In this video it shows the code to call the Print API for printing the content of a TextView widget. Since the PrintDocumentAdapter is created on WebView, so, in this demonstration, the TextView has been first changed to WebView using the LoadData API of WebView and then the respective Print API is called.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.printtextview;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.print.PrintAttributes;
import android.print.PrintDocumentAdapter;
import android.print.PrintManager;
import android.view.View;
import android.webkit.WebView;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
}
public void buttonPintTextView(View view){
String stringTextView = textView.getText().toString();
WebView webView = new WebView(this);
webView.loadData(stringTextView, "text/html", "UTF-8");
PrintManager printManager = (PrintManager) getSystemService(PRINT_SERVICE);
PrintDocumentAdapter printDocumentAdapter = webView.createPrintDocumentAdapter("TextView_PrintDemo");
printManager.print("TextView Print Test", printDocumentAdapter, new PrintAttributes.Builder().build());
}
}
No changes in manifest file as shown below:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.PrintTextView"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="332dp"
android:layout_height="285dp"
android:text="Hello Programmer World, this is a sample TextView content."
android:textSize="34sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.493"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.369" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="112dp"
android:layout_marginTop="72dp"
android:onClick="buttonPintTextView"
android:text="Button Print TextView"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
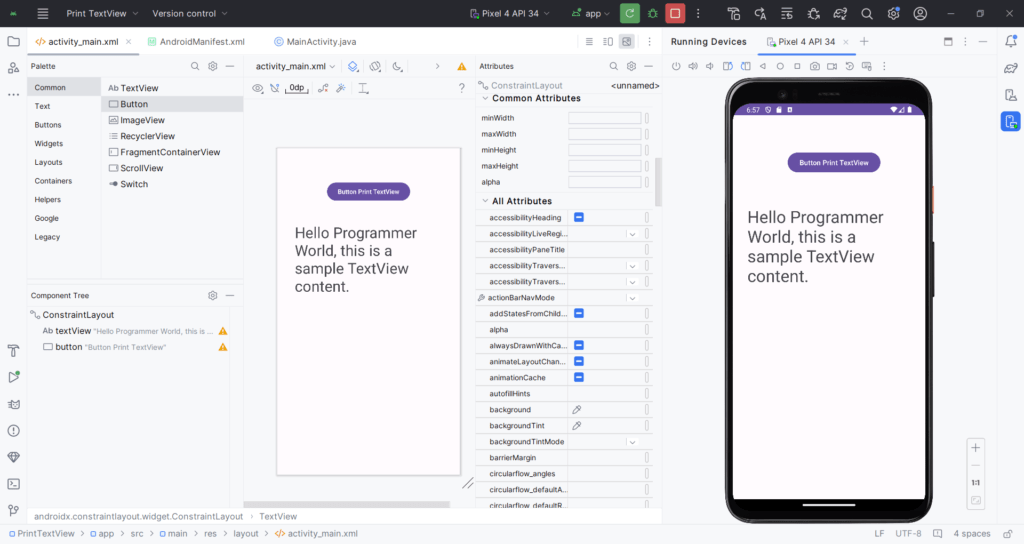
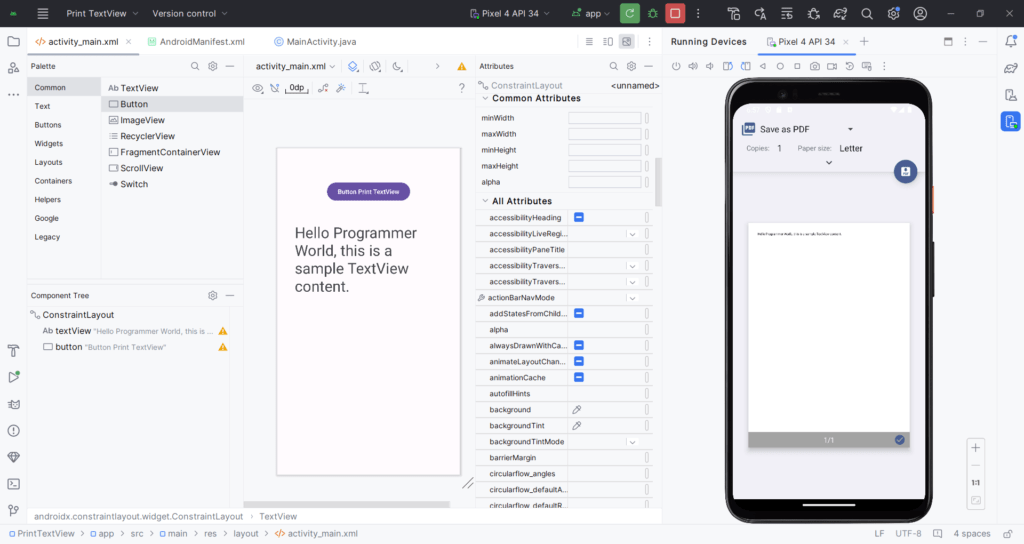
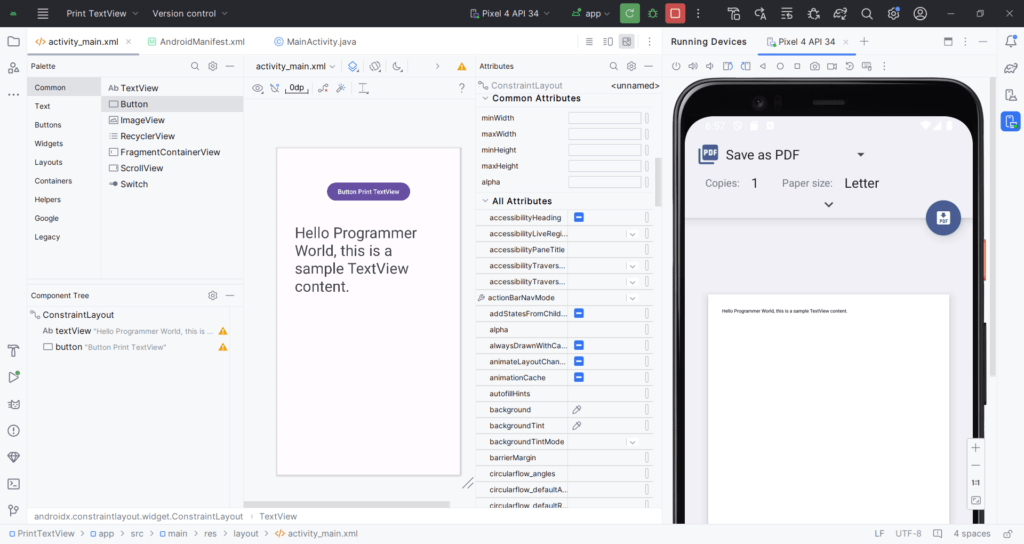
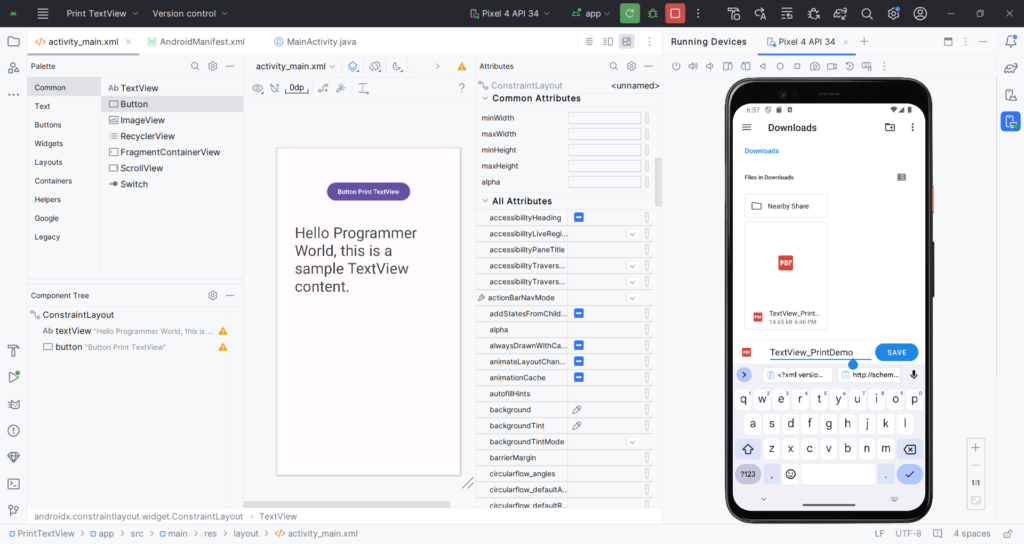
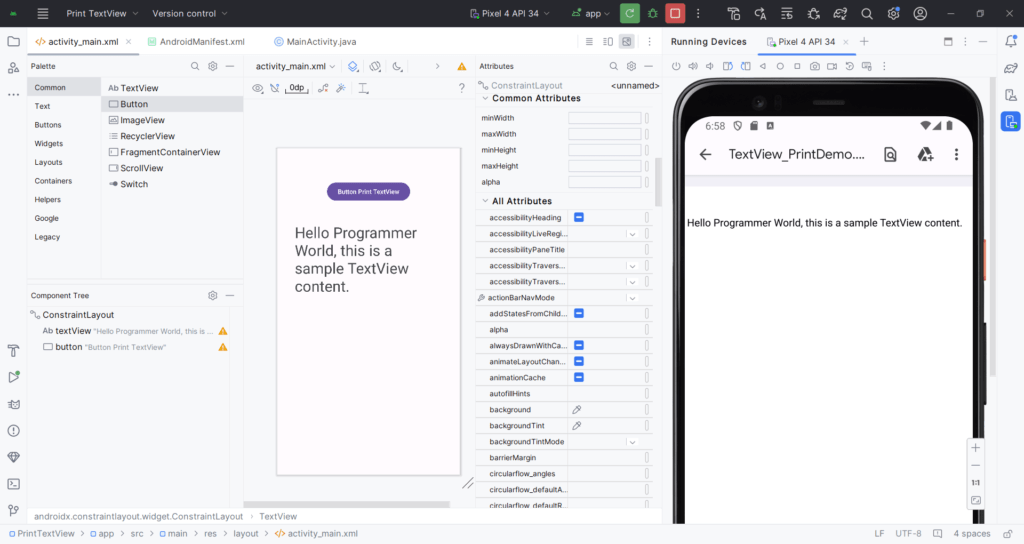
Text Used in this Demo:
“Hello Programmer World, this is a sample TextView content.”
Excerpt:
The video tutorial demonstrates how to use the Print API for printing content from a TextView widget in an Android application. In the tutorial, the TextView widget content is first converted into WebView format using the LoadData API. Then, the PrintDocumentAdapter, created on WebView, outputs the content via the Print API. The video provides step-by-step coding instructions along with a walkthrough of the Android application’s interface and functionalities. No changes are required in the manifest file for this process. The contact information for further queries or suggestions is also provided.