In this video it shows the code to read the Cache of your Android app. It also adds a WebView just to enable few folders and files in the cache directory of the App. It uses the Preference API from the AndroidX library. The dependencies for which is listed in the below link: https://developer.android.com/jetpack/androidx/releases/preference#kts
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.cachefilesanddirofapp;
import androidx.appcompat.app.AppCompatActivity;
import androidx.preference.Preference;
import android.os.Bundle;
import android.view.View;
import android.webkit.WebView;
import android.widget.ArrayAdapter;
import android.widget.ListAdapter;
import android.widget.ListView;
import android.widget.Toast;
import java.io.File;
public class MainActivity extends AppCompatActivity {
private ListView listView;
private WebView webView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView = findViewById(R.id.listView);
webView = findViewById(R.id.webView);
}
public void buttonListCacheFiles(View view){
webView.setVisibility(View.INVISIBLE);
Preference preference = new Preference(this);
File file = new File(preference.getContext().getCacheDir().getPath());
File[] files = file.listFiles();
if (files.length == 0){
Toast.makeText(this, "NO CACHE", Toast.LENGTH_LONG).show();
return;
}
StringBuilder stringBuilder = new StringBuilder();
for (File file1:files){
stringBuilder.append("\n*" + file1.toString());
File[] files1 = file1.listFiles();
for (File file2:files) {
stringBuilder.append("\n\n--" + file2.toString());
File[] files2 = file2.listFiles();
for (File file3:files) {
stringBuilder.append("\n\n++++" + file3.toString());
}
}
}
ListAdapter listAdapter = new ArrayAdapter<String>(MainActivity.this,
android.R.layout.simple_list_item_1,
new String[]{stringBuilder.toString()});
listView.setAdapter(listAdapter);
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.CacheFilesAndDirOfApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
plugins {
id("com.android.application")
}
android {
namespace = "com.programmerworld.cachefilesanddirofapp"
compileSdk = 34
defaultConfig {
applicationId = "com.programmerworld.cachefilesanddirofapp"
minSdk = 34
targetSdk = 34
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
}
dependencies {
implementation("androidx.appcompat:appcompat:1.6.1")
implementation("com.google.android.material:material:1.11.0")
implementation("androidx.constraintlayout:constraintlayout:2.1.4")
testImplementation("junit:junit:4.13.2")
androidTestImplementation("androidx.test.ext:junit:1.1.5")
androidTestImplementation("androidx.test.espresso:espresso-core:3.5.1")
val preference_version = "1.2.1"
// Java language implementation
implementation("androidx.preference:preference:$preference_version")
}
Screenshots:
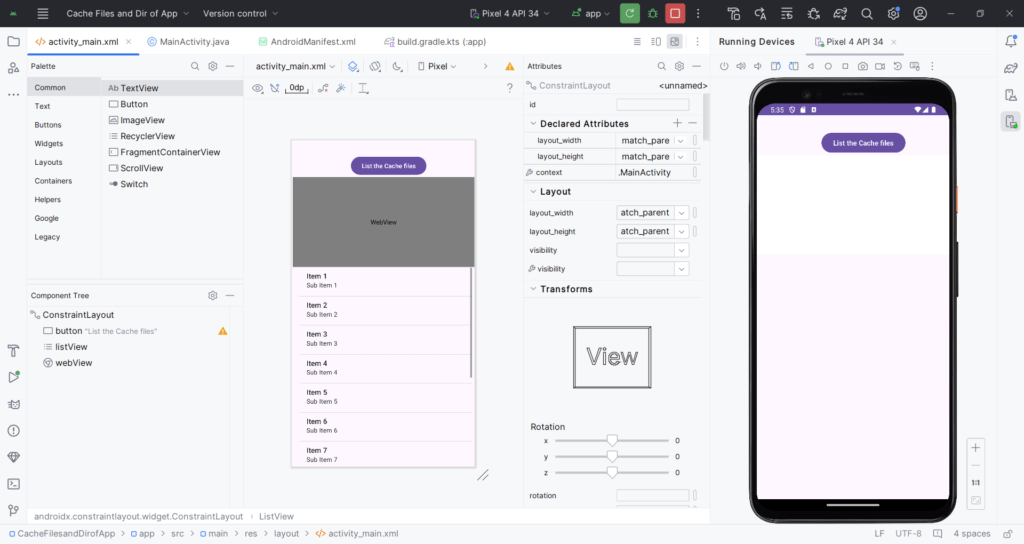
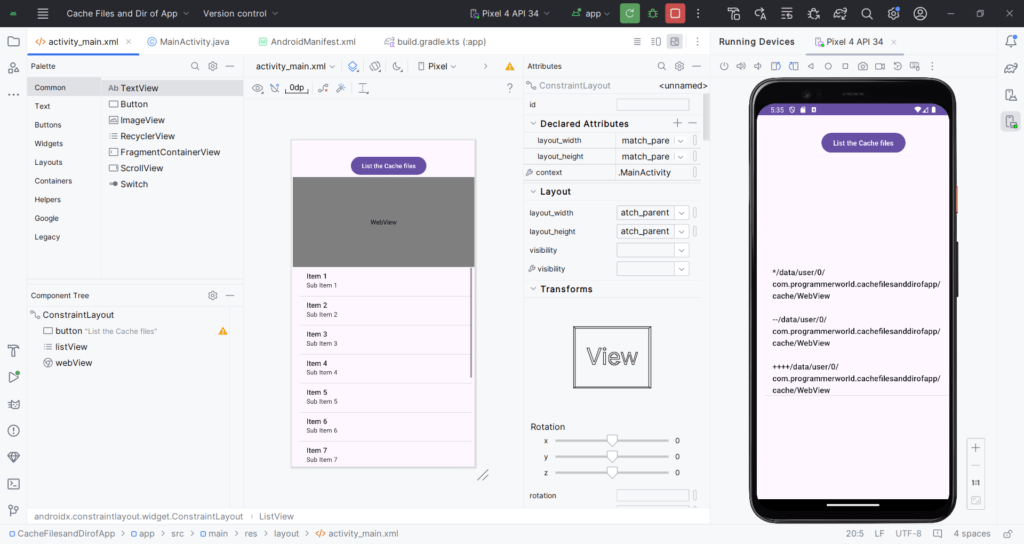
Excerpt:
The content includes a video tutorial showcasing how to read the Cache of an Android app using code. A WebView is added to allow folders and files to be visible in the app’s cache directory, utilizing the Preference API from the AndroidX library. The complete source code for the process is provided for developers, including the XML manifest and a list of necessary dependencies that need to be implemented. Communication channels for viewers to provide feedback, ask questions, or share their appreciation for the informative video have also been cited.