In this video it shows the steps to implement smart search in the Android App. In this smart search it scans the text on the go (live) while the user it is typing. It highlights the matching part of the string by underlining it. It uses the spannable string for underlining the string.
It uses a sample text to demonstrate the searching algorithm – “simple text message to test smart live search implementation in android app”.
In this code it converts everything in lower case and hence, ignores the case sensitive search mechanism. For case sensitive search, one should avoid converting the texts in lower case.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.smartlivetextsearchapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Editable;
import android.text.Spannable;
import android.text.SpannableStringBuilder;
import android.text.TextWatcher;
import android.text.style.UnderlineSpan;
import android.widget.EditText;
import java.util.Locale;
public class MainActivity extends AppCompatActivity {
private EditText editTextKey, editTextToBeSearched;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editTextKey = findViewById(R.id.editTextKey);
editTextToBeSearched = findViewById(R.id.editTextToBeSearched);
String stringToBeSearched = editTextToBeSearched.getText().toString().toLowerCase(Locale.ROOT);
editTextKey.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence charSequence, int i, int i1, int i2) {
}
@Override
public void onTextChanged(CharSequence charSequence, int i, int i1, int i2) {
editTextToBeSearched.setText(stringToBeSearched);
String stringKey = editTextKey.getText().toString().toLowerCase(Locale.ROOT);
if (stringToBeSearched.contains(stringKey)){
int intStartIndex = stringToBeSearched.indexOf(stringKey);
int intEndIndex = intStartIndex + stringKey.length();
Spannable spannableString = new SpannableStringBuilder(stringToBeSearched);
spannableString.setSpan(new UnderlineSpan(),
intStartIndex,
intEndIndex,
0);
editTextToBeSearched.setText(spannableString);
}
}
@Override
public void afterTextChanged(Editable editable) {
}
});
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editTextKey"
android:layout_width="255dp"
android:layout_height="72dp"
android:layout_marginStart="76dp"
android:layout_marginTop="16dp"
android:ems="10"
android:hint="Search Key here ..."
android:inputType="textPersonName"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editTextToBeSearched"
android:layout_width="318dp"
android:layout_height="191dp"
android:layout_marginStart="44dp"
android:layout_marginTop="20dp"
android:ems="10"
android:gravity="start|top"
android:inputType="textMultiLine"
android:text="simple text message to test smart live search implementation in android app"
android:textSize="28sp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextKey" />
</androidx.constraintlayout.widget.ConstraintLayout>
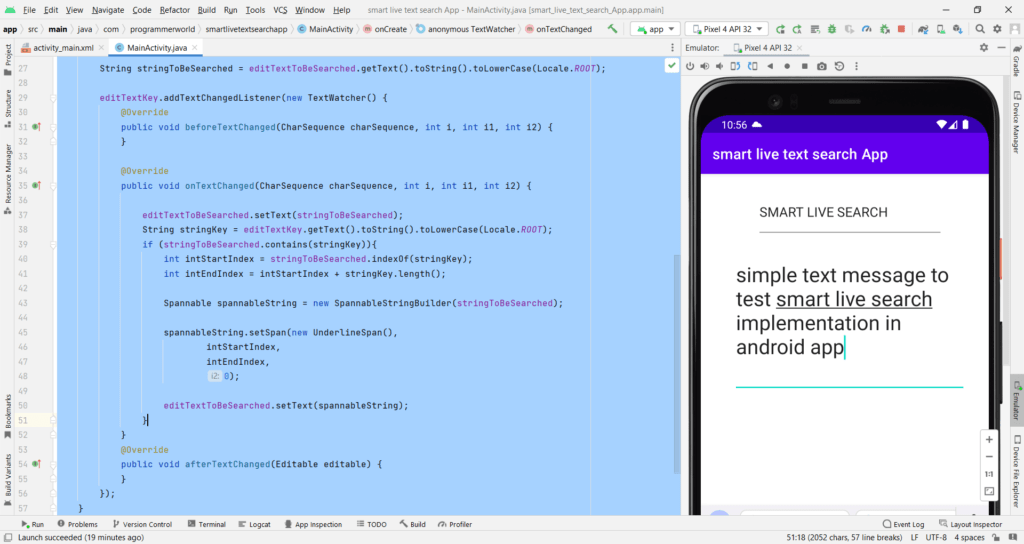
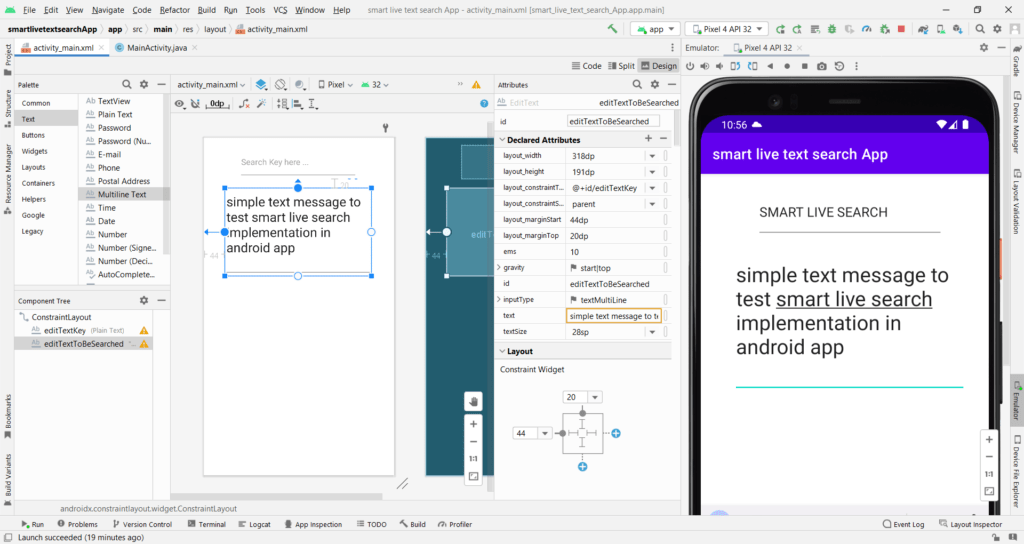