In this video it shows the steps to use Gemini AI tool to generate Android App code and write the respective Java unit Test cases.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details:
package com.programmerworld.gemini_appandunittestcodegeneration;
import android.os.Bundle;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
return insets;
});
TextView textView = findViewById(R.id.textView);
textView.setText(String.valueOf(AddCustomMethod(1, 2)));
}
public int AddCustomMethod(int a, int b) {
return a + b;
}
}
package com.programmerworld.gemini_appandunittestcodegeneration;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.robolectric.Robolectric;
import org.robolectric.RobolectricTestRunner;
import static org.junit.Assert.*;
/**
* Example local unit test, which will execute on the development machine (host).
*
* @see <a href="http://d.android.com/tools/testing">Testing documentation</a>
*/
@RunWith(RobolectricTestRunner.class)
public class ExampleUnitTest {
private MainActivity mainActivity;
@Before
public void setUp() {
mainActivity = Robolectric.buildActivity(MainActivity.class).create().get();
}
@Test
public void addition_isCorrect() {
assertEquals(4, 2 + 2);
}
@Test
public void add() {
// Test positive numbers
assertEquals(8, mainActivity.AddCustomMethod(5, 3));
assertEquals(10, mainActivity.AddCustomMethod(7, 3));
// Test negative numbers
assertEquals(-2, mainActivity.AddCustomMethod(-5, 3));
assertEquals(-10, mainActivity.AddCustomMethod(-7, -3));
// Test zero
assertEquals(0,mainActivity.AddCustomMethod(0, 0));
assertEquals(5, mainActivity.AddCustomMethod(5, 0));
assertEquals(-5, mainActivity.AddCustomMethod(0, -5));
}
}
plugins {
alias(libs.plugins.android.application)
}
android {
namespace = "com.programmerworld.gemini_appandunittestcodegeneration"
compileSdk = 34
defaultConfig {
