In this video it shows steps to modulate the sound of Text to Speech API by setting the pitch and speech rate using the seek bar in Android App.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.modulatespeechapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.speech.tts.TextToSpeech;
import android.widget.SeekBar;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView textView;
private SeekBar seekBarPitch, seekBarRate;
private TextToSpeech textToSpeech;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
seekBarPitch = findViewById(R.id.seekBarPitch);
seekBarRate = findViewById(R.id.seekBarRate);
textToSpeech = new TextToSpeech(getApplicationContext(), new TextToSpeech.OnInitListener() {
@Override
public void onInit(int i) {
}
});
seekBarRate.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int i, boolean b) {
textToSpeech.setSpeechRate((float) i/50);
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
if (textToSpeech.isSpeaking()){
textToSpeech.stop();
}
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
textToSpeech.speak(textView.getText().toString(),
TextToSpeech.QUEUE_FLUSH, null, null);
}
});
seekBarPitch.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int i, boolean b) {
textToSpeech.setPitch((float) i/50);
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
if (textToSpeech.isSpeaking()){
textToSpeech.stop();
}
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
textToSpeech.speak(textView.getText().toString(),
TextToSpeech.QUEUE_FLUSH, null, null);
}
});
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="266dp"
android:layout_height="223dp"
android:text="This is Progreammer World. This is an example of Speech modulation Android App. We will set different pitch and rate to modultate the sound of the text to speech API."
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<SeekBar
android:id="@+id/seekBarPitch"
android:layout_width="255dp"
android:layout_height="48dp"
android:layout_marginStart="73dp"
android:layout_marginTop="31dp"
android:progress="50"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<SeekBar
android:id="@+id/seekBarRate"
android:layout_width="234dp"
android:layout_height="53dp"
android:layout_marginStart="73dp"
android:layout_marginTop="43dp"
android:progress="50"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBarPitch" />
</androidx.constraintlayout.widget.ConstraintLayout>
Setting Pitch and speechRate for modulating the sound:
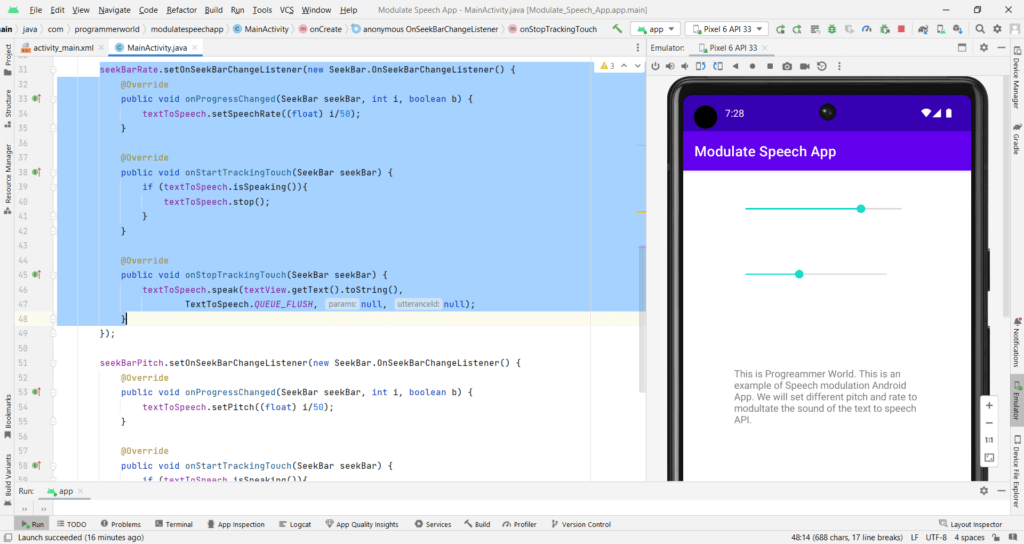
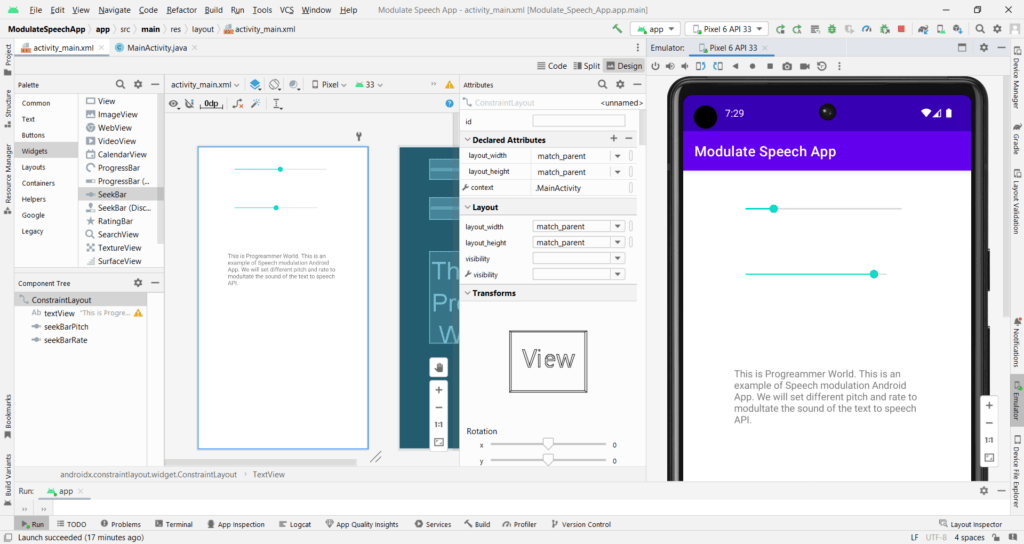