This video shows the steps to create a voice input or Audio recorder App using Speech Recognizer API in Android. This concept can be used to design your custom App in such a way that it takes voice command as an input to perform certain operations such as opening a file, starting another App like WhatsApp or calling a phone number or sending an SMS.
In this App it creates an ACTION_RECOGNIZE_SPEECH intent used to listen on a SpeechRecognizer API. It creates a simple Layout with a textView to show the words spoken and start/ stop button to start and stop listening the SpeechRecognizer. The testing of this App couldn’t be shown in the Emulator as the input source (microphone) of the Emulator sometimes doesn’t works with the Laptop. So, the testing during this video was done on a real phone connected to the laptop through USB cable.
We hope you like this video. For any query, suggestions or appreciations we will be glad to hear from you at: programmerworld1990@gmail.com
Source Code:
package com.example.myvoicecommandapp;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.speech.RecognitionListener;
import android.speech.RecognizerIntent;
import android.speech.SpeechRecognizer;
import android.view.View;
import android.widget.TextView;import java.util.ArrayList;
import static android.Manifest.permission.RECORD_AUDIO;
public class MainActivity extends AppCompatActivity {
private SpeechRecognizer speechRecognizer;
private Intent intentRecognizer;
private TextView textView;@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);ActivityCompat.requestPermissions(this, new String[]{RECORD_AUDIO}, PackageManager.PERMISSION_GRANTED);
textView = findViewById(R.id.textView);
intentRecognizer = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH);
intentRecognizer.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM);speechRecognizer = SpeechRecognizer.createSpeechRecognizer(this);
speechRecognizer.setRecognitionListener(new RecognitionListener() {
@Override
public void onReadyForSpeech(Bundle bundle) {}
@Override
public void onBeginningOfSpeech() {}
@Override
public void onRmsChanged(float v) {}
@Override
public void onBufferReceived(byte[] bytes) {}
@Override
public void onEndOfSpeech() {}
@Override
public void onError(int i) {}
@Override
public void onResults(Bundle bundle) {
ArrayList<String> matches = bundle.getStringArrayList(SpeechRecognizer.RESULTS_RECOGNITION);
String string = “”;
if(matches!=null){
string = matches.get(0);
textView.setText(string);
}}
@Override
public void onPartialResults(Bundle bundle) {}
@Override
public void onEvent(int i, Bundle bundle) {}
});
}public void StartButton(View view){
speechRecognizer.startListening(intentRecognizer);
}public void StopButton(View view){
speechRecognizer.stopListening();
}
}
<?xml version=”1.0″ encoding=”utf-8″?>
<manifest xmlns:android=”http://schemas.android.com/apk/res/android”
package=”com.example.myvoicecommandapp”><uses-permission android:name=”android.permission.RECORD_AUDIO”/>
<application
android:allowBackup=”true”
android:icon=”@mipmap/ic_launcher”
android:label=”@string/app_name”
android:roundIcon=”@mipmap/ic_launcher_round”
android:supportsRtl=”true”
android:theme=”@style/AppTheme”>
<activity android:name=”.MainActivity”>
<intent-filter>
<action android:name=”android.intent.action.MAIN” /><category android:name=”android.intent.category.LAUNCHER” />
</intent-filter>
</activity>
</application></manifest>
<?xml version=”1.0″ encoding=”utf-8″?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android=”http://schemas.android.com/apk/res/android”
xmlns:app=”http://schemas.android.com/apk/res-auto”
xmlns:tools=”http://schemas.android.com/tools”
android:layout_width=”match_parent”
android:layout_height=”match_parent”
tools:context=”.MainActivity”><TextView
android:id=”@+id/textView”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
android:text=”Hello World!”
