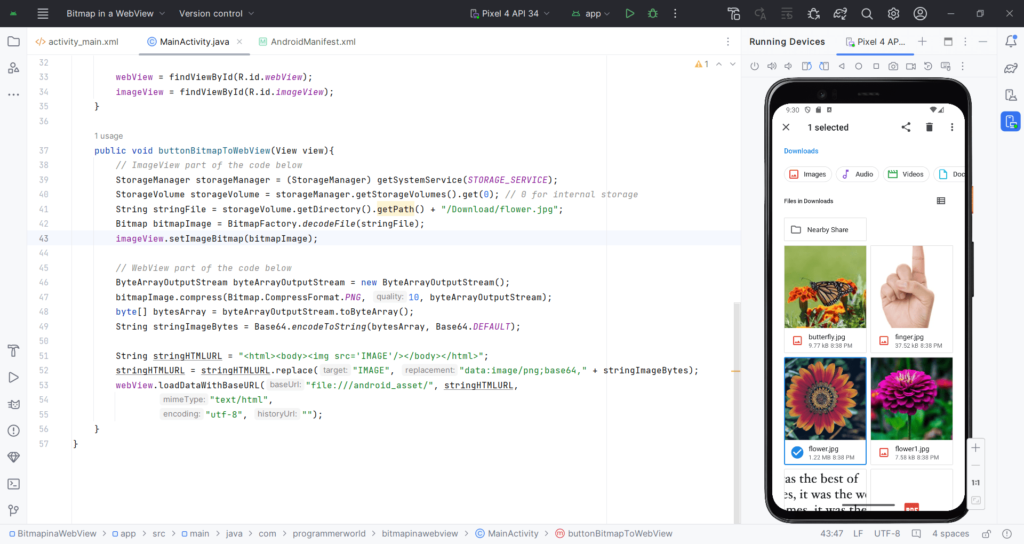
Excerpt:
The given post features a tutorial video and the complete source code for a procedure to display an image from Android’s local storage into a WebView. The image is retrieved from the download folder, converted into a Bitmap, and loaded into the WebView using the loadDataWithBaseURL API. It shares code for permissions, accessing storage, loading the image into an ImageView, and then converting it into a string using Base64 to load it onto a WebView. The detailed process further includes relevant Android XML design and manifest files. Links are provided for further assistance or queries.