In this video it shows how to place and show the images in recycler view in your Android App.
It creates the RecyclerViewCustomAdapter class for showing the images by setting the respective bitmaps of the images in the imageview.
It uses a Frame layout containing ImageView used for the RecyclerView layout.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.imageinrecyclerviewapp;
import static android.Manifest.permission.READ_MEDIA_IMAGES;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import androidx.recyclerview.widget.GridLayoutManager;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.storage.StorageManager;
import android.os.storage.StorageVolume;
import android.view.View;
import java.io.File;
public class MainActivity extends AppCompatActivity {
private RecyclerView recyclerView;
private RecyclerView.LayoutManager layoutManagerRecyclerView;
private RecyclerViewCustomAdapter recyclerViewCustomAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActivityCompat.requestPermissions(this,
new String[]{READ_MEDIA_IMAGES},
PackageManager.PERMISSION_GRANTED);
recyclerView = findViewById(R.id.recyclerView);
// Liner Layout
layoutManagerRecyclerView = new LinearLayoutManager(MainActivity.this);
// GRID Layout
//layoutManagerRecyclerView = new GridLayoutManager(MainActivity.this, 2);
recyclerView.setLayoutManager(layoutManagerRecyclerView);
}
public void buttonRecyclerViewUpdate(View view){
StorageManager storageManager = (StorageManager) getSystemService(STORAGE_SERVICE);
StorageVolume storageVolume = storageManager.getStorageVolumes().get(0); // internal memory/ storage
File fileImage = new File(storageVolume.getDirectory().getPath() + "/Download/images.jpeg");
File fileImage1 = new File(storageVolume.getDirectory().getPath() + "/Download/images1.jpeg");
Bitmap bitmap = BitmapFactory.decodeFile(fileImage.getPath());
Bitmap bitmap1 = BitmapFactory.decodeFile(fileImage1.getPath());
Bitmap[] bitmaps = {bitmap, bitmap1};
