In this video it shows how one can use createScaledBitmap method of Bitmap to resize or set the size of any image in the Bitmap.
In this demo it uses ImageView widget to display the raw (unscaled) and resized images.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.bitmapimagesize;
import static android.Manifest.permission.READ_MEDIA_IMAGES;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.storage.StorageManager;
import android.os.storage.StorageVolume;
import android.view.View;
import android.widget.ImageView;
import java.io.File;
public class MainActivity extends AppCompatActivity {
private ImageView imageView, imageView1;
private Bitmap bitmap, bitmap1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{READ_MEDIA_IMAGES},
PackageManager.PERMISSION_GRANTED);
imageView = findViewById(R.id.imageView);
imageView1 = findViewById(R.id.imageView2);
StorageManager storageManager = (StorageManager) getSystemService(STORAGE_SERVICE);
StorageVolume storageVolume = storageManager.getStorageVolumes().get(0); //0=internal storage
File fileImage = new File(storageVolume.getDirectory().getPath()+"/Download/images.jpeg");
File fileImage1 = new File(storageVolume.getDirectory().getPath()+"/Download/images1.jpeg");
bitmap = BitmapFactory.decodeFile(fileImage.getPath());
bitmap1 = BitmapFactory.decodeFile(fileImage1.getPath());
}
public void buttonRawImage(View view){
imageView.setImageBitmap(bitmap);
imageView1.setImageBitmap(bitmap1);
}
public void buttonSizedImage(View view){
Bitmap bitmap_resize = Bitmap.createScaledBitmap(bitmap, bitmap1.getWidth(), bitmap1.getHeight(), false);
Bitmap bitmap1_resize = Bitmap.createScaledBitmap(bitmap1, bitmap1.getWidth(), bitmap1.getHeight(), false);
imageView.setImageBitmap(bitmap_resize);
imageView1.setImageBitmap(bitmap1_resize);
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.READ_MEDIA_IMAGES"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/Theme.BitmapImageSize"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="80dp"
android:onClick="buttonRawImage"
android:text="Show Raw Image"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="141dp"
android:layout_marginTop="74dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
app:srcCompat="@drawable/ic_launcher_background" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="141dp"
android:layout_marginTop="81dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView"
app:srcCompat="@drawable/ic_launcher_background" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="27dp"
android:layout_marginTop="84dp"
android:onClick="buttonSizedImage"
android:text="Show sized Image"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Resized images:
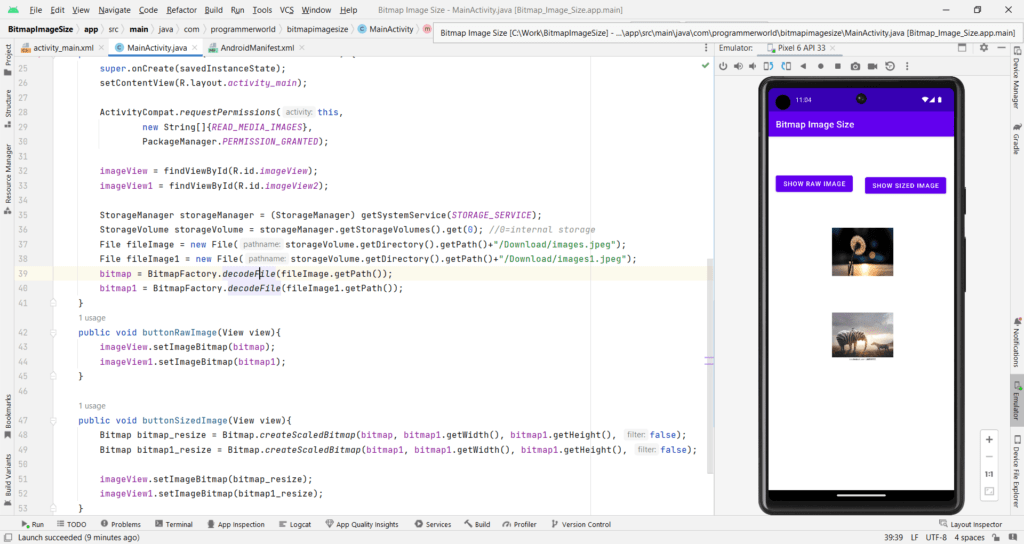
Raw Images:
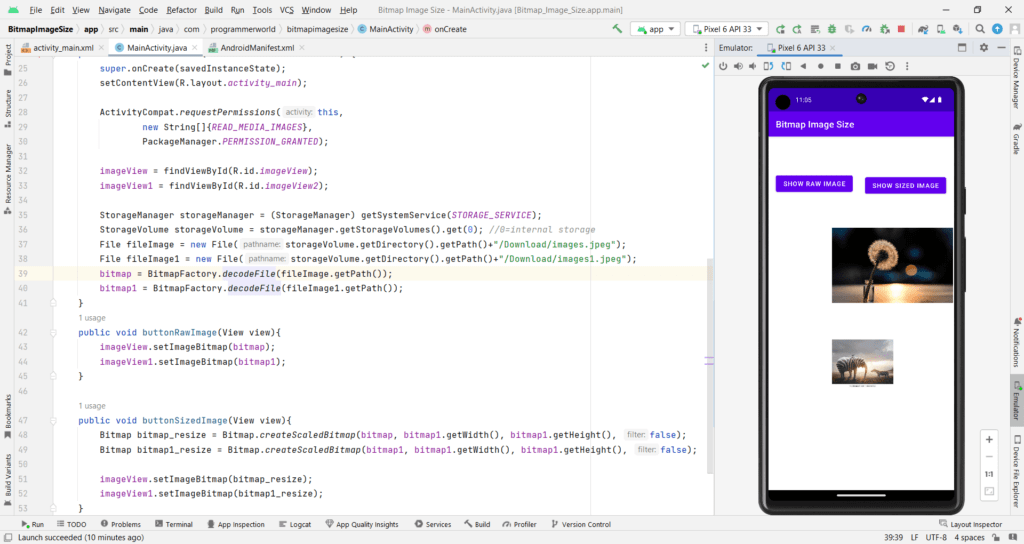