app:layout_constraintBottom_toBottomOf=”parent”
app:layout_constraintLeft_toLeftOf=”parent”
app:layout_constraintRight_toRightOf=”parent”
app:layout_constraintTop_toTopOf=”parent” /><Button
android:id=”@+id/button”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
android:layout_marginStart=”166dp”
android:layout_marginTop=”103dp”
android:onClick=”StartButton”
android:text=”@string/start”
app:layout_constraintStart_toStartOf=”parent”
app:layout_constraintTop_toTopOf=”parent” /><Button
android:id=”@+id/button2″
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
android:layout_marginStart=”167dp”
android:layout_marginTop=”105dp”
android:onClick=”StopButton”
android:text=”@string/stop”
app:layout_constraintStart_toStartOf=”parent”
app:layout_constraintTop_toBottomOf=”@+id/textView” /></androidx.constraintlayout.widget.ConstraintLayout>
Screenshots of Testing done on the real phone:
On speaking: “hello … hello world”
On Speaking: “this is programmer world”
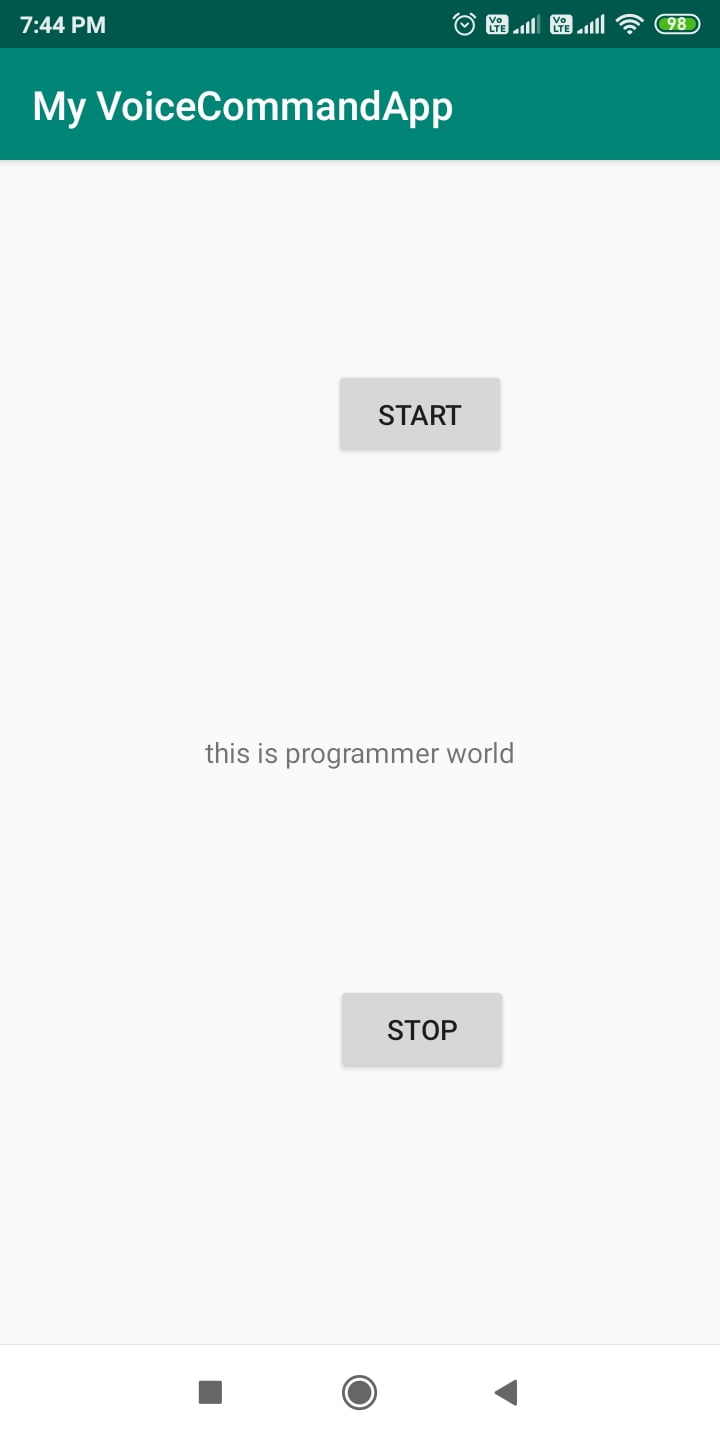
–
can you share this apk file?? Please
Sorry but the APK file is not available as of now. But the complete source code is shared in this webpage using which the APK can be created.
Cheers
Programmer World
–
Everything for free… love you.
welcome!
Cheers
Programmer World
–