In this video, it shows the steps to create java unit test for static methods of Android Studio Project.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code and other details/ steps of this video are posted in the below link:
MainActivity.java
package com.programmerworld.unittestsforstaticmethod;
import android.os.Bundle;
import android.widget.TextView;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
return insets;
});
TextView textView = findViewById(R.id.textView);
textView.setText(String.valueOf(staticAdditionMethod(1, 2)));
}
public static double staticAdditionMethod(double a, double b) {
return a + b;
}
}
ExampleUnitTest.java
package com.programmerworld.unittestsforstaticmethod;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* Example local unit test, which will execute on the development machine (host).
*
* @see <a href="http://d.android.com/tools/testing">Testing documentation</a>
*/
public class ExampleUnitTest {
private MainActivity activity;
@Test
public void addition_isCorrect() {
assertEquals(4, 2 + 2);
}
@Test
public void testStaticAdditionMethod(){
double result = activity.staticAdditionMethod(4,5);
assertEquals(9,result,0.0001);
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textSize="34sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Screenshots:
App running in emulator:
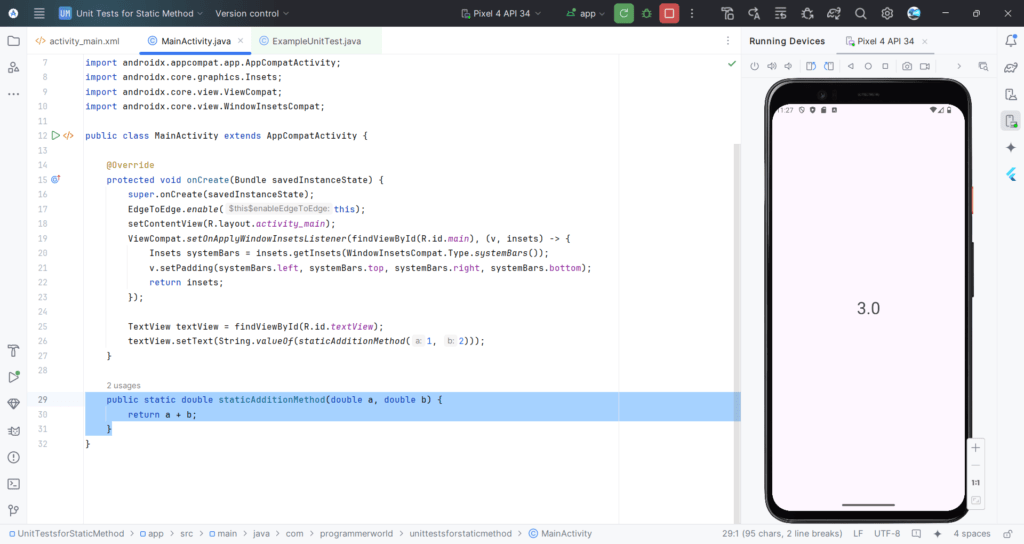
Running the Unit Tests:
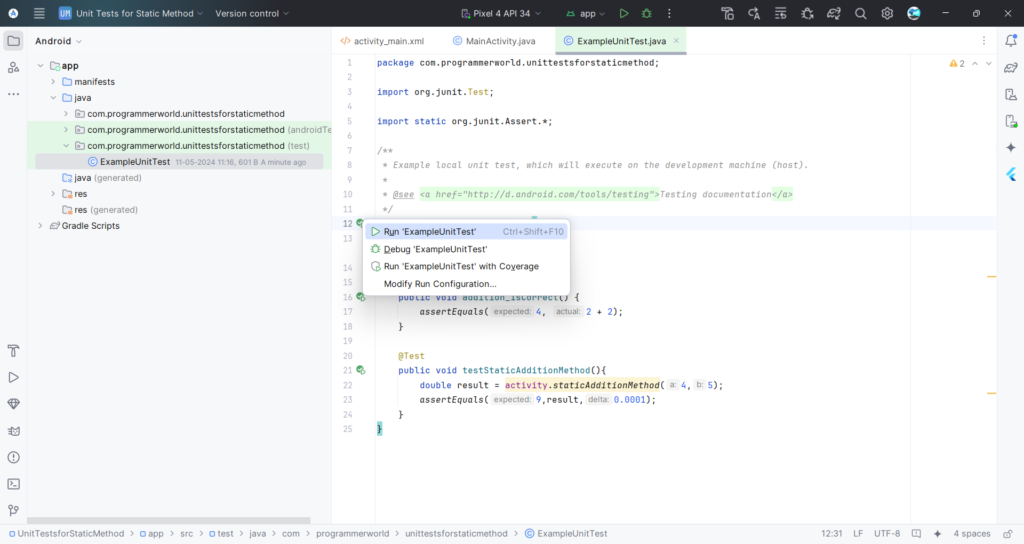
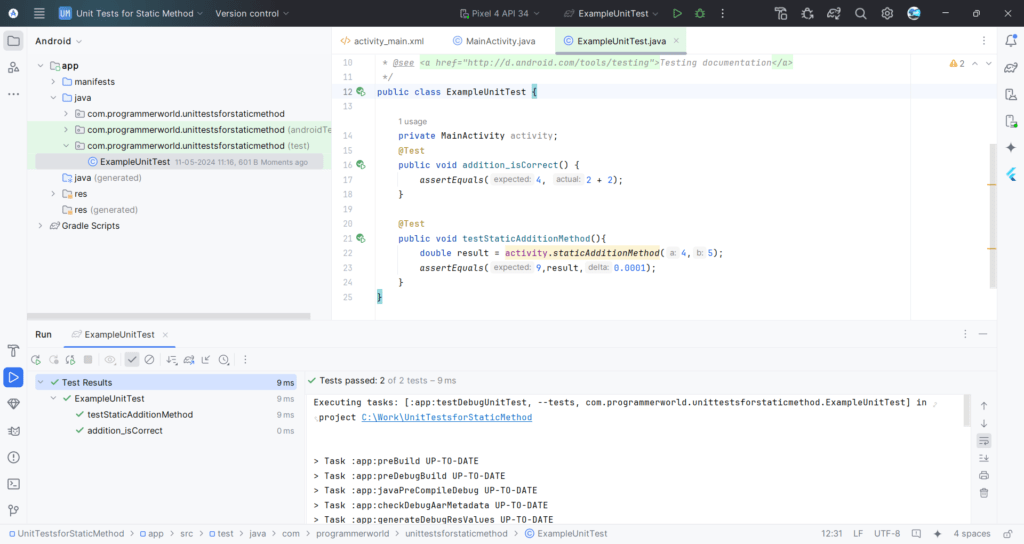
Exporting the test results:
