This video shows the steps to read the sensors data in your Android App. It uses the emulator and respective virtual sensors to demonstrate it for Android 13/ API 33 level.
In this video it shows the steps to read data from below type of sensors:
- TYPE_RELATIVE_HUMIDITY
- TYPE_LIGHT
- TYPE_MAGNETIC_FIELD_UNCALIBRATED
- TYPE_PRESSURE
- TYPE_AMBIENT_TEMPERATURE
- TYPE_PROXIMITY
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.readsensorsdataapp;
import androidx.appcompat.app.AppCompatActivity;
import android.hardware.Sensor;
import android.hardware.SensorEvent;
import android.hardware.SensorEventListener;
import android.hardware.SensorManager;
import android.os.Bundle;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView textViewMagnetic,textViewTemperature, textViewProximity,
textViewPressure, textViewLight, textViewHumidity;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textViewHumidity = findViewById(R.id.textViewHumidity);
textViewLight = findViewById(R.id.textViewLight);
textViewMagnetic = findViewById(R.id.textViewMagneticField);
textViewPressure = findViewById(R.id.textViewPressure);
textViewTemperature = findViewById(R.id.textViewTemperature);
textViewProximity = findViewById(R.id.textViewProximity);
SensorManager sensorManager = (SensorManager) getSystemService(SENSOR_SERVICE);
Sensor sensorHumidity = sensorManager.getDefaultSensor(Sensor.TYPE_RELATIVE_HUMIDITY);
Sensor sensorLight = sensorManager.getDefaultSensor(Sensor.TYPE_LIGHT);
Sensor sensorMagnetic = sensorManager.getDefaultSensor(Sensor.TYPE_MAGNETIC_FIELD_UNCALIBRATED);
Sensor sensorPressure = sensorManager.getDefaultSensor(Sensor.TYPE_PRESSURE);
Sensor sensorTemperature = sensorManager.getDefaultSensor(Sensor.TYPE_AMBIENT_TEMPERATURE);
Sensor sensorProximity = sensorManager.getDefaultSensor(Sensor.TYPE_PROXIMITY);
SensorEventListener sensorEventListenerHumidity = new SensorEventListener() {
@Override
public void onSensorChanged(SensorEvent sensorEvent) {
textViewHumidity.setText("Humidity = " + sensorEvent.values[0]);
}
@Override
public void onAccuracyChanged(Sensor sensor, int i) {
}
};
SensorEventListener sensorEventListenerLight = new SensorEventListener() {
@Override
public void onSensorChanged(SensorEvent sensorEvent) {
textViewLight.setText("Light = " + sensorEvent.values[0]);
}
@Override
public void onAccuracyChanged(Sensor sensor, int i) {
}
};
SensorEventListener sensorEventListenerPressure = new SensorEventListener() {
@Override
public void onSensorChanged(SensorEvent sensorEvent) {
textViewPressure.setText("Pressure = " + sensorEvent.values[0]);
}
@Override
public void onAccuracyChanged(Sensor sensor, int i) {
}
};
SensorEventListener sensorEventListenerMagnetic = new SensorEventListener() {
@Override
public void onSensorChanged(SensorEvent sensorEvent) {
textViewMagnetic.setText("Magnetic = " + sensorEvent.values[0]);
}
@Override
