In this video it shows the steps to develop your image recognition/ classification Android App using Google’s Artificial Intelligence (Gen AI) Gemini Tool.
To generate API Key – https://aistudio.google.com/app/apikey
To run Gemini App in browser – https://gemini.google.com/app
Webpage referred in this demonstration: https://programmerworld.co/android/how-to-integrate-googles-gemini-ai-in-android-app-java-code/
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.imagerecognitionusinggemini;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
import com.google.ai.client.generativeai.GenerativeModel;
import com.google.ai.client.generativeai.java.GenerativeModelFutures;
import com.google.ai.client.generativeai.type.Content;
import com.google.ai.client.generativeai.type.GenerateContentResponse;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
public class MainActivity extends AppCompatActivity {
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
textView = findViewById(R.id.textView);
return insets;
});
}
public void buttonImageRecognitionGemini(View view){
GenerativeModel generativeModel = new GenerativeModel("gemini-pro-vision",
"AIza5yDHr77CJNXjjWD1X8GQTePUG08kF-gCzwc");
GenerativeModelFutures model = GenerativeModelFutures.from(generativeModel);
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.mipmap.flower_foreground);
Content content = new Content.Builder()
.addText("What is this image?")
.addImage(bitmap)
.build();
ListenableFuture<GenerateContentResponse> response = model.generateContent(content);
Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() {
@Override
public void onSuccess(GenerateContentResponse result) {
String resultText = result.getText();
textView.setText(resultText);
}
@Override
public void onFailure(Throwable t) {
textView.setText(t.toString());
}
}, this.getMainExecutor());
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.ImageRecognitionUsingGemini"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
plugins {
alias(libs.plugins.androidApplication)
}
android {
namespace = "com.programmerworld.imagerecognitionusinggemini"
compileSdk = 34
defaultConfig {
applicationId = "com.programmerworld.imagerecognitionusinggemini"
minSdk = 30
targetSdk = 34
