In this video it shows how to create/ develop a Java program in Eclipse. It develops a counter Java program which will keep increasing the count every 500 milliseconds until the user presses the ENTER key from key board.
It runs the program both in Eclipse environment terminal and also in windows command prompt after building the respective jar file.
This functionality can be useful for developing java code which needs to continuously run for a longer duration.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Complete source code:
import java.util.Scanner;
public class InfiniteCounter implements Runnable {
volatile boolean booleanRun = true;
public InfiniteCounter() {
// TODO Auto-generated constructor stub
}
public static void main(String[] args) {
// TODO Auto-generated method stub
InfiniteCounter infiniteCounter = new InfiniteCounter();
Thread thread = new Thread(infiniteCounter);
thread.start();
Scanner scanner = new Scanner(System.in);
while(!scanner.hasNextLine());
infiniteCounter.booleanRun = false;
thread.interrupt();
}
@Override
public void run() {
// TODO Auto-generated method stub
int i = 1;
System.out.println("Starting the Counter ...");
while(booleanRun) {
System.out.println(i++);
try {
Thread.sleep(500);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
// e.printStackTrace();
}
}
System.out.println("Counter Stopped ...");
}
}
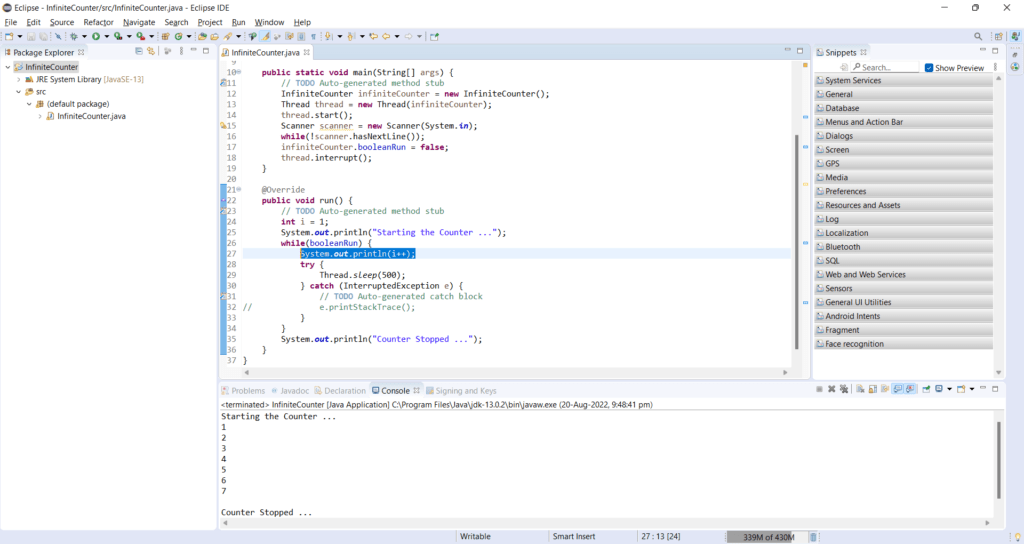
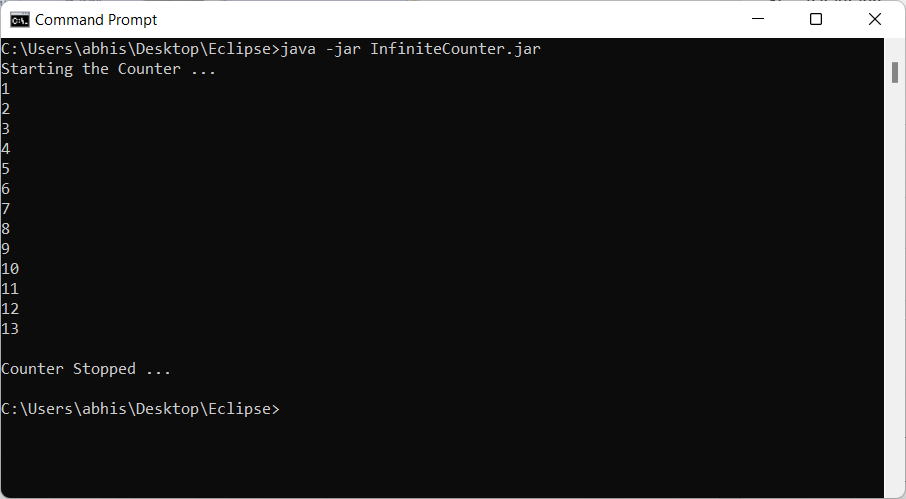