This video shows the steps to create an iOS App in which one can check whether the provided word is a Palindrome or not.
It uses a text field to get the input from the user to check whether a word is Palindrome or not.
It invokes the method (function) to check Palindrome using the UIButton event.
The App finally displays the result in a label in the App.
To check or validate whether the word is Palindrome it uses a very simple logic. It checks every character of the word from left side and right side one by one in a for loop. If all the characters match then it means it is Palindrome otherwise it can be inferred that it is not Palindrome.
This App is just for demonstration purpose that how a simple logic of Palindrome checking can be implemented for the iOS App.
I hope you like this video. For any questions, suggestions or appreciation please contact us at: https://programmerworld.co/contact/ or email at: programmerworld1990@gmail.com
Source Code
//
// ViewController.swift
// Palindrome App
//
// Created by HomePC on 16/12/20.
// Copyright © 2020 HomePC. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var uiTextField: UITextField!
@IBOutlet weak var uiLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func isPalindrome(Sender: UIButton){
let wordInput : String = uiTextField.text!
let lengthWord = wordInput.count
var varBoolean = true
for var i in 0..<lengthWord/2{
var firstCharacter = wordInput[(wordInput.index(wordInput.startIndex, offsetBy: i))]
var secondCharacter = wordInput[(wordInput.index(wordInput.startIndex, offsetBy: lengthWord-1-i))]
if(firstCharacter != secondCharacter){
varBoolean=false
break
}
}
if varBoolean {
uiLabel.text="Palindrome"
}
else{
uiLabel.text="Not Palindrome"
}
}
}
Screenshots:
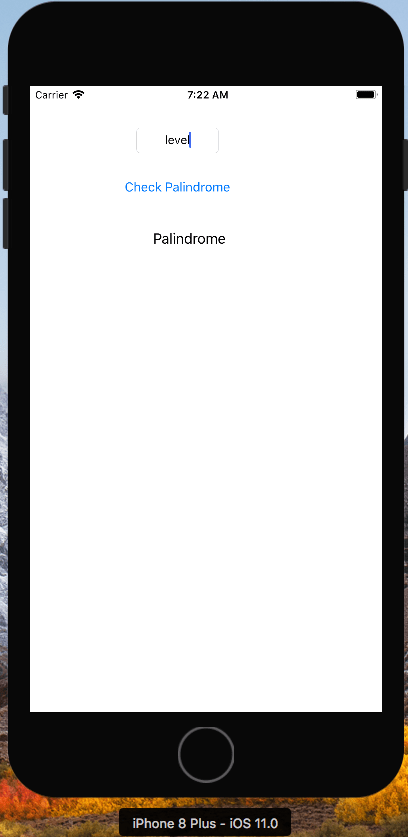
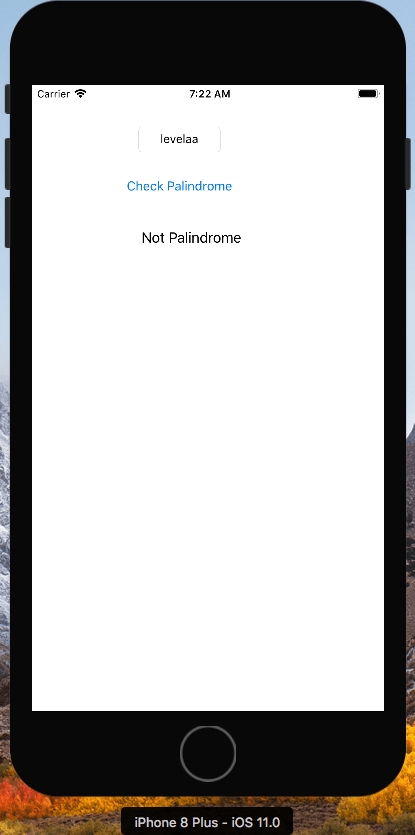
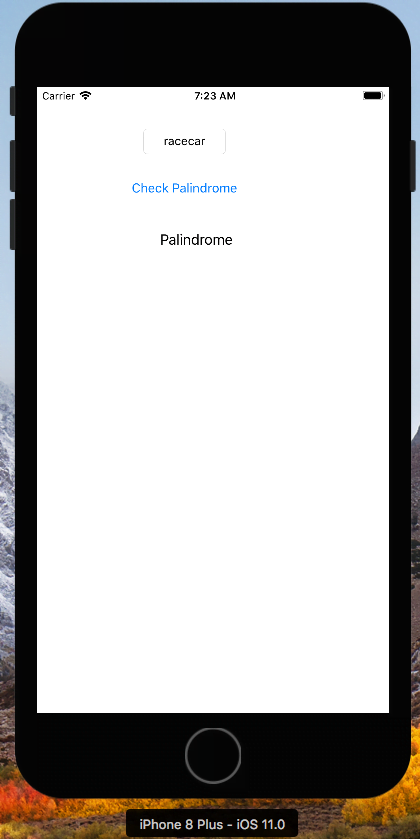