In this video it shows steps to create your own custom keyboard for Android device. The keyboard shown in this demo is system level keyboard and will work across any App of your Android device. It makes an entry at the system level and can be configured to be used in the settings of the Android device.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
No change required in MainActivity.java code
package com.programmerworld.customkeyboardsystemlevel;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
New Java class – CustomKeyboardApp
package com.programmerworld.customkeyboardsystemlevel;
import android.inputmethodservice.InputMethodService;
import android.inputmethodservice.Keyboard;
import android.inputmethodservice.KeyboardView;
import android.view.View;
import android.view.inputmethod.InputConnection;
public class CustomKeyboardApp extends InputMethodService
implements KeyboardView.OnKeyboardActionListener {
@Override
public View onCreateInputView() {
KeyboardView keyboardView = (KeyboardView) getLayoutInflater().inflate(R.layout.custom_keyboard_layout, null);
Keyboard keyboard = new Keyboard(this, R.xml.custom_keypad);
keyboardView.setKeyboard(keyboard);
keyboardView.setOnKeyboardActionListener(this);
return keyboardView;
}
@Override
public void onPress(int i) {
}
@Override
public void onRelease(int i) {
}
@Override
public void onKey(int i, int[] ints) {
InputConnection inputConnection = getCurrentInputConnection();
if (inputConnection == null){
return;
}
inputConnection.commitText(String.valueOf((char) i),1);
}
@Override
public void onText(CharSequence charSequence) {
}
@Override
public void swipeLeft() {
}
@Override
public void swipeRight() {
}
@Override
public void swipeDown() {
}
@Override
public void swipeUp() {
}
}
Layout Files:
<?xml version="1.0" encoding="utf-8"?>
<android.inputmethodservice.KeyboardView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:keyPreviewLayout="@layout/custom_keyboard_preview"
android:layout_alignParentBottom="true">
</android.inputmethodservice.KeyboardView>
<?xml version="1.0" encoding="utf-8"?>
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white"
android:gravity="center"
android:textColor="@color/black"
android:textSize="24sp">
</TextView>
XML Files:
<?xml version="1.0" encoding="utf-8"?>
<Keyboard xmlns:android="http://schemas.android.com/apk/res/android"
android:horizontalGap="5dp"
android:verticalGap="5dp"
android:keyHeight="60dp"
android:keyWidth="20%p">
<Row>
<Key android:keyLabel="1" android:keyEdgeFlags="left"/>
<Key android:keyLabel="2"/>
<Key android:keyLabel="3"/>
<Key android:keyLabel="4"/>
<Key android:keyLabel="5" android:keyEdgeFlags="right"/>
</Row>
<Row>
<Key android:keyLabel="6" android:keyEdgeFlags="left"/>
<Key android:keyLabel="7"/>
<Key android:keyLabel="8"/>
<Key android:keyLabel="9"/>
<Key android:keyLabel="0" android:keyEdgeFlags="right"/>
</Row>
<Row
android:keyWidth="33%p">
<Key android:keyLabel="A" android:keyEdgeFlags="left"/>
<Key android:keyLabel="B"/>
<Key android:keyLabel="C" android:keyEdgeFlags="right"/>
</Row>
</Keyboard>
<?xml version="1.0" encoding="utf-8"?>
<input-method xmlns:android="http://schemas.android.com/apk/res/android">
<subtype android:imeSubtypeMode = "keyboard"/>
</input-method>
Manifest file:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.CustomKeyBoardSystemLevel"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service android:name=".CustomKeyboardApp"
android:label="Programmer World Keyboard"
android:permission="android.permission.BIND_INPUT_METHOD"
android:exported="true">
<intent-filter>
<action android:name="android.view.InputMethod"/>
</intent-filter>
<meta-data
android:name="android.view.im"
android:resource="@xml/custom_method"/>
</service>
</application>
</manifest>
Screenshots:
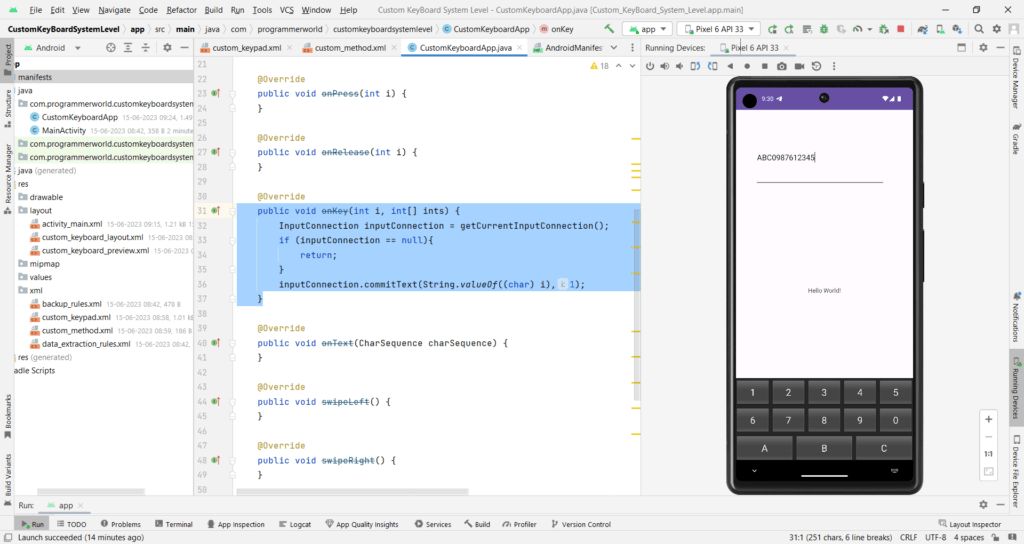
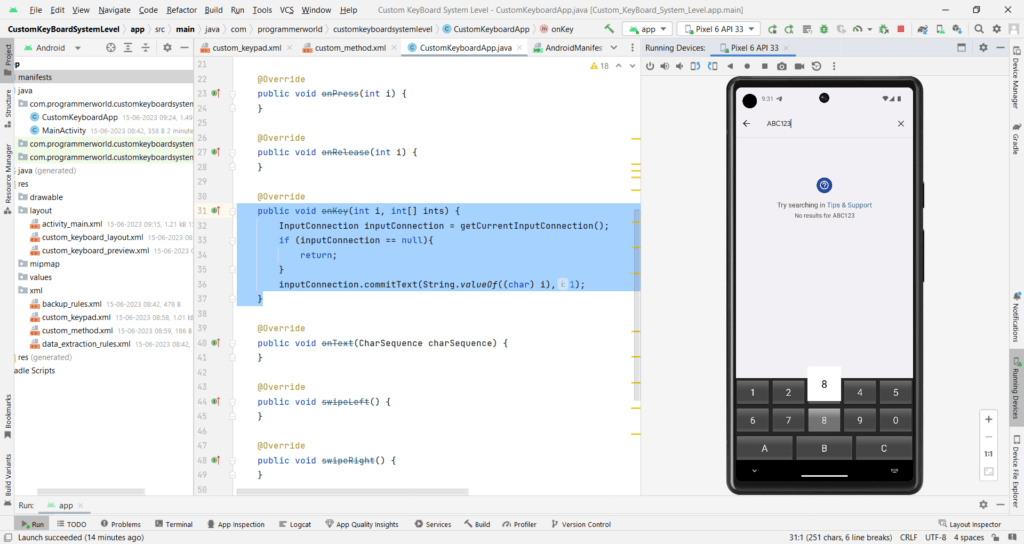