applicationId = "com.programmerworld.gemini_appandunittestcodegeneration"
minSdk = 33
targetSdk = 34
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
testOptions {
unitTests.isIncludeAndroidResources = true
}
}
dependencies {
implementation(libs.appcompat)
implementation(libs.material)
implementation(libs.activity)
implementation(libs.constraintlayout)
testImplementation(libs.junit)
androidTestImplementation(libs.ext.junit)
androidTestImplementation(libs.espresso.core)
testImplementation(libs.robolectric)
}
[versions]
agp = "8.4.0"
junit = "4.13.2"
junitVersion = "1.1.5"
espressoCore = "3.5.1"
appcompat = "1.6.1"
material = "1.12.0"
activity = "1.9.0"
constraintlayout = "2.1.4"
robolectric = "4.12.1"
[libraries]
junit = { group = "junit", name = "junit", version.ref = "junit" }
ext-junit = { group = "androidx.test.ext", name = "junit", version.ref = "junitVersion" }
espresso-core = { group = "androidx.test.espresso", name = "espresso-core", version.ref = "espressoCore" }
appcompat = { group = "androidx.appcompat", name = "appcompat", version.ref = "appcompat" }
material = { group = "com.google.android.material", name = "material", version.ref = "material" }
activity = { group = "androidx.activity", name = "activity", version.ref = "activity" }
constraintlayout = { group = "androidx.constraintlayout", name = "constraintlayout", version.ref = "constraintlayout" }
robolectric = { module = "org.robolectric:robolectric", version.ref = "robolectric" }
[plugins]
android-application = { id = "com.android.application", version.ref = "agp" }
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textSize="34sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
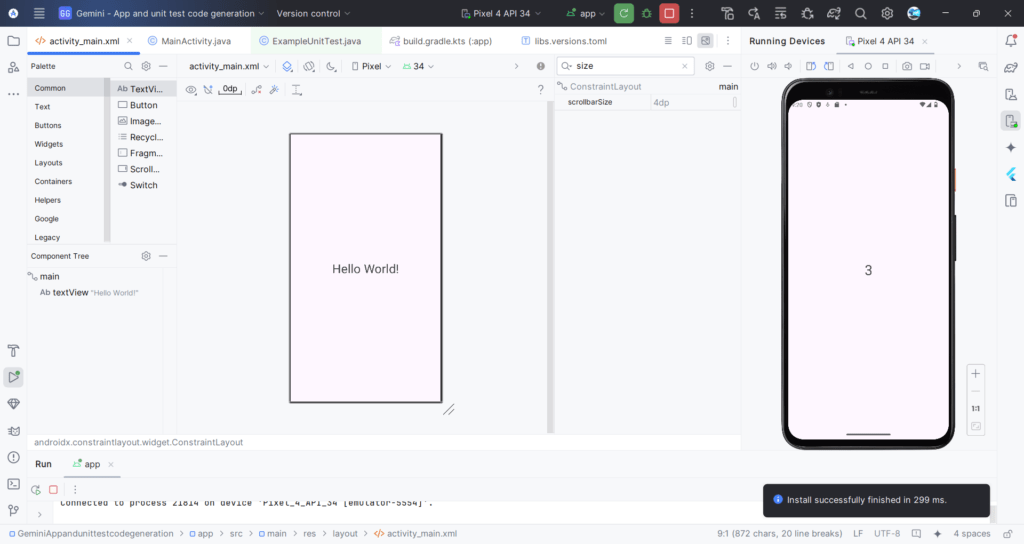
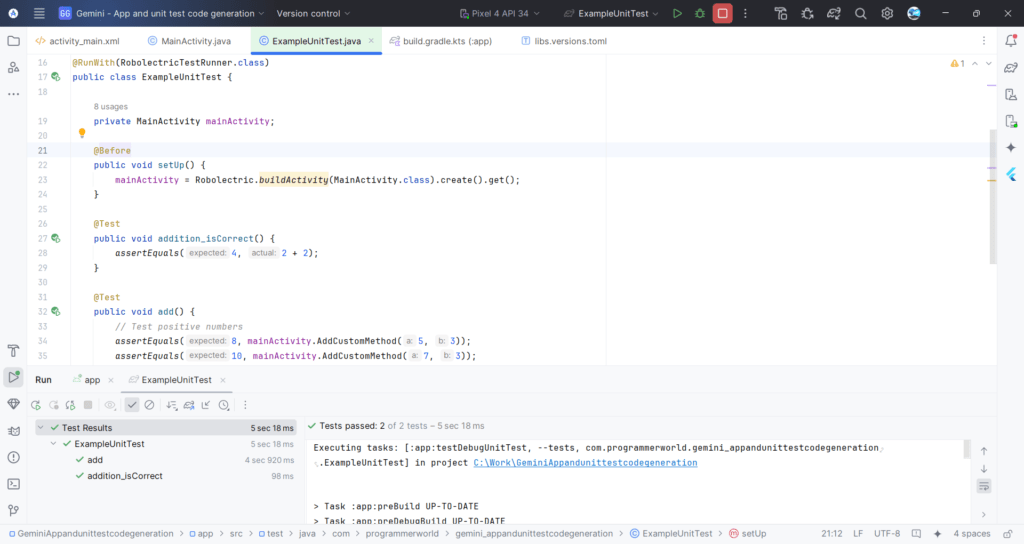
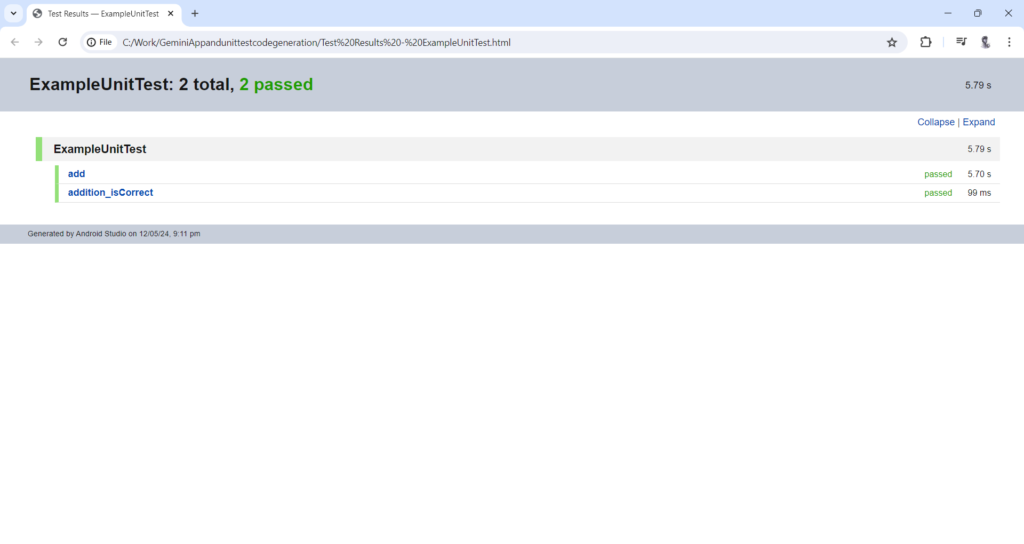
Test Report file:
Gemini:
Prompts used:
- Write a simple addition method for Android java code?
