In this video, it shows how to open a jpeg file from download folder and convert it into Bitmap in your Android App. It then uses ImageView to display the image within the App layout.
It uses StorageManager to access the internal storage volume. And then access the jpeg file in the download folder. It uses BitmapFactory.decodeFile method to convert the file in bitmap within the App’s code. Finally, it uses ImageView to display it.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.jpegtobitmap;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.storage.StorageManager;
import android.os.storage.StorageVolume;
import android.view.View;
import android.widget.ImageView;
import java.io.File;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this, new String[]{
Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE},
PackageManager.PERMISSION_GRANTED);
imageView = findViewById(R.id.imageView);
}
public void buttonJPEGToBitmap(View view){
StorageManager storageManager = (StorageManager) getSystemService(STORAGE_SERVICE);
List<StorageVolume> storageVolumes = storageManager.getStorageVolumes();
StorageVolume storageVolume = storageVolumes.get(0); // 0 for internal storage
File fileImage = new File(storageVolume.getDirectory().getPath()+"/Download/images.jpeg");
Bitmap bitmap = BitmapFactory.decodeFile(fileImage.getPath());
imageView.setImageBitmap(bitmap);
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="124dp"
android:layout_marginTop="52dp"
android:onClick="buttonJPEGToBitmap"
android:text="JPEG To Bitmap"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="286dp"
android:layout_height="237dp"
android:layout_marginStart="62dp"
android:layout_marginTop="103dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
app:srcCompat="@drawable/ic_launcher_background" />
</androidx.constraintlayout.widget.ConstraintLayout>
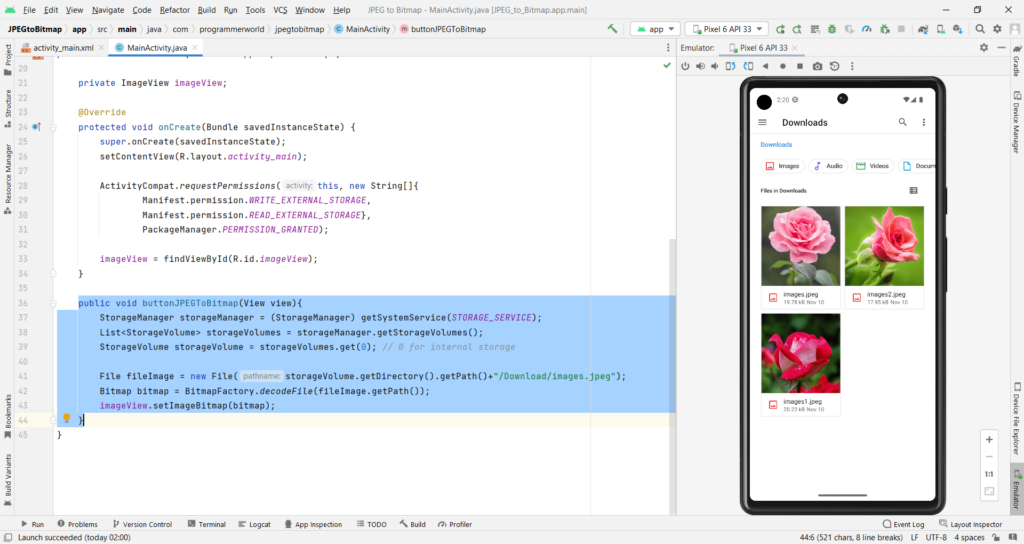
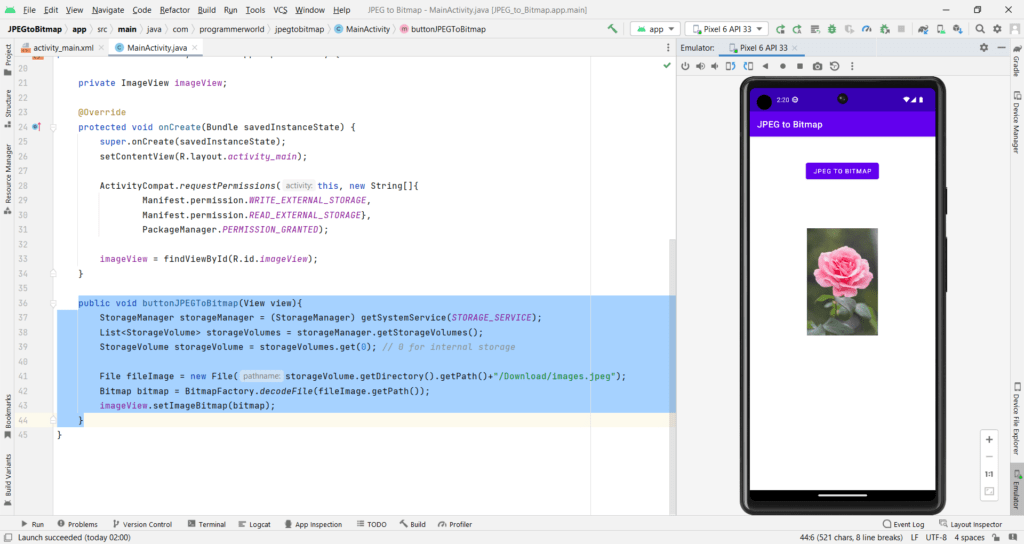
Images used in this demo:
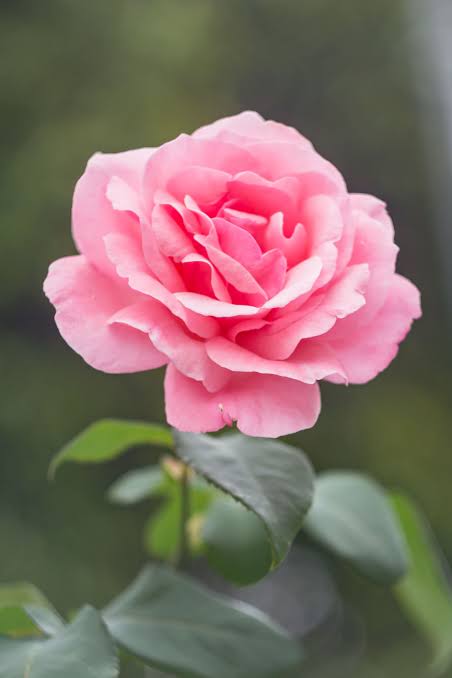
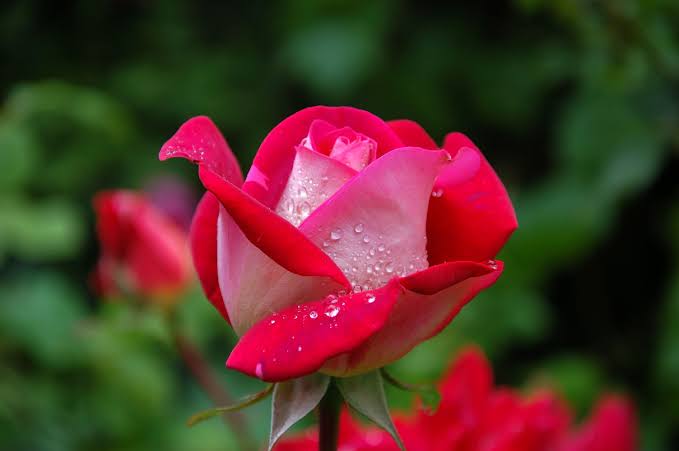
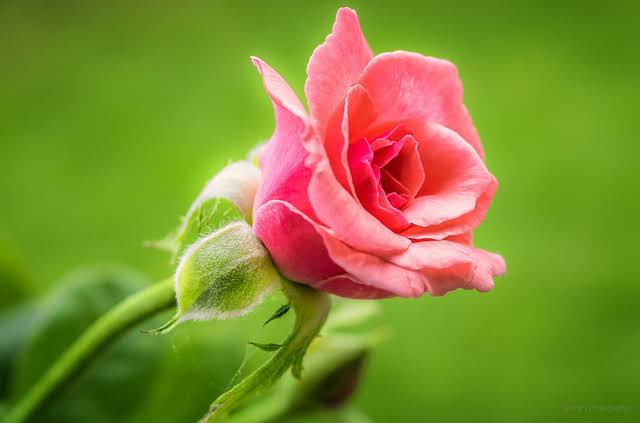