In this video it shows the steps to run a python code in Android App’s code. It calls the python script from the Java code. It uses chaquopy plugin, which is Python SDK for Android. Details are available at: https://chaquo.com/chaquopy/
The steps for setting the Gradle is taken from this page: https://chaquo.com/chaquopy/doc/current/android.html
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
Android Java code:
package com.programmerworld.pythininandroidapp;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
import com.chaquo.python.PyObject;
import com.chaquo.python.Python;
import com.chaquo.python.android.AndroidPlatform;
public class MainActivity extends AppCompatActivity {
private TextView textViewOutput;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
textViewOutput = findViewById(R.id.textViewOutput);
Python.start(new AndroidPlatform(getApplicationContext()));
return insets;
});
}
public void buttonPythonRun(View view){
Python python = Python.getInstance();
PyObject pyObjectResult = python.getModule("add_numbers")
.callAttr("add_numbers", 10, 25);
textViewOutput.setText(pyObjectResult.toString());
}
}
Gradle Files:
Project Level:
// Top-level build file where you can add configuration options common to all sub-projects/modules.
plugins {
alias(libs.plugins.android.application) apply false
id("com.chaquo.python") version "15.0.1" apply false
}
App Level:
plugins {
alias(libs.plugins.android.application)
id("com.chaquo.python")
}
android {
namespace = "com.programmerworld.pythininandroidapp"
compileSdk = 34
defaultConfig {
applicationId = "com.programmerworld.pythininandroidapp"
minSdk = 33