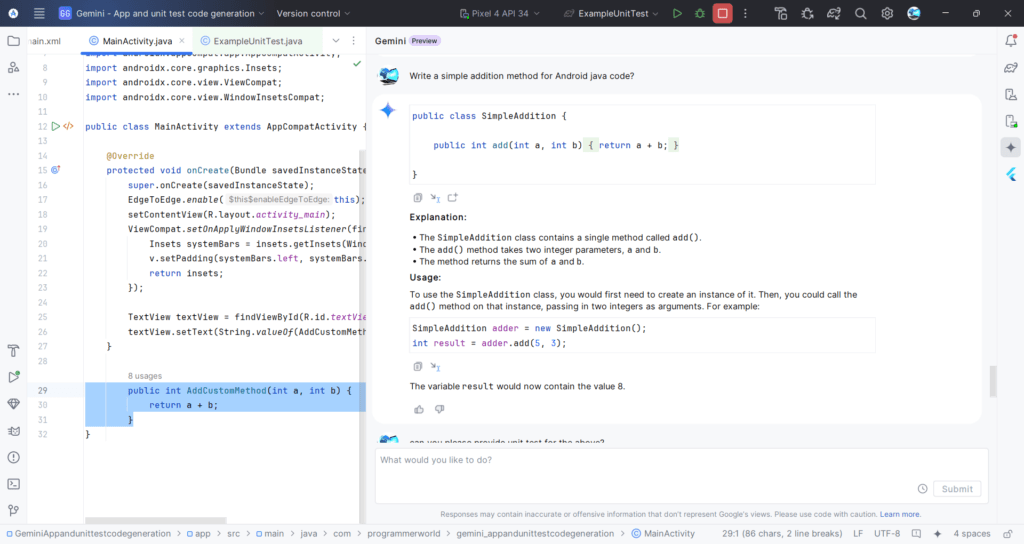
2. can you please provide unit test for the above?
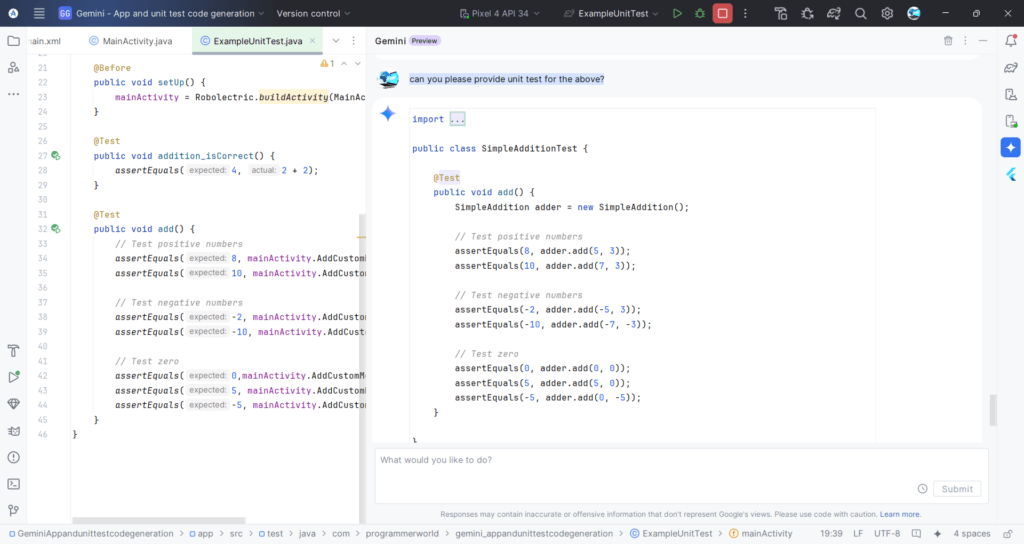
Project folder can be accessed through the following google drive link on payment of USD 9:
https://drive.google.com/file/d/1dpSjaLnvlL9RciXhu_L1XK04eJKQwz1g/view?usp=drive_link
Discover how to utilize the Gemini AI tool for Android app development with our comprehensive video tutorial. Learn to generate code and write Java unit tests efficiently. Access full source code, example unit tests, and additional resources. Ideal for developers looking to streamline their Android application and testing process.