This video shows the steps to share an image over other applications such as Telegram, WhatsApp, google photos, etc. directly from your Android App.
In this video it shares the image in bitmap form (as an image) and not as a file.
To share the complete files one can refer to the details shown in the below link:
https://programmerworld.co/android/how-to-share-file-or-text-on-gmail-whatsapp-sms-bluetooth-from-your-android-app-share-button/
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.sharebitmapimage_telegramwhatsapp;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.net.Uri;
import android.os.Bundle;
import android.os.storage.StorageManager;
import android.os.storage.StorageVolume;
import android.provider.MediaStore;
import android.view.View;
import android.widget.ImageView;
import java.io.File;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.READ_MEDIA_IMAGES},
PackageManager.PERMISSION_GRANTED);
imageView = findViewById(R.id.imageView);
}
public void buttonShareBitmap(View view){
StorageManager storageManager = (StorageManager) getSystemService(STORAGE_SERVICE);
StorageVolume storageVolume = storageManager.getStorageVolumes().get(0); // internal memory
File fileImage = new File(storageVolume.getDirectory().getPath() + "/Download/images.jpeg");
Bitmap bitmap = BitmapFactory.decodeFile(fileImage.getPath());
imageView.setImageBitmap(bitmap);
String stringPath = MediaStore.Images.Media.insertImage(this.getContentResolver(),
bitmap,"Shared Image", null);
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("image/*");
intent.putExtra(Intent.EXTRA_STREAM, Uri.parse(stringPath));
startActivity(Intent.createChooser(intent, "Share the Image ... "));
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.READ_MEDIA_IMAGES"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/Theme.ShareBitmapImageTelegramWhatsapp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data
android:name="android.app.lib_name"
android:value="" />
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="126dp"
android:layout_marginTop="110dp"
android:onClick="buttonShareBitmap"
android:text="Share Bitmap"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="316dp"
android:layout_height="268dp"
android:layout_marginStart="44dp"
android:layout_marginTop="52dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
app:srcCompat="@drawable/ic_launcher_background" />
</androidx.constraintlayout.widget.ConstraintLayout>
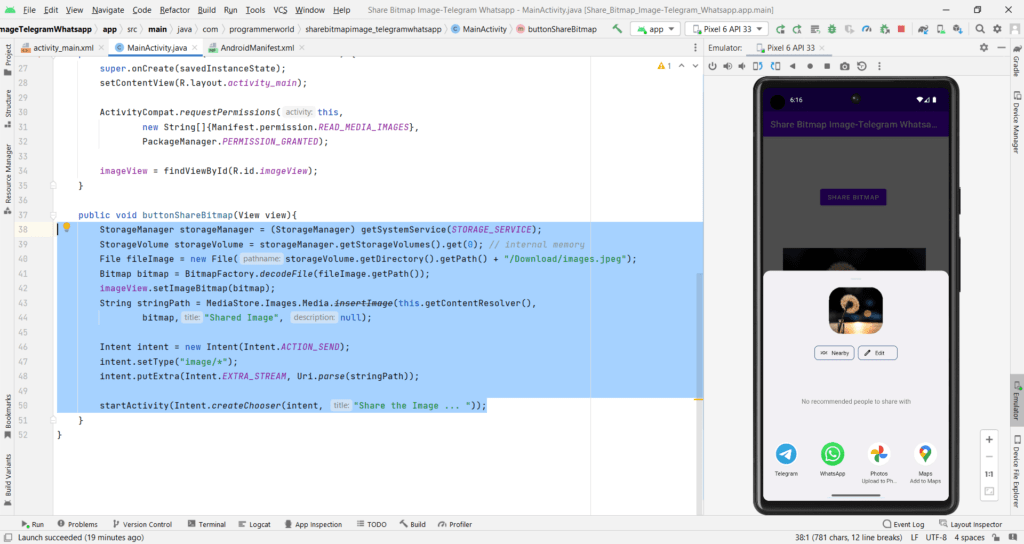
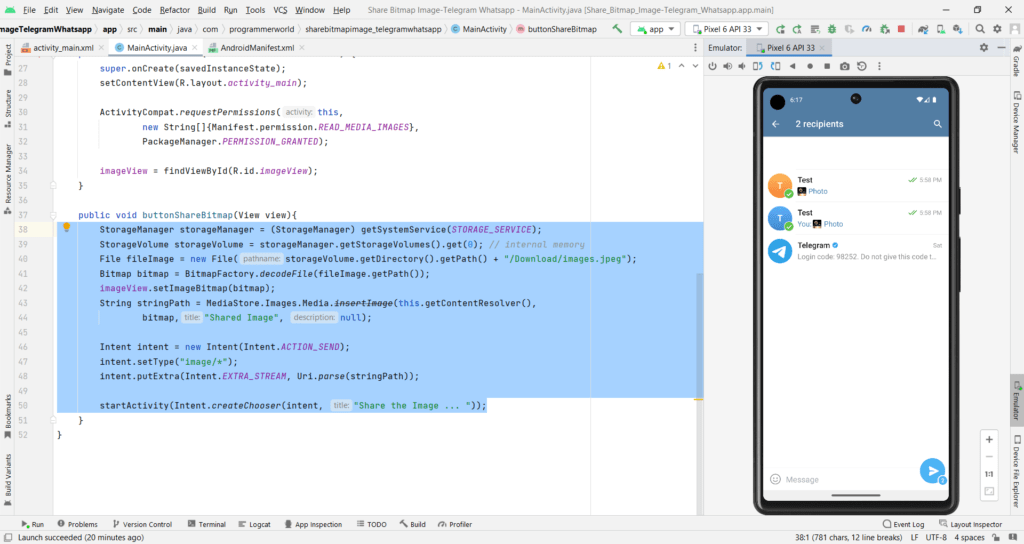
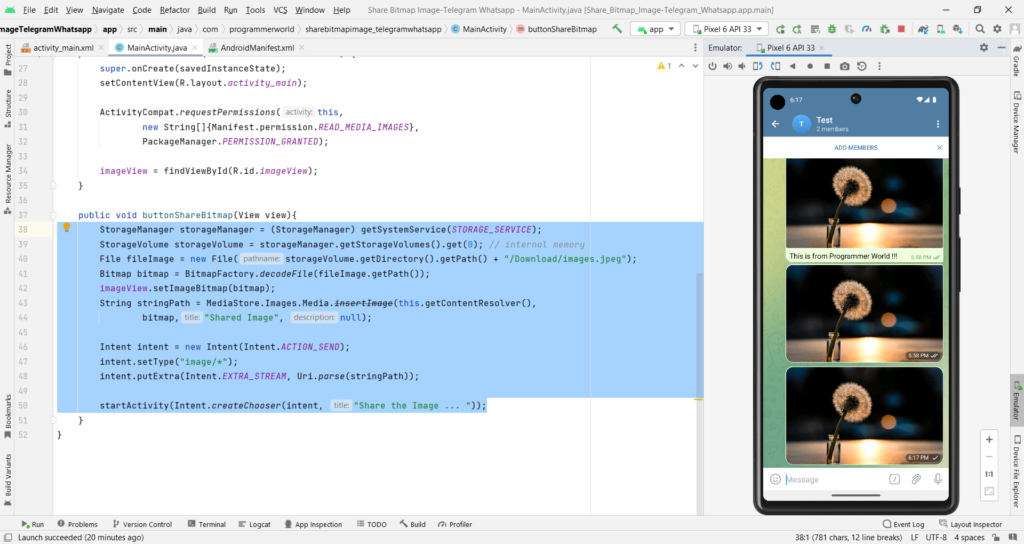
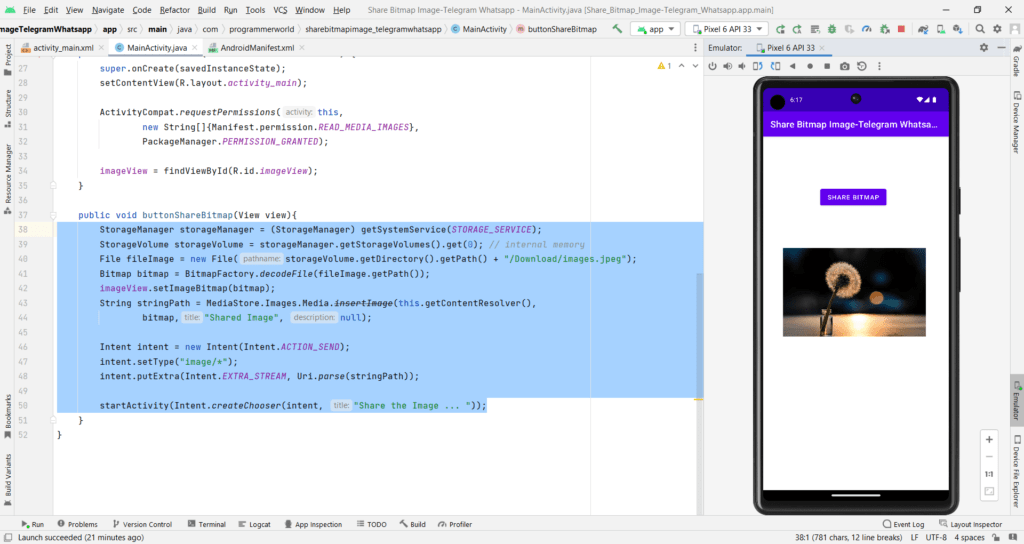