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
}
dependencies {
implementation(libs.appcompat)
implementation(libs.material)
implementation(libs.activity)
implementation(libs.constraintlayout)
implementation(libs.generativeai)
testImplementation(libs.junit)
androidTestImplementation(libs.ext.junit)
androidTestImplementation(libs.espresso.core)
// Required for one-shot operations (to use `ListenableFuture` from Reactive Streams)
implementation(libs.guava)
// Required for streaming operations (to use `Publisher` from Guava Android)
implementation(libs.reactive.streams)
}
[versions]
agp = "8.3.1"
guava = "31.0.1-android"
junit = "4.13.2"
junitVersion = "1.1.5"
espressoCore = "3.5.1"
appcompat = "1.6.1"
material = "1.11.0"
activity = "1.8.0"
constraintlayout = "2.1.4"
generativeai = "0.2.2"
reactiveStreams = "1.0.4"
[libraries]
guava = { module = "com.google.guava:guava", version.ref = "guava" }
junit = { group = "junit", name = "junit", version.ref = "junit" }
ext-junit = { group = "androidx.test.ext", name = "junit", version.ref = "junitVersion" }
espresso-core = { group = "androidx.test.espresso", name = "espresso-core", version.ref = "espressoCore" }
appcompat = { group = "androidx.appcompat", name = "appcompat", version.ref = "appcompat" }
material = { group = "com.google.android.material", name = "material", version.ref = "material" }
activity = { group = "androidx.activity", name = "activity", version.ref = "activity" }
constraintlayout = { group = "androidx.constraintlayout", name = "constraintlayout", version.ref = "constraintlayout" }
generativeai = { group = "com.google.ai.client.generativeai", name = "generativeai", version.ref = "generativeai" }
reactive-streams = { module = "org.reactivestreams:reactive-streams", version.ref = "reactiveStreams" }
[plugins]
androidApplication = { id = "com.android.application", version.ref = "agp" }
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textSize="34sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:onClick="buttonImageRecognitionGemini"
android:text="Image Recognition Gemini"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="203dp"
android:layout_height="162dp"
android:layout_marginTop="23dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