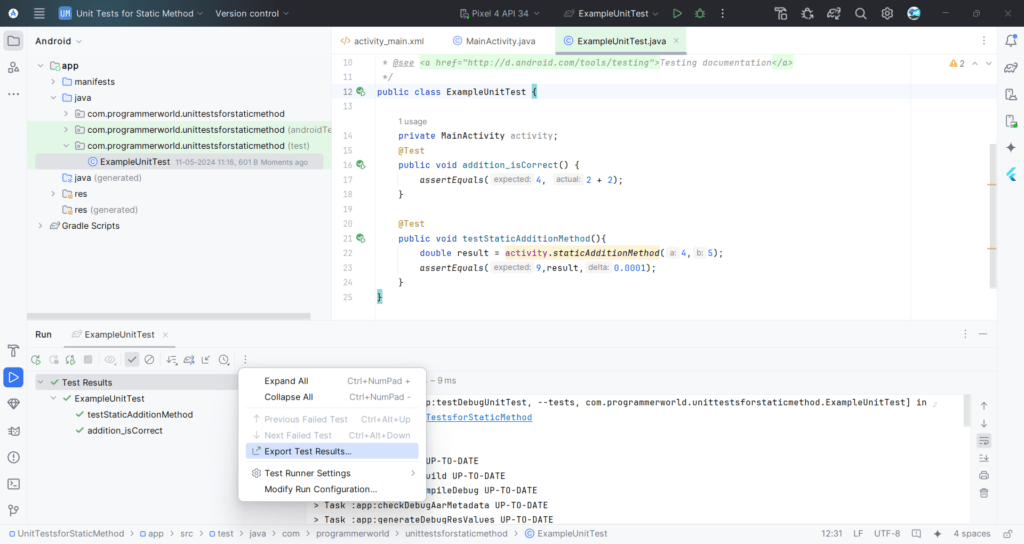
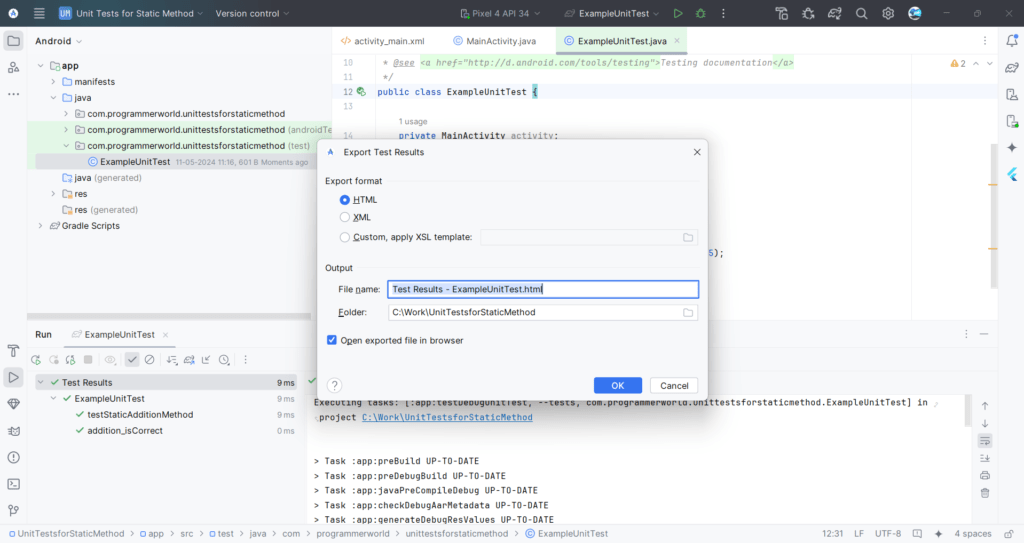
Test Results:
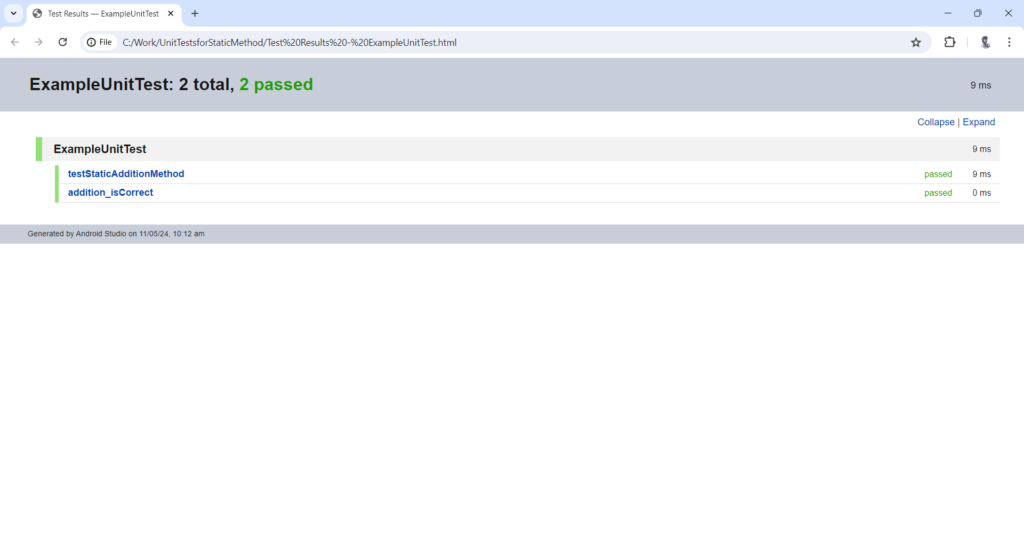
Test Results file:
Project folder shown in this tutorial can be accessed from the below google drive link on payment of USD 9:
https://drive.google.com/file/d/1loIt2kyLhKgILjuitQpM7CUTmP3i4SnJ/view?usp=drive_link
Creating a Java unit test for static methods in an Android Studio Project is straightforward and efficient. This detailed guide includes example code, a video tutorial, related screenshots, and links to the complete source code to facilitate understanding and application in your own Android development environment. Perfect for developers looking to enhance their testing skills in Android apps.