public void onAccuracyChanged(Sensor sensor, int i) {
}
};
SensorEventListener sensorEventListenerProximity = new SensorEventListener() {
@Override
public void onSensorChanged(SensorEvent sensorEvent) {
textViewProximity.setText("Proximity = " + sensorEvent.values[0]);
}
@Override
public void onAccuracyChanged(Sensor sensor, int i) {
}
};
SensorEventListener sensorEventListenerTemperature = new SensorEventListener() {
@Override
public void onSensorChanged(SensorEvent sensorEvent) {
textViewTemperature.setText("Ambient Temperature = " + sensorEvent.values[0]);
}
@Override
public void onAccuracyChanged(Sensor sensor, int i) {
}
};
sensorManager.registerListener(sensorEventListenerHumidity, sensorHumidity, SensorManager.SENSOR_DELAY_NORMAL);
sensorManager.registerListener(sensorEventListenerLight, sensorLight, SensorManager.SENSOR_DELAY_NORMAL);
sensorManager.registerListener(sensorEventListenerPressure, sensorPressure, SensorManager.SENSOR_DELAY_NORMAL);
sensorManager.registerListener(sensorEventListenerProximity, sensorProximity, SensorManager.SENSOR_DELAY_NORMAL);
sensorManager.registerListener(sensorEventListenerMagnetic, sensorMagnetic, SensorManager.SENSOR_DELAY_NORMAL);
sensorManager.registerListener(sensorEventListenerTemperature, sensorTemperature, SensorManager.SENSOR_DELAY_NORMAL);
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textViewTemperature"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Ambient Temperature"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.41"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.049" />
<TextView
android:id="@+id/textViewMagneticField"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="124dp"
android:layout_marginTop="28dp"
android:text="Magnetic Field"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textViewTemperature" />
<TextView
android:id="@+id/textViewProximity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="124dp"
android:layout_marginTop="36dp"
android:text="Proximity"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textViewMagneticField" />
<TextView
android:id="@+id/textViewPressure"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="112dp"
android:layout_marginTop="48dp"
android:text="Pressure"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textViewProximity" />
<TextView
android:id="@+id/textViewLight"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="112dp"
android:layout_marginTop="36dp"
android:text="Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textViewPressure" />
<TextView
android:id="@+id/textViewHumidity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="112dp"
android:layout_marginTop="40dp"
android:text="Humidity"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textViewLight" />
</androidx.constraintlayout.widget.ConstraintLayout>
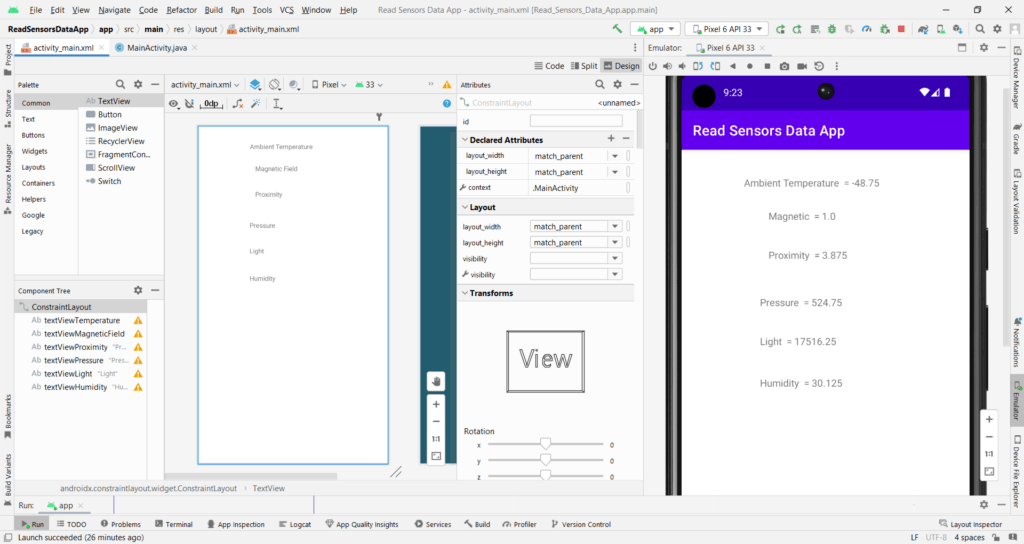
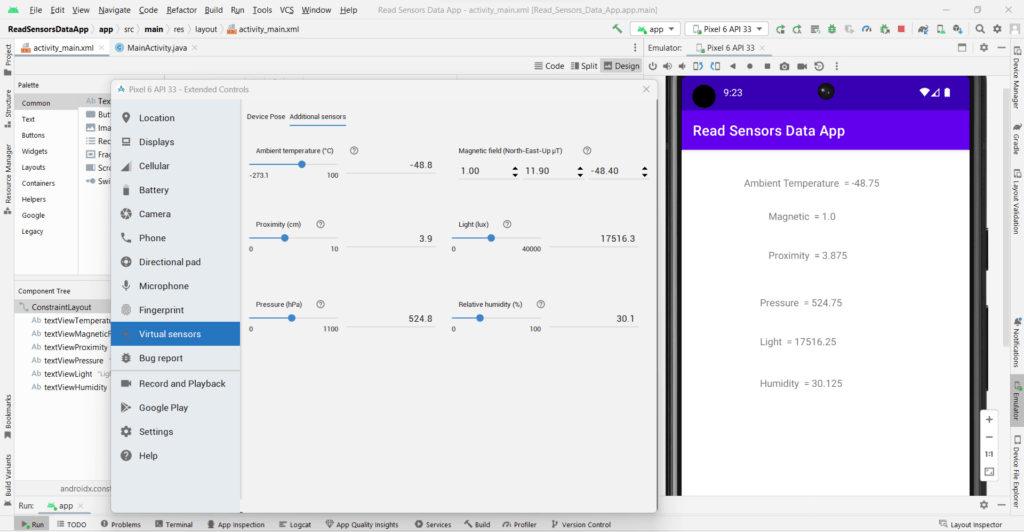