recyclerViewCustomAdapter = new RecyclerViewCustomAdapter(bitmaps);
recyclerView.setAdapter(recyclerViewCustomAdapter);
}
}
package com.programmerworld.imageinrecyclerviewapp;
import android.graphics.Bitmap;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
public class RecyclerViewCustomAdapter extends RecyclerView.Adapter<RecyclerViewCustomAdapter.ViewHolder> {
private Bitmap[] bitmapsLocal;
public RecyclerViewCustomAdapter(Bitmap[] bitmaps) {
bitmapsLocal = bitmaps;
}
public static class ViewHolder extends RecyclerView.ViewHolder{
private final ImageView imageView;
public ViewHolder(@NonNull View itemView) {
super(itemView);
imageView = itemView.findViewById(R.id.imageView);
}
public ImageView getImageView(){
return imageView;
}
}
@NonNull
@Override
public RecyclerViewCustomAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.image_row_items,
parent,
false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull RecyclerViewCustomAdapter.ViewHolder holder, int position) {
holder.getImageView().setImageBitmap(bitmapsLocal[position]);
}
@Override
public int getItemCount() {
return bitmapsLocal.length;
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="96dp"
android:layout_marginTop="28dp"
android:onClick="buttonRecyclerViewUpdate"
android:text="Recycler View Update"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="264dp"
android:layout_height="283dp"
android:layout_marginStart="73dp"
android:layout_marginTop="56dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="@drawable/ic_launcher_background" />
</FrameLayout>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.READ_MEDIA_IMAGES"/>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/Theme.ImageInRecyclerViewApp"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Liner Layout:
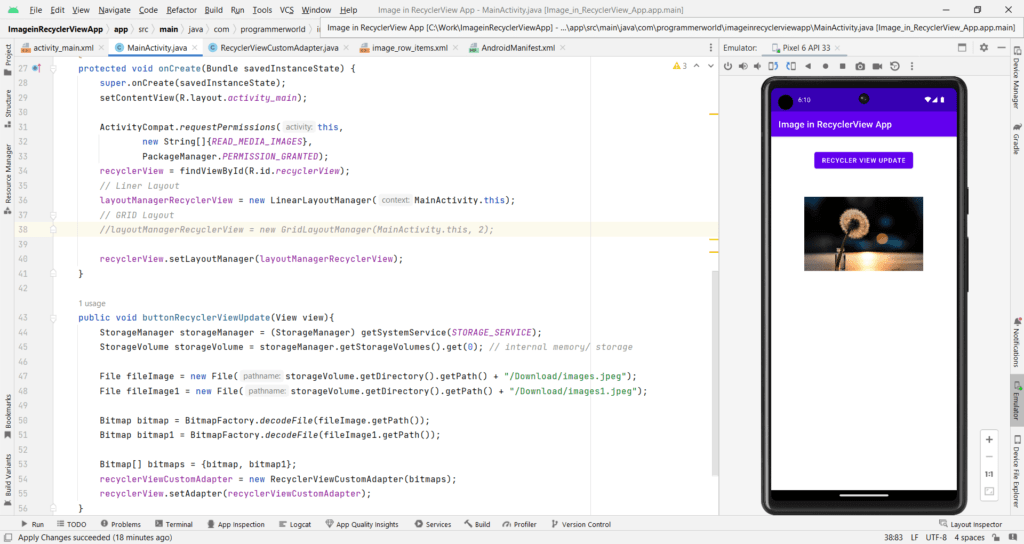
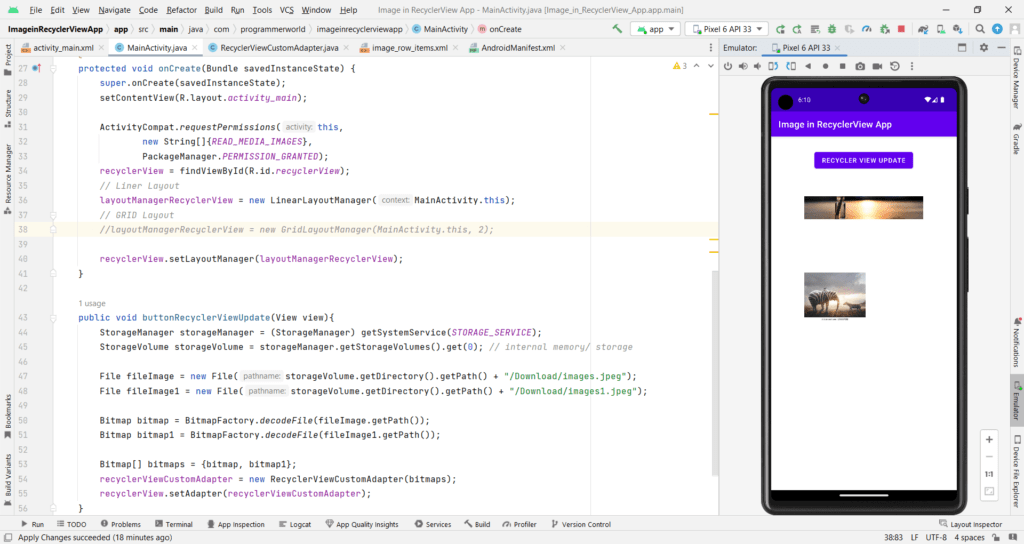
Grid Layout below:
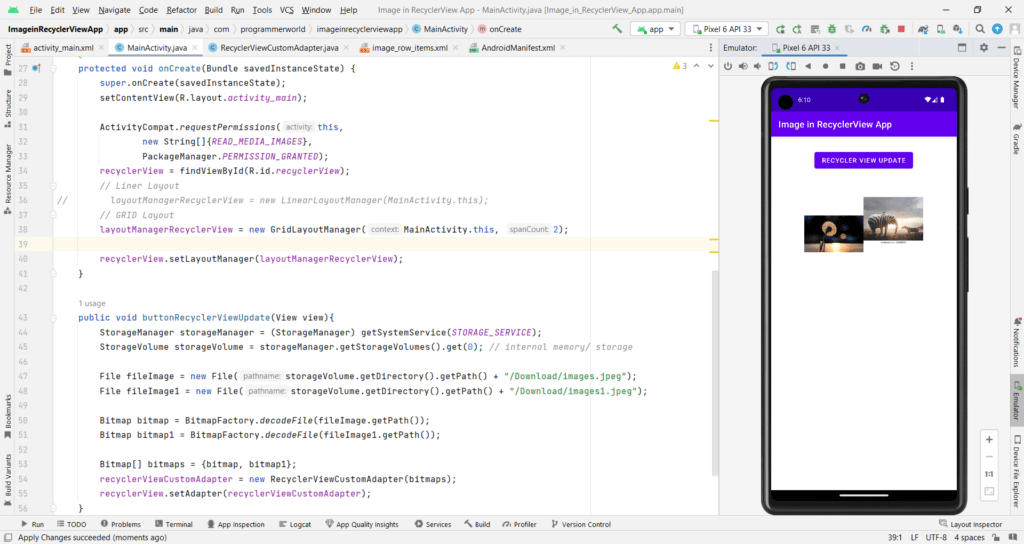