app:srcCompat="@mipmap/flower_foreground" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
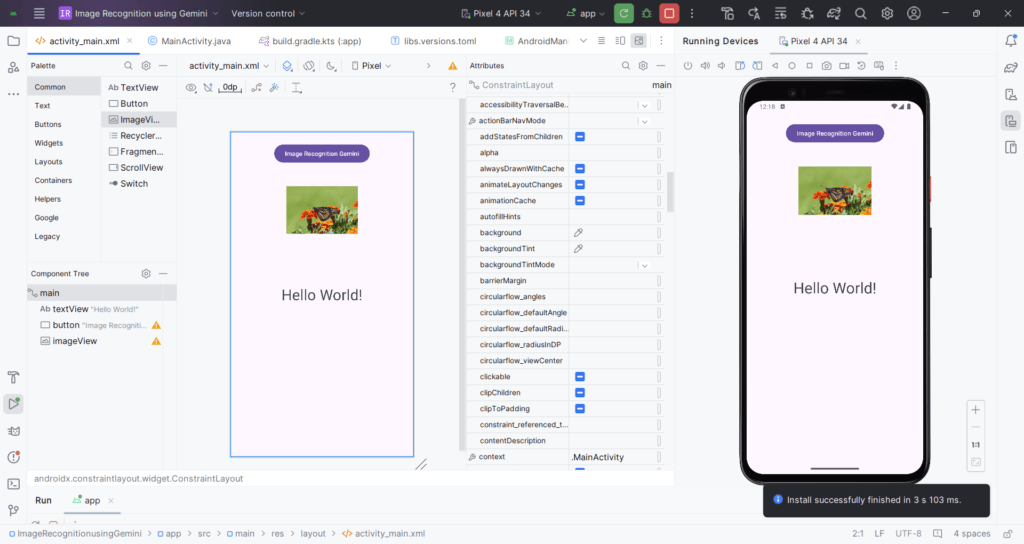
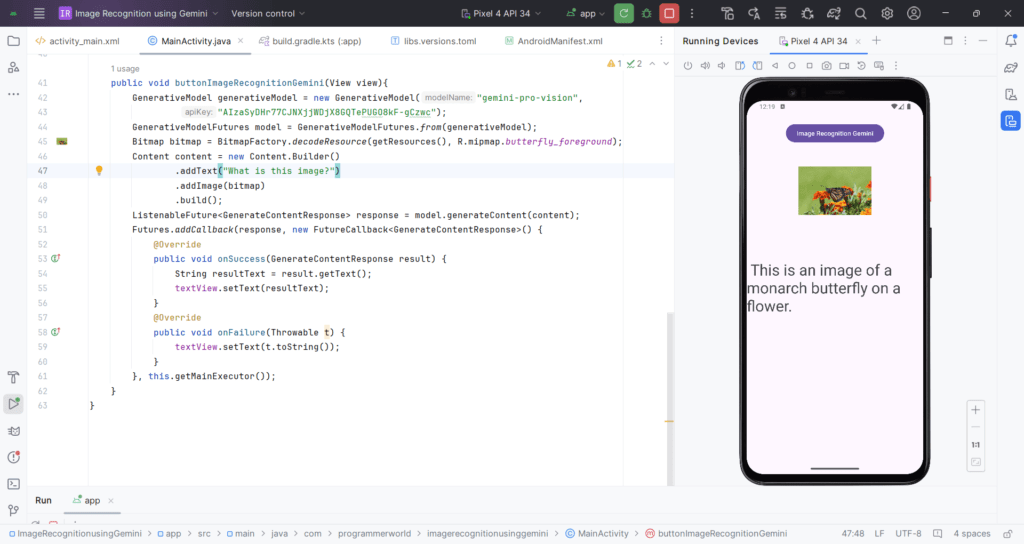
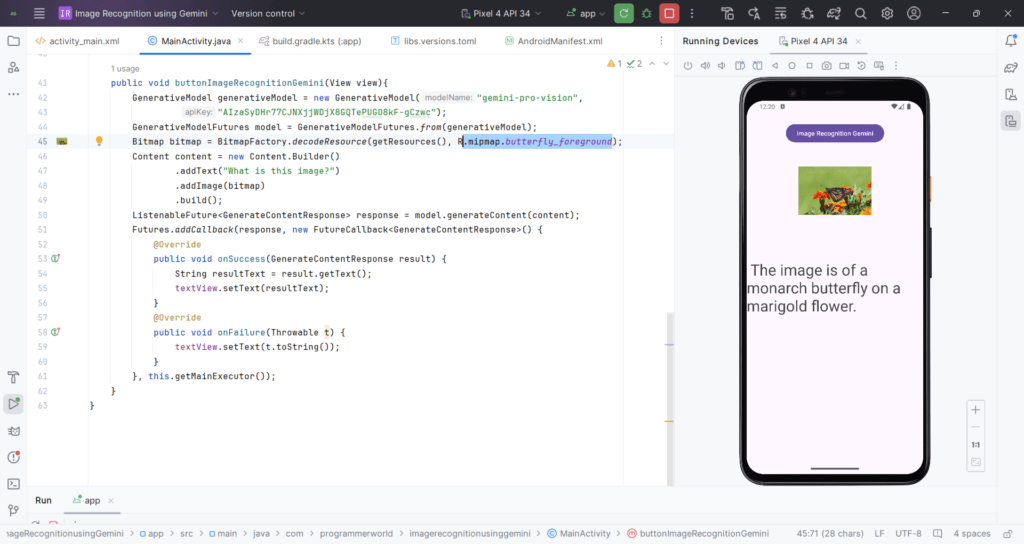
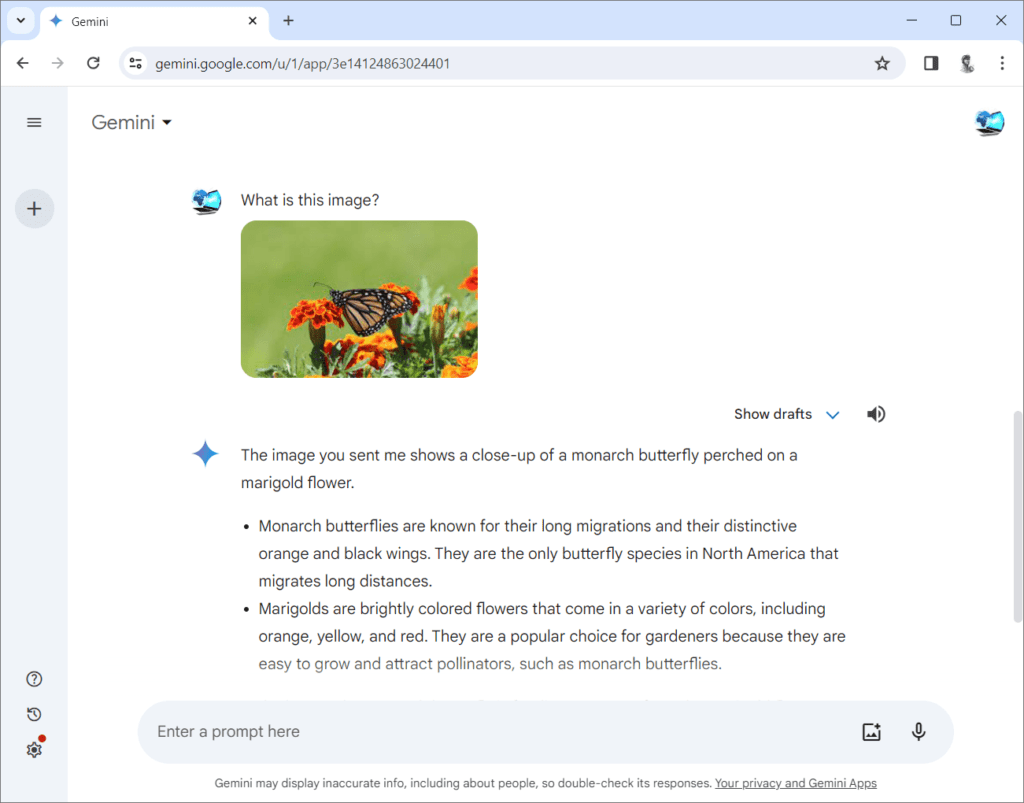
Testing with another image:
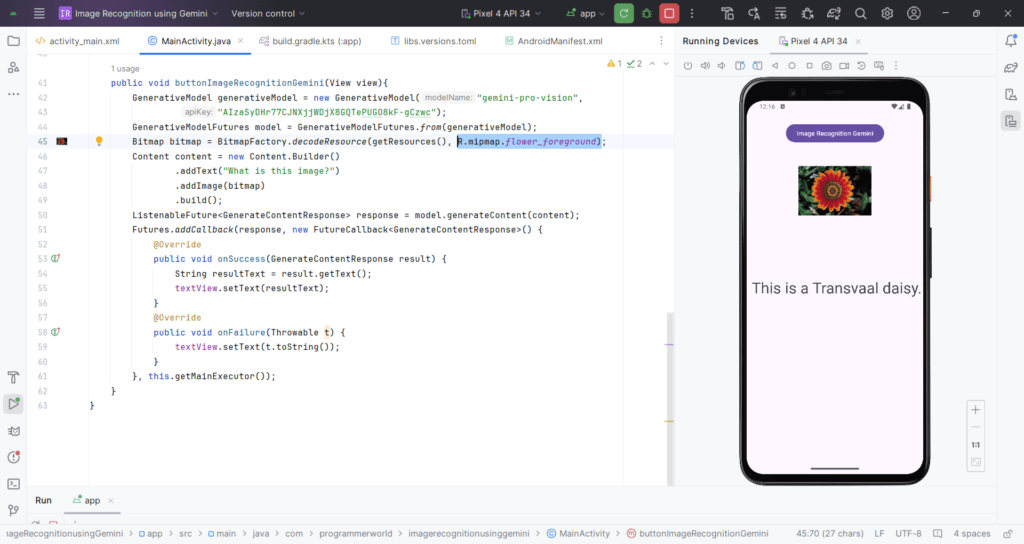
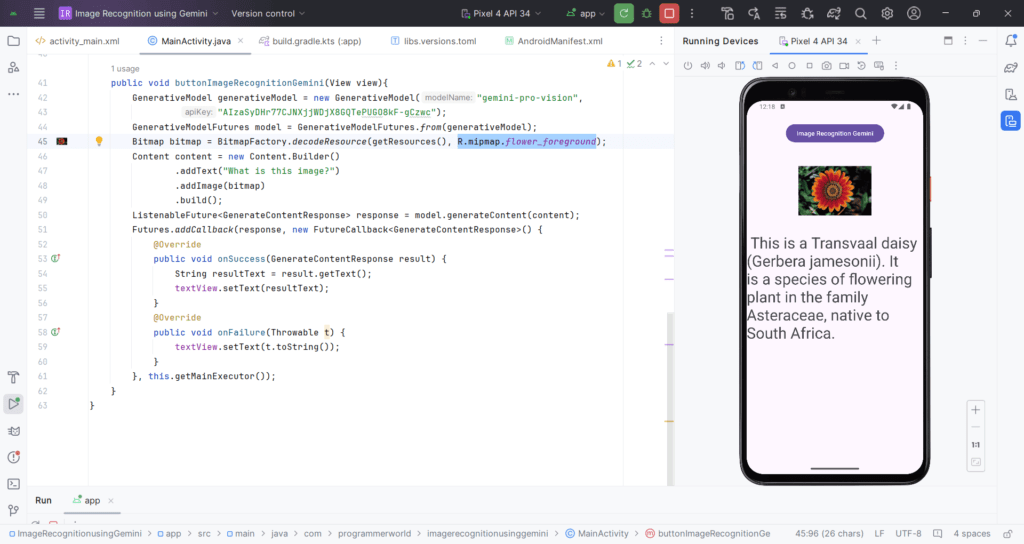
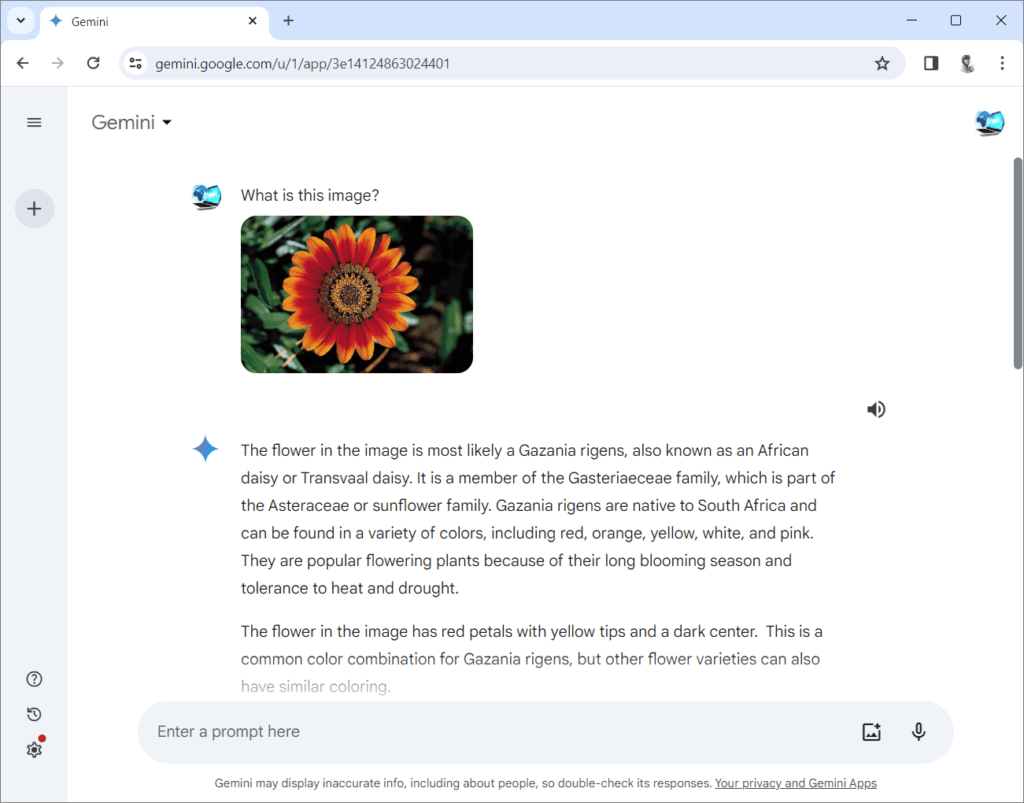
Project Folder: Access the complete project folder at the below link on payment of USD 9:
https://drive.google.com/file/d/1xdCkNc7kWJzaJV6z6hXLZJePZhaJOrn-/view?usp=sharing
Excerpt by GenAI:
The provided content describes the steps to develop an image recognition/classification Android app using Google’s Artificial Intelligence (Gen AI) Gemini Tool. It includes details on generating the API key and running the Gemini app in the browser. The demonstration references a webpage and provides contact information for inquiries. Additionally, the content includes the source code for the Android app, XML layout, and necessary dependencies. Screenshots of the app with sample images are also provided.