This video shows the steps to quickly fix android.os.NetworkOnMainThreadException in your Android Studio Java code.
The fix shown is more of a workaround. Ideal fix would be to run all the network related tasks in a separate thread using async methods.
However, in this video it shows how one can quickly override StrictMode Thread Poilcy by creating a local thread policy and allowing the network related tasks on the main thread which is not the perfect solution. This solution should be used only if the developer knows what kind of Network operation are being done in the main thread. He should be confident that it will not lead to long waiting period in the main thread as it can create issues for the complete App.
In this video it first reproduces the issue by loading the image from below URL in an imageView:
https://i0.wp.com/programmerworld.co/wp-content/uploads/2023/05/flower.png?w=977&ssl=1
Then it uses the local thread policy to override it in the StrictMode’s Thread policy.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.networkonmainthreadexceptionfix;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.StrictMode;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URL;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = findViewById(R.id.imageView);
textView = findViewById(R.id.textView);
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder()
.permitAll().build();
StrictMode.setThreadPolicy(policy);
}
public void buttonInternetUsage(View view){
String stringURL = "https://i0.wp.com/programmerworld.co/wp-content/uploads/2023/05/flower.png?w=977&ssl=1";
try {
InputStream inputStream = (InputStream) new URL(stringURL).getContent();
Bitmap bitmap = BitmapFactory.decodeStream(inputStream);
inputStream.close();
imageView.setImageBitmap(bitmap);
} catch (Exception e) {
textView.setText(e.toString());
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.NetworkOnMainThreadExceptionFix"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="249dp"
android:layout_height="86dp"
android:layout_marginStart="88dp"
android:layout_marginTop="55dp"
android:onClick="buttonInternetUsage"
android:text="Load from URL"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="239dp"
android:layout_height="217dp"
android:layout_marginStart="98dp"
android:layout_marginTop="260dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
app:srcCompat="@drawable/ic_launcher_background" />
<TextView
android:id="@+id/textView"
android:layout_width="321dp"
android:layout_height="54dp"
android:layout_marginStart="45dp"
android:layout_marginTop="32dp"
android:text="TextView"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
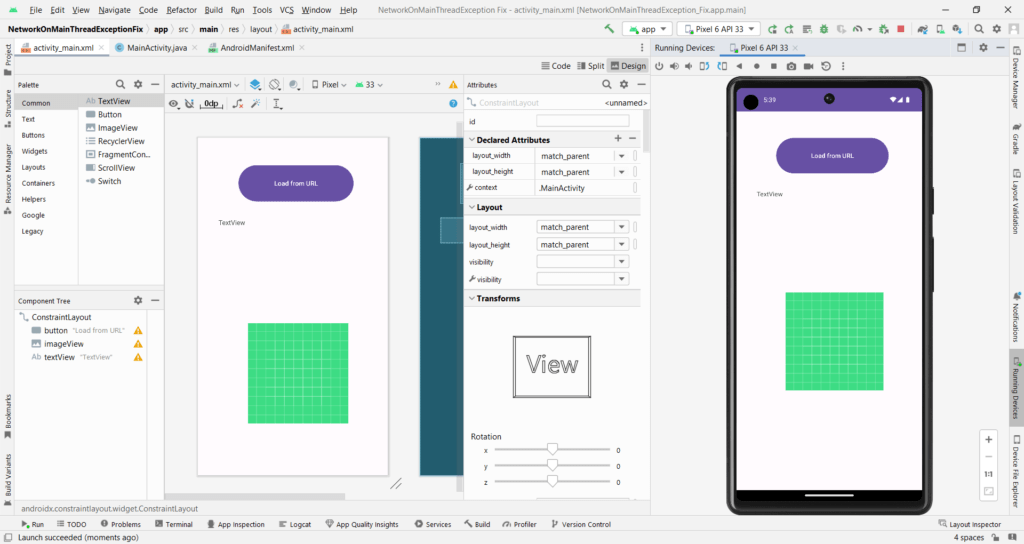
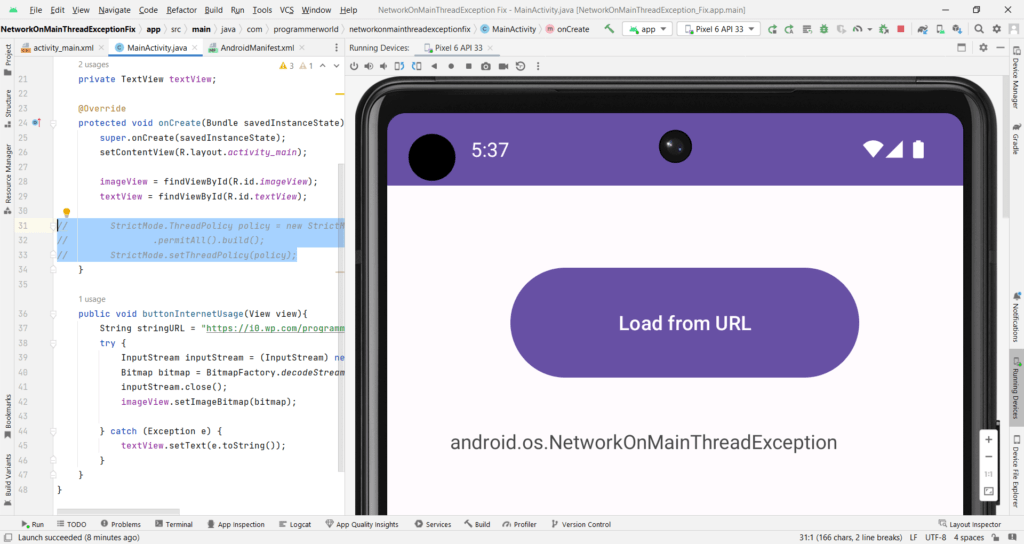
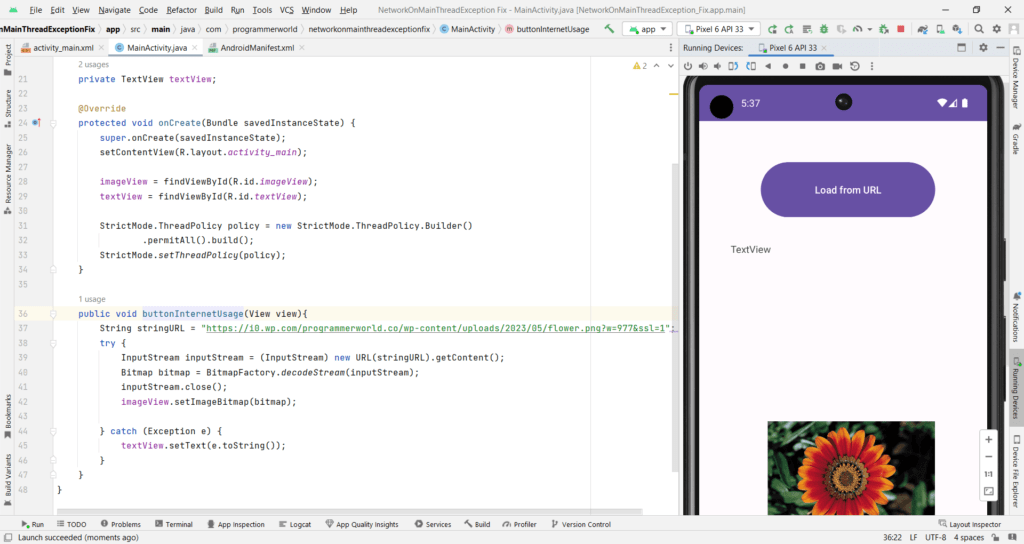