targetSdk = 34
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
ndk {
// On Apple silicon, you can omit x86_64.
abiFilters += listOf("arm64-v8a", "x86_64")
}
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
flavorDimensions += "pyVersion"
productFlavors {
create("py310") { dimension = "pyVersion" }
create("py311") { dimension = "pyVersion" }
}
}
chaquopy {
productFlavors {
getByName("py310") { version = "3.10" }
getByName("py311") { version = "3.11" }
}
}
dependencies {
implementation(libs.appcompat)
implementation(libs.material)
implementation(libs.activity)
implementation(libs.constraintlayout)
testImplementation(libs.junit)
androidTestImplementation(libs.ext.junit)
androidTestImplementation(libs.espresso.core)
}
Python Sample code:
def add_numbers(x, y):
return x + y
Layout/ XML file:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textViewOutput"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textSize="34sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.291" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="80dp"
android:onClick="buttonPythonRun"
android:text="Python Execution"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshot:
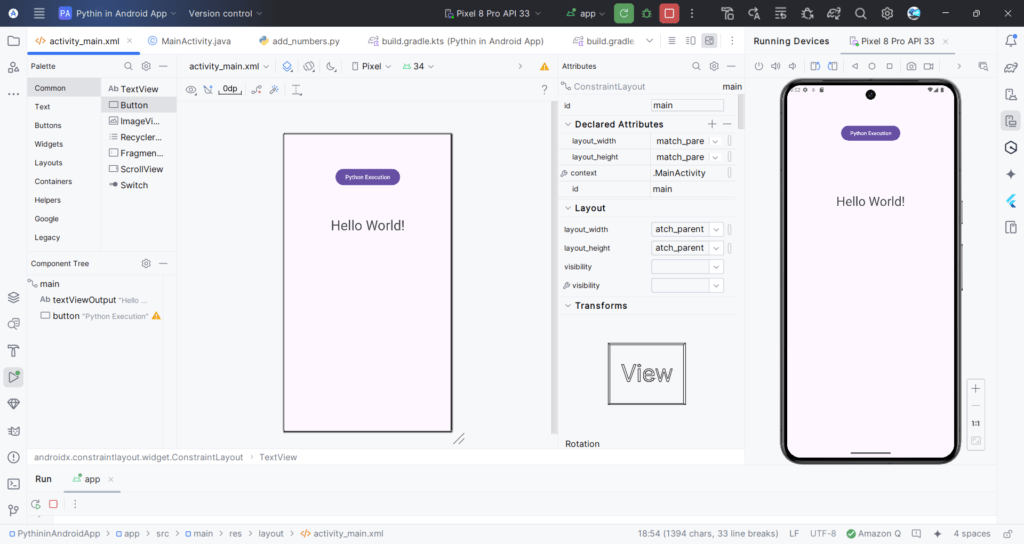
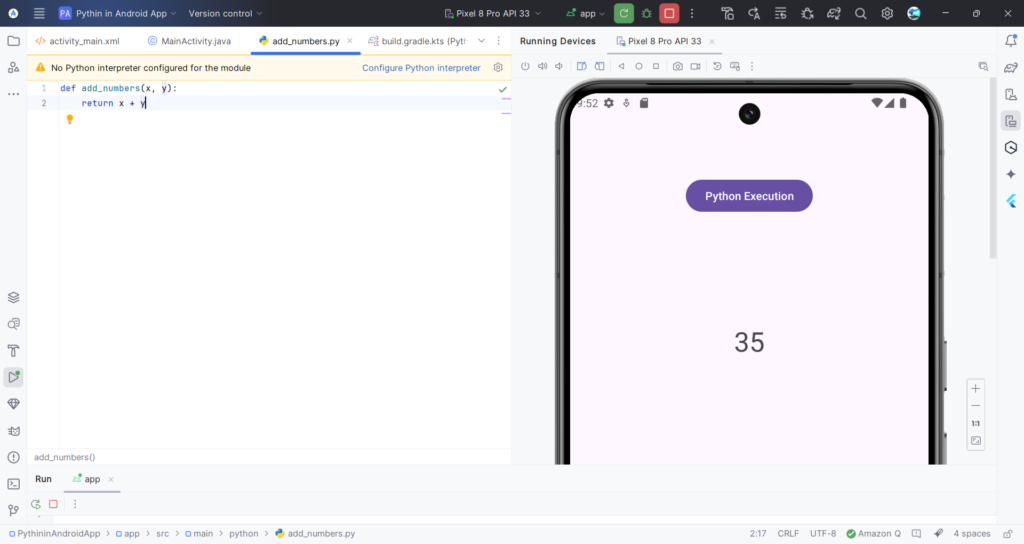
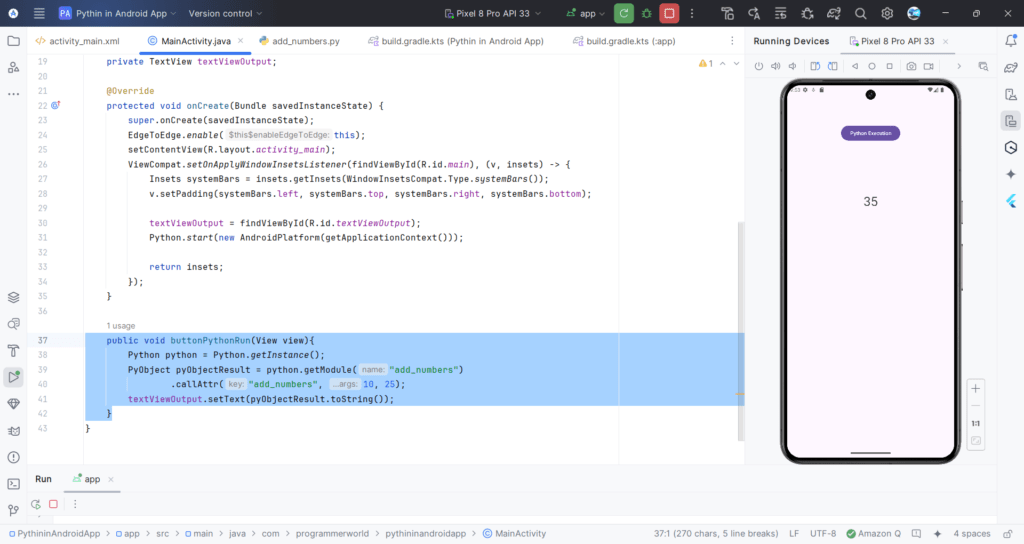
Complete project folder can be accessed at the following link on payment of USD 9:
https://drive.google.com/file/d/1YN6QmeVcl9XLlj-JXo0_rkfYjWf5Z01i/view?usp=drive_link
Learn how to run Python script in Android App. It shows the steps to integrate the Python code in the Android Java code in Android Studio IDE.
Thank You!!!